Overview of RFID
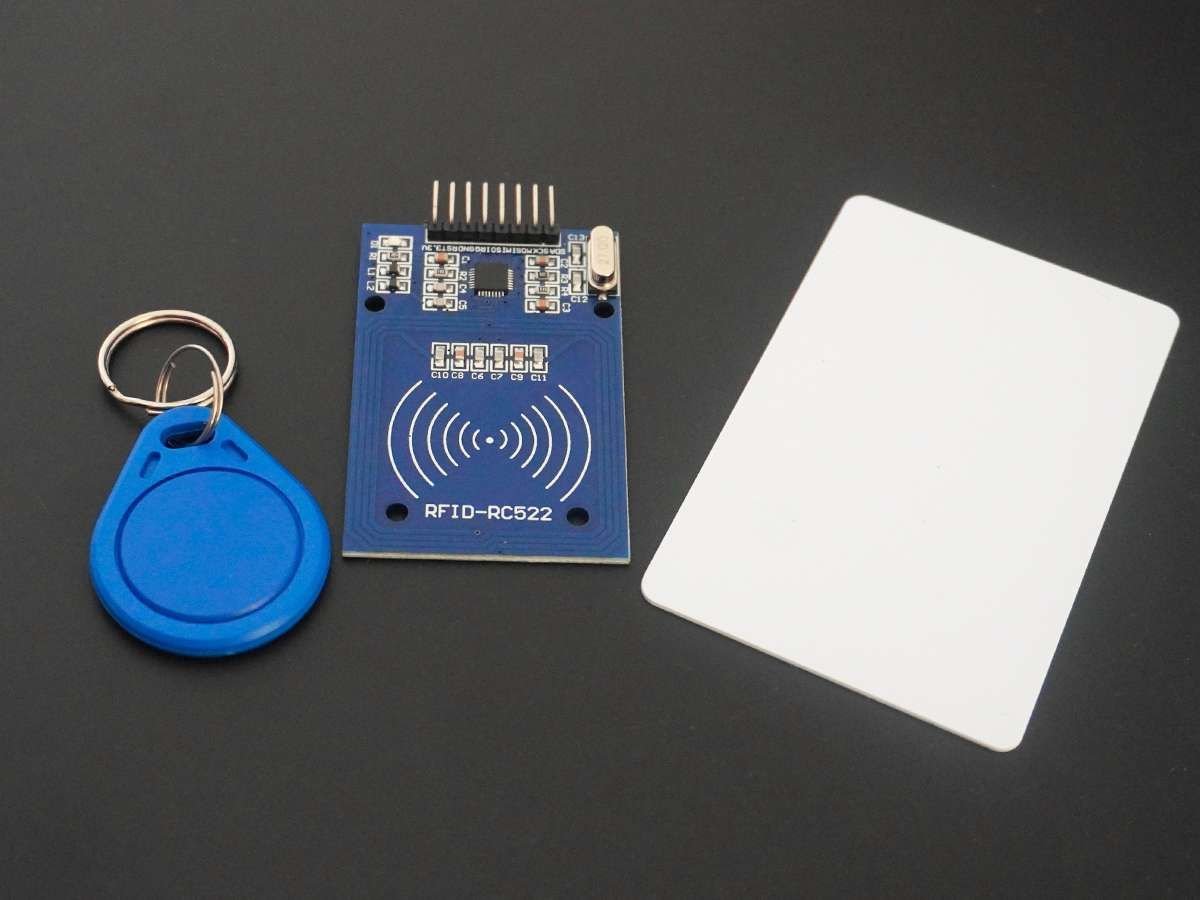
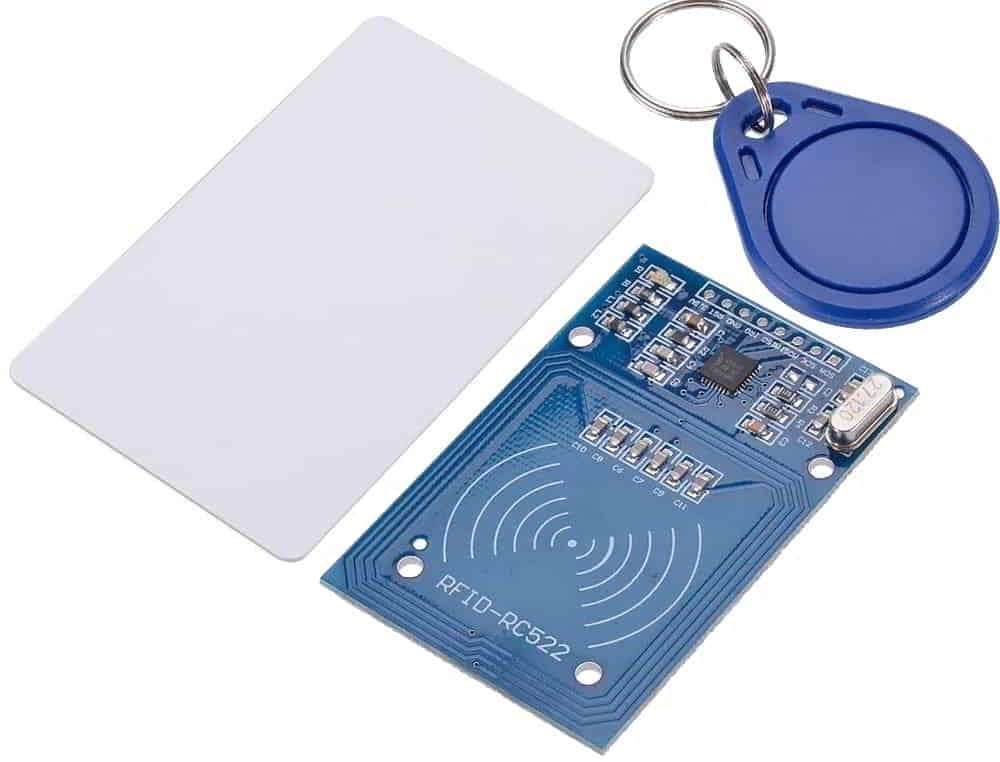
RC522 RFID Modules work on 13.56MHz which is based on the MFRC522 controller which is designed by NXP Semiconductor.
The RC522 module supports SPI and I2C communication protocols. Here we are using the SPI communication Interface with the ESP32 board.
This module operates on a +3.3V/13-26mA Power Supply. The RFID reader automatically goes to power save mode after its operation is complete and it takes a 10-13mA standby current.
We can use this RFID for inventory management, attendance system, access control system, etc.
RFID RC522 Hardware Connection with ESP32
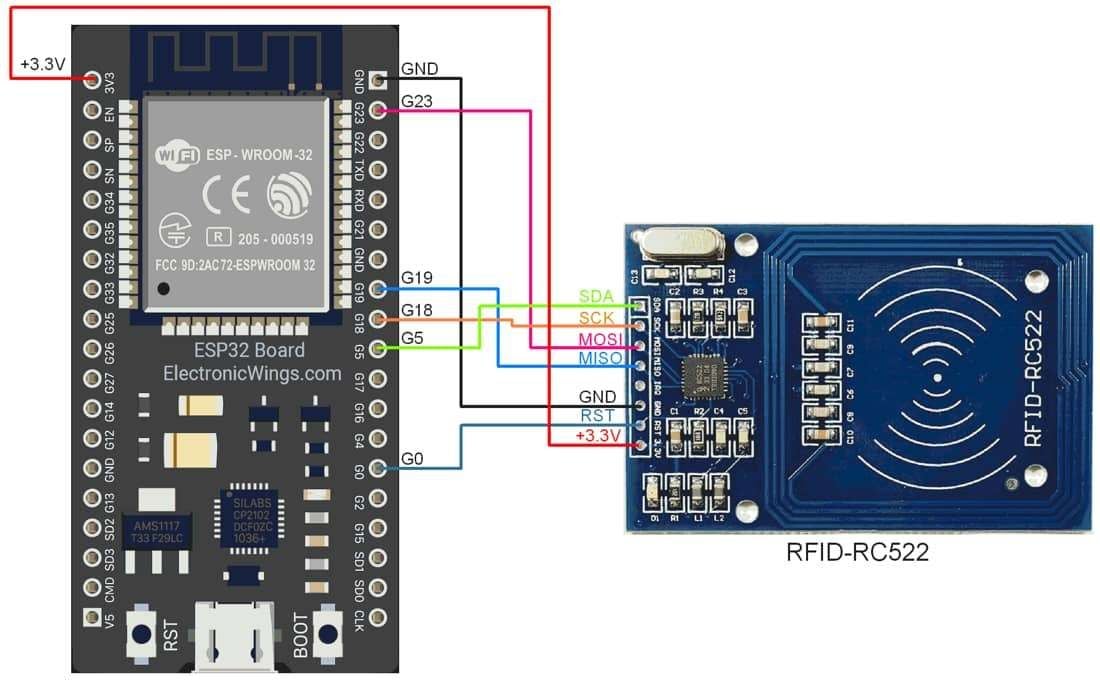
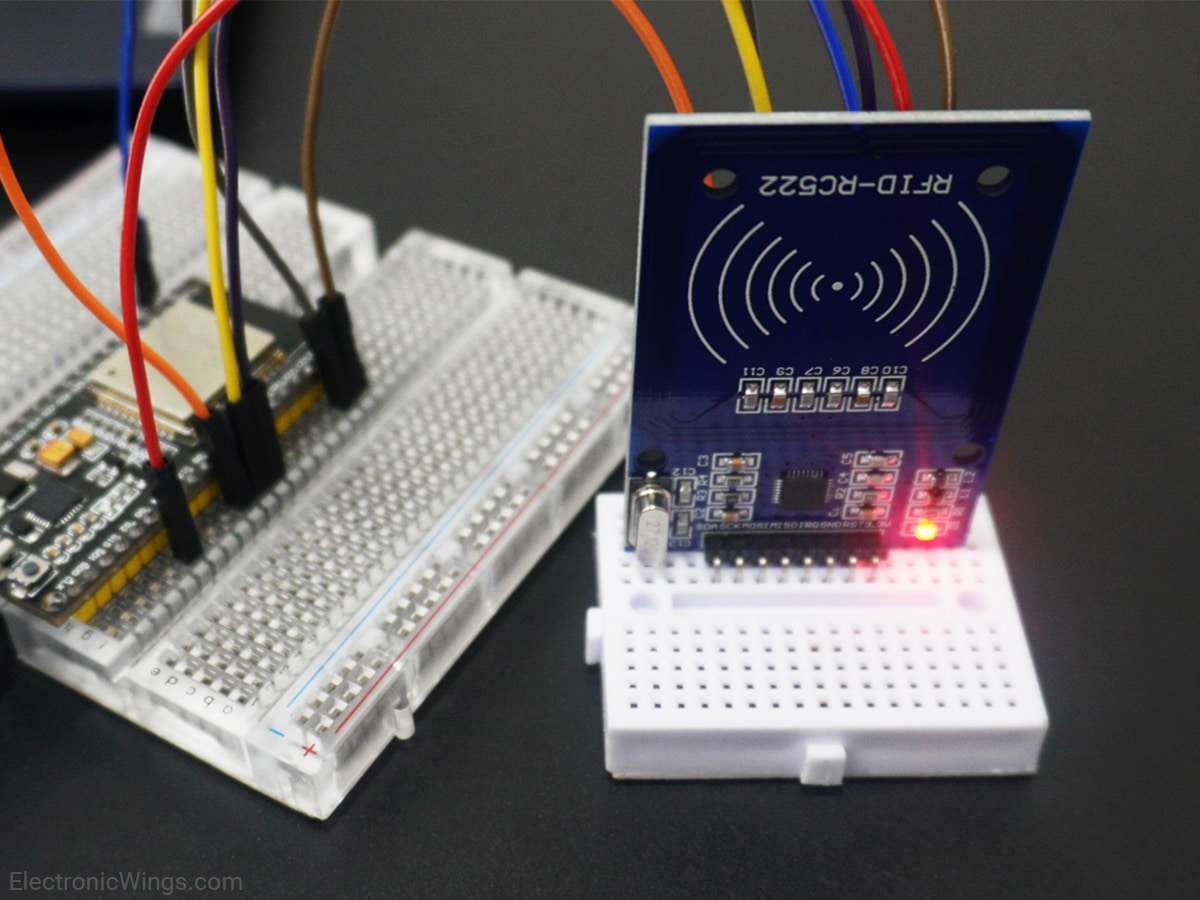
Sr No | RFID RC522 Pins | ESP32 Pins |
1 | VCC | +3.3V |
2 | RST | D0 |
3 | GND | GND |
4 | MISO | 19 |
5 | MOSI | 23 |
6 | SCK | 18 |
7 | SS/SDA | 5 |
Read the MFRC522 RFID using ESP32
Here we are using MFRC522 libraries to read the RFID tag example. We will need to install the MFRC522 library using the Arduino Library Manager.
Open the Arduino IDE and navigate to Sketch ► Include Library ► Manage Libraries…
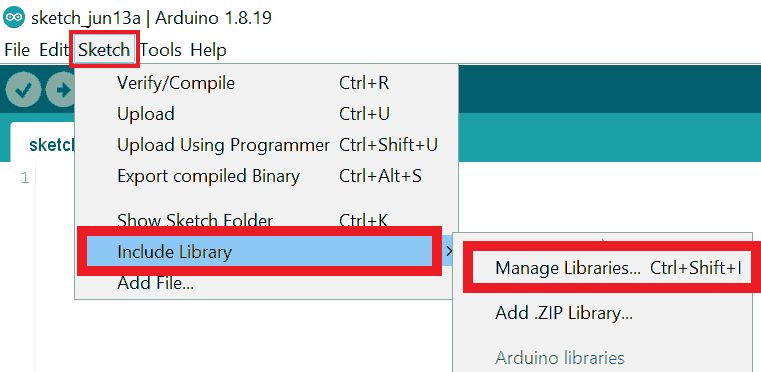
The library Manager window will pop up. Now enter MFRC522 into the search box and click Install on the MFRC522 option to install version 1.4.10 or higher.
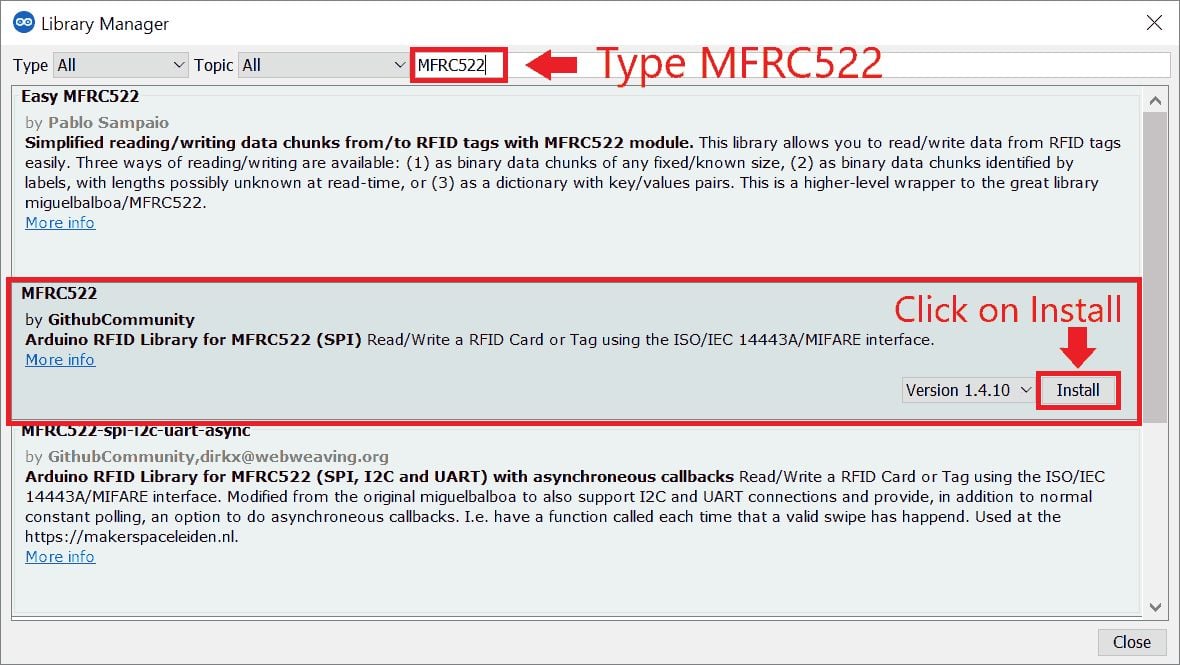
Now open the example of MFRC522. To open it navigate to File ► Examples ► MFRC522 ► ReadNUID
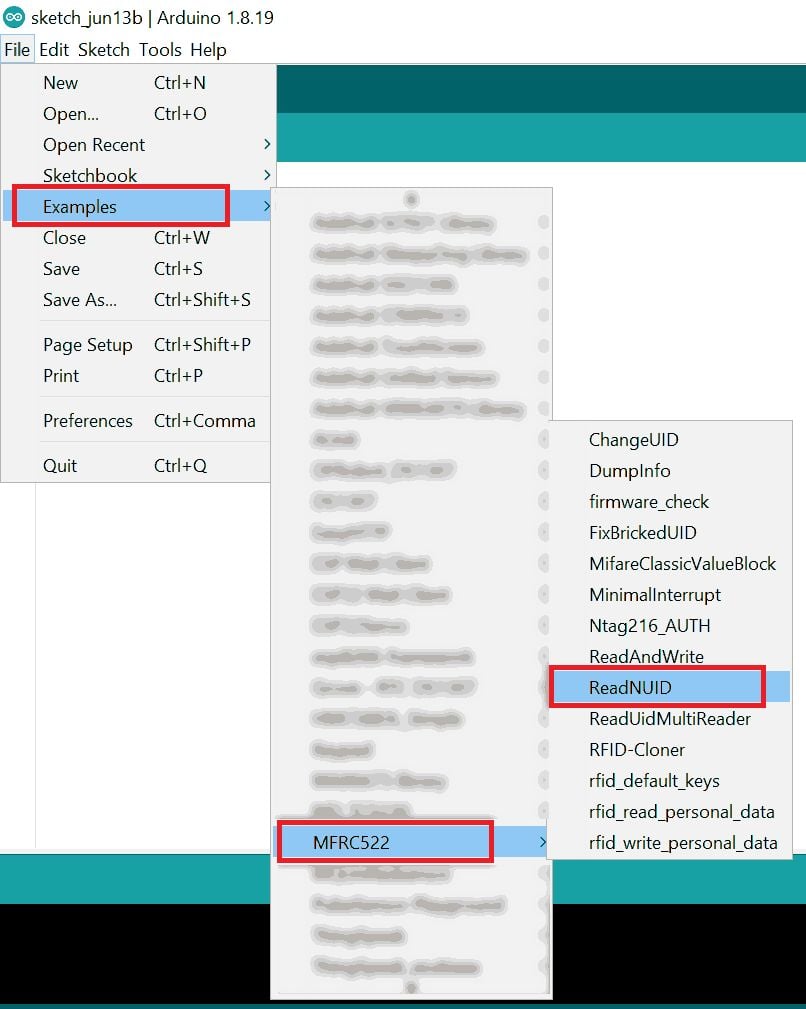
Note: When you open the code, please change the SS and RST pin numbers in the code as shown below.
#define SS_PIN 5
#define RST_PIN 0
Or use the below code
Code for RC522 RFID using ESP32
/*
* --------------------------------------------------------------------------------------------------------------------
* Example sketch/program showing how to read new NUID from a PICC to serial.
* --------------------------------------------------------------------------------------------------------------------
* This is a MFRC522 library example; for further details and other examples see: https://github.com/miguelbalboa/rfid
*
* Example sketch/program showing how to the read data from a PICC (that is: a RFID Tag or Card) using a MFRC522 based RFID
* Reader on the Arduino SPI interface.
*
* When the Arduino and the MFRC522 module are connected (see the pin layout below), load this sketch into Arduino IDE
* then verify/compile and upload it. To see the output: use Tools, Serial Monitor of the IDE (hit Ctrl+Shft+M). When
* you present a PICC (that is: a RFID Tag or Card) at reading distance of the MFRC522 Reader/PCD, the serial output
* will show the type, and the NUID if a new card has been detected. Note: you may see "Timeout in communication" messages
* when removing the PICC from reading distance too early.
*
* @license Released into the public domain.
*
* Typical pin layout used:
* -----------------------------------------------------------------------------------------
* MFRC522 Arduino Arduino Arduino Arduino Arduino
* Reader/PCD Uno/101 Mega Nano v3 Leonardo/Micro Pro Micro
* Signal Pin Pin Pin Pin Pin Pin
* -----------------------------------------------------------------------------------------
* RST/Reset RST 9 5 D9 RESET/ICSP-5 RST
* SPI SS SDA(SS) 10 53 D10 10 10
* SPI MOSI MOSI 11 / ICSP-4 51 D11 ICSP-4 16
* SPI MISO MISO 12 / ICSP-1 50 D12 ICSP-1 14
* SPI SCK SCK 13 / ICSP-3 52 D13 ICSP-3 15
*
* More pin layouts for other boards can be found here: https://github.com/miguelbalboa/rfid#pin-layout
*/
#include <SPI.h>
#include <MFRC522.h>
#define SS_PIN 5
#define RST_PIN 0
MFRC522 rfid(SS_PIN, RST_PIN); // Instance of the class
MFRC522::MIFARE_Key key;
// Init array that will store new NUID
byte nuidPICC[4];
void setup() {
Serial.begin(9600);
SPI.begin(); // Init SPI bus
rfid.PCD_Init(); // Init MFRC522
for (byte i = 0; i < 6; i++) {
key.keyByte[i] = 0xFF;
}
Serial.println(F("This code scan the MIFARE Classsic NUID."));
Serial.print(F("Using the following key:"));
printHex(key.keyByte, MFRC522::MF_KEY_SIZE);
}
void loop() {
// Reset the loop if no new card present on the sensor/reader. This saves the entire process when idle.
if ( ! rfid.PICC_IsNewCardPresent())
return;
// Verify if the NUID has been readed
if ( ! rfid.PICC_ReadCardSerial())
return;
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = rfid.PICC_GetType(rfid.uid.sak);
Serial.println(rfid.PICC_GetTypeName(piccType));
// Check is the PICC of Classic MIFARE type
if (piccType != MFRC522::PICC_TYPE_MIFARE_MINI &&
piccType != MFRC522::PICC_TYPE_MIFARE_1K &&
piccType != MFRC522::PICC_TYPE_MIFARE_4K) {
Serial.println(F("Your tag is not of type MIFARE Classic."));
return;
}
if (rfid.uid.uidByte[0] != nuidPICC[0] ||
rfid.uid.uidByte[1] != nuidPICC[1] ||
rfid.uid.uidByte[2] != nuidPICC[2] ||
rfid.uid.uidByte[3] != nuidPICC[3] ) {
Serial.println(F("A new card has been detected."));
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
Serial.println(F("The NUID tag is:"));
Serial.print(F("In hex: "));
printHex(rfid.uid.uidByte, rfid.uid.size);
Serial.println();
Serial.print(F("In dec: "));
printDec(rfid.uid.uidByte, rfid.uid.size);
Serial.println();
}
else Serial.println(F("Card read previously."));
// Halt PICC
rfid.PICC_HaltA();
// Stop encryption on PCD
rfid.PCD_StopCrypto1();
}
/**
* Helper routine to dump a byte array as hex values to Serial.
*/
void printHex(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], HEX);
}
}
/**
* Helper routine to dump a byte array as dec values to Serial.
*/
void printDec(byte *buffer, byte bufferSize) {
for (byte i = 0; i < bufferSize; i++) {
Serial.print(buffer[i] < 0x10 ? " 0" : " ");
Serial.print(buffer[i], DEC);
}
}
MFRC522 RFID Output on Serial Window
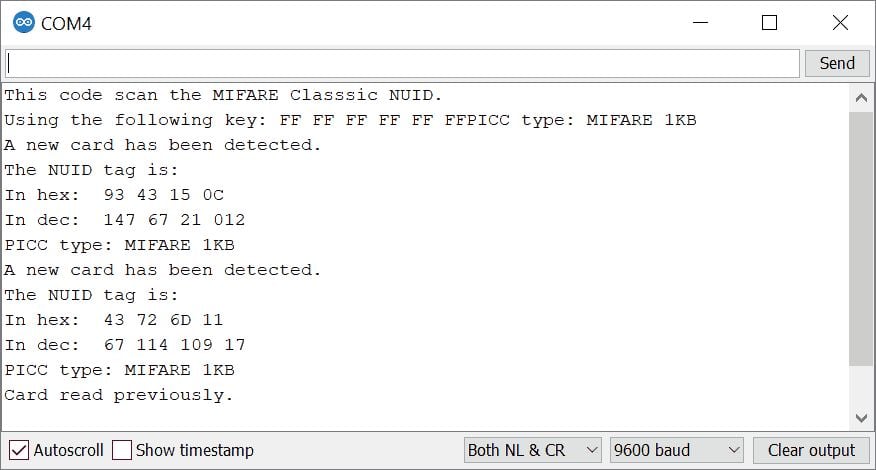
Let’s understand the code
First, include the SPI and MFRC522 libraries
#include <SPI.h>
#include <MFRC522.h>
Define the ESP32 pins to which the RC522 is connected and instantiate the MFRC522 reader object.
#define SS_PIN 5
#define RST_PIN 0
MFRC522 rfid(SS_PIN, RST_PIN); // Instance of the class
MFRC522::MIFARE_Key key;
Then define the 4 bytes of array called nuidPICC, to hold the message we want to write to the block.
If you want to delete the block then simply set nuidPICC to 0.
// Init array that will store new NUID
byte nuidPICC[4];
In setup function
In the setup function, We have initiated the serial communication with a 9600 Baud rate, the SPI library, and the MFRC522 object.
For read and write operations we prepare a security key. Here all six bytes of the key are set to 0xFF.
Serial.begin(9600);
SPI.begin(); // Init SPI bus
rfid.PCD_Init(); // Init MFRC522
for (byte i = 0; i < 6; i++) {
key.keyByte[i] = 0xFF;
}
Serial.println(F("This code scan the MIFARE Classsic NUID."));
Serial.print(F("Using the following key:"));
printHex(key.keyByte, MFRC522::MF_KEY_SIZE);
In loop function
In the loop, we will check the PICC type and read the card ID in hexadecimal and decimal format.
Reset the loop if no new card is present on the reader using rfid.PICC_IsNewCardPresent()
function. This saves the entire process when idle and verifies if the NUID has been readed using rfid.PICC_ReadCardSerial()
function
if ( ! rfid.PICC_IsNewCardPresent())
return;
if ( ! rfid.PICC_ReadCardSerial())
return;
Check the PICC (Proximity Integrated Circuit Card) type here
Serial.print(F("PICC type: "));
MFRC522::PICC_Type piccType = rfid.PICC_GetType(rfid.uid.sak);
Serial.println(rfid.PICC_GetTypeName(piccType));
// Check is the PICC of Classic MIFARE type
if (piccType != MFRC522::PICC_TYPE_MIFARE_MINI &&
piccType != MFRC522::PICC_TYPE_MIFARE_1K &&
piccType != MFRC522::PICC_TYPE_MIFARE_4K) {
Serial.println(F("Your tag is not of type MIFARE Classic."));
return;
}
Now here read the card and check the NUID and print on the serial monitor in hexadecimal and decimal format. If the previous card is read again then Card read previously will be printed on the serial monitor.
if (rfid.uid.uidByte[0] != nuidPICC[0] ||
rfid.uid.uidByte[1] != nuidPICC[1] ||
rfid.uid.uidByte[2] != nuidPICC[2] ||
rfid.uid.uidByte[3] != nuidPICC[3] ) {
Serial.println(F("A new card has been detected."));
// Store NUID into nuidPICC array
for (byte i = 0; i < 4; i++) {
nuidPICC[i] = rfid.uid.uidByte[i];
}
Serial.println(F("The NUID tag is:"));
Serial.print(F("In hex: "));
printHex(rfid.uid.uidByte, rfid.uid.size);
Serial.println();
Serial.print(F("In dec: "));
printDec(rfid.uid.uidByte, rfid.uid.size);
Serial.println();
}
else Serial.println(F("Card read previously."));
Components Used |
||
---|---|---|
RC522 RFID MFRC522 is a highly integrated reader/writer IC for contactless communication at 13.56MHz. |
X 1 | |
RFID CARD RFID CARD |
X 5 | |
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 |
Downloads |
||
---|---|---|
|
ReadNUID | Download |