Overview of DC Motor
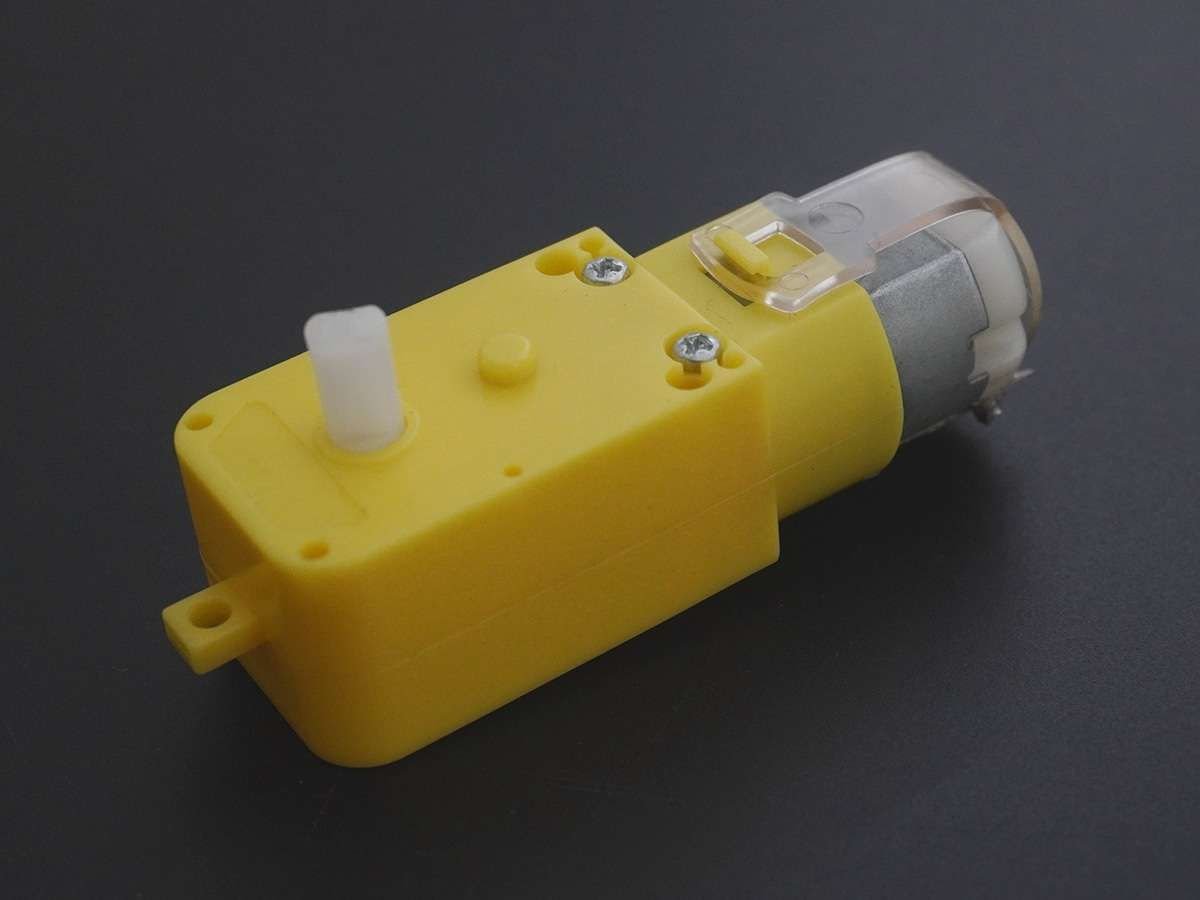
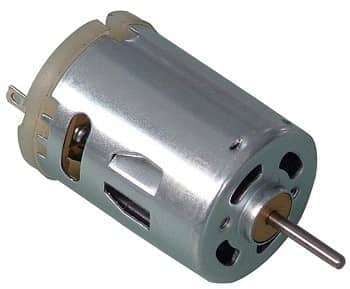
- DC motor converts electrical energy in the form of Direct Current into mechanical energy in the form of rotational motion of the motor shaft.
- The DC motor speed can be controlled by applying varying DC voltage; whereas the direction of rotation of the motor can be changed by reversing the direction of current through it.
- For applying varying voltage, we can make use of the PWM technique.
- For reversing the current, we can make use of an H-Bridge circuit or motor driver ICs that employ the H-Bridge technique.
- For more information about DC motors and how to use them, H-Bridge circuit configurations, and the PWM technique, refer the topic DC Motors in the sensors and modules section.
DC Motor Hardware Connection with ESP32
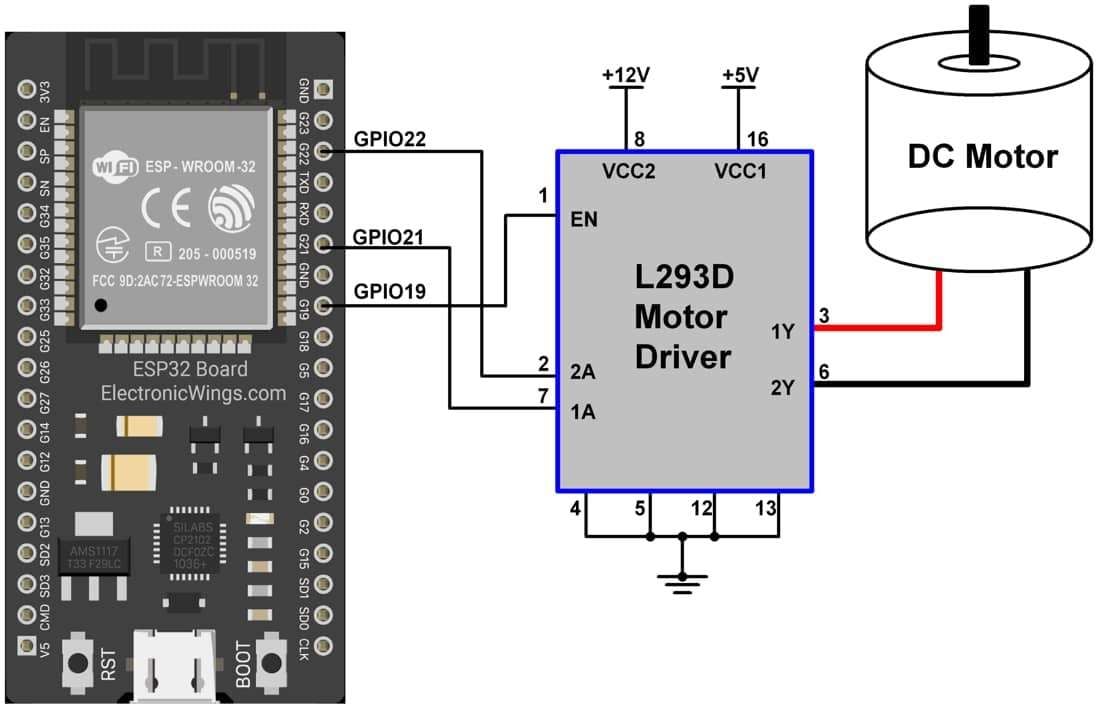
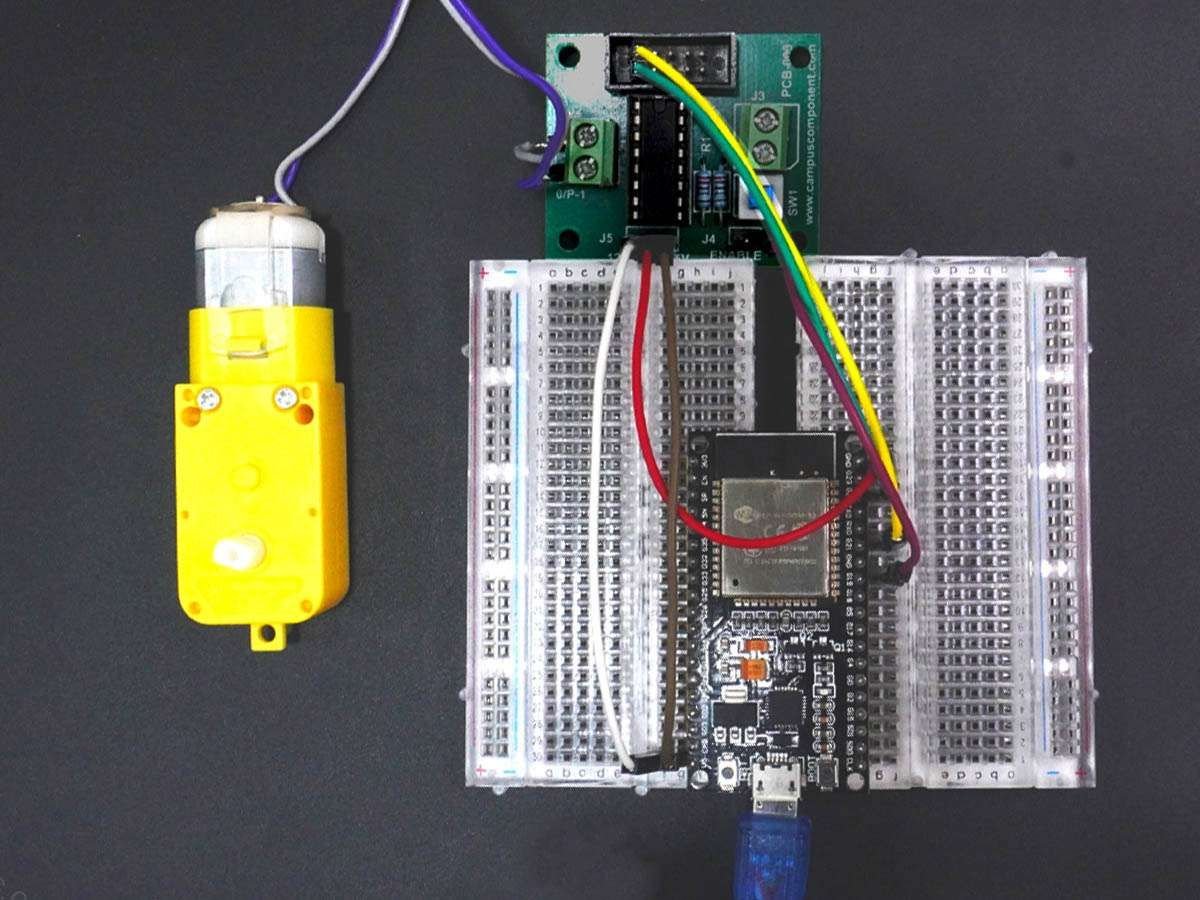
DC Motor Control using ESP32 and Arduino IDE
Here, we are going to change the rotational direction of the DC motor using ESP32.
Here, to change the direction of the motor here we have used the web server.
L293D motor driver IC is used to drive the DC motor.
Now let’s take another example to display the same readings on the web server using the Arduino IDE.
Before uploading the code make sure you have added your SSID and Password as follow.
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
Code for DC Motor Control over Web Server using ESP32
/*
ESP32 DC Motor Control over Web Server
http:://www.electronicwings.com
*/
#include <WiFi.h>
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
WiFiServer server(80); /* Instance of WiFiServer with port number 80 */
String request;
#define M1 21
#define M2 22
#define EN 19
int Motor_Status;
WiFiClient client;
void setup()
{
Serial.begin(115200);
pinMode(M1, OUTPUT);
pinMode(M2, OUTPUT);
pinMode(EN, OUTPUT);
digitalWrite(M1, LOW);
digitalWrite(M2, LOW);
digitalWrite(EN, HIGH);
Serial.print("Connecting to: ");
Serial.println(ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while(WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(100);
}
Serial.print("\n");
Serial.print("Connected to Wi-Fi ");
Serial.println(WiFi.SSID());
delay(1000);
server.begin(); /* Start the HTTP web Server */
Serial.print("Connect to IP Address: ");
Serial.print("http://");
Serial.println(WiFi.localIP());
// Serial.print("\n");
}
void html (){
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head>");
client.println("<meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("<style>");
client.println("html { font-family: Roboto; display: inline-block; margin: 0px auto; text-align: center;}");
client.println(".button {background-color: #4CAF50; border: none; color: white; padding: 15px 32px; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; margin: 4px 2px; cursor: pointer;");
client.println("text-decoration: none; font-size: 25px; margin: 2px; cursor: pointer;}");
client.println(".button_ON {background-color: white; color: black; border: 2px solid #4CAF50;}");
client.println(".button_OFF {background-color: white; color: black; border: 2px solid #f44336;}");
client.println("</style>");
client.println("</head>");
client.println("<body>");
client.println("<h2>DC Motor Control using ESP32</h2>");
client.println("<p>Click to Forward and Reverse the DC Motor</p>");
if(Motor_Status == LOW)
{
client.print("<p><a href=\"/For\n\"><button class=\"button button_ON\">Forward</button></a></p>");
}
else
{
client.print("<p><a href=\"/Rev\n\"><button class=\"button button_OFF\">Reverse</button></a></p>");
}
client.println("</body>");
client.println("</html>");
}
void loop()
{
client = server.available();
if(!client)
{
return;
}
while (client.connected())
{
if (client.available())
{
char c = client.read();
request += c;
if (c == '\n')
{
if (request.indexOf("GET /For") != -1)
{
Serial.println("Motor is Running in Forward Direction");
digitalWrite(M1, HIGH);
digitalWrite(M2, LOW);
Motor_Status = HIGH;
}
if (request.indexOf("GET /Rev") != -1)
{
Serial.println("Motor is Running in Reverse Direction");
digitalWrite(M1, LOW);
digitalWrite(M2, HIGH);
Motor_Status = LOW;
}
html();
break;
}
}
}
delay(1);
request="";
client.stop();
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
- After uploading the code open the serial monitor and set the baud rate to 115200 then reset the ESP32 board and check the IP address as shown in the below image
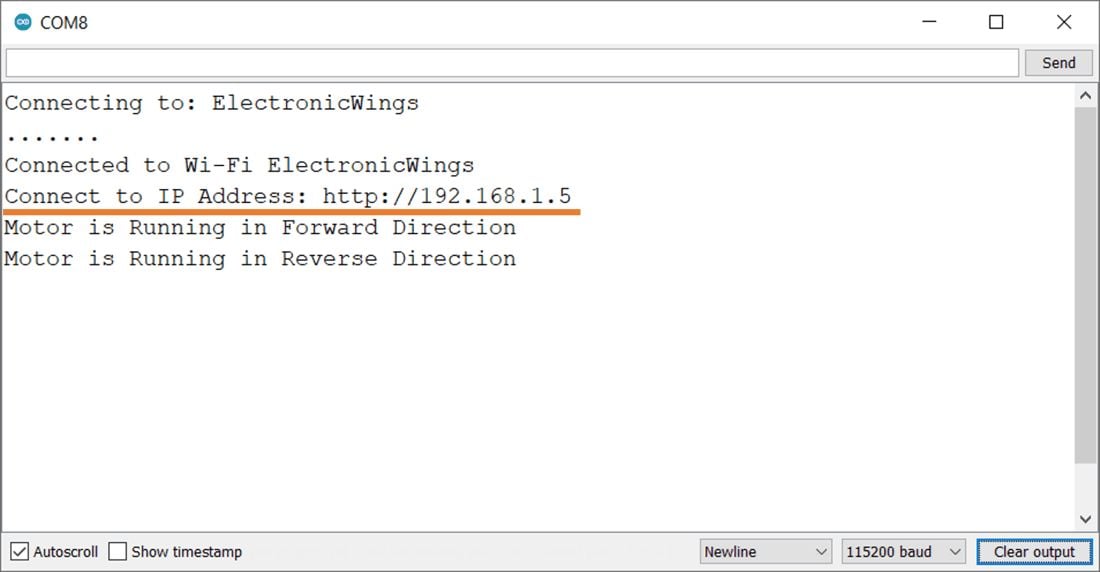
Output on the webserver
- Now open any mobile browser and type the IP address which is shown in the serial monitor and hit the enter button.
- If all are ok, then the web page will start showing the motor control page on the web server like in the below image.
Note: make sure your ESP32 and mobile are connected to the same router/server, if they are connected to the same router or server then only you will be able to visible the web page.
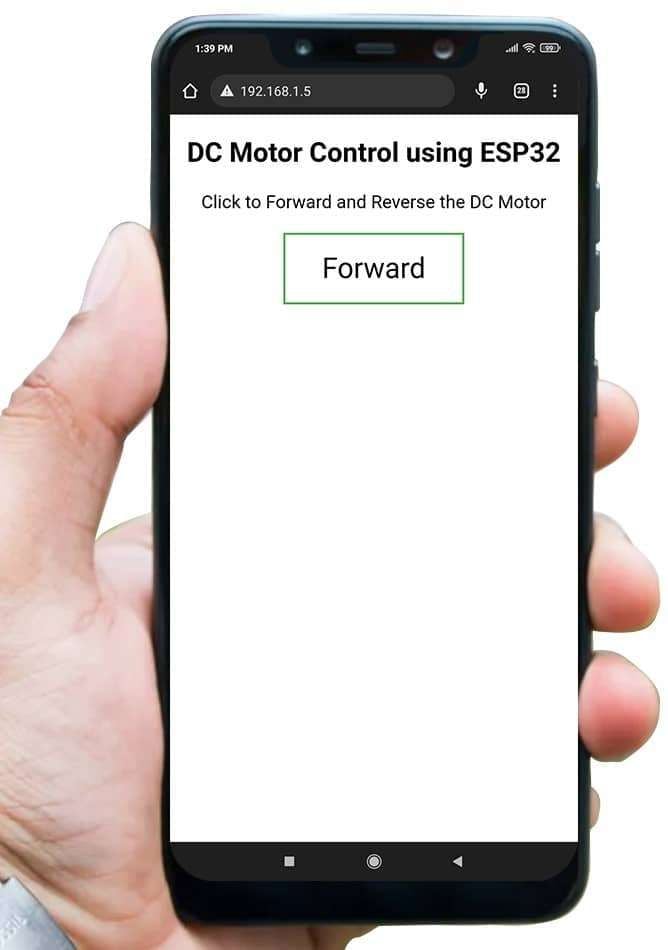
Let’s Understand the code.
To understand this code, please refer to the basics guide of “How to create the ESP32 Server”.
Once you get the basics of ESP32 server creation, it will be very simple to understand the code.
This code starts with important header files and libraries, In WiFi.h
file contains all ESP32 WiFi related definitions, here we have used them for network connection purposes.
#include <WiFi.h>
Let’s define HTTP port i.e., Port 80 as follows
WebServer server(80);
Set the GPIO pins of ESP32 for the DC Motor and define the variables.
String request;
#define M1 21
#define M2 22
#define EN 19
int Motor_Status;
Create the object for the WiFiClient class
WiFiClient client;
Setup Function
In the setup function, first configure all motor control pins as an OUTPUT mode, and the direction control pins are set to LOW (turn off the DC motor) and enable pin set HIGH for motor driver IC.
pinMode(M1, OUTPUT);
pinMode(M2, OUTPUT);
pinMode(EN, OUTPUT);
digitalWrite(M1, LOW);
digitalWrite(M2, LOW);
digitalWrite(EN, HIGH);
We set the WiFi as an STA mode and connect to the given SSID and password
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");}
After successfully connecting to the server print the local IP address on the serial window.
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
Now start the server using server.begin()
function.
server.begin(); /*Start Server*/
Loop Function
In the loop function, we will read the client request and execute the program accordingly.
The ESP32 always listens to the incoming clients using server.available()
function.
client = server.available();
if(!client)
{
return;
}
When a request is received from a client, we’ll save the incoming data.
The while loop that follows will be running as long as the client stays connected.
while (client.connected())
Now read the client’s request and check which button was pressed on your web page, and control the DC motor direction accordingly.
Here if receive For then the motor will rotate in the forward direction and if receive Rev then the motor will rotate in the reverse direction.
if (client.available())
{
char c = client.read();
request += c;
if (c == '\n')
{
if (request.indexOf("GET /For") != -1)
{
Serial.println("Motor is Running in Forward Direction");
digitalWrite(M1, HIGH);
digitalWrite(M2, LOW);
Motor_Status = HIGH;
}
if (request.indexOf("GET /Rev") != -1)
{
Serial.println("Motor is Running in Reverse Direction");
digitalWrite(M1, LOW);
digitalWrite(M2, HIGH);
Motor_Status = LOW;
}
html();
break;
}
}
Let’s understand the html code step by step
All html pages start with the <!DOCTYPE html>
declaration, it is just information to the browser about what type of document is expected.
client.println("<!DOCTYPE HTML>");
The html tag is the container of the complete html page which represents on the top of the html code.
client.println("<html>");
Now here we are defining the style information for a web page using the <style>
tag. Inside the style tag, we have defined the font name, size, color, and test alignment.
client.println("<style>");
client.println("html { font-family: Roboto; display: inline-block; margin: 0px auto; text-align: center;}");
client.println(".button {background-color: #4CAF50; border: none; color: white; padding: 15px 32px; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; margin: 4px 2px; cursor: pointer;");
client.println("text-decoration: none; font-size: 25px; margin: 2px; cursor: pointer;}");
client.println(".button_ON {background-color: white; color: black; border: 2px solid #4CAF50;}");
client.println(".button_OFF {background-color: white; color: black; border: 2px solid #f44336;}");
client.println("</style>");
Inside the body, we are defining the document body, in below we have used headings, and paragraphs if you want you can add images, hyperlinks, tables, lists, etc. also.
On the web page, we are displaying the heading of the page, and inside a paragraph, instruction to control the DC motor.
client.println("<body>");
client.println("<h2>DC Motor Control using ESP32</h2>");
client.println("<p>Click to Forward and Reverse the DC Motor</p>");
Change the button according to the motor direction state
Here check the motor direction and change the button name and style according.
In the below code if the motor is rotating in the forward direction, then the button will display Reverse otherwise display Forward
if(Motor_Status == LOW)
{
client.print("<p><a href=\"/For\n\"><button class=\"button button_ON\">Forward</button></a></p>");
}
else
{
client.print("<p><a href=\"/Rev\n\"><button class=\"button button_OFF\">Reverse</button></a></p>");
}
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 | |
DC Toy or Hobby Motor Adafruit Accessories DC Toy/Hobby Motor 130 size |
X 1 |
Downloads |
||
---|---|---|
|
ESP32_DC_Motor | Download |