Overview of MQ6 Gas Sensor
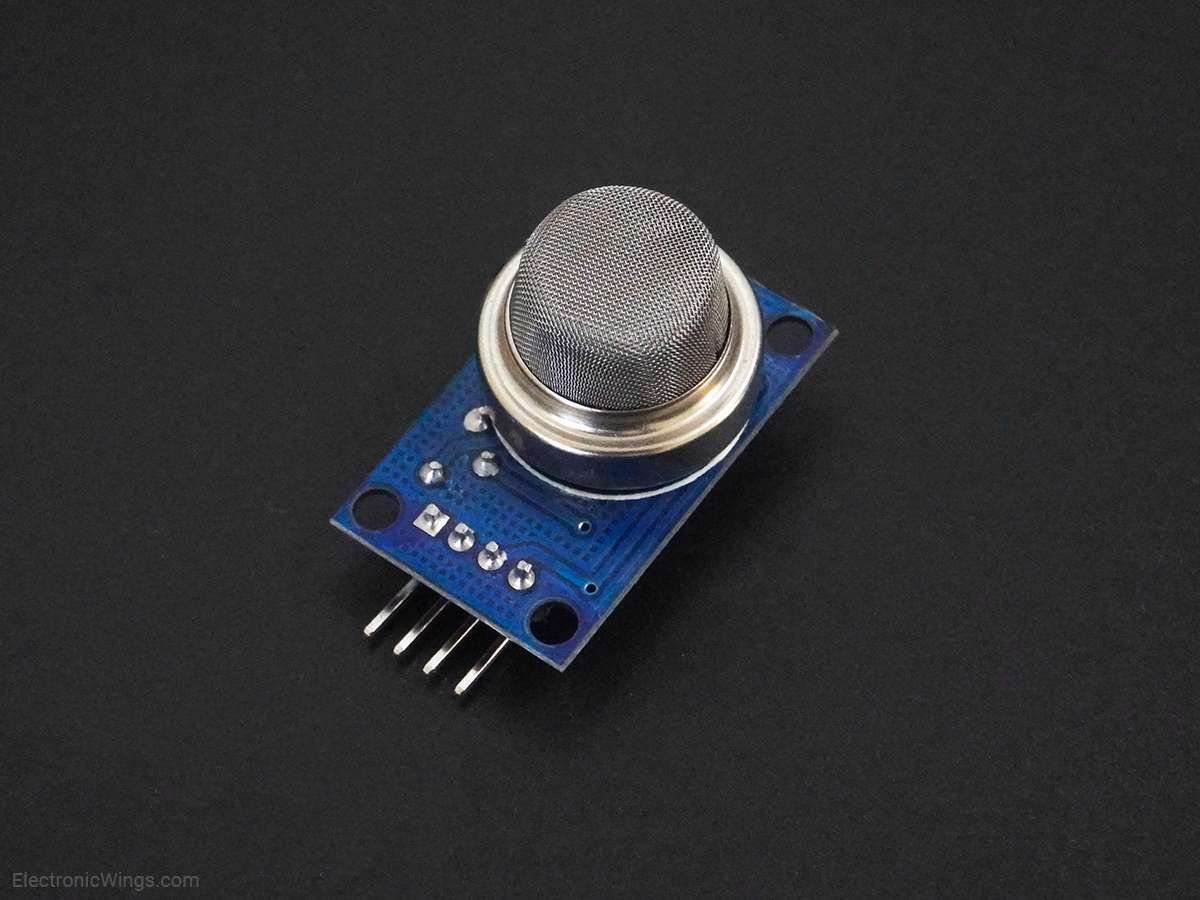
.jpg)
- MQ6 is a Gas Sensor that measures or detects the LPG, iso-butane, propane, and LNG. They also have a small sensitivity to alcohol and smoke.
- This sensor detects the gas in the air and gives an output reading in analog voltage.
- The standard sensitivity working temperature is 20℃±2℃ and Humidity is 65%±5%.
- The resistance of the sensor varies depending on the amount of gas.
Detectable Gas:
Gas | Range |
LPG, Isobutane, Propane, LNG | 200-10000ppm |
Where, ppm: parts per million
They can be used in applications such as domestic gas leakage detection, industrial gas detection, and portable gas detection.
MQ6 Pinout and Description
MQ6 has 6 pins, 4 of them are used to fetch signals, and the other 2 are Heater coils used for providing heating current.
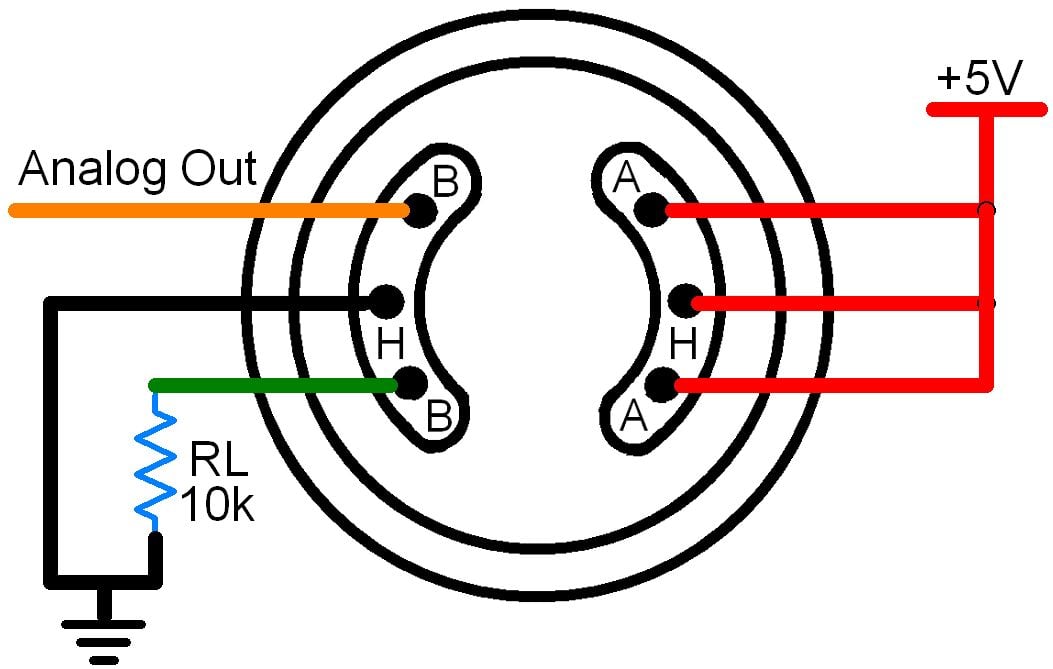
The required voltage is 5V DC or AC.
Pin H is a heater pin that goes to the power and the other one is connected to the ground.
Pin A (you can connect both pins A) is connected between the power and the ground.
Pin B gets an analog voltage when the sensor is active. Also, across the output, you need the resistor RL.
MQ6 Hardware Connection with ESP32
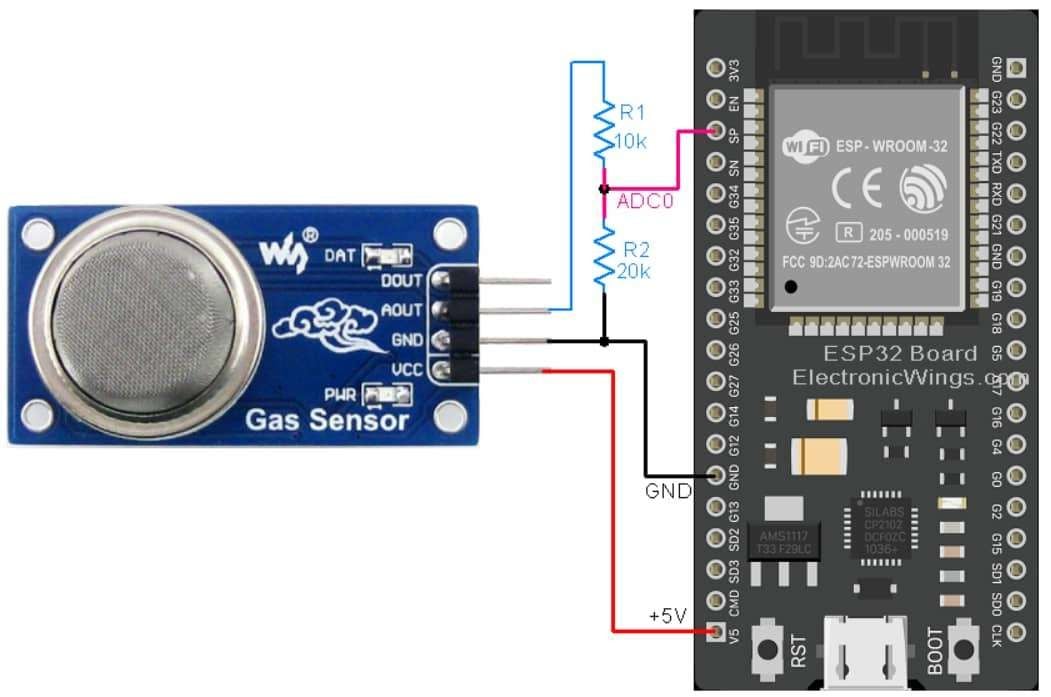
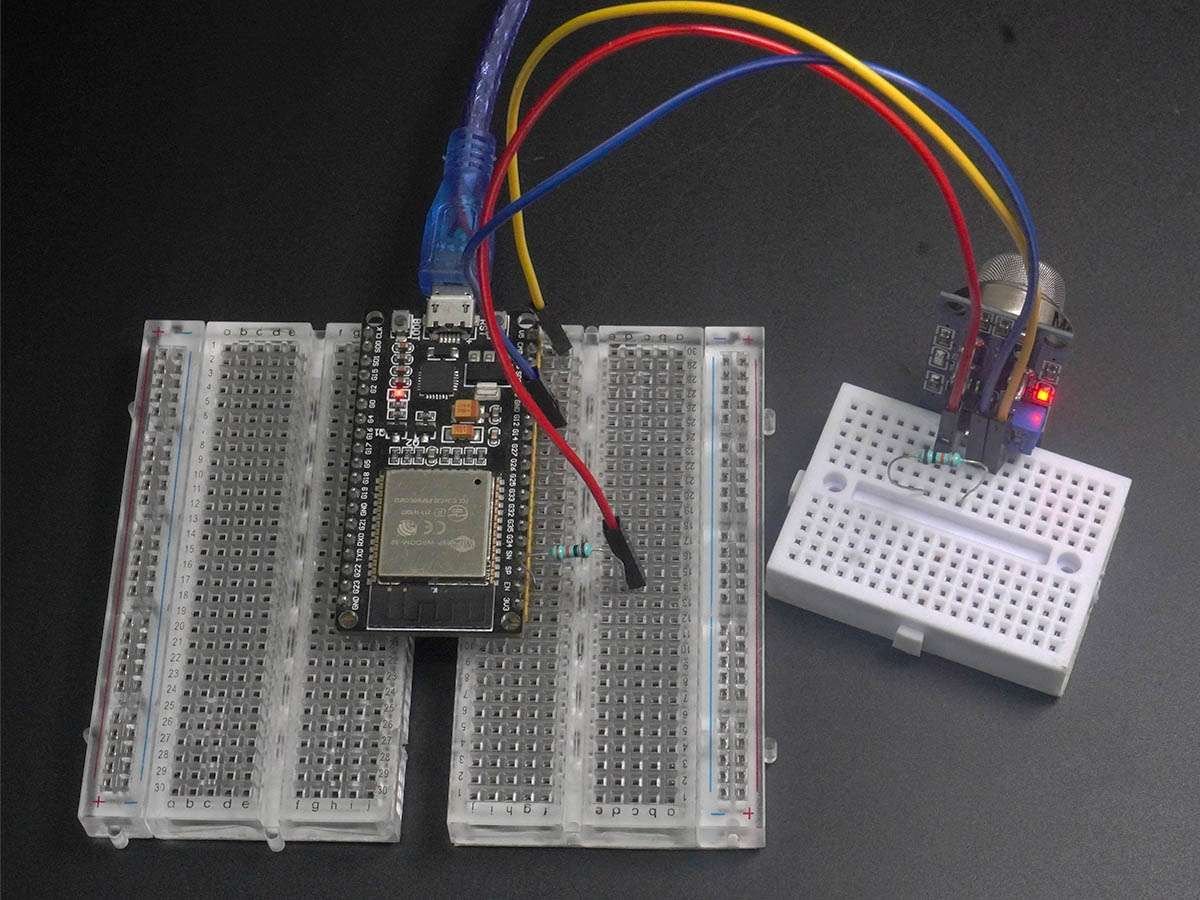
MQ6 PPM Calculations
- Get the ADC value and convert it into voltage using the below equation
Where,
Reference Voltage for ESP32 = 3.3V
ADC Resolution for ESP32 = 4096
- Calculate the Rs from the below formula
Where,
RL = 1k ohm which is present in our MQ6 Module
- Calculate the Ro from the below formula
Where,
10 is the clean air factor
- Calculate the LPG PPM from the below formula
Where,
RsRo_Ration = Rs/Ro
Code for MQ6 PPM Reading using ESP32
/*
ESP32 MQ6 LPG Gas and PPM Calculation
http:://www.electronicwings.com
*/
byte MQ6_Pin = A0; /* Define A0 for MQ Sensor Pin */
float Referance_V = 3300.0; /* ESP32 Referance Voltage in mV */
float RL = 1.0; /* In Module RL value is 1k Ohm */
float Ro = 10.0; /* The Ro value is 10k Ohm */
float mVolt = 0.0;
const float Ro_clean_air_factor = 10.0;
void setup() {
Serial.begin(9600); /* Set baudrate to 9600 */
pinMode(MQ6_Pin, INPUT); /* Define A0 as a INPUT Pin */
delay(500);
Serial.println("Wait for 30 sec warmup");
delay(30000); /* Set the warmup delay wich is 30 Sec */
Serial.println("Warmup Complete");
for(int i=0; i<30; i++){
mVolt += Get_mVolt(MQ6_Pin);
}
mVolt = mVolt /30.0; /* Get the volatage in mV for 30 Samples */
Serial.print("Voltage at A0 Pin = ");
Serial.print(mVolt);
Serial.println("mVolt");
Serial.print("Rs = ");
Serial.println(Calculate_Rs(mVolt));
Ro = Calculate_Rs(mVolt) / Ro_clean_air_factor;
Serial.print("Ro = ");
Serial.println(Ro);
Serial.println(" ");
mVolt = 0.0;
}
void loop() {
for(int i=0; i<500; i++){
mVolt += Get_mVolt(MQ6_Pin);
}
mVolt = mVolt/500.0; /* Get the volatage in mV for 500 Samples */
Serial.print("Voltage at A0 Pin = ");
Serial.print(mVolt); /* Print the mV in Serial Monitor */
Serial.println(" mV");
float Rs = Calculate_Rs(mVolt);
Serial.print("Rs = ");
Serial.println(Rs); /* Print the Rs value in Serial Monitor */
float Ratio_RsRo = Rs/Ro;
Serial.print("RsRo = ");
Serial.println(Ratio_RsRo);
Serial.print("LPG ppm = ");
unsigned int LPG_ppm = LPG_PPM(Ratio_RsRo);
Serial.println(LPG_ppm); /* Print the Gas PPM value in Serial Monitor */
Serial.println("");
mVolt = 0.0; /* Set the mVolt variable to 0 */
}
float Calculate_Rs(float Vo) {
/*
* Calculate the Rs value
* The equation Rs = (Vc - Vo)*(RL/Vo)
*/
float Rs = (Referance_V - Vo) * (RL / Vo);
return Rs;
}
unsigned int LPG_PPM(float RsRo_ratio) {
/*
* Calculate the PPM using below equation
* LPG ppm = [(Rs/Ro)/18.446]^(1/-0.421)
*/
float ppm;
ppm = pow((RsRo_ratio/18.446), (1/-0.421));
return (unsigned int) ppm;
}
float Get_mVolt(byte AnalogPin) {
/* Calculate the ADC Voltage using below equation
* mVolt = ADC_Count * (ADC_Referance_Voltage / ADC_Resolution)
*/
int ADC_Value = analogRead(AnalogPin);
delay(1);
float mVolt = ADC_Value * (Referance_V / 4096.0);
return mVolt;
}
ESP32 serial monitor output for MQ6
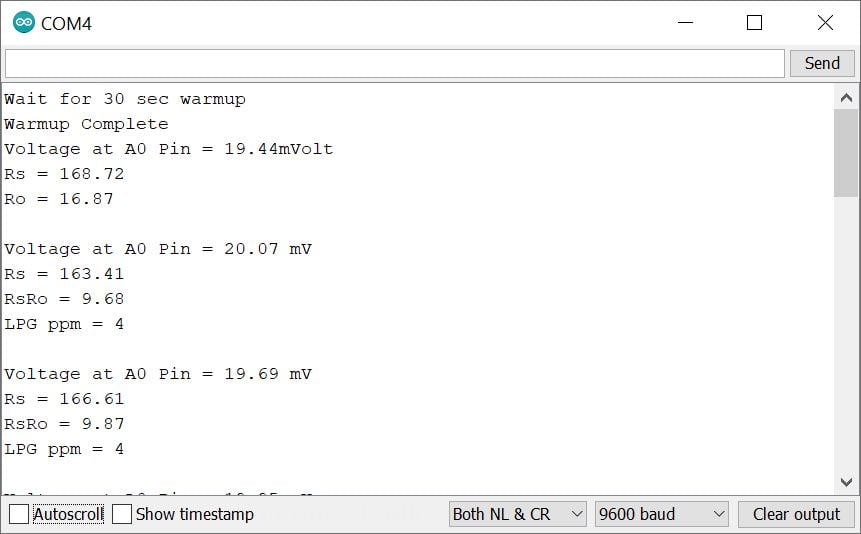
Let’s Understand the code
The code is begin by defining the analog input pin which is A0
byte MQ6_Pin = A0; /* Define A0 for MQ Sensor Pin */
Set the parameters for MQ6 and ESP32.
float Referance_V = 3300.0; /* ESP32 Referance Voltage in mV */
float RL = 1.0; /* In Module RL value is 1k Ohm */
float Ro = 10.0; /* The Ro value is 10k Ohm */
float mVolt = 0.0;
constfloat Ro_clean_air_factor =10.0;
In setup function
We have initiated the serial communication with a 115200 Baud rate.
Serial.begin(9600); /* Set baudrate to 9600 */
Set the MQ6 Sensor pin as an INPUT Mode
pinMode(MQ6_Pin, INPUT); /* Define A0 as a INPUT Pin */
delay(500);
Now wait for the warmup it will take 30 Sec for a complete warmup
Serial.println("Wait for 30 sec warmup");
delay(30000); /* Set the warmup delay wich is 30 Sec */
Serial.println("Warmup Complete");
Now measure the output voltage of the MQ6 sensor using Get_mVolt()
function. While getting this voltage kept your MQ6 sensor in the fresh air. In this measurement, we are taking 30 samples and averaging them.
for(int i=0; i<30; i++){
mVolt += Get_mVolt(MQ6_Pin);
}
mVolt = mVolt /30.0; /* Get the voltage in mV for 30 Samples */
Serial.print("Voltage at A0 Pin = ");
Serial.print(mVolt);
Serial.println("mVolt");
Now get the Rs value from Calculate_Rs()
function
Serial.print("Rs = ");
Serial.println(Calculate_Rs(mVolt));
Then calculate the Ro
Ro = Calculate_Rs(mVolt) / Ro_clean_air_factor;
Serial.print("Ro = ");
Serial.println(Ro);
Serial.println(" ");
mVolt = 0.0;
In loop function
In the loop, we will read the MQ6 output voltage, Rs, the ratio of RsRo, and then the PPM of the LPG gas sensor.
Now first read the MQ6 output voltage using Get_mVolt()
function and print on the serial monitor. All other readings depend on the MQ6 Output voltage.
for(int i=0; i<500; i++){
mVolt += Get_mVolt(MQ6_Pin);
}
mVolt = mVolt/500.0; /* Get the volatage in mV for 500 Samples */
Serial.print("Voltage at A0 Pin = ");
Serial.print(mVolt); /* Print the mV in Serial Monitor */
Serial.println(" mV");
Here calculate the Rs resistance using Calculate_Rs()
function and calculate the ratio of RsRo and print on the serial monitor.
float Rs = Calculate_Rs(mVolt);
Serial.print("Rs = ");
Serial.println(Rs); /* Print the Rs value in Serial Monitor */
float Ratio_RsRo = Rs/Ro;
Serial.print("RsRo = ");
Serial.println(Ratio_RsRo);
LPG_PPM()
function is used to calculate the PPM of LPG gas sensor and print on the serial monitor.
Serial.print("LPG ppm = ");
unsigned int LPG_ppm = LPG_PPM(Ratio_RsRo);
Serial.println(LPG_ppm); /* Print the Gas PPM value in Serial Monitor */
Other function
This function calculates the Rs value as per the above formula and return the calculated Rs value
float Calculate_Rs(float Vo) {
/*
* Calculate the Rs value
* The equation Rs = (Vc - Vo)*(RL/Vo)
*/
float Rs = (Referance_V - Vo) * (RL / Vo);
return Rs;
}
Calculate and measure the LPG gas in PPM using the RsRo ratio by using the LPG_PPM()
function.
unsigned int LPG_PPM(float RsRo_ratio) {
/*
* Calculate the PPM using below equation
* LPG ppm = [(Rs/Ro)/18.446]^(1/-0.421)
*/
float ppm;
ppm = pow((RsRo_ratio/18.446), (1/-0.421));
return (unsigned int) ppm;
}
To calculate the voltage in mV from the ADC reading and return using the below Get_mVolt()
function.
float Get_mVolt(byte AnalogPin) {
/* Calculate the ADC Voltage using below equation
* mVolt = ADC_Count * (ADC_Referance_Voltage / ADC_Resolution)
*/
int ADC_Value = analogRead(AnalogPin);
delay(1);
float mVolt = ADC_Value * (Referance_V / 4096.0);
return mVolt;
}
Components Used |
||
---|---|---|
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 | |
MQ6 Gas Sensor MQ6 is a gas leakage detection sensor. |
X 1 |
Downloads |
||
---|---|---|
|
ESP32_MQ6 | Download |