Description
What is WhatsApp?
- As we all know, WhatsApp is a popular messaging application that allows users to send text messages, voice messages, make voice and video calls, share multimedia files such as photos and videos, and create group chats.
- It was developed by WhatsApp Inc.
- WhatsApp is available for smartphones running various operating systems, including iOS, Android, and Windows Phone.
Application:
- We can set the ESP32 to send Alterts, Sensor data, notifications etc. directly on the WhatsApp.
- We can send message via WhatsApp to ESP32 for certain actions, i.e. Light control, Appliances control,etc.
Now Let’s start.
Step 1
Visit the CallMeBot website (www.callmebot.com).
Click on Free WhatsApp API and then click on Send Messages
.png)
Now let’s do the setup as given in CallMeBot Website below:
- Add the phone number +34 611 04 87 48 to your Phone Contacts. (Name it as you wish)
- Send this message "I allow callmebot to send me messages" to the new Contact created (using WhatsApp of course)
- Wait until you receive the message "API Activated for your phone number. Your APIKEY is 123123" from the bot.
Note: If you don't receive the ApiKey in 2 minutes, please try again after 24hs.
.png)
.jpg)
Send the text message to WhatsApp using ESP32
Let’s install the required library.
Here we are using URLEncode libraries for the above example. We need to install the URLEncode library using the Arduino Library Manager.
- Open the Arduino IDE
- Navigate to Sketch ► Include Library ► Manage Libraries…
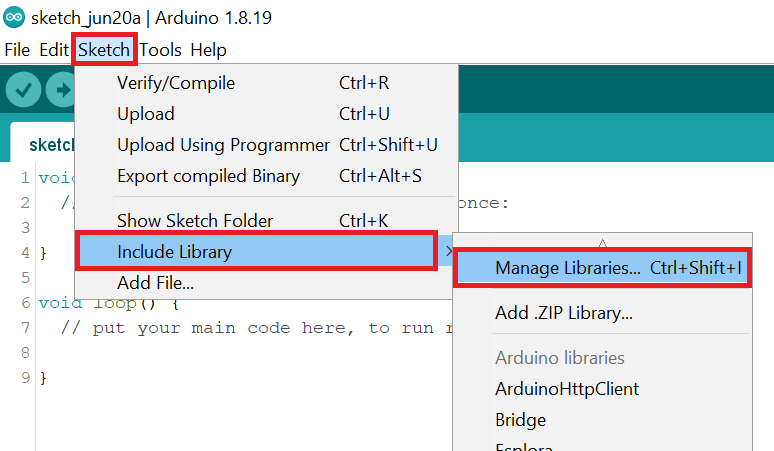
- The library Manager window will pop up. Now enter URLEncode into the search box, and click Install on the URLEncode option to install version 1.0.1 or higher. As shown below image.
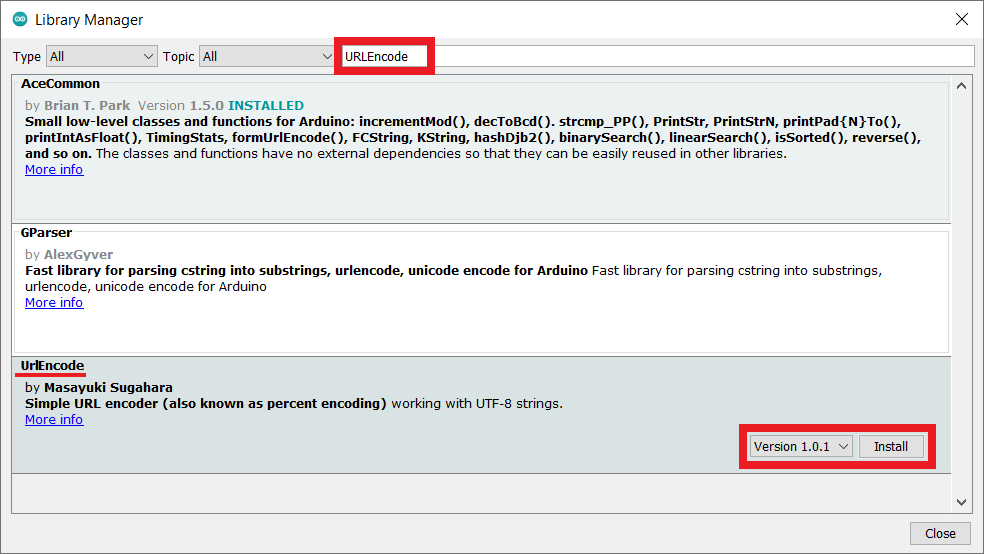
Send WhatsApp messages using ESP32
ESP32 has oh-chip Wi-Fi, we can utilize it and monitor the readings over our smartphone, Laptop, or even smart TV. This can offer much more flexibility and real world applications.
We can use the ESP32 web server to monitor the Temperature.
Let’s send the Hello World! Message to the WhatsApp using the ESP32 and Arduino IDE.
Before uploading the code make sure you have added your SSID, Password, Mobile Number, and API Key as follows.
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASS";
String MobileNumber = "ADD_YOUR_PHONE_NUMBER";
String APIKey = "ADD_YOUR_API_KEY";
WhatsApp Message code for ESP32
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASS";
String MobileNumber = "ADD_YOUR_PHONE_NUMBER";
String APIKey = "ADD_YOUR_API_KEY";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println("Connecting to WiFi");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP());
delay(1000);
sendMessage("Hello World!");
}
void loop() {}
void sendMessage(String message){
String url = "https://api.callmebot.com/whatsapp.php?phone=" + MobileNumber + "&apikey=" + APIKey + "&text=" + urlEncode(message);
HTTPClient http;
http.begin(url);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int httpResponseCode = http.POST(url);
if (httpResponseCode == 200){
Serial.print("Message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(httpResponseCode);
}
http.end();
}
Output
.jpg)
Downloads |
||
---|---|---|
|
ESP32_whatsapp | Download |