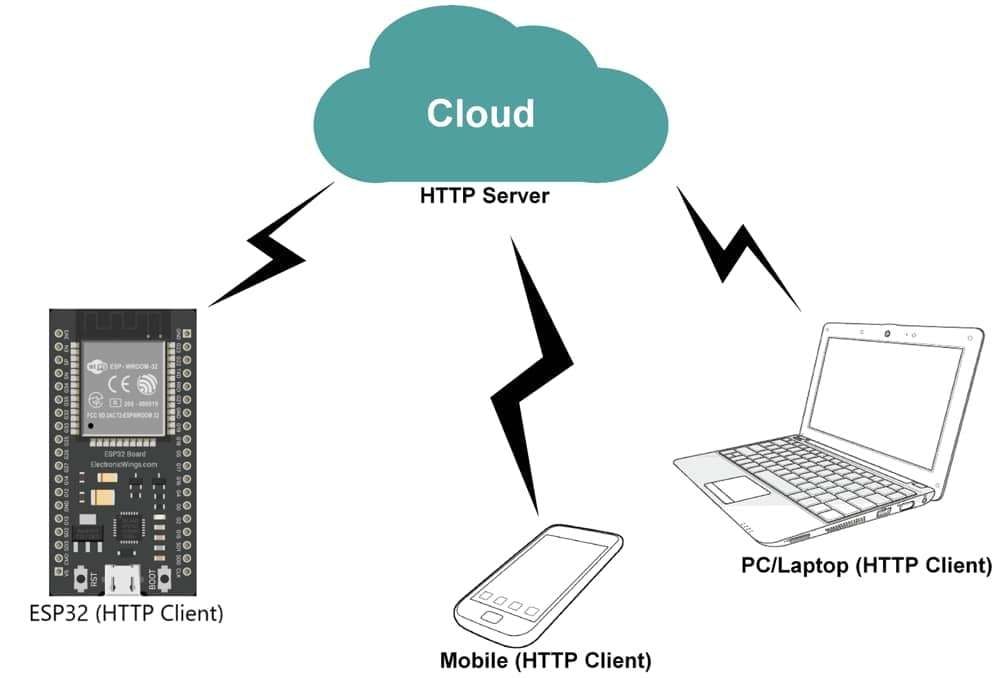
- HTTP (Hypertext Transfer Protocol) is a standard application layer protocol.
- The function of HTTP is to request-response protocol between client and server.
- HTTP client helps to send requests and receive responses from the HTTP server.
- It is widely used in IoT-based embedded applications like Home Automation, vehicle engine parameter monitoring remotely for analysis, etc.
- ESP32 is a hardware platform. It has onboard 2.4 GHz Wi-Fi, Bluetooth, and Bluetooth LE module available for a wide range of applications.
Example
Let’s write a code for ESP32 as an HTTP Client and GET/POST the data from to the ThingSpeak server.
Here, we are using the ThingSpeak server for HTTP Client demo purposes.
What is ThingSpeak?
ThingSpeak is an open IoT platform where anyone can visualize and analyze live data from their sensor devices. Also, we can perform data analysis on data posted by remote devices with Matlab code in ThingSpeak. To learn more about ThingSpeak refer link
https://thingspeak.com/pages/learn_more
Install required libraries
Here we are using ThingSpeak’s official library to get and post the data. We need to install the ThingSpeak library using the Arduino Library Manager.
- Open the Arduino IDE and navigate to Sketch ► Include Library ► Manage Libraries…
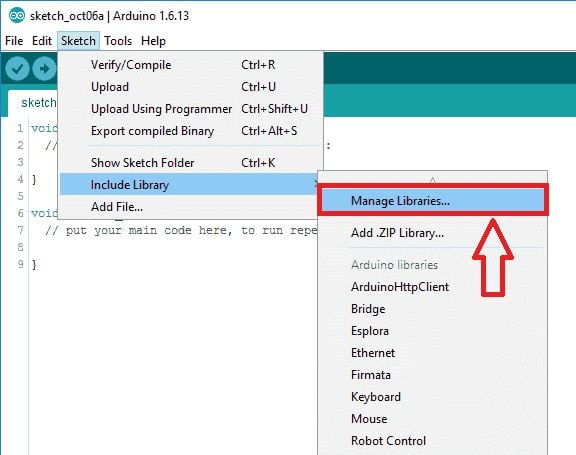
- The Library Manager window will pop up. Now enter ThingSpeak into the search box and click Install on the ThingSpeak library option to install version 2.0.1 or higher.
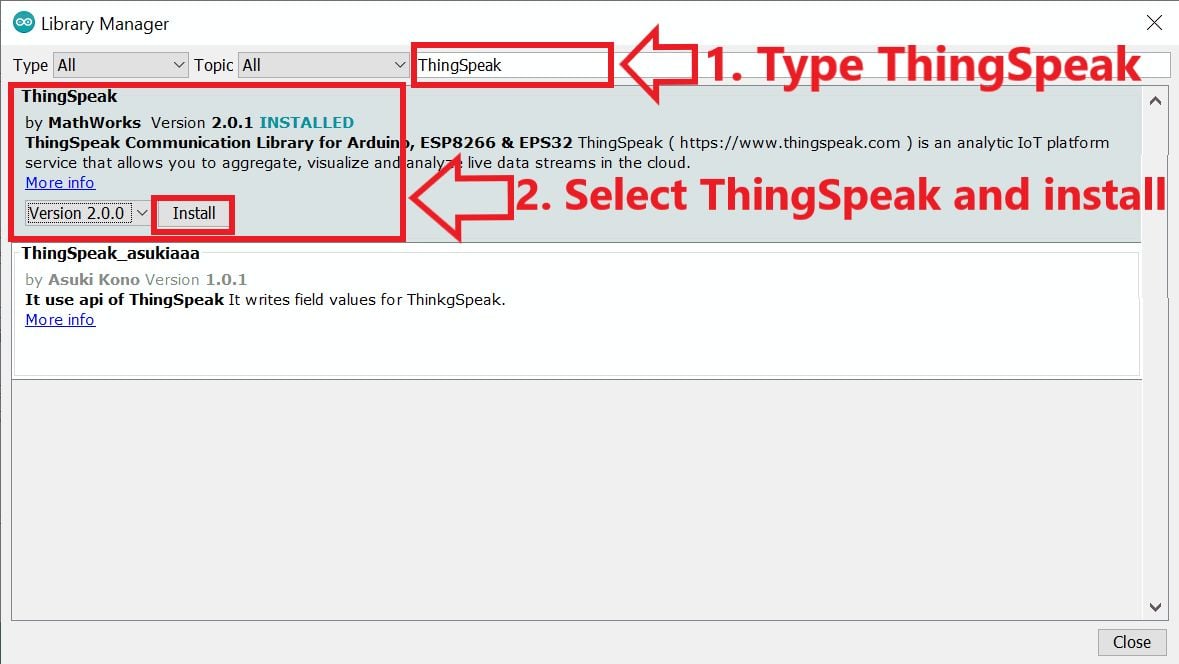
Arduino sketch for HTTP Client Get
- Now open an example of ReadField. To open it navigate to File ► Examples ► ThingSpeak ► ESP32 ► ReadField
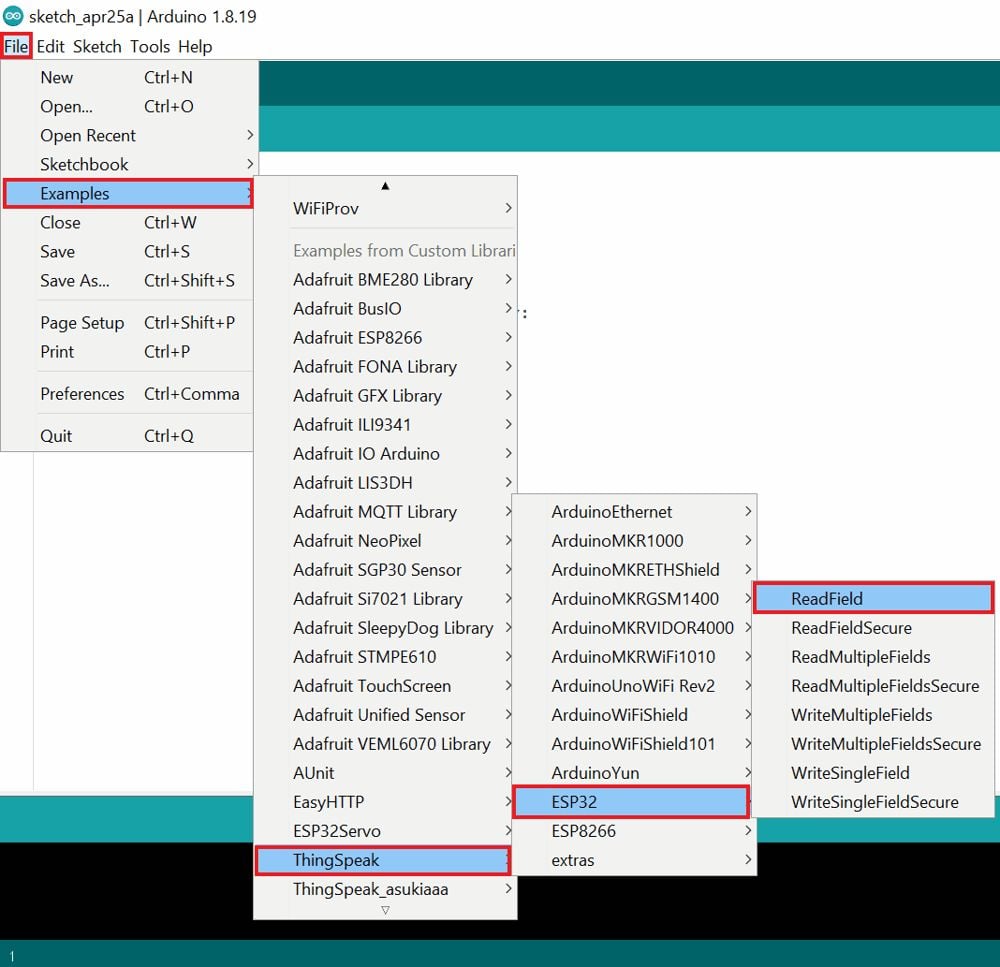
- Now edit the Wi-Fi credentials, ThingSpeak channel ID, and Read API Key with the correct information for example as shown in the below image.
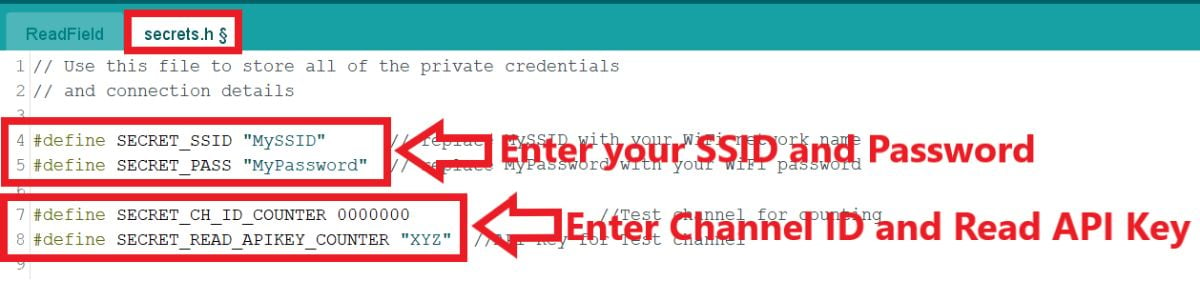
- We have modified the Readfield example as per our above example below
Code:
/*
ReadField
Description: Demonstates reading from a public channel which requires no API key and reading from a private channel which requires a read API key.
The value read from the public channel is the current outside temperature at MathWorks headquaters in Natick, MA. The value from the
private channel is an example counter that increments every 10 seconds.
Hardware: ESP32 based boards
!!! IMPORTANT - Modify the secrets.h file for this project with your network connection and ThingSpeak channel details. !!!
Note:
- Requires installation of EPS32 core. See https://github.com/espressif/arduino-esp32/blob/master/docs/arduino-ide/boards_manager.md for details.
- Select the target hardware from the Tools->Board menu
- This example is written for a network using WPA encryption. For WEP or WPA, change the WiFi.begin() call accordingly.
ThingSpeak ( https://www.thingspeak.com ) is an analytic IoT platform service that allows you to aggregate, visualize, and
analyze live data streams in the cloud. Visit https://www.thingspeak.com to sign up for a free account and create a channel.
Documentation for the ThingSpeak Communication Library for Arduino is in the README.md folder where the library was installed.
See https://www.mathworks.com/help/thingspeak/index.html for the full ThingSpeak documentation.
For licensing information, see the accompanying license file.
Copyright 2020, The MathWorks, Inc.
*/
#include <WiFi.h>
#include "ThingSpeak.h" // always include thingspeak header file after other header files and custom macros
int keyIndex = 0; // your network key Index number (needed only for WEP)
WiFiClient client;
#define SECRET_SSID "****SSID****" // replace MySSID with your WiFi network name
#define SECRET_PASS "****PASSWORD****" // replace MyPassword with your WiFi password
#define SECRET_CH_ID_COUNTER 0000000 //Test channel for counting
#define SECRET_READ_APIKEY_COUNTER " xxxxxxxxxxxxxxxx " //API Key for Test channel
// Counting channel details
unsigned long counterChannelNumber = SECRET_CH_ID_COUNTER;
const char * myCounterReadAPIKey = SECRET_READ_APIKEY_COUNTER;
unsigned int counterFieldNumber = 1;
void setup() {
Serial.begin(115200); //Initialize serial
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo native USB port only
}
WiFi.mode(WIFI_STA);
ThingSpeak.begin(client); // Initialize ThingSpeak
}
void loop() {
int statusCode = 0;
// Connect or reconnect to WiFi
if(WiFi.status() != WL_CONNECTED){
Serial.print("Attempting to connect to SSID: ");
Serial.println(SECRET_SSID);
while(WiFi.status() != WL_CONNECTED){
WiFi.begin(SECRET_SSID, SECRET_PASS); // Connect to WPA/WPA2 network. Change this line if using open or WEP network
Serial.print(".");
delay(5000);
}
Serial.println("\nConnected");
}
// Read in field 1 of the private channel which is a counter
long count = ThingSpeak.readLongField(counterChannelNumber, counterFieldNumber, myCounterReadAPIKey);
// Check the status of the read operation to see if it was successful
statusCode = ThingSpeak.getLastReadStatus();
if(statusCode == 200){
Serial.println("Counter: " + String(count));
}
else{
Serial.println("Problem reading channel. HTTP error code " + String(statusCode));
}
delay(15000); // No need to read the counter too often.
}
Output Window for HTTP Get:
In ThingSpeak feed 1, the last updated value is 34
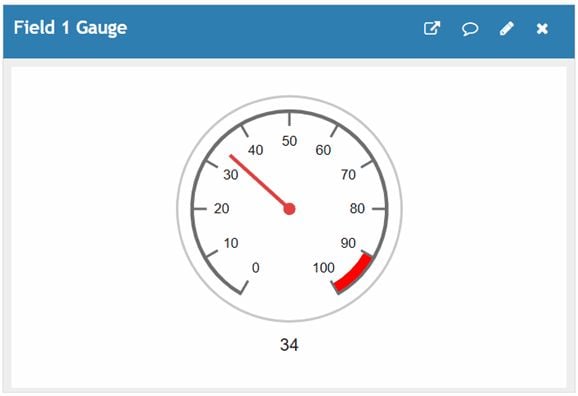
Reading the last updated value from ThingSpeak feed 1
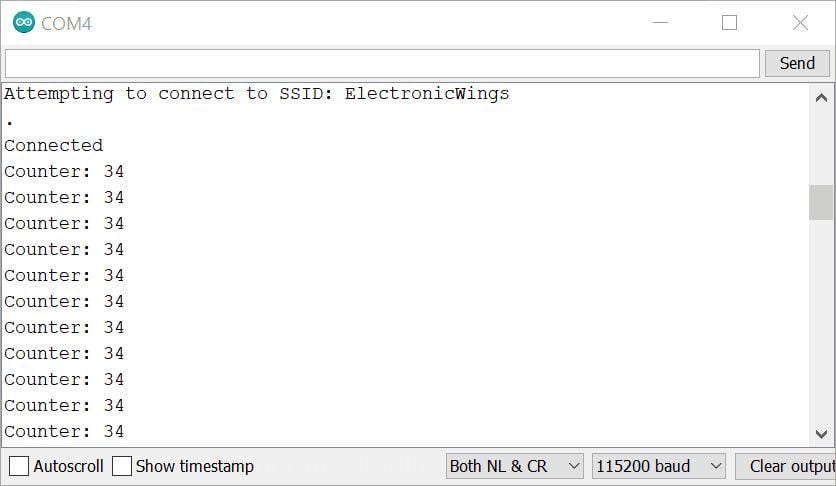
Arduino sketch for HTTP Client Post
- Now open example of WriteSingleField. To open it navigate to File ► Examples ► ThingSpeak ► ESP32 ► WriteSingleField
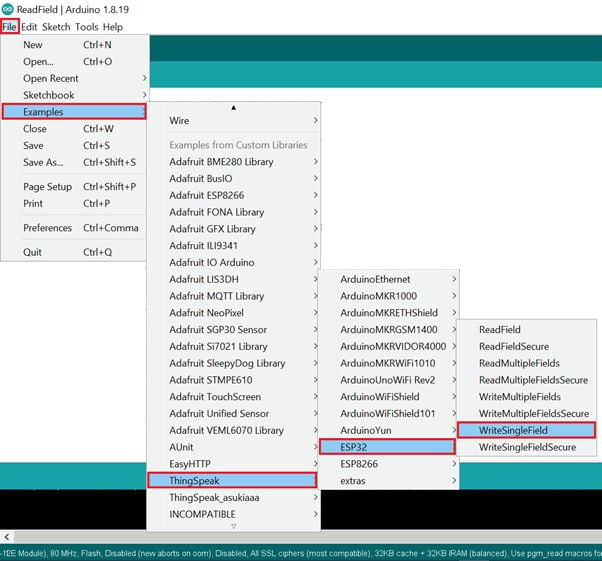
- Now edit the Wi-Fi credentials, ThingSpeak channel ID, and Write API Key with correct information of example as shown in below image.
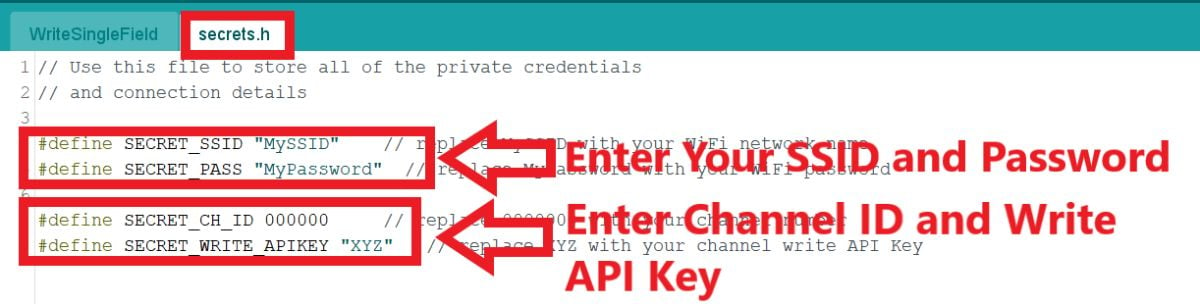
- Now upload the program into your ESP32 development board.
- Or we have modified the Readfield example as per our above example below
Code
/*
WriteSingleField
Description: Writes a value to a channel on ThingSpeak every 20 seconds.
Hardware: ESP32 based boards
!!! IMPORTANT - Modify the secrets.h file for this project with your network connection and ThingSpeak channel details. !!!
Note:
- Requires installation of EPS32 core. See https://github.com/espressif/arduino-esp32/blob/master/docs/arduino-ide/boards_manager.md for details.
- Select the target hardware from the Tools->Board menu
- This example is written for a network using WPA encryption. For WEP or WPA, change the WiFi.begin() call accordingly.
ThingSpeak ( https://www.thingspeak.com ) is an analytic IoT platform service that allows you to aggregate, visualize, and
analyze live data streams in the cloud. Visit https://www.thingspeak.com to sign up for a free account and create a channel.
Documentation for the ThingSpeak Communication Library for Arduino is in the README.md folder where the library was installed.
See https://www.mathworks.com/help/thingspeak/index.html for the full ThingSpeak documentation.
For licensing information, see the accompanying license file.
Copyright 2020, The MathWorks, Inc.
*/
#include <WiFi.h>
#include "ThingSpeak.h" // always include thingspeak header file after other header files and custom macros
#define SECRET_SSID "****SSID****" // replace MySSID with your WiFi network name
#define SECRET_PASS "****PASS****" // replace MyPassword with your WiFi password
#define SECRET_CH_ID 0000000 // replace 0000000 with your channel number
#define SECRET_WRITE_APIKEY "xxxxxxxxxxxxxxxx " // replace XYZ with your channel write API Key
unsigned long myChannelNumber = SECRET_CH_ID;
const char * myWriteAPIKey = SECRET_WRITE_APIKEY;
int number = 0;
int keyIndex = 0; // your network key Index number (needed only for WEP)
WiFiClient client;
void setup() {
Serial.begin(115200); //Initialize serial
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo native USB port only
}
WiFi.mode(WIFI_STA);
ThingSpeak.begin(client); // Initialize ThingSpeak
}
void loop() {
// Connect or reconnect to WiFi
if(WiFi.status() != WL_CONNECTED){
Serial.print("Attempting to connect to SSID: ");
Serial.println(SECRET_SSID);
while(WiFi.status() != WL_CONNECTED){
WiFi.begin(SECRET_SSID, SECRET_PASS); // Connect to WPA/WPA2 network. Change this line if using open or WEP network
Serial.print(".");
delay(5000);
}
Serial.println("\nConnected.");
}
// Write to ThingSpeak. There are up to 8 fields in a channel, allowing you to store up to 8 different
// pieces of information in a channel. Here, we write to field 1.
int x = ThingSpeak.writeField(myChannelNumber, 1, number, myWriteAPIKey);
if(x == 200){
Serial.println("Channel update successful.");
}
else{
Serial.println("Problem updating channel. HTTP error code " + String(x));
}
// change the value
number++;
if(number > 99){
number = 0;
}
delay(20000); // Wait 20 seconds to update the channel again
}
Output Window for HTTP Post
Below is the output window of counts at the ThingSpeak server.
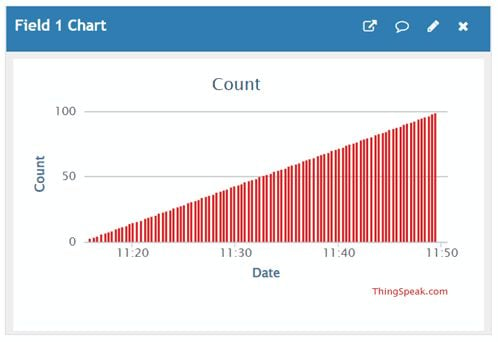
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 |