Description
ESP32 has inbuilt Wi-Fi functionalities. It has three Wi-Fi modes.
Before that, let’s understand the terms Wi-Fi station and Access Point
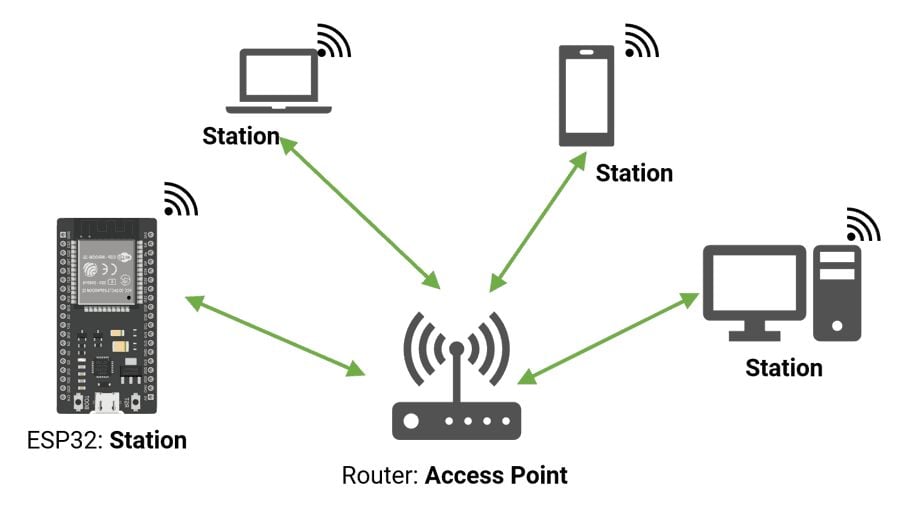
- Wi-Fi Station: It is a device that can connect to other Wi-Fi networks, such as Wi-Fi routers. It is also referred to as a node or wireless client.
- Access Point: Device such as Wi-Fi Router, which allows other Wi-Fi devices to connect to it. Generally, Access points are connected to a wired connection like Ethernet.
- SoftAP (Software Enabled Access Point): Devices that are not specifically routers, but can be enabled as an access point called SoftAP. e.g., Smartphone Hotspot.
ESP32 Wi-Fi Modes
Wi-Fi modes are defined in the WiFi.h
library. We need to include it.
#include <WiFi.h>
We need to use WiFi.mode()
function to set the mode.
1 | Station Mode: ESP32 connects to an Access point. | WiFi.mode(WIFI_STA) |
2 | Access Point Mode: Stations can connect to the ESP32 | WiFi.mode(WIFI_AP) |
3 | Access point and Hotspot Mode: both at the same time | WiFi.mode(WIFI_AP_STA) |
Mode 1: ESP32 Wi-Fi Station
In this mode, ESP32 connects to the other network such as a Wi-Fi router. In the connection process, the Wi-Fi router assigns the unique IP address to the ESP32.
The router is connected to the internet, so we can request data from the internet i.e. Cloud, APIs, also we can send the data to the internet.
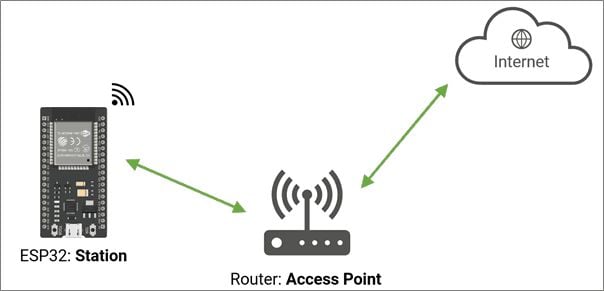
Mode 2: ESP32 Access Point
In Access point mode, ESP32 creates its own Wi-Fi network. It acts as a router and other devices can connect to the ESP32 like smartphones and laptops.
This mode is mentioned as soft-AP (Soft Access Point).
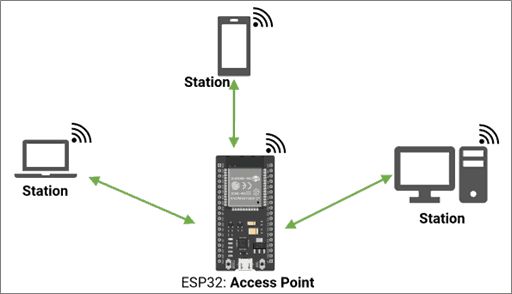
Mode 3: ESP32 Access point and Hotspot Mode
ESP32 acts in both modes at the same time.
Note: Mode 2,3 are not covered in this guide. We will cover it in another guide.
Now, we will see how to connect with the Wi-Fi using ESP32 in MODE 1:
ESP32 Connecting to the Wi-Fi
Step-1: Set Wi-Fi Mode to Wi-Fi Station using
WiFi.mode(WIFI_STA);
Step-2: Connect to Wi-Fi by providing your network’s Wi-Fi SSID
and Password
to connect with using
WiFi.begin(ssid, password);
Before this, we need to set this parameter as follows.
// Replace with your network credentials
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
Step3: Done! Connecting to the Wi-Fi can take a while, so we need to add a while loop for checking the connection status. Using WiFi.status()
. After a successful connection, it returns WL_CONNECTED
.
Code
/*
ESP32 Connect to Wi-Fi
http:://www.electronicwings.com
*/
#include <WiFi.h>
// Replace with your network credentials
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
void initWiFi() {
WiFi.mode(WIFI_STA); //Set Wi-Fi Mode as station
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi ..");
while (WiFi.status() != WL_CONNECTED) {
Serial.print('.');
delay(1000);
}
Serial.println(WiFi.localIP());
Serial.print("RRSI: ");
Serial.println(WiFi.RSSI());
}
void setup() {
Serial.begin(115200);
initWiFi();
}
void loop() {
// put your main code here, to run repeatedly:
}
Output
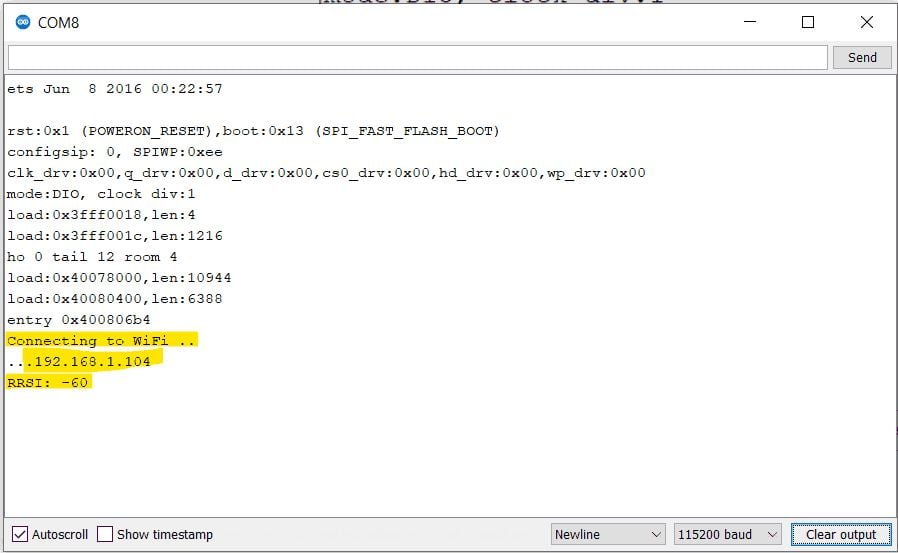
ESP32 Wi-Fi Connection Strength
simply call WiFi.RSSI()
after a Wi-Fi connection to get connection strength.
Get ESP32 IP Address
call WiFi.localIP()
after establishing a connection with your network.
We can also set the static IP Address to the ESP32. Please check this guide “How to set the static IP address to the ESP32” (Set URL after gets Live)
ESP32 Wi-Fi Connection Status
WiFi.status()
returns one of the following values that correspond to the constants on the table:
Constant | Meaning |
WL_IDLE_STATUS | temporary status assigned when WiFi.begin() is called |
WL_NO_SSID_AVAIL | when no SSID are available |
WL_SCAN_COMPLETED | scan networks is completed |
WL_CONNECTED | when connected to a WiFi network |
WL_CONNECT_FAILED | when the connection fails for all the attempts |
WL_CONNECTION_LOST | when the connection is lost |
WL_DISCONNECTED | when disconnected from a network |
Disconnect from Wi-Fi Network
If we need to disconnect from Wi-Fi, Use WiFi.disconnect();
WiFi.disconnect();
So we have successfully covered the ESP32 Wi-Fi basics, its modes, and how we can connect with the Wi-Fi.
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 |
Downloads |
||
---|---|---|
|
ESP32_WiFI_Station_Mode_Code | Download |