Description
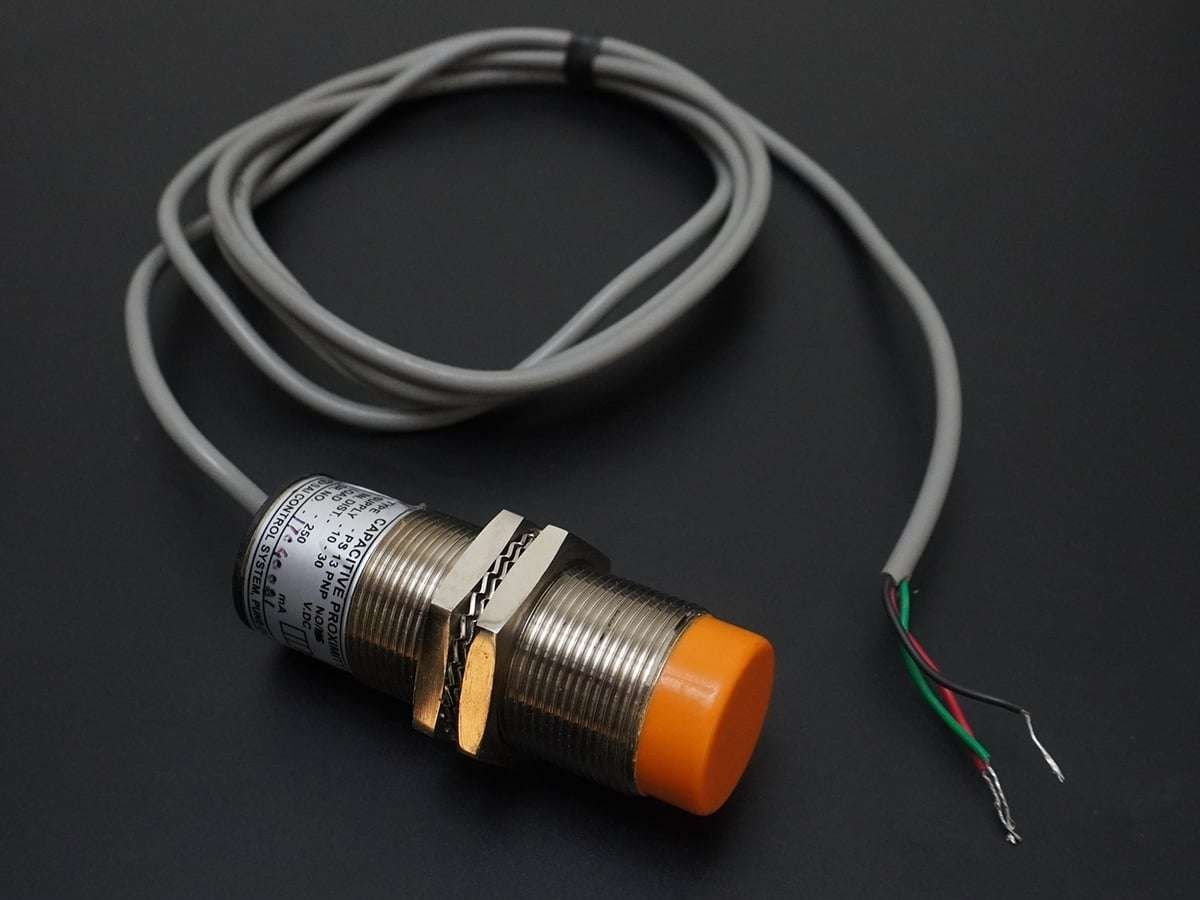
.jpg)
- The capacitive proximity sensor is a device that can detect the presence or absence of solid or liquid objects without physically touching them.
- It works by emitting an electrical field, and when an object comes close to the sensor, it changes the electrical field, which changes the capacitance of the sensor.
- This change is detected and converted into an electrical signal that can be used by other electronic devices.
- Capacitive sensors are commonly used in touch screens, proximity sensors, and other applications where a non-contact method of sensing is required.
- They operate on a power supply of +12V to +24V AC or DC.
Capacitive Sensor Hardware Connection with NodeMCU
.png)
.jpg)
.jpg)
Capacitive Proximity Sensor Detection using NodeMCU
Now, let’s write the code for the detection of solid or liquid objects using a Capacitive Sensor and NodeMCU.
Code for Capacitive Sensor using NodeMCU
const int SENSOR_OUTPUT_PIN = D0; /* Capacitive sensor O/P pin */
void setup() {
pinMode(SENSOR_OUTPUT_PIN, INPUT);
Serial.begin(9600); /* Define baud rate for serial communication */
delay(1000); /* Power On Delay */
}
void loop() {
int sensor_output;
sensor_output = digitalRead(SENSOR_OUTPUT_PIN);
if( sensor_output == HIGH) {
Serial.print("Object detected\n\n");
}
delay(100);
}
Capacitive Sensor Output on Serial Monitor
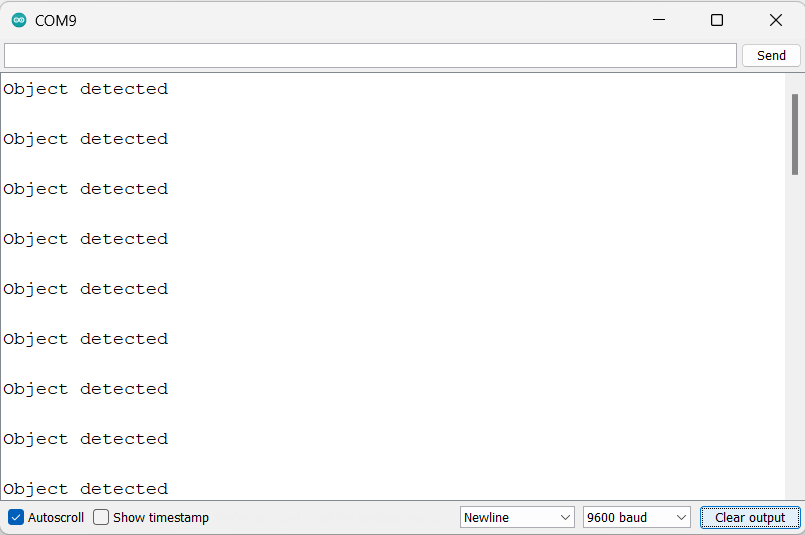
Let’s understand the code
The code starts with defining the NodeMCU pin to the data pin of the capacitive sensor.
const int SENSOR_OUTPUT_PIN = D0; /* Capacitive sensor O/P pin */
In the Setup Function
First, we have set the baud rate for serial communication at 9600.
Then, we declared the sensor pin as INPUT to receive the data from the capacitive sensor.
void setup() {
pinMode(SENSOR_OUTPUT_PIN, INPUT);
Serial.begin(9600); /* Define baud rate for serial communication */
delay(1000); /* Power On Delay */
}
In the Loop Function
Using the digitalRead function we have detected the output of the sensor and stored it in the sensor_output
variable.
When the output goes HIGH then we will print on Serial Monitor as Object Detected
.
void loop() {
int sensor_output;
sensor_output = digitalRead(SENSOR_OUTPUT_PIN);
if( sensor_output == HIGH) {
Serial.print("Object detected\n\n");
}
delay(100);
}
Components Used |
||
---|---|---|
NodeMCU NodeMCUNodeMCU |
X 1 |
Downloads |
||
---|---|---|
|
Capacitive_Sensor_NodeMCU_Code | Download |