Description
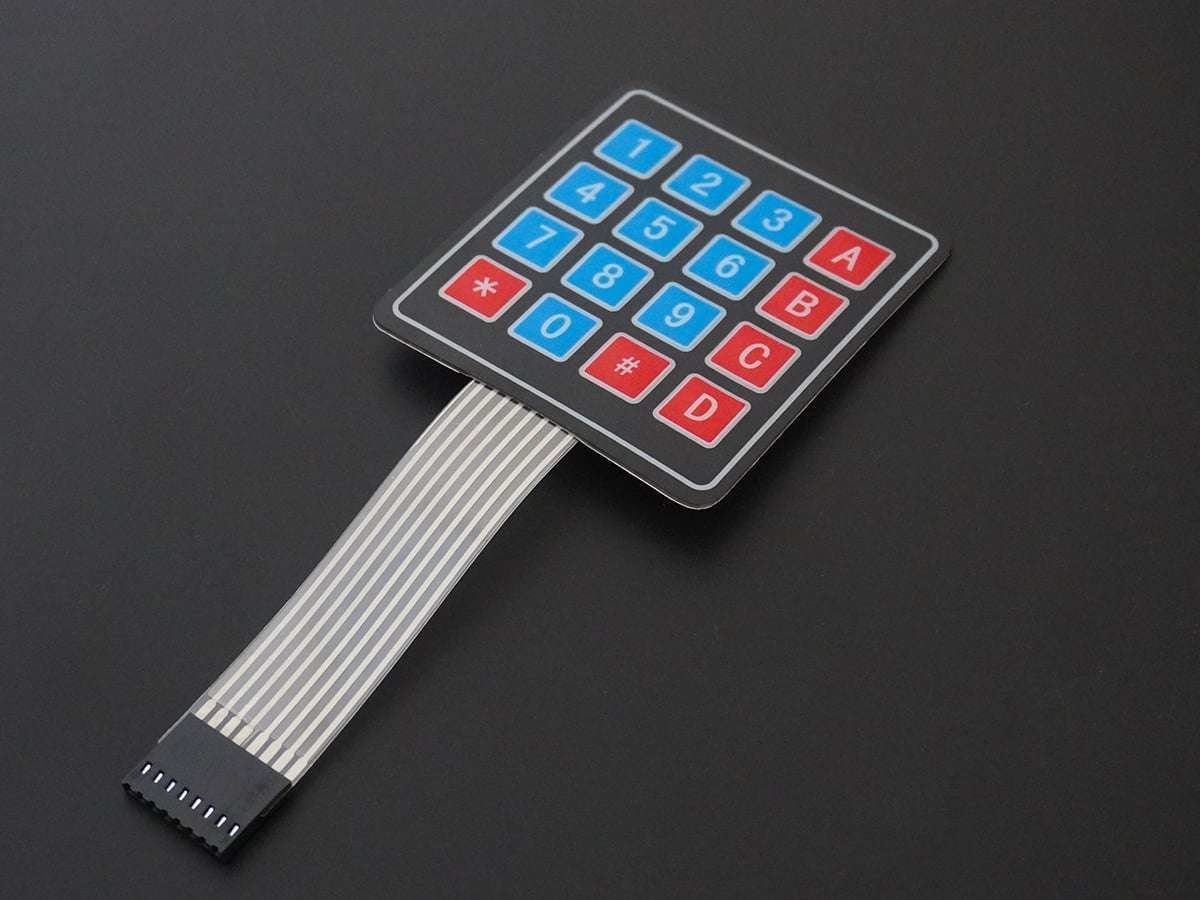
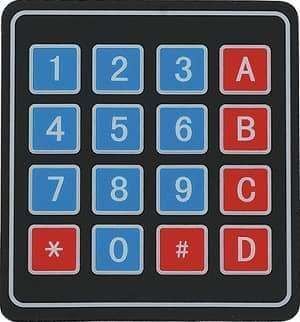
- A keypad is used as an input device to read and process the user's keystrokes.
- In the A 4x4 keypad contains four rows and four columns.
- Between the rows and columns, switches are positioned.
- By pressing the corresponding key establishes a connection between the row and column are connected of that switch.
- To know more information about the 4x4 keypad refer topic 4x4 Keypad in the sensors and modules section.
4x4 Matrix Keypad Hardware Connection with NodeMCU
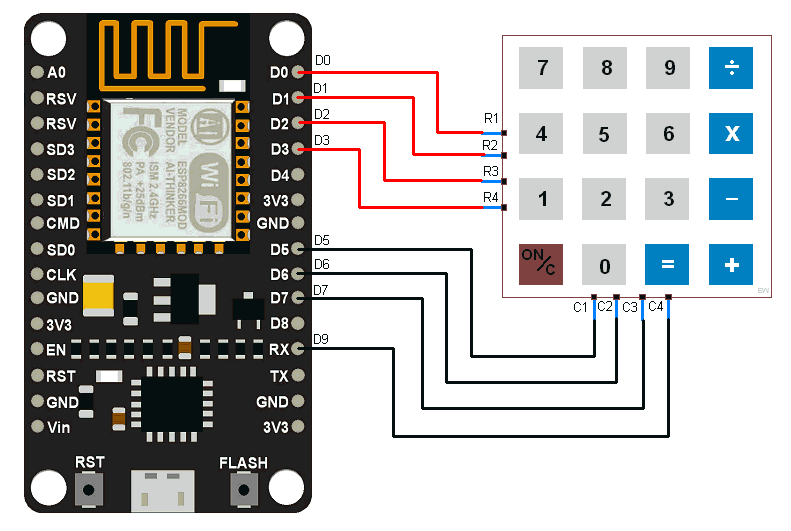
.jpg)
Read values using Keypad and NodeMCU
Reading a key press from a 4x4 keypad and showing it on the Arduino's serial terminal.
Here, we will be using the Keypad library by Mark Stanley and Alexander Brevig.
Download it from here
Extract the library and add it to the libraries folder path of Arduino IDE.
For more information about how to add a custom library to the Arduino IDE and use examples from it, refer Adding Library To Arduino IDE in the Basics section.
Now Open the Arduino IDE after the library has been added,
Now open an example of Adafruit ADXL345 io dashboard. To open it navigate to File ► Examples ► Keypad ► CustomKeypad
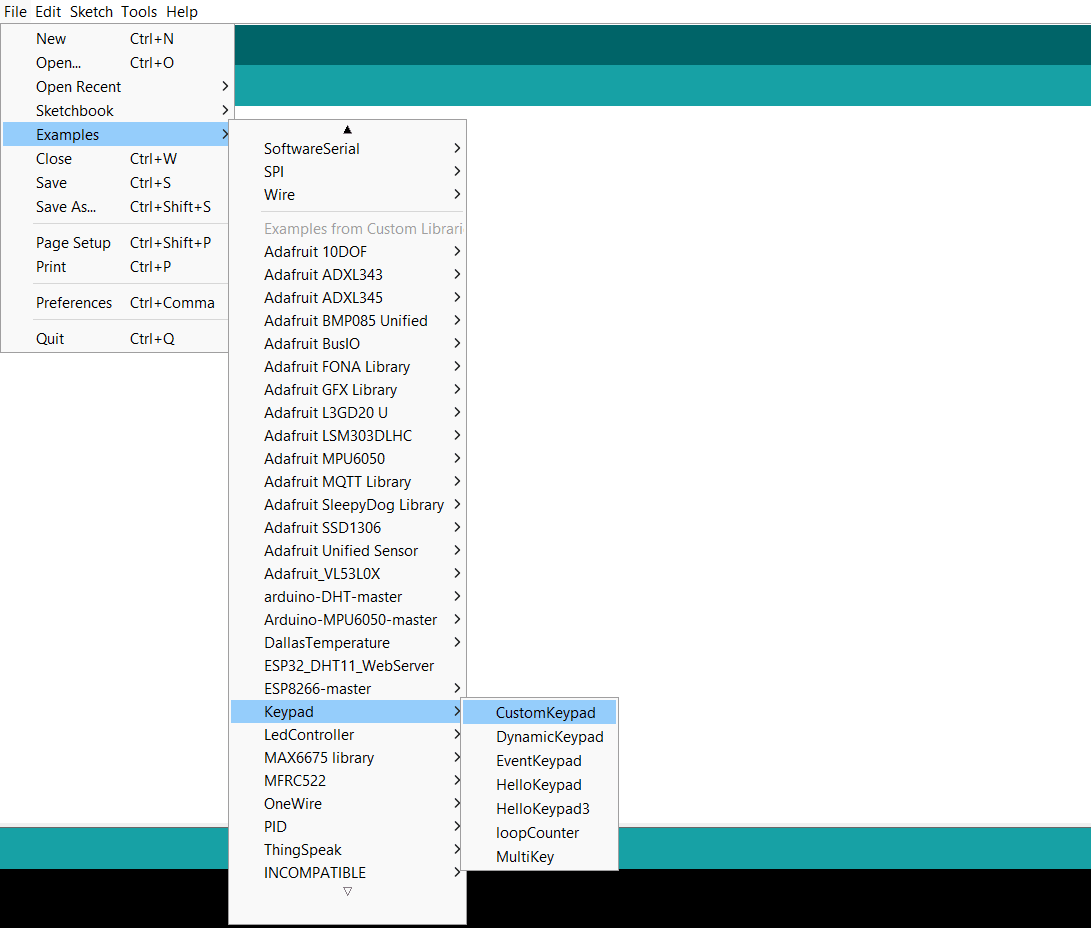
Here we have used the same example below
Note: It should be noted that if the pins are not connected in accordance with this function, the key press recognition won't produce results that match the keypad you've specified.
byte rowPins[ROWS] = {R1, R2, R3, R4}; /* connect to the row pinouts of the keypad */
byte colPins[COLS] = {C1, C2, C3, C4}; /* connect to the column pinouts of the keypad */
4x4 Matrix Keypad Code for NodeMCU
/* @file CustomKeypad.pde
|| @version 1.0
|| @author Alexander Brevig
|| @contact [email protected]
||
|| @description
|| | Demonstrates changing the keypad size and key values.
|| #
*/
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
//define the cymbols on the buttons of the keypads
char hexaKeys[ROWS][COLS] = {
{'0','1','2','3'},
{'4','5','6','7'},
{'8','9','A','B'},
{'C','D','E','F'}
};
byte rowPins[ROWS] = {D0,D1,D2,D3}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {D5,D6,D7,D9}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
void setup(){
Serial.begin(9600);
}
void loop(){
char customKey = customKeypad.getKey();
if (customKey){
Serial.println(customKey);
}
}
- Now upload the code on NodeMCU.
- After uploading the code open the serial monitor and set the baud rate to 9600 to see the output.
Output on the serial monitor
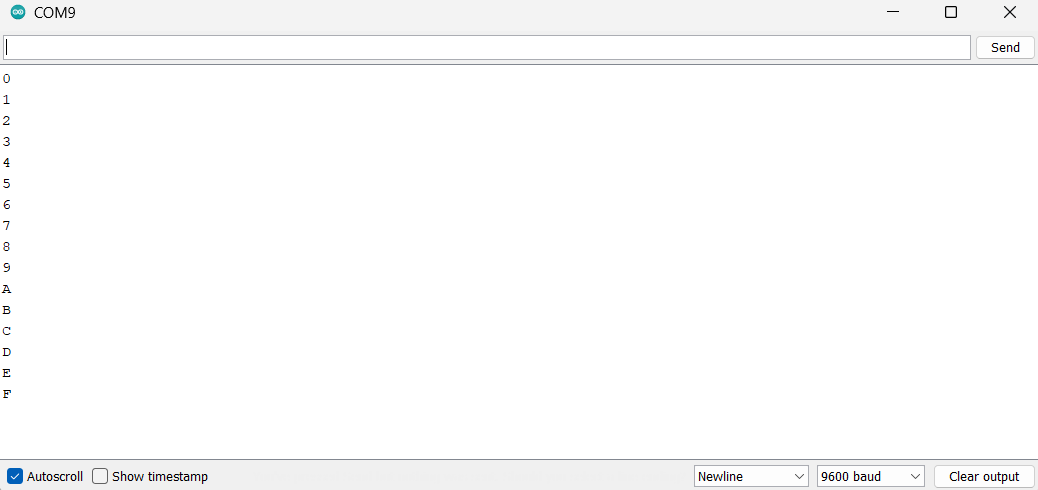
Let’s understand the code
First initialize the required library.
#include <Keypad.h>
Define the key matrix here we have used 4x4 keypad which means 4 rows and 4 columns
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
Define the 4x4 Array for the keypad
char hexaKeys[ROWS][COLS] = {
{'0','1','2','3'},
{'4','5','6','7'},
{'8','9','A','B'},
{'C','D','E','F'}
};
Set the pin numbers for rows and columns of the keypad
byte rowPins[ROWS] = {D0,D1,D2,D3}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {D5,D6,D7,D9}; //connect to the column pinouts of the keypad
Initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
In setup function
We have initiated the serial communication with a 9600 Baud rate.
void setup(){
Serial.begin(9600);
}
In loop function
Here we can detect the key using customKeypad.getKey()
function and print it on the serial monitor.
void loop()
{
char customKey = customKeypad.getKey();
if (customKey){
Serial.println(customKey);
}
}
Components Used |
||
---|---|---|
4x4 Keypad Module Keypad is an input device which is generally used in applications such as calculator, ATM machines, computer etc. |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 |