Description
- Let’s build WhatsApp Temperature Alert System using ESP32.
- For Temperature measurement, we will use the DS18B20 temperature sensor as an example. It is a digital temperature sensor that measures temperature in the range of -55°C to 125°C
What is WhatsApp?
- We all know WhatsApp!, It is a popular messaging application that allows users to send text messages, voice messages, make voice and video calls, share multimedia files such as photos and videos, and create group chats.
- WhatsApp is available for smartphones running various operating systems, including iOS, Android, and Windows Phone.
CallMeBot WhatsApp API
- Before we start, need to know we can not send messages directly to WhatsApp.
- We need an API that acts as a mediator between esp32 and WhatsApp to send the message.
- In this case, we will take the help of CallMeBot which is a simple API to send the message to WhatsApp from your program, scripts, etc.
- To know more about CallMeBot visit the below link
- www.callmebot.com
Now Let’s start.
Step 1
Visit the CallMeBot website (www.callmebot.com).
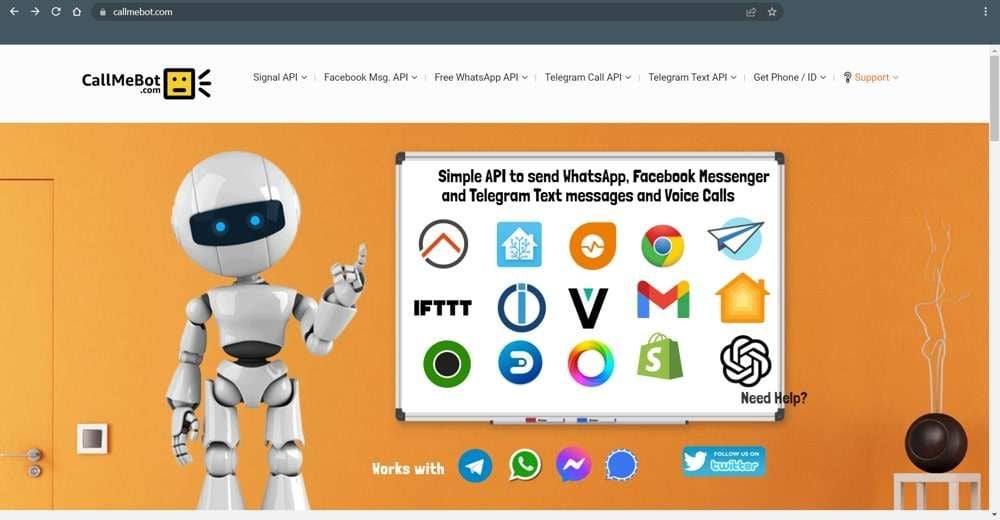
Step 2
Click on Free WhatsApp API and then click on Send Messages
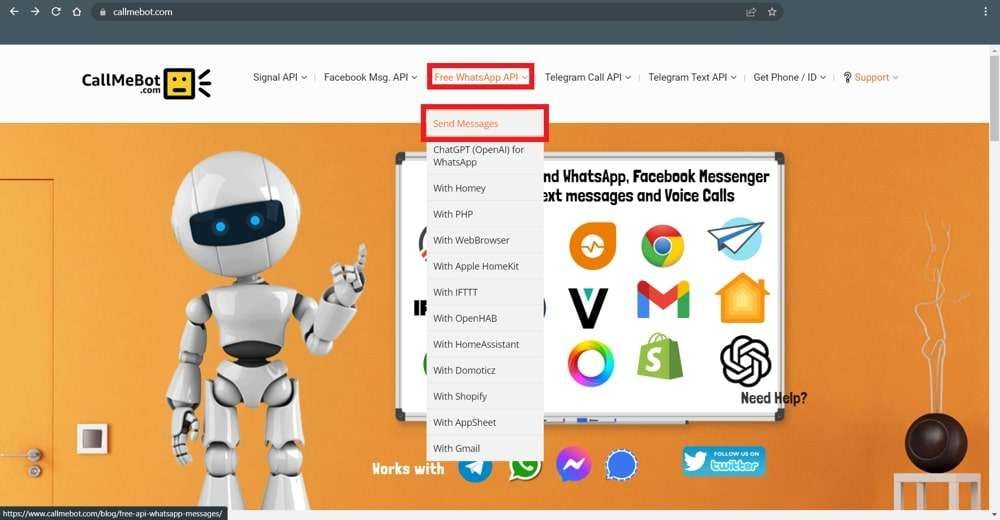
Step 3
Now let’s do the setup as given in CallMeBot Website below:
- Add the phone number +34 611 04 87 48 to your Phone Contacts. (Name it as you wish)
- Send this message "I allow callmebot to send me messages" to the new Contact created (using WhatsApp of course)
- Wait until you receive the message "API Activated for your phone number. Your APIKEY is 123123" from the bot.
Note: If you don't receive the ApiKey in 2 minutes, please try again after 24hs.
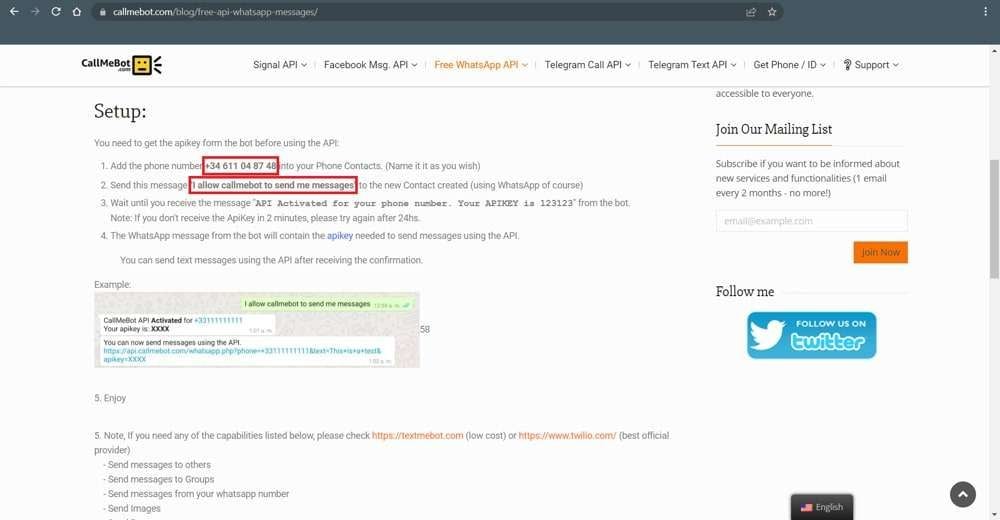
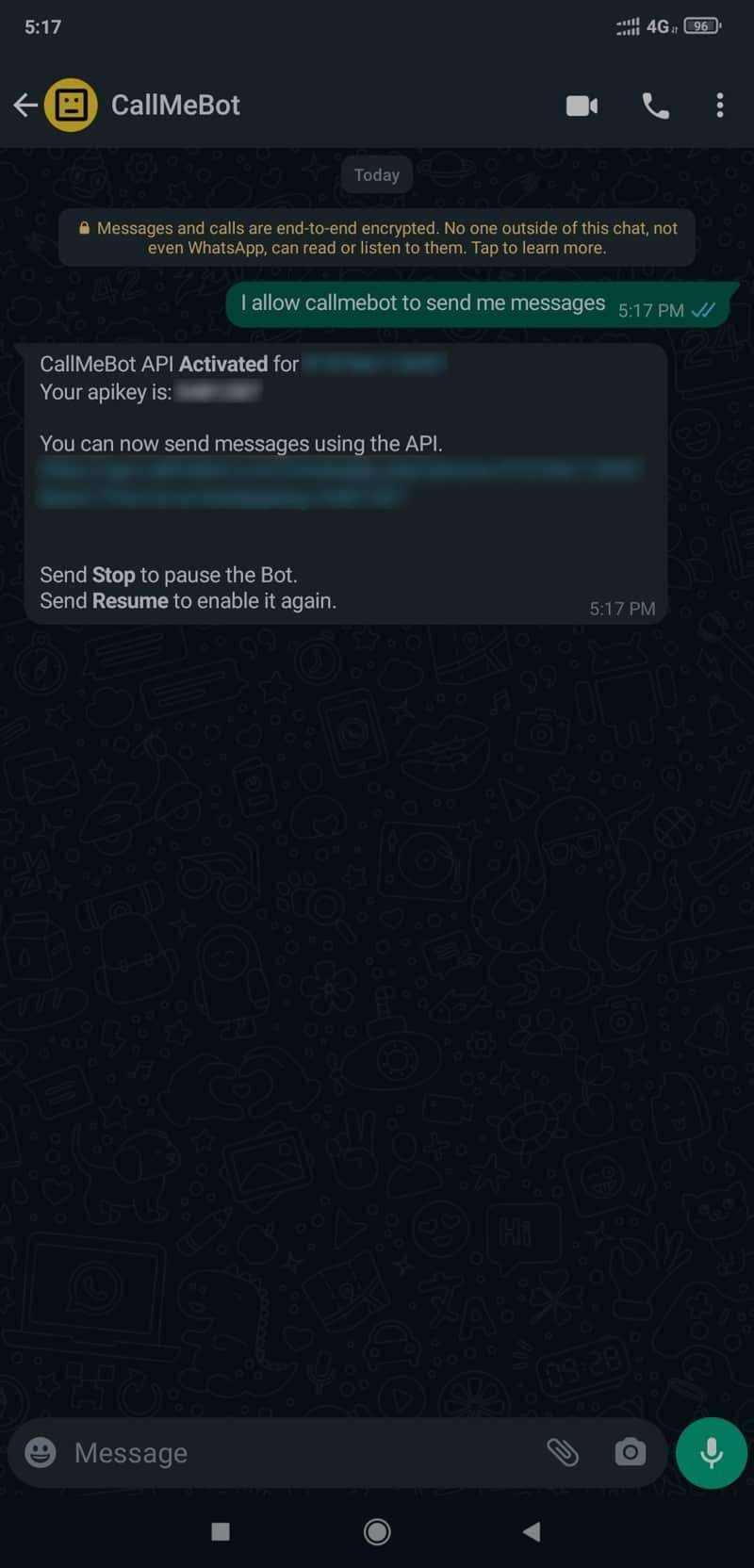
DS18B20 Interfacing Diagram with ESP32
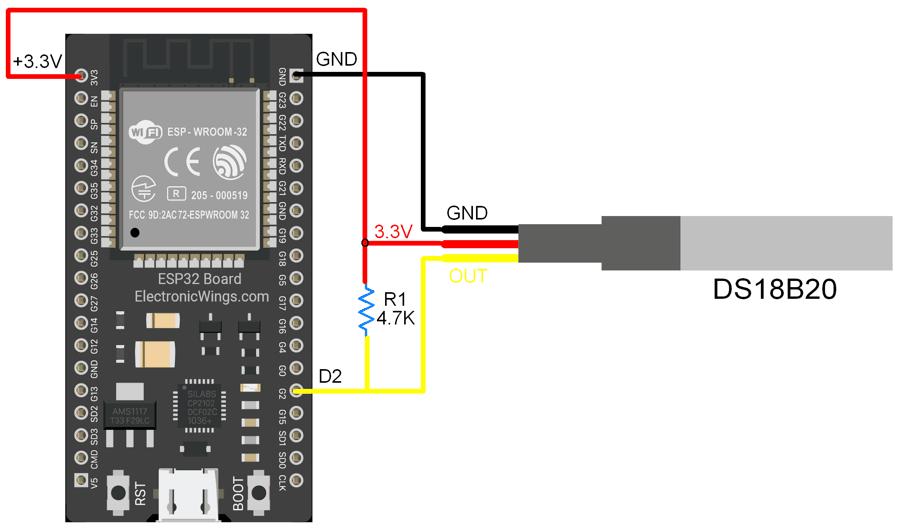
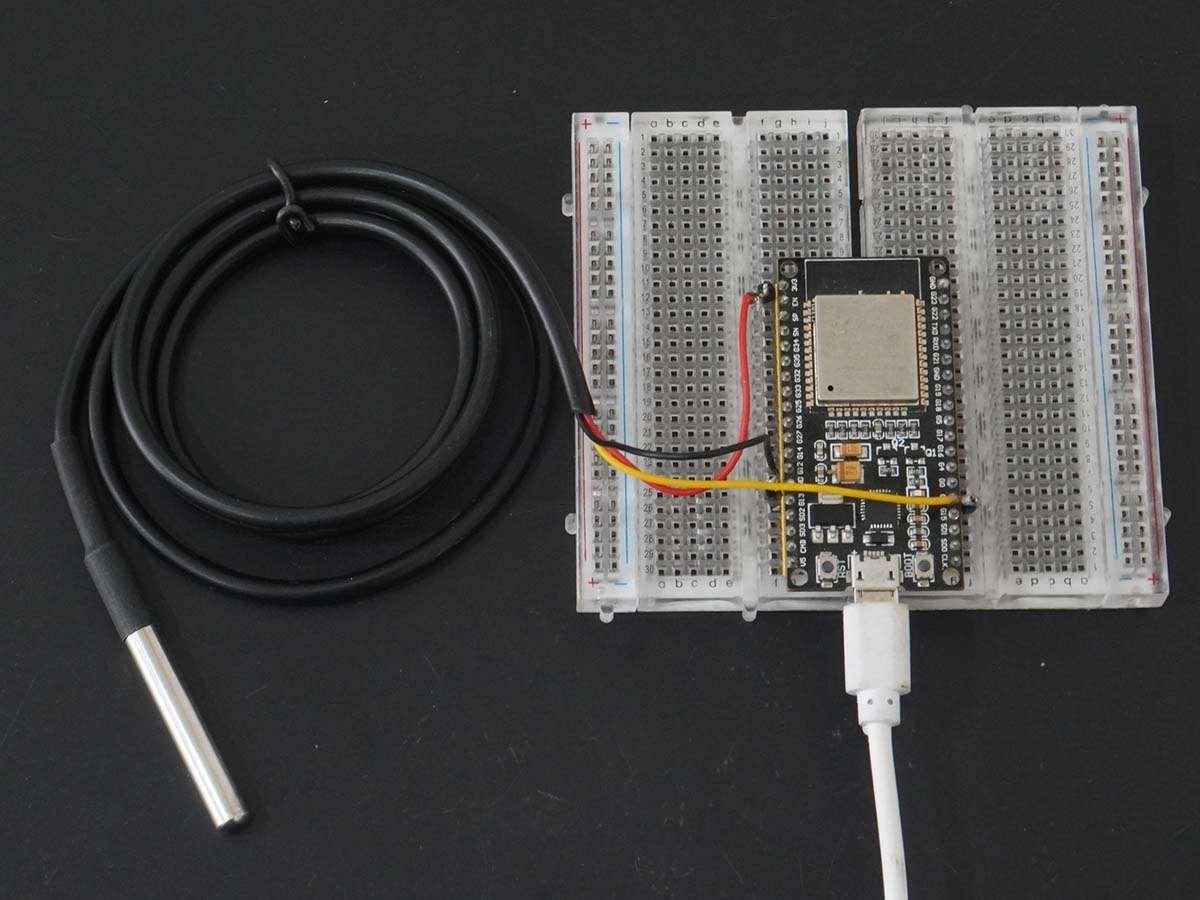
Send the text message to WhatsApp using ESP32
Let’s install the required library.
Here we are using URLEncode libraries for the above example. We need to install the URLEncode library using the Arduino Library Manager.
- Open the Arduino IDE
- Navigate to Sketch ► Include Library ► Manage Libraries…
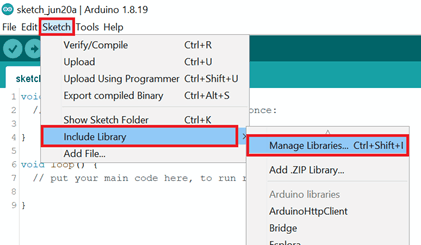
- The library Manager window will pop up. Now enter URLEncode into the search box, and click Install on the URLEncode option to install version 1.0.1 or higher. As shown below image.
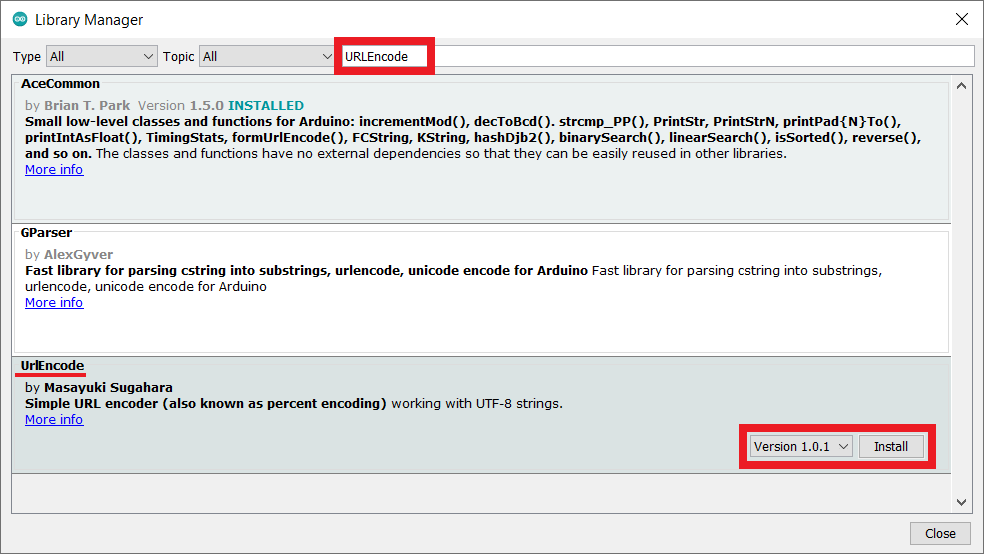
- Next, add the library for the DS18B20 temperature sensor, type DS18B20 into the search box, and click Install on the DallasTemperature option to install version 3.9.0 or higher. As shown below image.
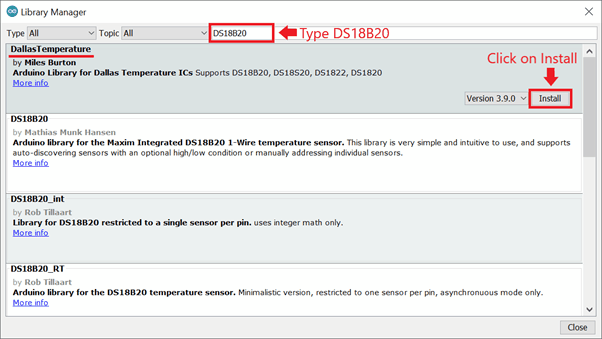
- If you don’t have the OneWire library, then this pop-up will come then click on Install all.
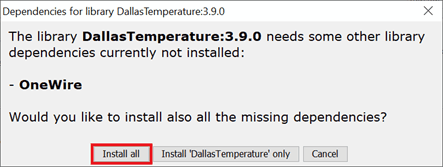
Send Over temperature WhatsApp notification using ESP32
Before uploading the code make sure you have added your SSID, Password, Mobile Number, and API Key as follows.
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASS";
String MobileNumber = "ADD_YOUR_PHONE_NUMBER";
String APIKey = "ADD_YOUR_API_KEY";
Over Temperature Alert on WhatsApp code for ESP32
#include <WiFi.h>
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
#include <OneWire.h>
#include <DallasTemperature.h>
// Data wire is plugged into port 2 on the Arduino
#define ONE_WIRE_BUS 2
// Setup a oneWire instance to communicate with any OneWire devices (not just Maxim/Dallas temperature ICs)
OneWire oneWire(ONE_WIRE_BUS);
// Pass our oneWire reference to Dallas Temperature.
DallasTemperature sensors(&oneWire);
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASS";
String MobileNumber = "ADD_YOUR_PHONE_NUMBER";
String APIKey = "ADD_YOUR_API_KEY";
void setup() {
Serial.begin(115200);
pinMode (2, INPUT_PULLUP);
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP());
delay(1000);
sensors.begin();
}
void loop() {
// call sensors.requestTemperatures() to issue a global temperature
// request to all devices on the bus
Serial.print("Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperatures
Serial.println("DONE");
// After we got the temperatures, we can print them here.
// We use the function ByIndex, and as an example get the temperature from the first sensor only.
float tempC = sensors.getTempCByIndex(0);
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
if(tempC > 30) {
String message = "Temperature is above the threshold!\n";
message += "Current temperature: " + String(tempC, 2) + " °C";
sendMessage(message);
}
}
else
{
Serial.println("Error: Could not read temperature data");
}
delay(10000);
}
void sendMessage(String message){
String url = "https://api.callmebot.com/whatsapp.php?phone=" + MobileNumber + "&apikey=" + APIKey + "&text=" + urlEncode(message);
HTTPClient http;
http.begin(url);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpResponseCode = http.POST(url);
if (httpResponseCode == 200){
Serial.print("Message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(httpResponseCode);
}
http.end();
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
Output on serial monitor
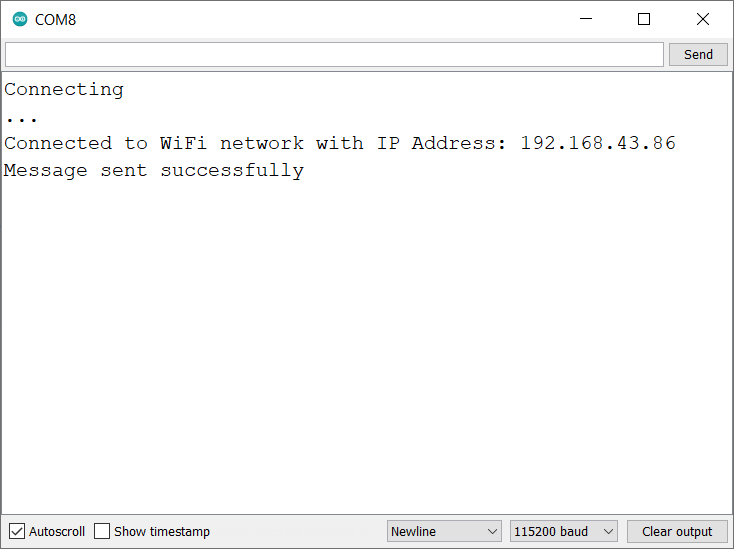
Output on Web Server
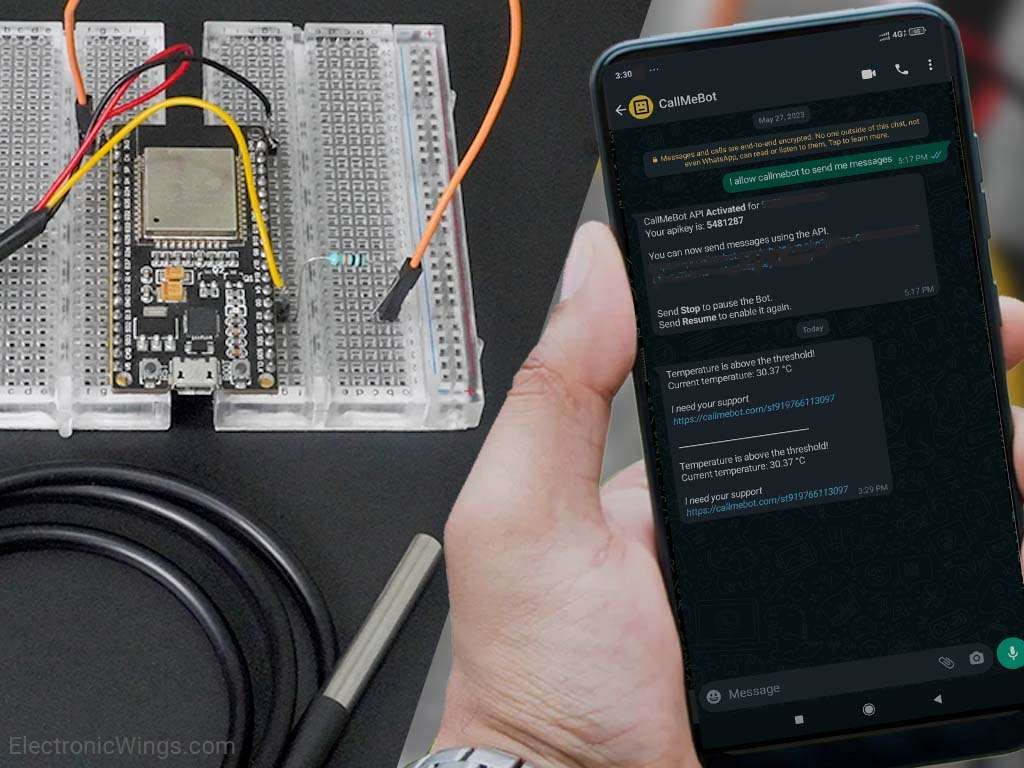
Lets understand the code
Add the required libraries
#include <WiFi.h>
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
#include <OneWire.h>
#include <DallasTemperature.h>
Set the pin number 2 for DS1307 of ESP32
#define ONE_WIRE_BUS 2
Setup a oneWire instance to communicate with any OneWire devices
OneWire oneWire(ONE_WIRE_BUS);
Pass oneWire reference to DallasTemperature.
DallasTemperature sensors(&oneWire);
In the setup function
Set the baud rate to 115200
Serial.begin(115200);
Now set the WiFi as an STA mode and connect to the given SSID and password
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
client.setCACert(TELEGRAM_CERTIFICATE_ROOT);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
In loop function
Call sensors.requestTemperatures()
to issue a global temperature request to all devices on the bus
sensors.requestTemperatures(); // Send the command to get temperatures
After getting the temperature, we saved it on the tempC
float variable.
Here we have connected only one temperature sensor, if you have multiple sensors then add in ByIndex function.
float tempC = sensors.getTempCByIndex(0);
Now check the received temperature status reading and print on the serial monitor, if temperature gove above 30 degree then send the message to the whatsapp
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
if(tempC > 30) {
String message = "Temperature is above the threshold!\n";
message += "Current temperature: " + String(tempC, 2) + " °C";
sendMessage(message);
}
}
else
{
Serial.println("Error: Could not read temperature data");
}
Wait for 10 seconds for the next reading
delay(10000)
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 | |
DS18B20 Waterproof temperature sensor DS18B20 Waterproof temperature sensor |
X 1 |
Downloads |
||
---|---|---|
|
ESP32_whatsapp_alert | Download |