Description
- Let’s build Telegram Temperature Alert System using ESP32.
- We will use the DS18B20 temperature sensor as an example. It is a digital temperature sensor that measures temperature in the range of -55°C to 125°C
What is Telegram?
- Similar to WhatsApp, Telegram is a cloud-based instant messaging platform and communication app.
- It allows users to send and receive messages, make voice and video calls, and share photos, videos, documents, and other files.
- Telegram is available for smartphones running various operating systems, including iOS, Android, and Windows.
BotFather Bot
- Before we start, need to know we can not send messages directly to Telegram we need an API that acts as a mediator between esp32 and Telegram to send the message.
- So we will take the help of BotFather which is a Bot available on Telegram that manages all the bots that you create via your account on Telegram to send or receive the from your program or scripts.
- To know more about BotFather visit the below link
- https://core.telegram.org/bots/tutorial
Now Let’s start
Step 1
Open telegram and find BotFather
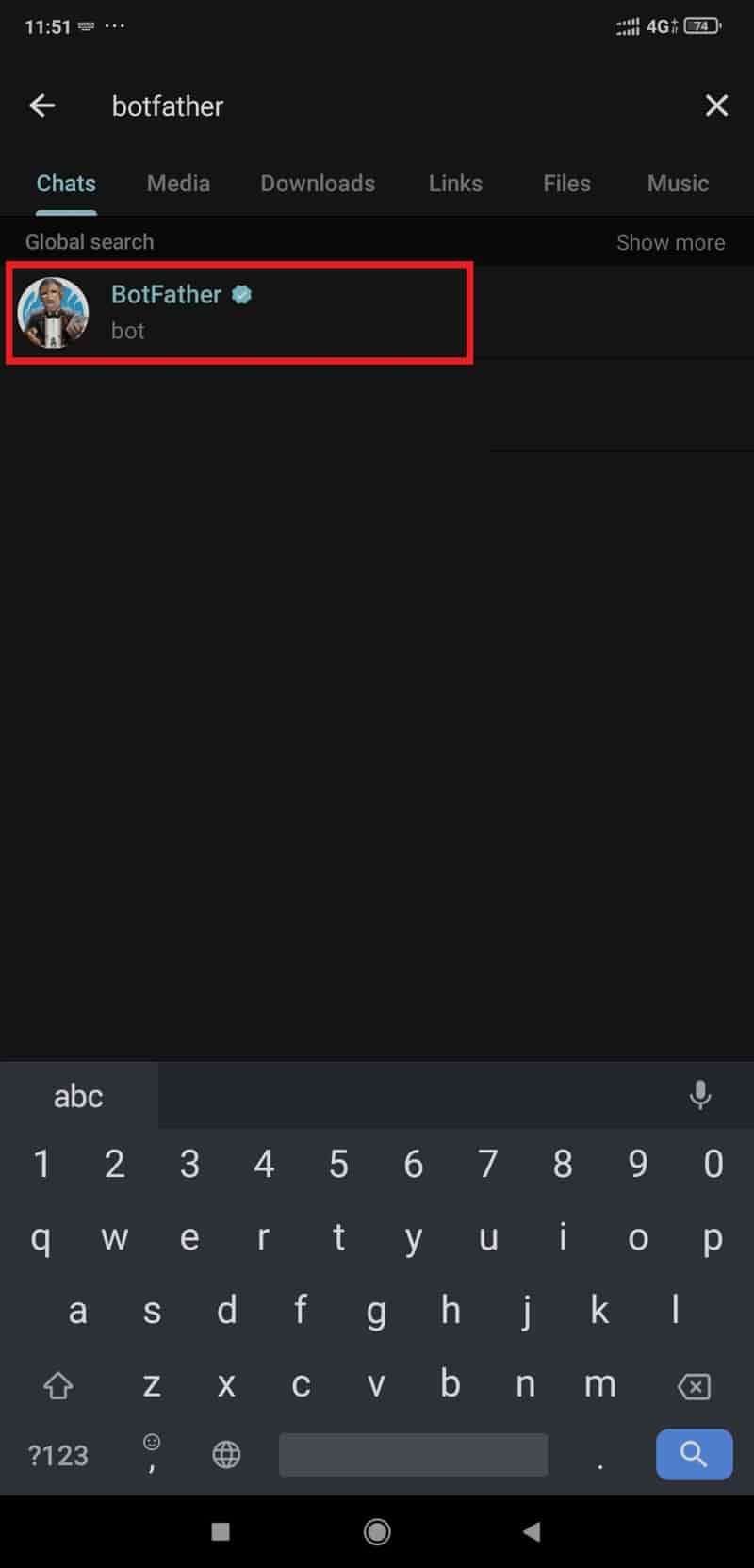
Now find the Botfather in the result and open it and click ok start.
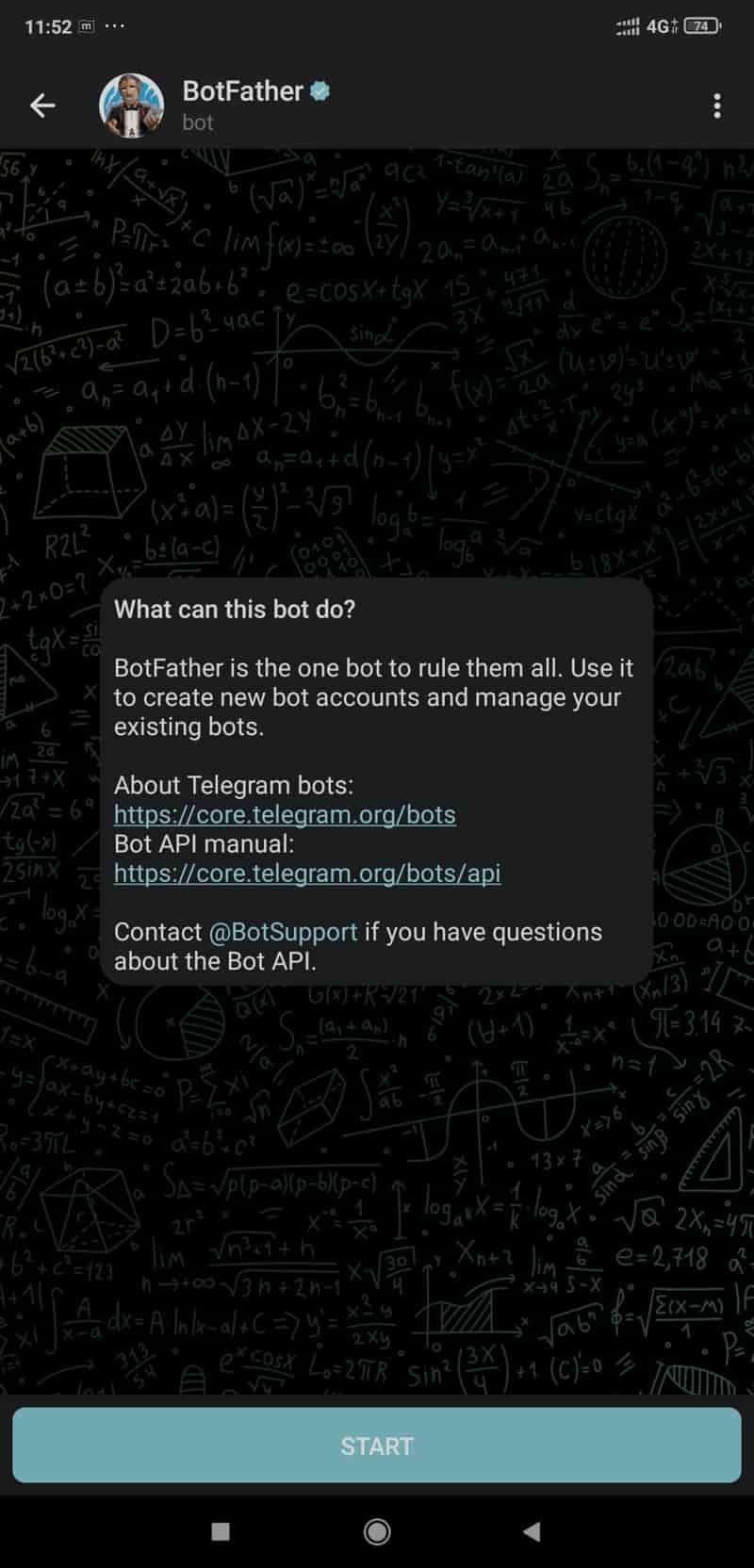
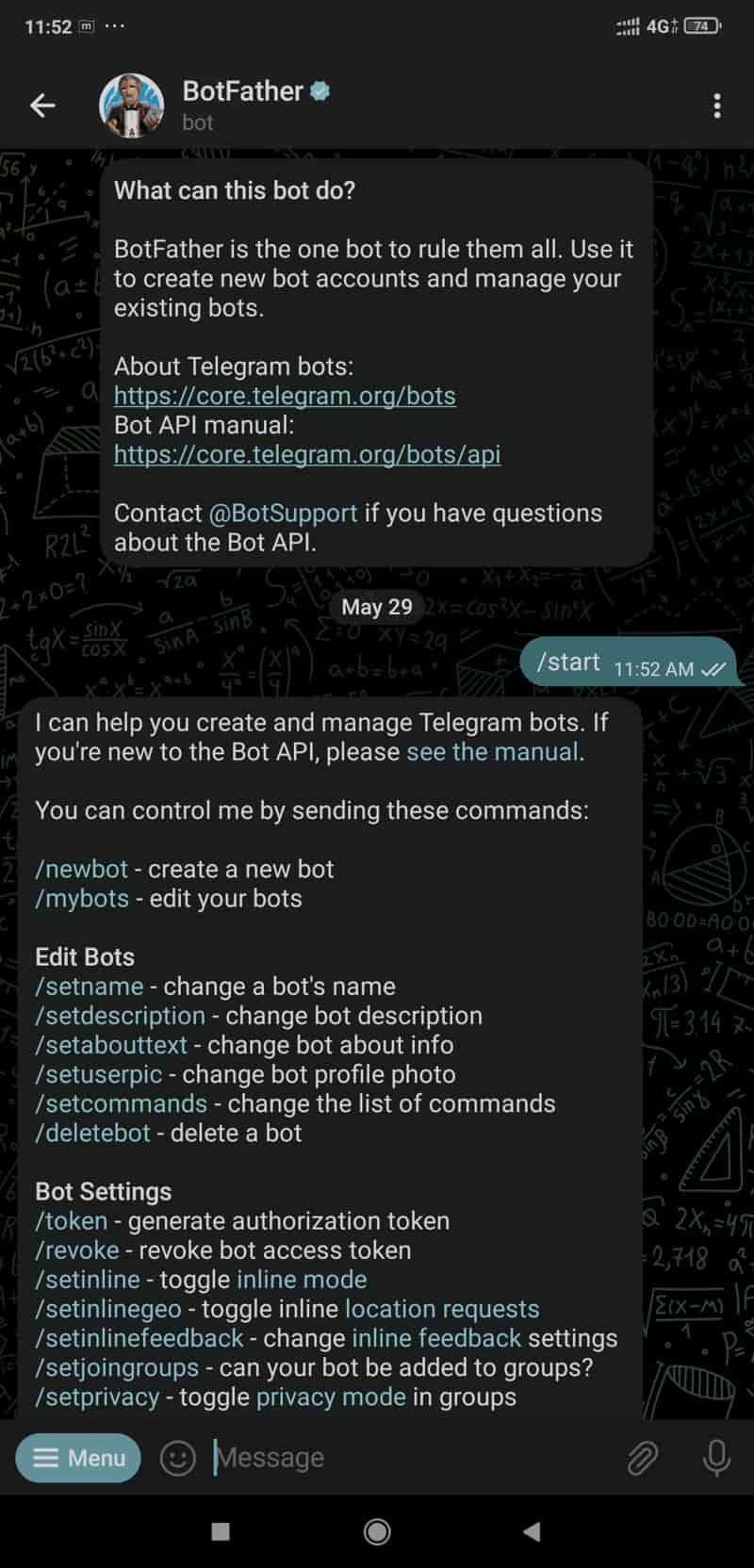
Now type /newbot and press enter.
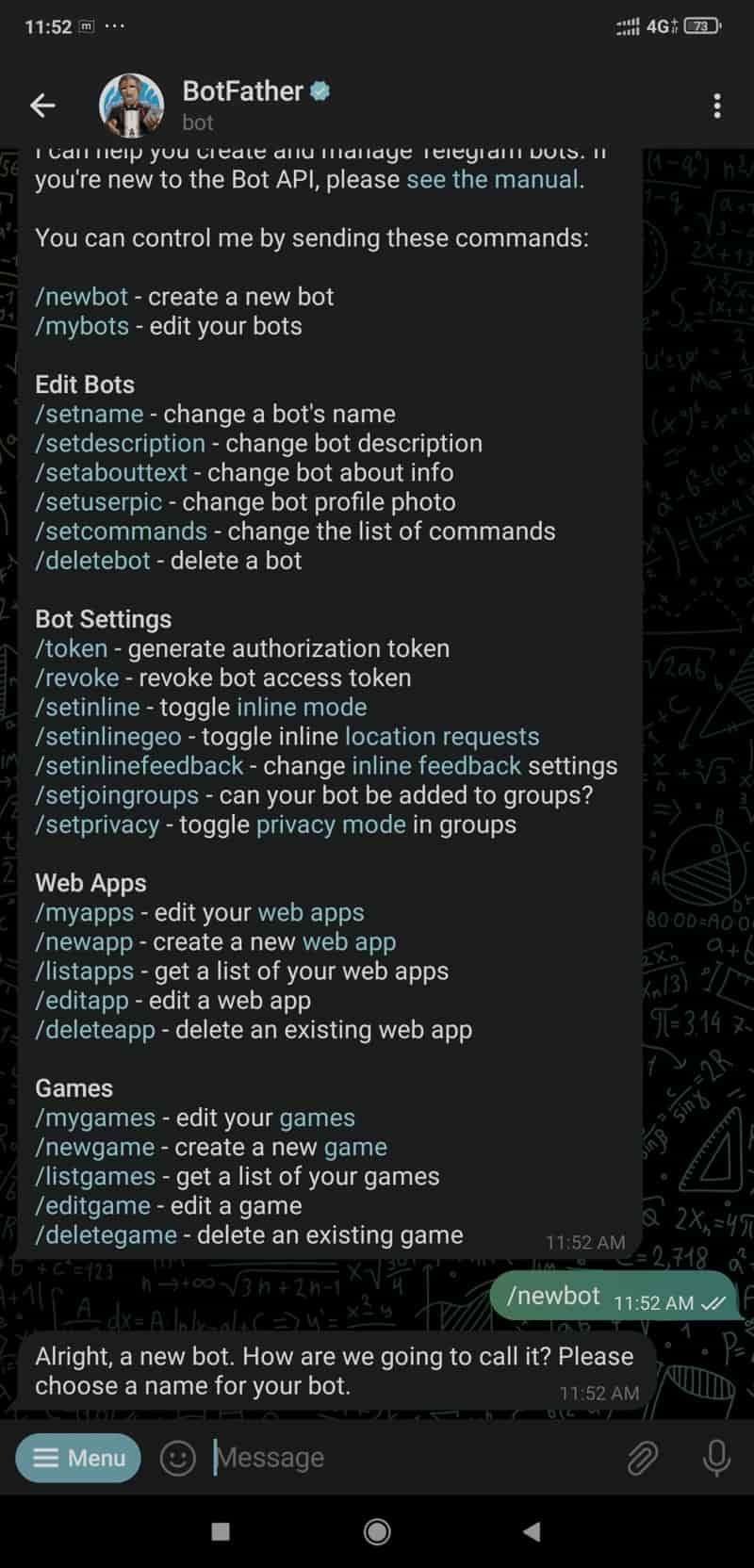
Now set the username for your bot.
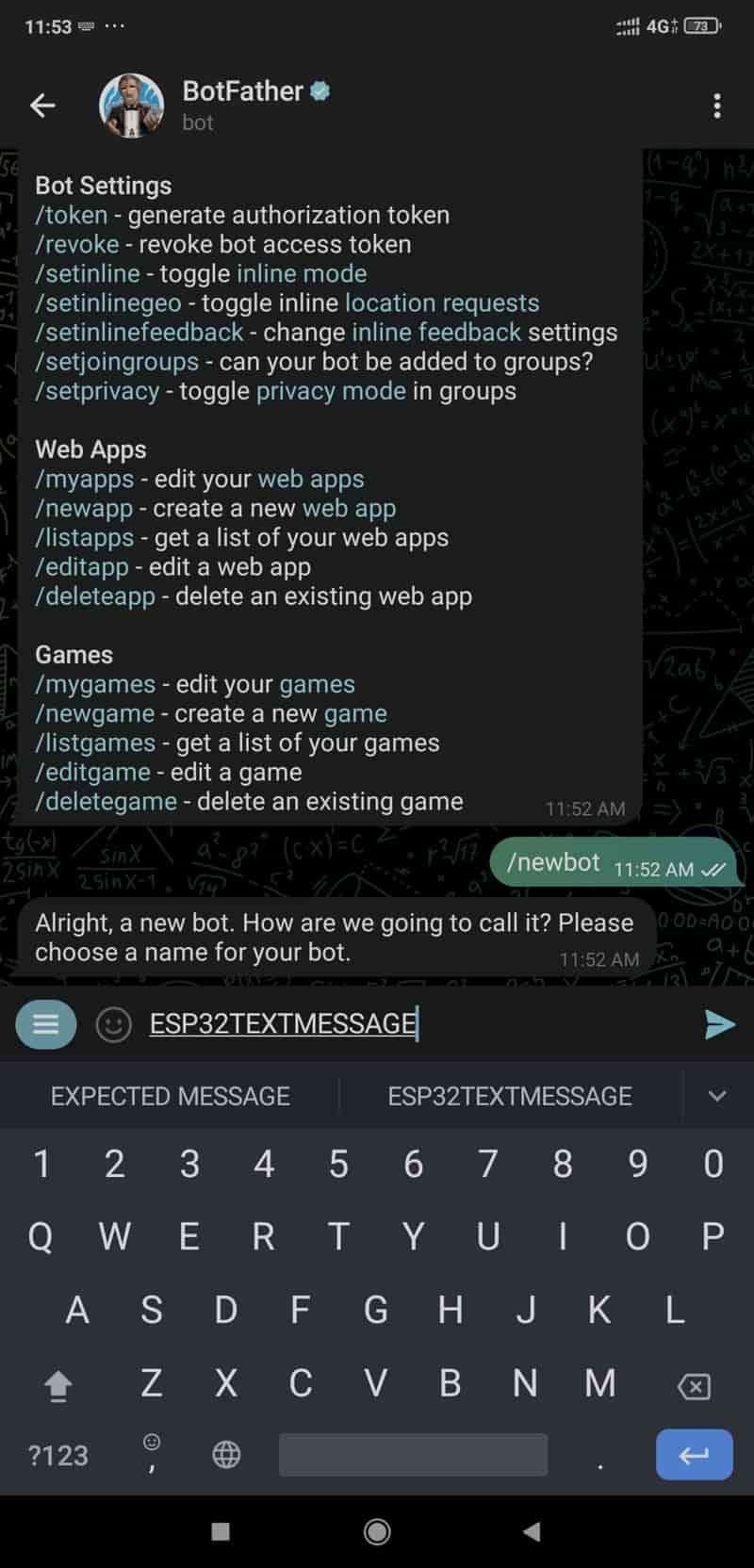
Now set the username for your bot (it must me end with bot)
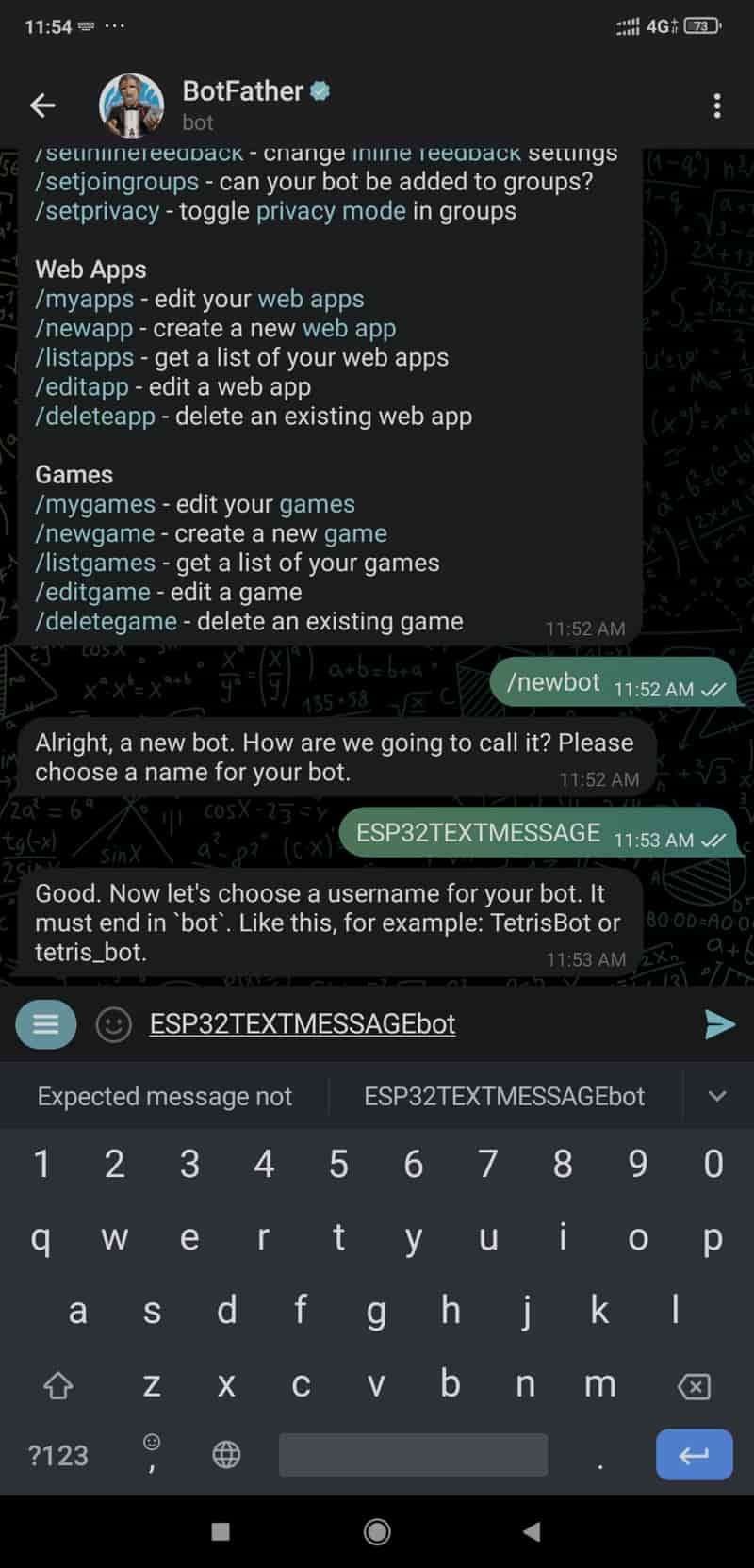
Now search IDBot in search and open it and click on start.
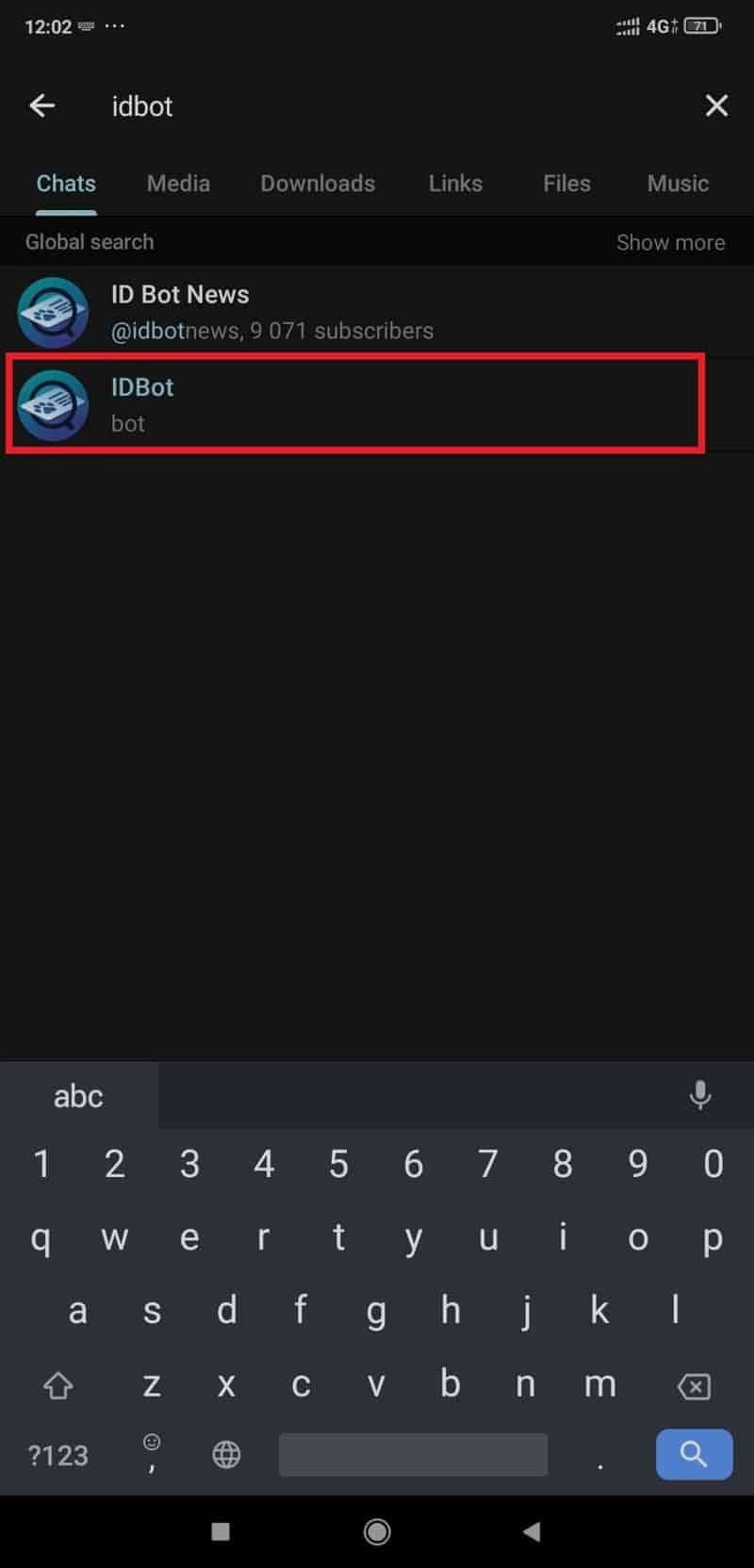
The bot will return the ID after you click on start as shown in below.
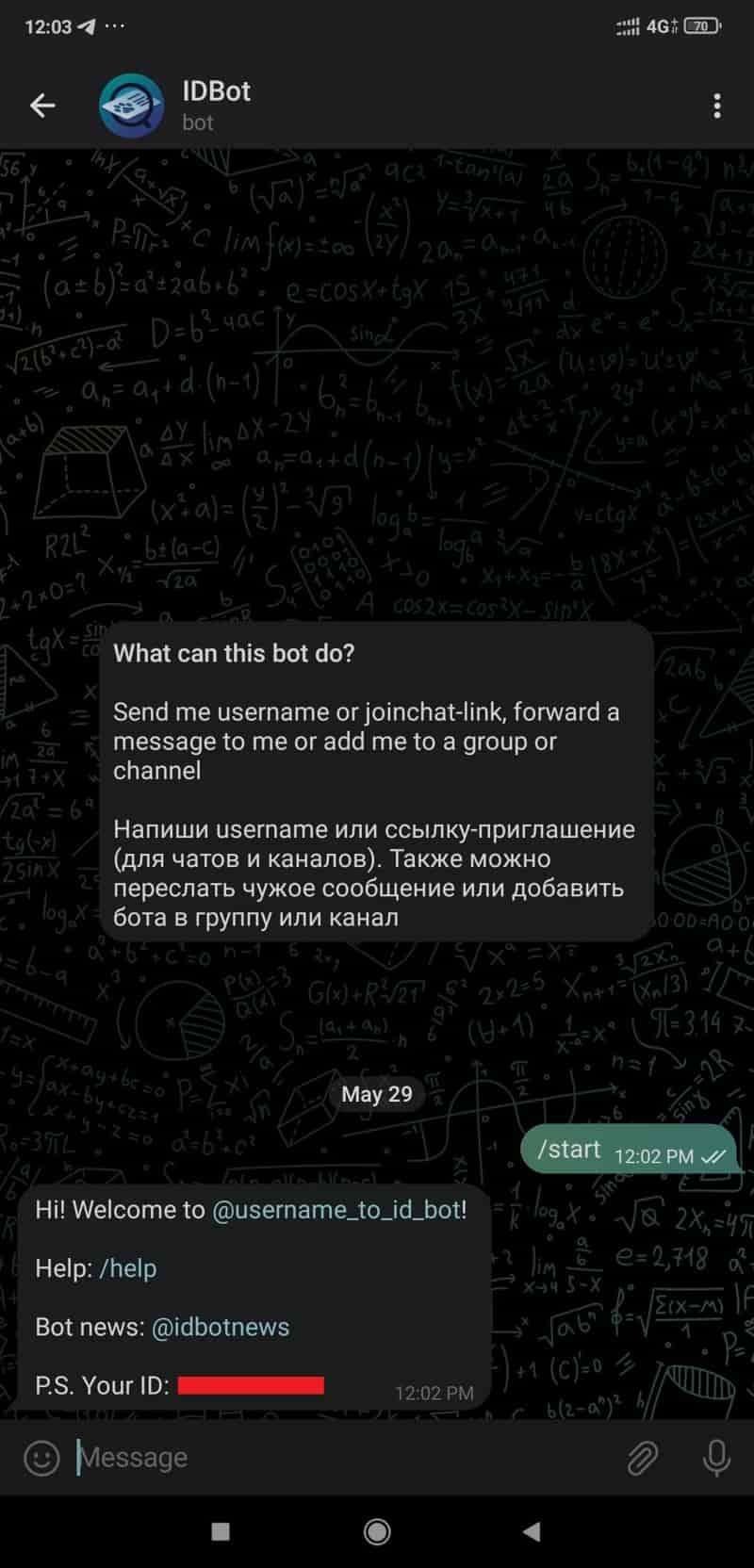
DS18B20 Interfacing Diagram with ESP32
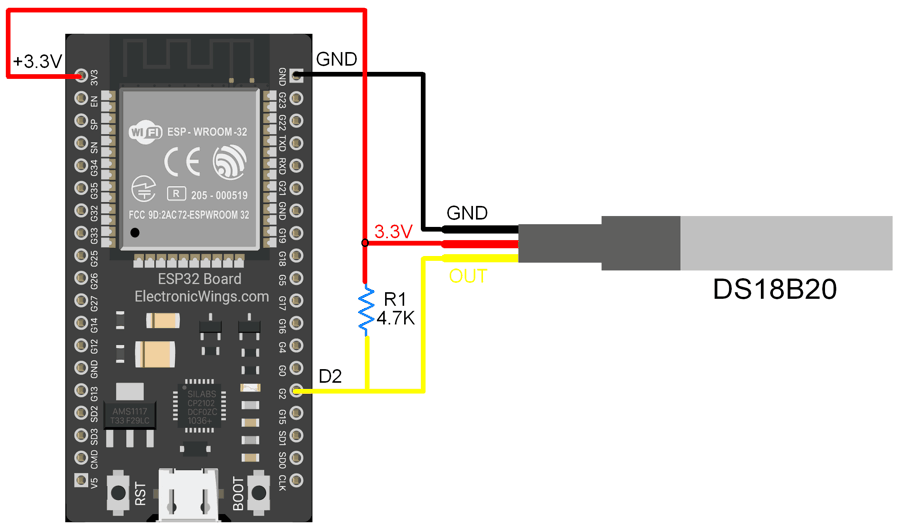
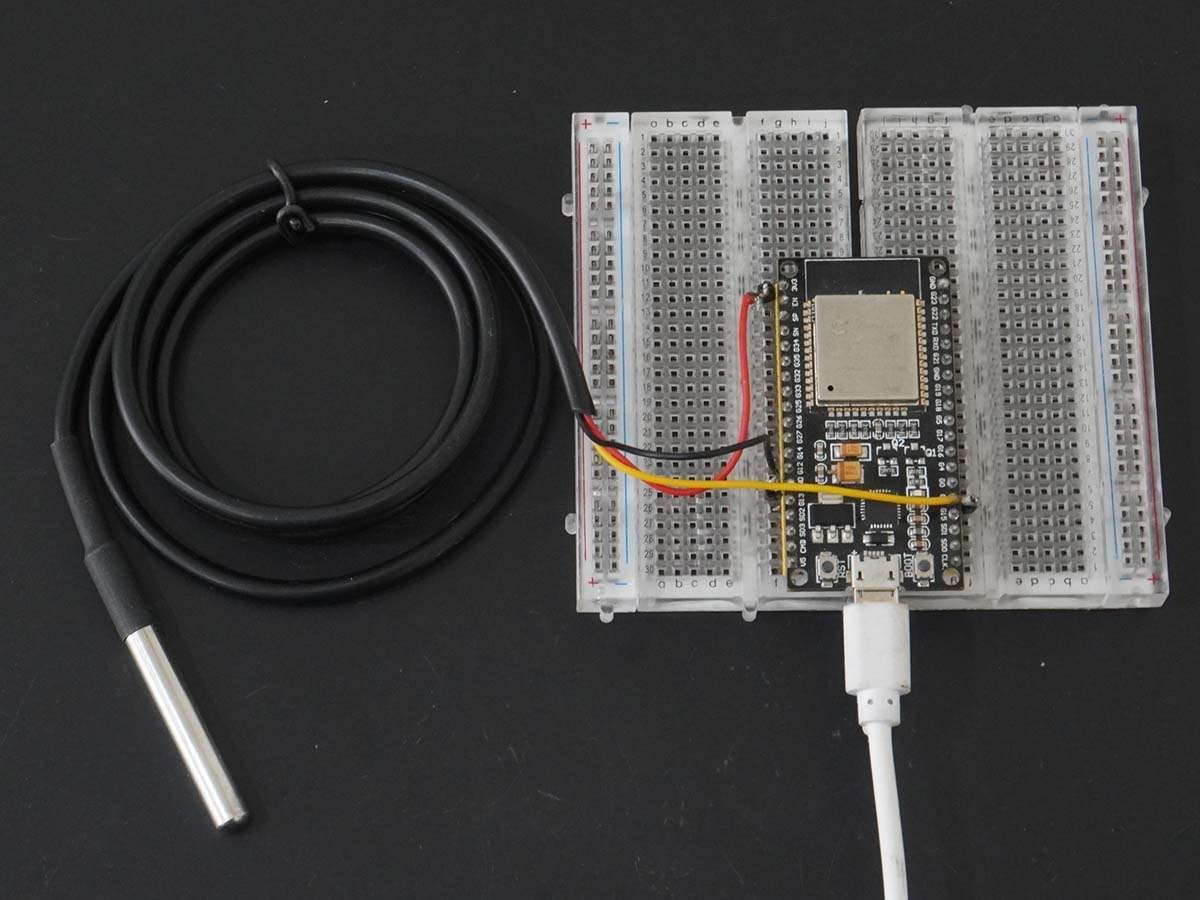
Send the temperature alert on telegram using ESP32
Here, we will be using witnessmenow’s Universal-Arduino-Telegram-Bot library from GitHub.
https://github.com/witnessmenow/Universal-Arduino-Telegram-Bot
Extract the library and add it to the libraries folder path of Arduino IDE.
For information about how to add a custom library to the Arduino IDE and use examples from it, refer Adding Library To Arduino IDE in the Basics section.
Now add another library for the DS18B20 temperature sensor
Here we are using DallasTemperature libraries for the above example. We need to install the DallasTemperature library using the Arduino Library Manager.
- Open the Arduino IDE
- Navigate to Sketch ► Include Library ► Manage Libraries…
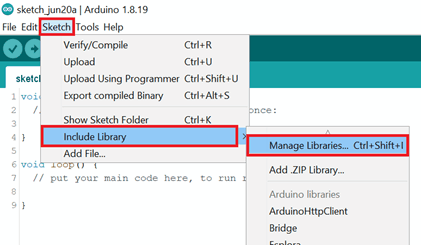
- The library Manager window will pop up. Now enter DS18B20 into the search box, and click Install on the DallasTemperature option to install version 3.9.0 or higher. As shown below image.
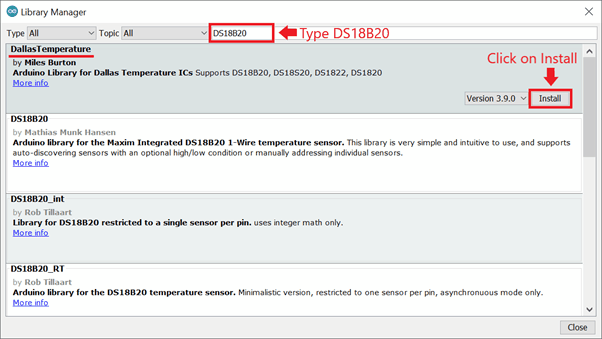
- If you don’t have the OneWire library, then this pop-up will come then click on Install all.
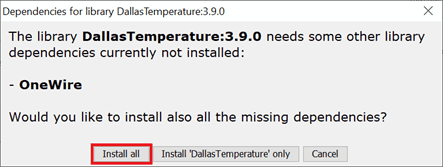
Send Alert to Telegram using ESP32
Let’s send the Hello World! Message to the telegram using the ESP32 and Arduino IDE.
Before uploading the code make sure you have added your SSID, Password, Token ID, and Chat ID as follows.
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASS";
#define BOTtoken "XXXXXXXXXX:XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
#define CHAT_ID "XXXXXXXXXX"
Over Temperature Alert to Telegram code for ESP32
#include <WiFi.h>
#include <WiFiClientSecure.h>
#include <UniversalTelegramBot.h>
#include <OneWire.h>
#include <DallasTemperature.h>
const char* ssid = "SSID";
const char* password = "PASSWORD";
#define ONE_WIRE_BUS 2
#define BOTtoken "6058832500:AAFxF2RdfruZYoqROMDjGcvhh3GVXngJ1Rw"
#define CHAT_ID "1621685513"
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
WiFiClientSecure client;
UniversalTelegramBot bot(BOTtoken, client);
void setup() {
Serial.begin(115200);
pinMode (2, INPUT_PULLUP);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
client.setCACert(TELEGRAM_CERTIFICATE_ROOT);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
sensors.begin();
}
void loop() {
// call sensors.requestTemperatures() to issue a global temperature
// request to all devices on the bus
Serial.print("Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperatures
Serial.println("DONE");
// After we got the temperatures, we can print them here.
// We use the function ByIndex, and as an example get the temperature from the first sensor only.
float tempC = sensors.getTempCByIndex(0);
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
if(tempC > 30) {
String message = "Temperature is above the threshold!\n";
message += "Current temperature: " + String(tempC, 2) + " °C";
bot.sendMessage(CHAT_ID, message, "");
}
}
else
{
Serial.println("Error: Could not read temperature data");
}
delay(5000);
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
Output on Serial Monitor
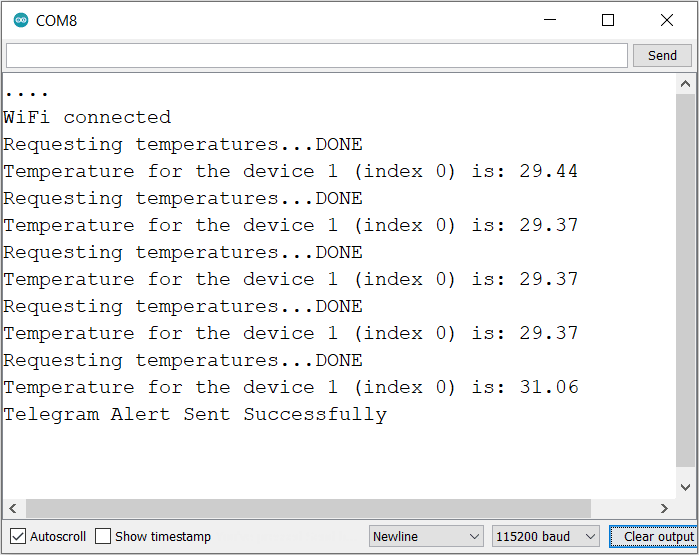
Output on Telegram
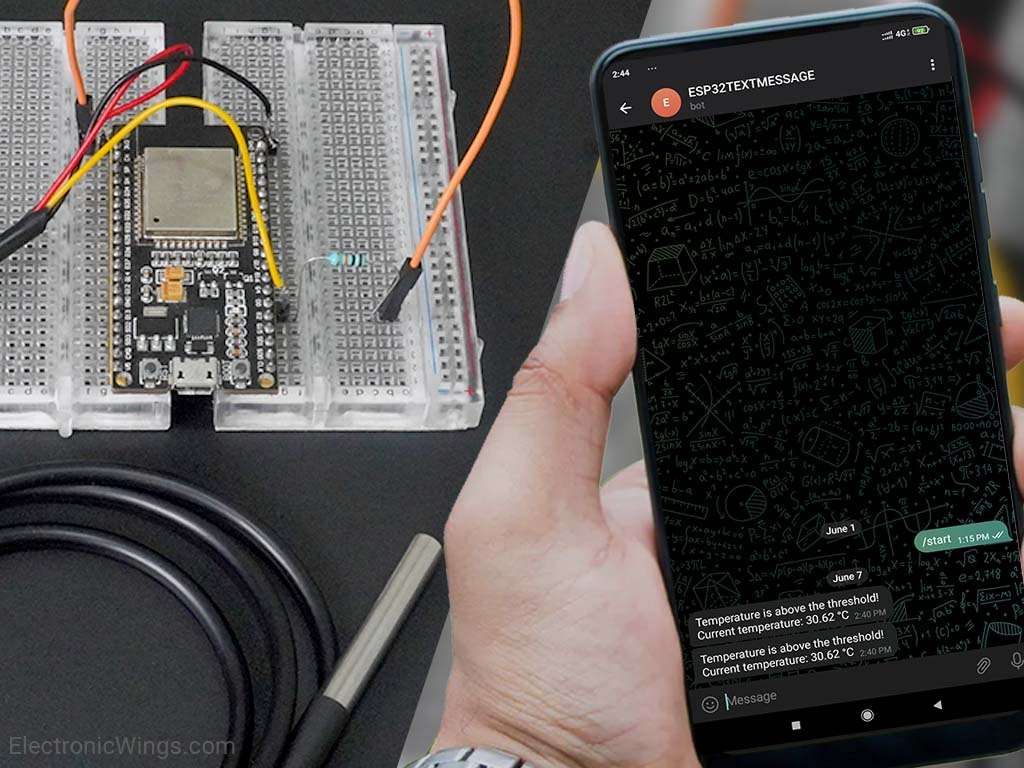
let’s understand the code
Add the required libraries
#include <WiFi.h>
#include <WiFiClientSecure.h>
#include <UniversalTelegramBot.h>
#include <OneWire.h>
#include <DallasTemperature.h>
Add your wifi credentials to connect ESP32 to wifi
const char* ssid = "Your SSID";
const char* password = "Your PASS";
set your bot token ID and Chat ID as given in telegram setup
#define BOTtoken "XXXXXXXXXX:XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
#define CHAT_ID "XXXXXXXXXX"
Set the pin number 2 for DS1307 of ESP32
#define ONE_WIRE_BUS 2
Setup a oneWire
instance to communicate with any OneWire devices
OneWire oneWire(ONE_WIRE_BUS);
Pass oneWire
reference to DallasTemperature.
DallasTemperature sensors(&oneWire);
Set the baud rate to 115200
Serial.begin(115200);
In the setup function
first, we set the WiFi as an STA mode and connect to the given SSID and password
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
client.setCACert(TELEGRAM_CERTIFICATE_ROOT);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
In loop function
Call sensors.requestTemperatures()
to issue a global temperature request to all devices on the bus
sensors.requestTemperatures(); // Send the command to get temperatures
After getting the temperature, we saved it on the tempC
float variable.
Here we have connected only one temperature sensor if you have multiple sensors then add in ByIndex function.
float tempC = sensors.getTempCByIndex(0);
Now check the received temperature status reading and print on the serial monitor if the temperature gove above 30 degree the send the notification on telegram.
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
if(tempC > 30) {
String message = "Temperature is above the threshold!\n";
message += "Current temperature: " + String(tempC, 2) + " °C";
bot.sendMessage(CHAT_ID, message, "");
}
}
else
{
Serial.println("Error: Could not read temperature data");
}
Wait for 5 seconds for next reading
delay(5000);
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 | |
DS18B20 Waterproof temperature sensor DS18B20 Waterproof temperature sensor |
X 1 |
Downloads |
||
---|---|---|
|
ESP32_telegram_alert | Download |