Overview of LM35
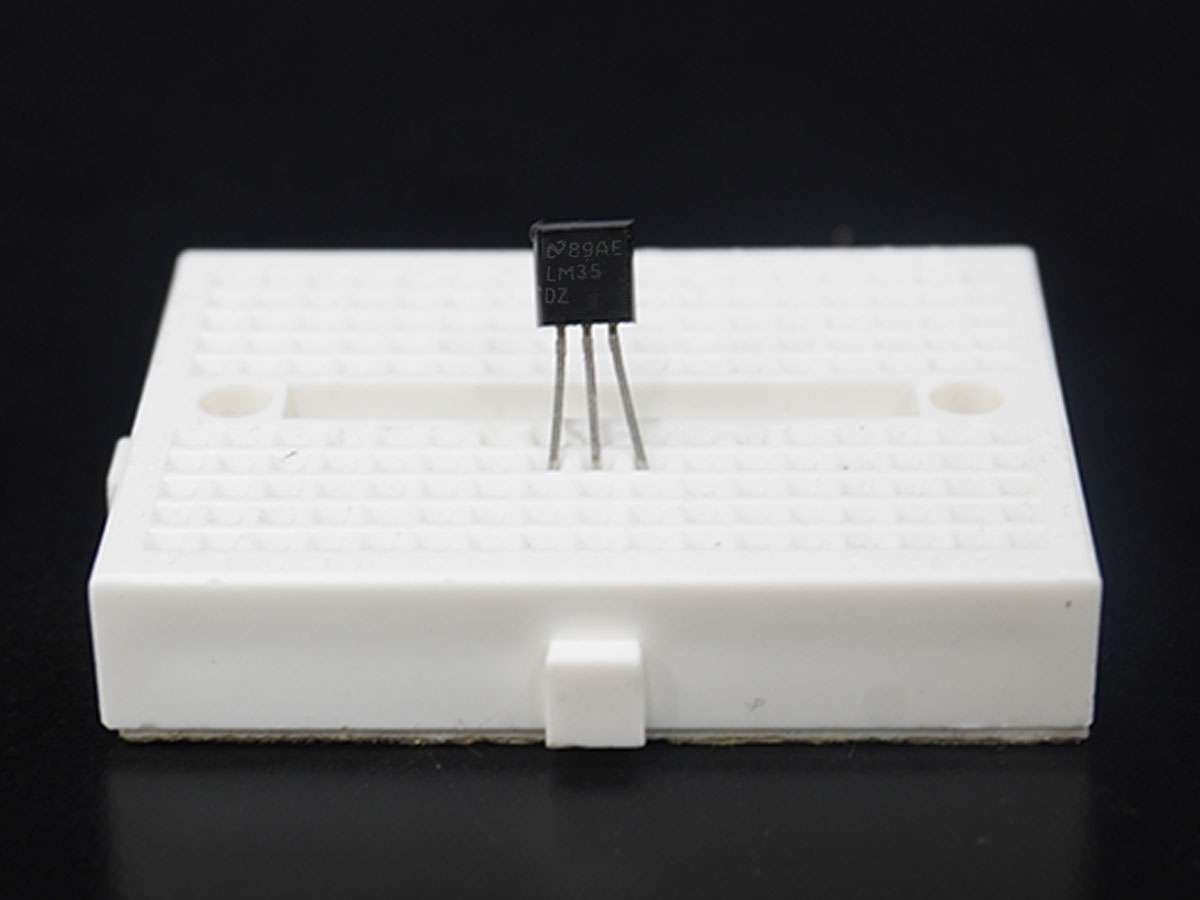
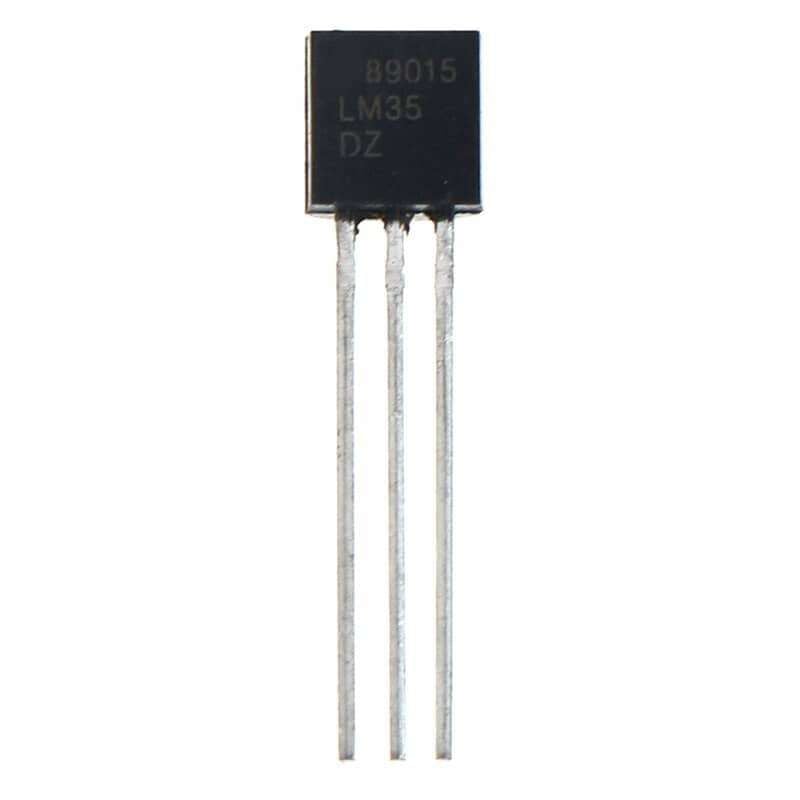
- LM35 is a temperature sensor that can measure temperature in the range of -55°C to 150°C.
- It is a 3-terminal device that provides an analog voltage proportional to the temperature. The higher temperature gives a higher output voltage.
- The output analog voltage can be converted to digital form using ADC so that a microcontroller can process it.
- LM35 drains less than 60-μA current.
LM35 Pinout
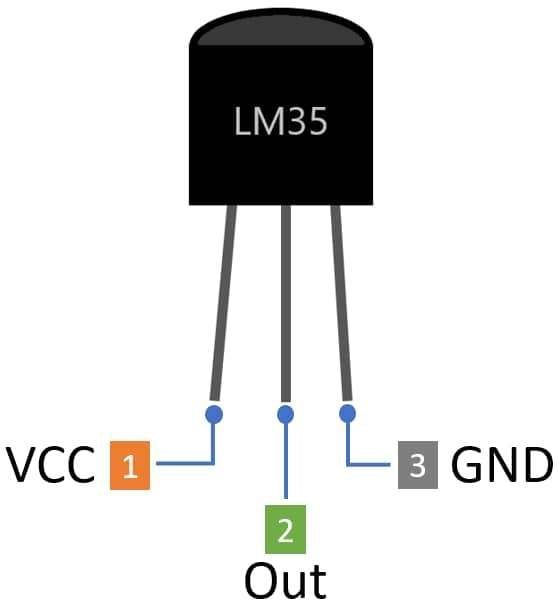
VCC: Supply Voltage in the range of 4V – 30V
Out: It gives analog output voltage which is proportional to the temperature (in degree Celsius).
GND: Supply Ground
LM35 Sensor Application Setup:
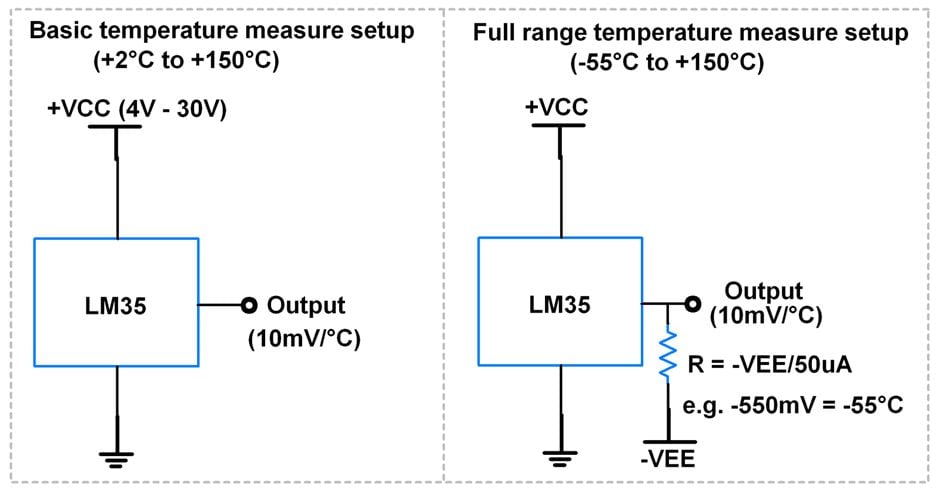
We have to consider that LM35 gives the voltage per Celsius i.e., 10mV/`c.
Accordingly, we can convert the ADC value into a temperature reading.
For more information about LM35 and how to use it, refer to the topic LM35 Temperature Sensor in the sensors and modules section.
Connection Diagram of LM35 with ESP32
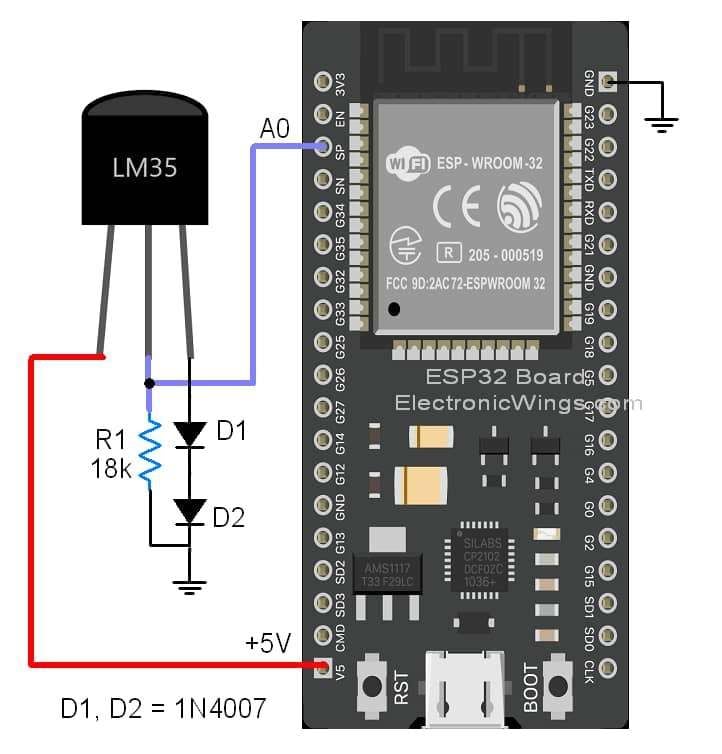
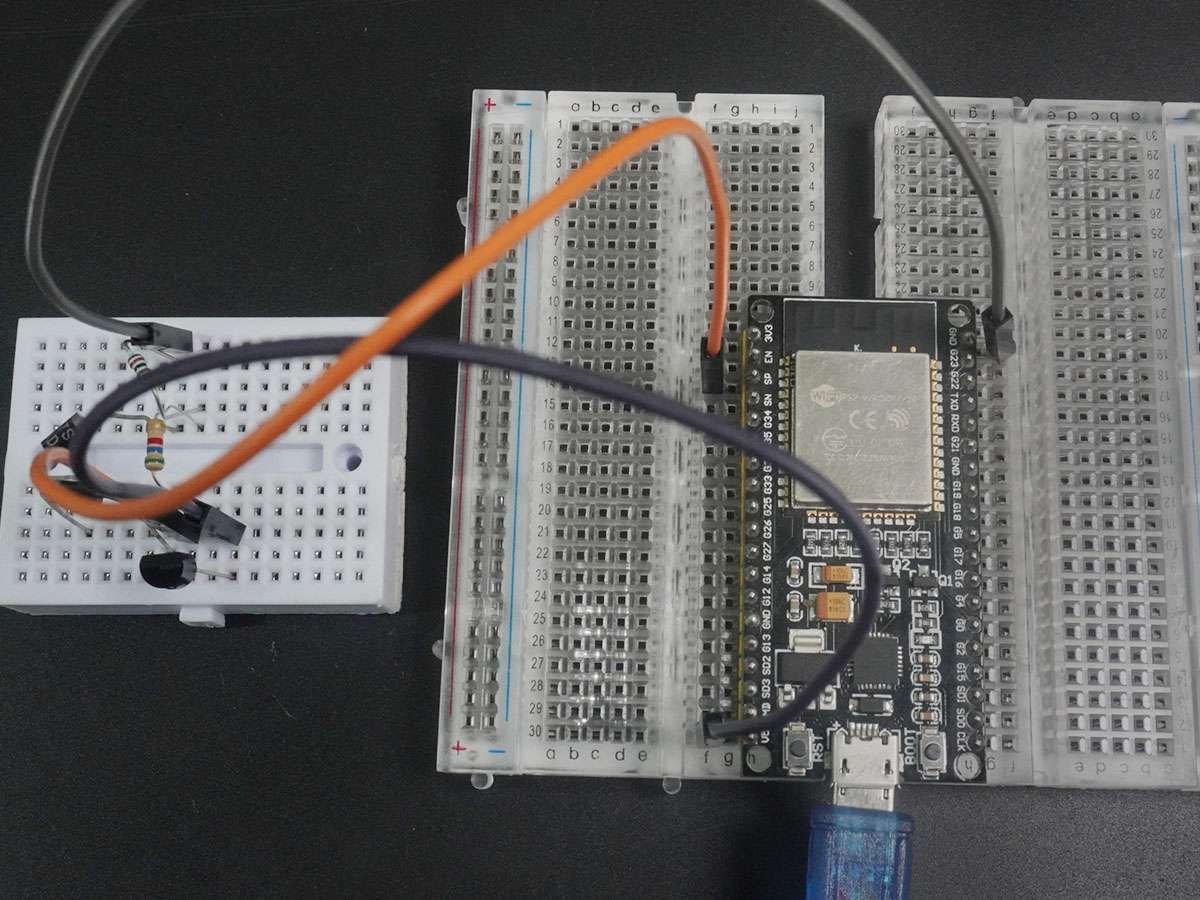
Measure Temperature using LM35 and ESP32
Let’s take a simple example to interface the LM35 temperature sensor and monitor the room temperature and display it on the serial window.
Wait before moving forward we need to know the issue of ESP32 ADC. Yes, there is one issue in ESP32 ADC. ESP32 ADC readings are not in a proper manner. Let’s see the observation in below.
ESP32 ADC Observation
As we know the ADC gives the linear response proportional to the input. But in the ESP32 we have observed it gives a non-linear response. Let’s check our lab report in short.
Here we have connected the setup as per the above interfacing diagram the extra tool is we have connected DSO to measure the voltage. Now when we decrease the voltage from 3.3 to 0 linearly when it comes to 3.21 Volt still ESP32 shows 4095 Reding at the serial monitor after 3.32 Volt is starts decreasing the ADC count. Shown in below diagram.
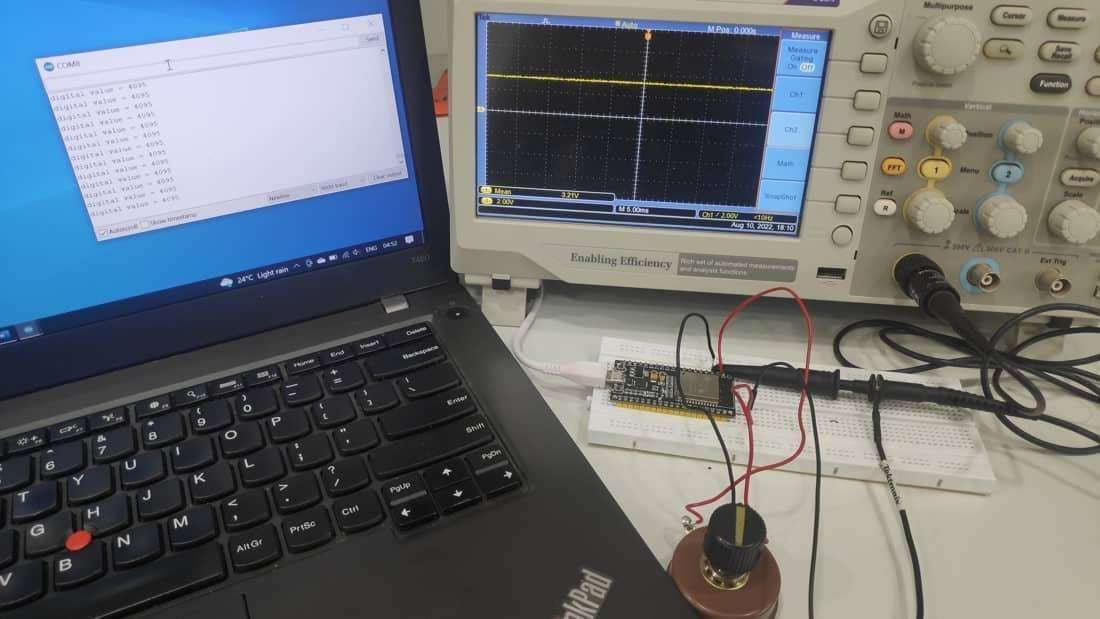
Same when we increase the voltage from 0 to 3.3 volt it shows 0 reading still voltage at 0.138 Volt. The response is shown in below diagram.
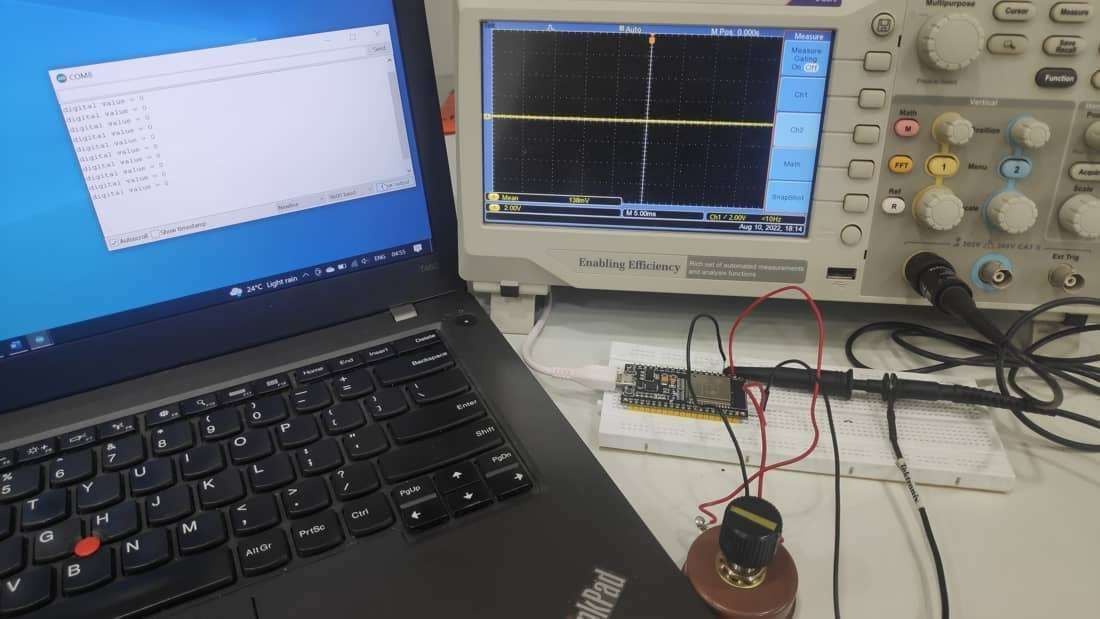
The responsive curve is shown in below chart.
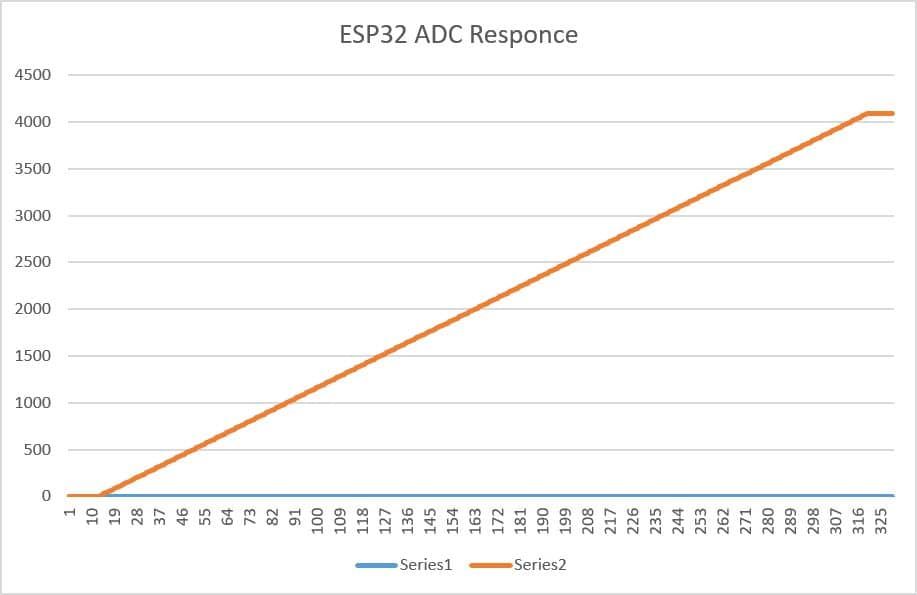
As we know the LM35 gives 10mV for 1°C which means as per nonresponsive ADC we can’t measure the temperature between 0°C to 14°C.
Solution
The solution is to shift the LM35 output voltage by reference voltage.
Let’s see in the below diagram
As per the datasheet, two diodes are connected between the ground and the LM35 ground pin.
Note: in this example, we have used the 1N4007 diode instead of the 1N914 diode.
The forward voltage drop for the 1N4007 diode is 0.6V but in practical, we are getting 0.4 VDC. In the diagram, two diodes are connected back-to-back so the total voltage drop is 0.8V.
It means we can use 0.8V as the reference voltage, (The output of LM35 is shifted by 0.8V) which gives to ESP32 ADC pin.
So, it is better to measure practical digital value at room temperature and subtract LM35 to get a 0°C reference digital value
Step to simplify calculating Ref digital value for 0°C
- Measure ESP32 ADC value at room temperature.
for example, room temperature is 30°C so the ADC value is 1252.
- Now subtract the LM35 Output value from 30°C i.e 300mV which is 372
0°C Voltage Ref Value = 1252 – 372
= 880
Let’s see how to calculate LM35 Temperature in code
Now we know 0°C Vref Digital value = 880
Now Subtract 880 digital value from LM35 reading output value and we have to divide it by digital value i.e., 10mV/°C which is 12.41 when 12-bit ADC is used
Simple Code to read temperature using LM35 with ESP32
/*
ESP32 LM35 temperature Sensor Code
http:://www.electronicwings.com
*/
#define vRef 3.30
#define ADC_Resolution 4095
#define LM35_Per_Degree_Volt 0.01
#define Zero_Deg_ADC_Value 879.00
const int lm35_pin = A0; /* Connect LM35 out pin to A0 of ESP32*/
float _temperature, temp_val, ADC_Per_Degree_Val;
int temp_adc_val;
void setup(void){
Serial.begin(9600);
ADC_Per_Degree_Val = (ADC_Resolution/vRef)*LM35_Per_Degree_Volt;
}
void loop(void){
for (int i = 0; i < 10; i++) {
temp_adc_val += analogRead(lm35_pin); /* Read ADC value */
delay(10);
}
temp_adc_val = temp_adc_val/10.0;
temp_adc_val = temp_adc_val - Zero_Deg_ADC_Value;
temp_adc_val=(temp_adc_val/ADC_Per_Degree_Val);
Serial.print("LM35 Temperature = ");
Serial.print(temp_adc_val); /* Print Temperature on the serial window */
Serial.print("°C\n");
delay(100);
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
- After uploading the code open the serial monitor and set the baud rate to 115200 to see the output.
ESP32 serial monitor output for LM35
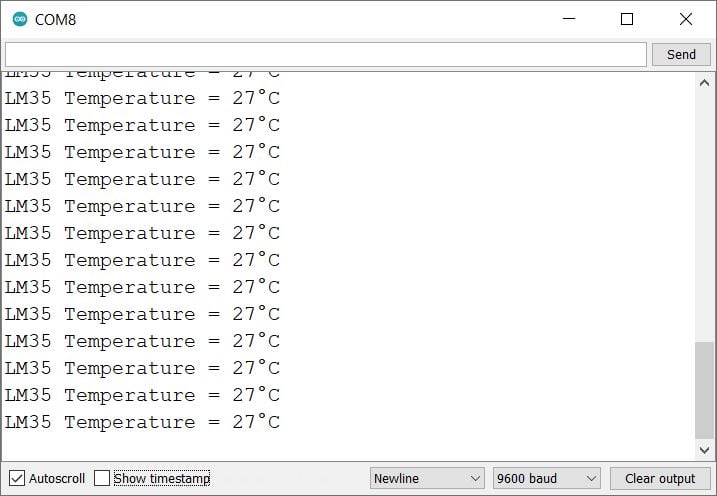
Let’s understand the code
Define the ESP32 ADC parameter and LM35 parameter
#define vRef 3.30
#define ADC_Resolution 4095
#define LM35_Per_Degree_Volt 0.01
#define Zero_Deg_ADC_Value 879.00
Set the pin number for LM35 out and variables.
const int lm35_pin = A0;
float _temperature, temp_val, ADC_Per_Degree_Val;
int temp_adc_val;
In setup function
We have initiated the serial communication with a 9600 Baud rate and initialized
Serial.begin(9600);
ADC_Per_Degree_Val = (ADC_Resolution/vRef)*LM35_Per_Degree_Volt;
In loop function
Read the ADC Value and take the average of 10 numbers
for (int i = 0; i < 10; i++) {
temp_adc_val += analogRead(lm35_pin); /* Read ADC value */
delay(10);
}
temp_adc_val = temp_adc_val/10.0;
Now subtract the shifted voltage from the output reading i.e. 879
temp_adc_val = temp_adc_val - Zero_Deg_ADC_Value;
To get the actual temperature reading divide by the ADC value of 1°C (10mV)
temp_adc_val=(temp_adc_val/ADC_Per_Degree_Val);
Print the temperature on the serial monitor
Serial.print("LM35 Temperature = ");
Serial.print(temp_adc_val); /* Print Temperature on the serial window */
Serial.print("°C\n");
delay(100);
Measure Temperature using LM35 over ESP32 Web Server
ESP32 has oh-chip Wi-Fi, we can utilize it and monitor the readings over our smartphone, Laptop, or even smart TV. This can offer much more flexibility and real world applications.
We can use the ESP32 web server to monitor the Temperature.
Now let’s take another example to display the surrounding temperature on the webserver using WiFi.h and WebServer.h library of Arduino IDE.
Let’s display the same readings on the web server using the ESP32 and Arduino IDE.
Before uploading the code make sure you have added your SSID, and Password as follows.
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
Code to read temperature using LM35 over ESP32 Web Server
/*
ESP32 LM35 temperature Sensor Code
http:://www.electronicwings.com
*/
#include <WiFi.h>
#include <WebServer.h>
#include "html.h"
WebServer server(80);
#define vRef 3.30
#define ADC_Resolution 4095
#define LM35_Per_Degree_Volt 0.01
#define Zero_Deg_ADC_Value 879.00
const int lm35_pin = A0; /* Connect LM35 out pin to A0 of ESP32*/
float _temperature, temp_val, ADC_Per_Degree_Val;
int temp_adc_val;
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send the code to the web server*/
}
void Temp() {
String TempValue = String(temp_adc_val); //Convert it into string
server.send(200, "text/plane", TempValue); //Send updated temperature value to the web server
}
void setup(void){
Serial.begin(115200); /*Set the baudrate to 115200*/
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");}
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
server.on("/", MainPage); /*Display the Web/HTML Page*/
server.on("/readTemp", Temp); /*Display the updated Temperature and Humidity value*/
server.begin(); /*Start Server*/
delay(1000); /*Wait for 1000mS*/
ADC_Per_Degree_Val = (ADC_Resolution/vRef)*LM35_Per_Degree_Volt;
}
void loop(void){
for (int i = 0; i < 10; i++) {
temp_adc_val += analogRead(lm35_pin); /* Read ADC value */
delay(10);
}
temp_adc_val = temp_adc_val/10.0;
temp_adc_val = temp_adc_val - Zero_Deg_ADC_Value;
temp_adc_val=(temp_adc_val/ADC_Per_Degree_Val);
server.handleClient();
Serial.print("LM35 Temperature = ");
Serial.print(temp_adc_val); /* Print Temperature on the serial window */
Serial.print("°C\n");
delay(1000); /* Wait for 1000mS */
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
- After uploading the code open the serial monitor and set the baud rate to 115200 then reset the ESP32 board and check the IP address as shown in the below image
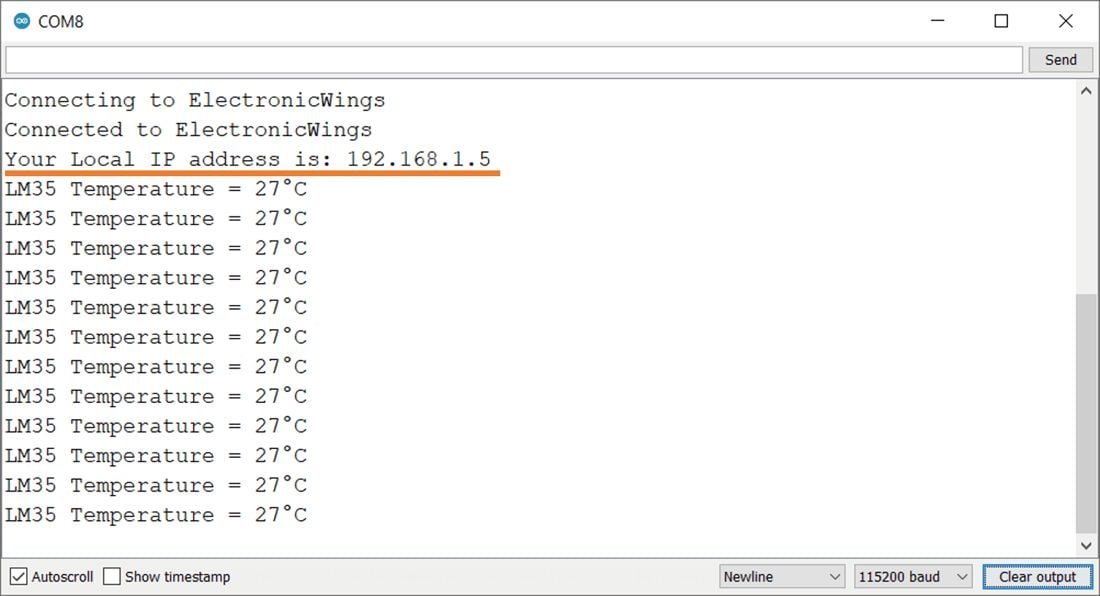
- Now open any mobile browser and type the IP address which is shown in the serial monitor and hit the enter button.
- If all are ok, then the web page will start the showing current temperature on the web server like in the below image.
Note: make sure your ESP32 and mobile are connected to the same router/server, if they are connected to the same router or server then only you will be able to visible the web page.
Final Output on the webserver
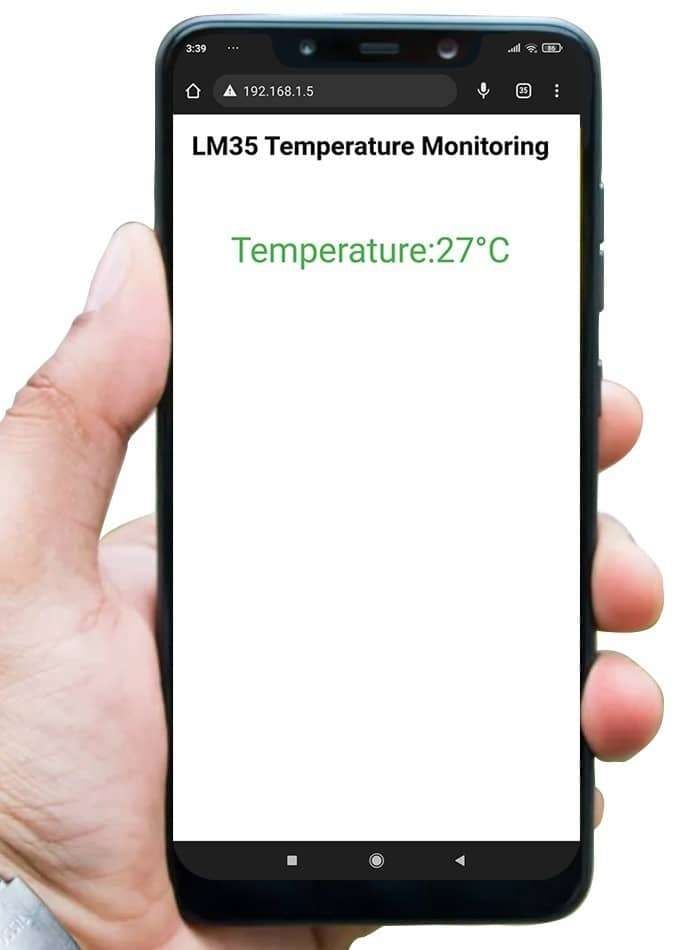
Let’s Understand the code
To understand this code, please refer the basics guide of “How to create the ESP32 Server”.
Once you get the basics of ESP32 server creation, it will be very simple to understand the code.
This code starts with important header files and libraries, In WiFi.h
file contains all ESP32 WiFi related definitions, here we have used them for network connection purposes.
The WebServer.h
file supports handling the HTTP GET and POST requests as well as setting up a server. In the html.h
file contains all the web page code.
#include <WiFi.h>
#include <WebServer.h>
#include "html.h"
Let’s define HTTP port i.e., Port 80 as follows
WebServer server(80);
Setup Function
In setup function, first we set the WiFi as an STA mode and connect to the given SSID and password
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/WiFi.begin(ssid, password);Serial.print("Connecting to ");Serial.println(ssid);delay(1000); /*Wait for 1000mS*/while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");}
After successfully connecting to the server print the local IP address on the serial window.
Serial.print("Your Local IP address is: ");Serial.println(WiFi.localIP()); /*Print the Local IP*/
Handling Client Requests and Serving the Page
To handle the client request, we use server
.on()
function.
It takes two parameters, The first is requested URL path, and the second is the function name, which we want to execute.
As per the below code, when a client requests the root (/)
path, the “MainPage()”
function executes.
Also, when a client requests the “/readTemp”
path, The Temp()
function will be called.
server.on("/", MainPage); /*Client request handling: calls the function to serve HTML page */
server.on("/readTemp", Temp);/*Display the updated Temperature and Humidity value*/
Now start the server using server.begin()
function.
server.begin(); /*Start Server*/
Functions for Serving HTML
We have defined the complete HTML page in file named “html.h”
and added it in header file. With following function, we are sending complete page to client via server.send()
function.
While sending, we are passing the first parameter “200”
which is the status response code as OK
(Standard response for successful HTTP requests).
The second parameter is content type as “text/html”
, and the third parameter is html page code.
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send HTM page to Client*/
}
Now in the below function only we are sending the updated temperature values to the web page.
void Temp() {
String TempValue = String(_temperature); //Convert it into string
server.send(200, "text/plane", TempValue); //Send updated temperature value to the web server
}
Loop Function
Now to handle the incoming client requests and serve the relevant HTML page, we can use handleClient()
function. It executing relevant server.on()
as a callback function although it is defined in void setup()
.
So, it continuously serves the client requests.
server.handleClient();
HTML Web Page Code
This is a code for the web page that shows the measured temperature using a LM35 temperature sensor and ESP32.
/*
ESP32 HTML WebServer Page Code
http:://www.electronicwings.com
*/
const char html_page[] PROGMEM = R"rawSrting(
<!DOCTYPE html>
<html>
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
<body>
<h1>LM35 Temperature Monitoring</h1><br>
<p>Temperature:<span id="TempValue">0</span>°C</p><br>
<script>
setInterval(function() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("TempValue").innerHTML = this.responseText;
}
};
xhttp.open("GET", "readTemp", true);
xhttp.send();
},50);
</script>
</body>
</html>
)rawSrting";
Let’s understand the code step by step
All html pages start with the <!DOCTYPE html>
declaration, it is just information to the browser about what type of document is expected.
<!DOCTYPE html>
The html tag is the container of the complete html page which represents on the top of the html code.
<html>
Now here we are defining the style information for a web page using the <style>
tag. Inside the style tag we have defined the font name, size, color, and test alignment.
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
Inside the body, we are defining the document body, in below we have used headings, and paragraphs if you want you can add images, hyperlinks, tables, lists, etc. also.
On the web page, we are displaying the heading of the page, and inside a paragraph temperature values.
Now temperature value updates under the span id which is manipulated with JavaScript using the id attribute.
<body>
<h1>LM35 Temperature Monitoring</h1><br>
<p>Temperature:<span id="TempValue">0</span>°C</p><br>
Now, this is the javascript that comes under the <script>
tag, this is also called a client-side script.
<script>
In setInterval()
method we are calling the function at every 50mS intervals.
setInterval(function() {},50);
Here we are creating the html XMLHttpRequest
object
var xhttp = new XMLHttpRequest();
The xhttp.onreadystatechange
event is triggered every time the readyState changes and the readyState holds the status of the XMLHttpRequest
.
Now in the below code, the ready state is 4 means the request finished and response is ready and the status is 200
which means OK
.
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
const myArr = JSON.parse(this.responseText);
Now here is the main thing, we are updating the temperature values in html page using TempValue
id.
document.getElementById("TempValue").innerHTML = this.responseText;
Here we used the AJAX method to send the updated values to the server without refreshing the page.
In the below function we have used the GET method and sent the readTemp
function which we defined in the main code asynchronously.
xhttp.open("GET", "readTemp", true);
Send the request to the server using xhttp.send();
function.
xhttp.send();
Close the script
</script>
Close the body
</body>
Close the html.
</html>
Components Used |
||
---|---|---|
LM35 Temperature Sensor LM35 is a sensor which is used to measure temperature. It provides electrical output proportional to the temperature (in Celsius). |
X 1 | |
ESP32-DevKitM-1 ESP32-DevKitM-1 |
X 1 | |
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 |