Description
- As we know the ESP-NOW is a wireless communication protocol developed by Espressif Systems for its Wi-Fi chips.
- It allows devices to communicate directly with each other without the need for a Wi-Fi access point.
- In this guide, we have used the three ESP8266 boards, In that Two boards are masters and one is a slave.
- In those two master boards, the first board sends the temperature and humidity data and the second board sends the random values to the slave.
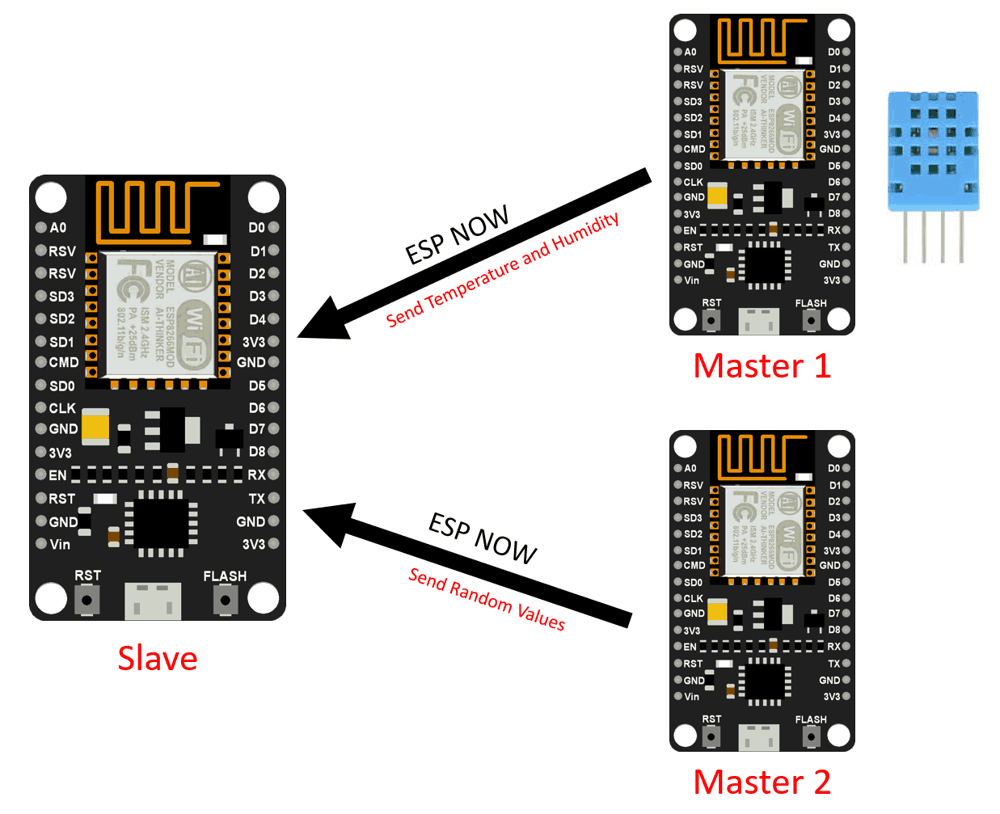
Before going forward we need to know the MAC address of the ESP32 Board.
So first get the MAC address from the below code and note down it.
Getting Board MAC Address
#include <ESP8266WiFi.h>
void setup(){
Serial.begin(115200);
Serial.println();
Serial.println(WiFi.macAddress());
}
void loop(){}
Output
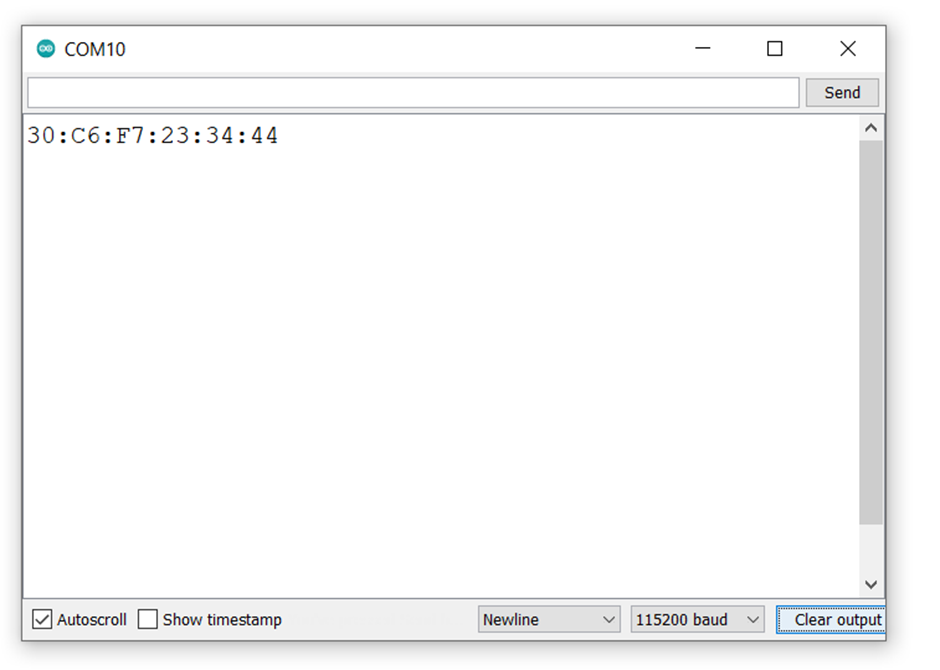
Connection Diagram of DHT11 with NodeMCU
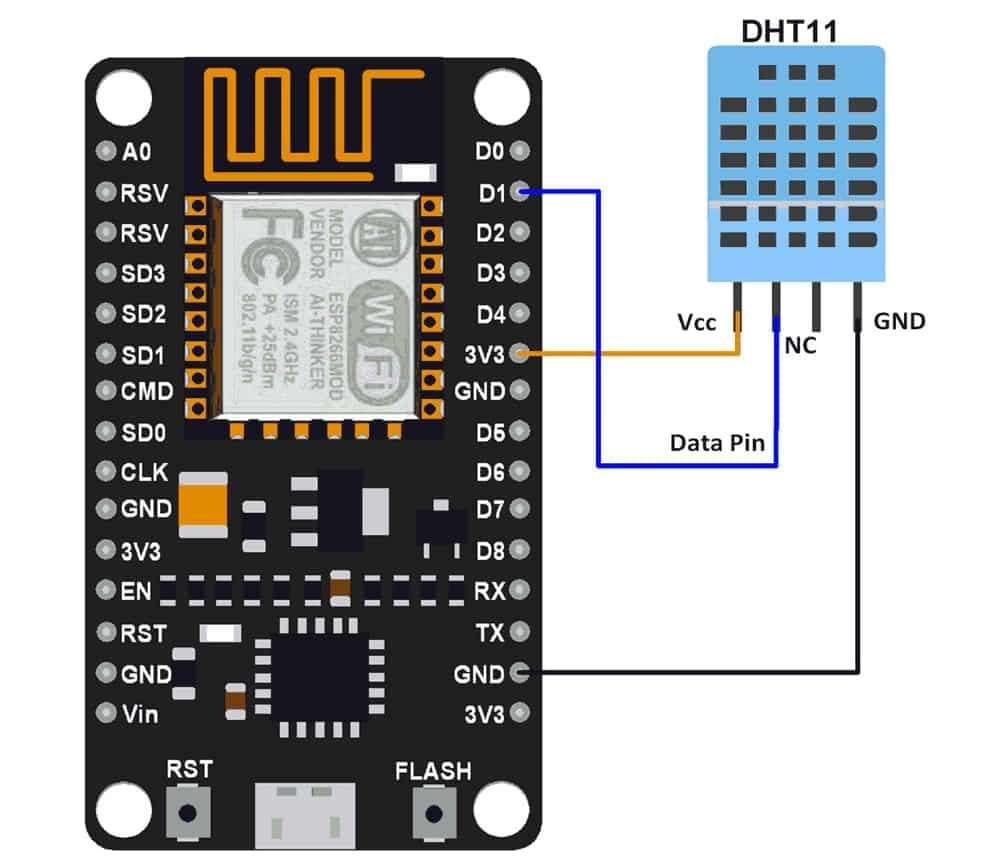
Now copy the below code and upload it on the first esp32 device. Before uploading you must have to enter the MAC address of the slave device
uint8_t broadcastAddress1[] = {0x30, 0xC6, 0xF7, 0x23, 0x34, 0x44};
ESP Now DHT11 Sensor Code
#include <espnow.h>
#include <ESP8266WiFi.h>
#include "DHT.h"
// Include the DHT library
DHT dht;
// Enter the MAC address of the receiver
uint8_t broadcastAddress[] = {0x08, 0x3A, 0x8D, 0xD3, 0x6C, 0xEE};
// String to hold delivery status
String success;
// Structure to hold DHT11 data
typedef struct struct_dht {
float temp;
float hum;
} struct_dht;
// Create an instance of the struct_dht structure
struct_dht DHT11_data;
// Callback function to handle data send status
void OnDataSent(uint8_t *mac_addr, uint8_t espStatus) {
char macStr[18];
Serial.print("Delivery Status of: ");
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",
mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
Serial.print(macStr);
Serial.println(espStatus == 0 ? " Send Successfully" : " Fail to send");
Serial.println("");
}
void setup() {
Serial.begin(115200);
// Set WiFi mode to Station mode
WiFi.mode(WIFI_STA);
// Initialize the DHT sensor on GPIO pin D1
dht.setup(D1);
// Initialize ESP-NOW
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
// Set the role of this device as a controller
esp_now_set_self_role(ESP_NOW_ROLE_CONTROLLER);
// Register callback for data send status
esp_now_register_send_cb(OnDataSent);
// Add a peer (receiver) with the specified MAC address
esp_now_add_peer(broadcastAddress, ESP_NOW_ROLE_CONTROLLER, 1, NULL, 0);
}
void loop() {
// Get temperature and humidity from the DHT11 sensor
DHT11_data.temp = dht.getTemperature();
DHT11_data.hum = dht.getHumidity();
// Print temperature and humidity
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.print("\t\t");
Serial.print("Humidity: ");
Serial.println(DHT11_data.hum);
// Send DHT11 data to the specified receiver
esp_now_send(0, (uint8_t *) &DHT11_data, sizeof(DHT11_data));
delay(3000); // Wait for a few seconds before sending the next data
}
Understand the code
Add espnow.h, DHT.h, and WiFi.h libraries.
#include <espnow.h>
#include <ESP8266WiFi.h>
#include "DHT.h"
Create the object below
DHT dht;
Now add the MAC address of receiver ESP8266
Our MAC address is 30:C6:F7:23:34:44
uint8_t broadcastAddress1[] = {0x30, 0xC6, 0xF7, 0x23, 0x34, 0x44};
uint8_t broadcastAddress1[] = {0x30, 0xC6, 0xF7, 0x23, 0x34, 0x44};
Here we have created a structure to send the data.
typedef struct struct_message {
float temp;
float hum;
} struct_message;
Create a struct_message
variable called DHT11_data
.
struct_message DHT11_data;
OnDataSent()
is a callback function that will be executed when a message is sent.
void OnDataSent(uint8_t *mac_addr, uint8_t espStatus) {
char macStr[18];
Serial.print("Delivery Status of: ");
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
Serial.print(macStr);
Serial.println(espStatus == 0 ? " Send Successfully" : " Fail to send");
In the setup function,
Initialize the serial monitor
Serial.begin(115200);
set the D1
pin as a data communication Pin.
dht.setup(D1);
Set ESP32 in a Wi-Fi station Mode:
WiFi.mode(WIFI_STA);
Initialize ESP-NOW:
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
Set the ESP8266 role as a controller, to sends data to other devices in the network
esp_now_set_self_role(ESP_NOW_ROLE_CONTROLLER);
Now, register the callback function that will be called when a message is sent.
esp_now_register_send_cb(OnDataSent);
Pair with the slave device to send the data
esp_now_add_peer(broadcastAddress, ESP_NOW_ROLE_SLAVE, 1, NULL, 0);
The arguments for esp_now_add_peer
are:
esp_now_add_peer (mac address, role, wi-fi channel, key, key length)
In the loop function
Get the temperature and humidity reading from DHT11 and store it.
DHT11_data.temp = dht.getTemperature(); /*Get the Temperature value*/
DHT11_data.hum = dht.getHumidity(); /*Get the Humidity value*/
Here we’ll send the temperature and humidity every three seconds.
esp_now_send(0, (uint8_t *) &msg,sizeof(msg));
Wait for three second
delay(3000);
Output
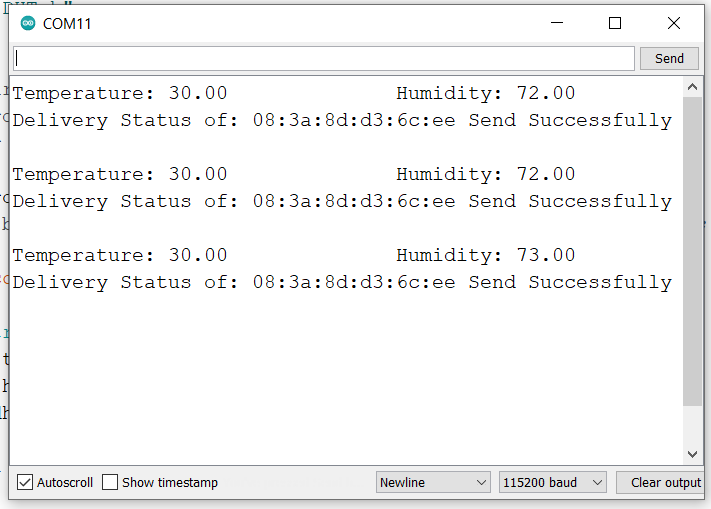
Now copy the below code and upload it on the second device and check the result on the serial monitor.
Before uploading make sure you have updated your MAC address
uint8_t broadcastAddress[] = {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF};
ESP Now ESP8266 Code to send Random Values
#include <espnow.h>
#include <ESP8266WiFi.h>
// Structure to hold DHT11 sensor data
typedef struct struct_dht {
float temp;
float hum;
} struct_dht;
// Create an instance of the struct_dht structure
struct_dht DHT11_data;
// Structure to hold custom message data
typedef struct struct_message {
int x;
int y;
} struct_message;
// Create an instance of the struct_message structure
struct_message myData;
// MAC addresses of the senders
const char dhtSenderMac[] = "84:f3:eb:cb:49:61";
const char bmpSenderMac[] = "84:f3:eb:ca:f8:d3";
// Callback function to handle received data
void OnDataRecv(uint8_t * mac_addr, uint8_t *incomingData, uint8_t len) {
char macStr[18];
// Convert MAC address to string format
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",
mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
// Check if data is from DHT sender
if (memcmp(macStr, dhtSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&DHT11_data, incomingData, sizeof(DHT11_data));
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.print("°C, Humidity: ");
Serial.print(DHT11_data.hum);
Serial.println("%");
Serial.println("");
}
// Check if data is from BMP sender
else if (memcmp(macStr, bmpSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&myData, incomingData, sizeof(myData));
Serial.print("X: ");
Serial.print(myData.x);
Serial.print(", Y: ");
Serial.println(myData.y);
Serial.println("");
}
}
void setup() {
Serial.begin(115200);
// Set WiFi mode to Station mode and disconnect
WiFi.mode(WIFI_STA);
WiFi.disconnect();
// Initialize ESP-NOW
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
// Set the role of this device as a slave
esp_now_set_self_role(ESP_NOW_ROLE_SLAVE);
// Register callback for received data
esp_now_register_recv_cb(OnDataRecv);
}
void loop() {
delay(10000); // Delay for 10 seconds
}
Understand the code
Add espnow.h
and ESP8266WiFi.h
libraries.
#include <espnow.h>
#include <ESP8266WiFi.h>
Here we have created a structure to send the data.
typedef struct struct_message {
int x;
int y;
} struct_message;
Create a struct_message
variable called DHT11_data
and BMP180_data
.
struct_message myData;
OnDataSent()
is a callback function that will be executed when a message is sent.
void OnDataSent(uint8_t *mac_addr, uint8_t espStatus) {
Serial.print("\r\nLast Packet Send Status:\t");
Serial.println(espStatus == ESP_NOW_SEND_SUCCESS ? "Delivery Success" : "Delivery Fail");
}
In the setup function,
Initialize the serial monitor
Serial.begin(115200);
Set ESP32 in a Wi-Fi station Mode:
WiFi.mode(WIFI_STA);
Initialize ESP-NOW:
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
Set the ESP8266 role as a controller, to send data to other devices in the network
esp_now_set_self_role(ESP_NOW_ROLE_CONTROLLER);
Now, register the callback function that will be called when a message is sent.
esp_now_register_send_cb(OnDataSent);
Pair with the slave device to send the data
esp_now_add_peer(broadcastAddress, ESP_NOW_ROLE_SLAVE, 1, NULL, 0);
The arguments for esp_now_add_peer
are:
esp_now_add_peer (mac address, role, wi-fi channel, key, key length)
In the loop function
Get the random values and store them in X and Y.
myData.x = random(0,50);
myData.y = random(0,50);
Send the random values every three seconds.
esp_now_send(broadcastAddress,(uint8_t *) &myData, sizeof(myData));
Wait for three seconds
delay(3000);
Output
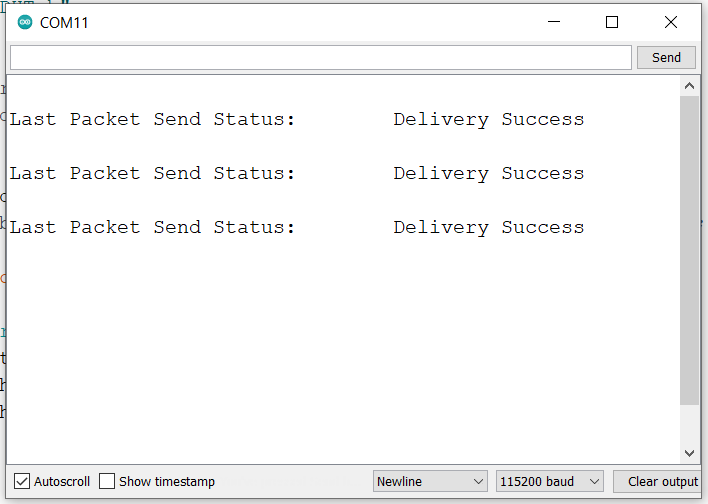
ESP Now NodeMCU Slave Receiver Code
#include <espnow.h>
#include <ESP8266WiFi.h>
// Structure to hold DHT11 sensor data
typedef struct struct_dht {
float temp;
float hum;
} struct_dht;
// Create an instance of the struct_dht structure
struct_dht DHT11_data;
// Structure to hold custom message data
typedef struct struct_message {
int x;
int y;
} struct_message;
// Create an instance of the struct_message structure
struct_message myData;
// MAC addresses of the senders
const char dhtSenderMac[] = "84:f3:eb:cb:49:61";
const char bmpSenderMac[] = "84:f3:eb:ca:f8:d3";
// Callback function to handle received data
void OnDataRecv(uint8_t * mac_addr, uint8_t *incomingData, uint8_t len) {
char macStr[18];
// Convert MAC address to string format
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",
mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
// Check if data is from DHT sender
if (memcmp(macStr, dhtSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&DHT11_data, incomingData, sizeof(DHT11_data));
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.print("°C, Humidity: ");
Serial.print(DHT11_data.hum);
Serial.println("%");
Serial.println("");
}
// Check if data is from BMP sender
else if (memcmp(macStr, bmpSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&myData, incomingData, sizeof(myData));
Serial.print("X: ");
Serial.print(myData.x);
Serial.print(", Y: ");
Serial.println(myData.y);
Serial.println("");
}
}
void setup() {
Serial.begin(115200);
// Set WiFi mode to Station mode and disconnect
WiFi.mode(WIFI_STA);
WiFi.disconnect();
// Initialize ESP-NOW
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
// Set the role of this device as a slave
esp_now_set_self_role(ESP_NOW_ROLE_SLAVE);
// Register callback for received data
esp_now_register_recv_cb(OnDataRecv);
}
void loop() {
delay(10000); // Delay for 10 seconds
}
Understand the code
Add esp_now.h
and WiFi.h
libraries.
#include <esp_now.h>
#include <WiFi.h>
Here we have created a structure to send the data.
typedef struct struct_message {
int x;
int y;
} struct_message;
Create a struct_message
variable called DHT11_data
and BMP180_data
.
struct_message myData;
Here we have created a structure to send and receive the data.
typedef struct struct_message {
float temp;
float hum;
} struct_message;
Create a struct_message
variable called DHT11_data
and BMP180_data
.
struct_message DHT11_data;
Se the MAC address of sender ESP32 boards
const char dhtSenderMac[] = "84:f3:eb:cb:49:61";
const char bmpSenderMac[] ="84:f3:eb:ca:f8:d3";
Create a callback function that will be called when the ESP32 receives the data via ESP-NOW.
void OnDataRecv(uint8_t * mac_addr, uint8_t *incomingData, uint8_t len) {
char macStr[18];
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x", mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
if (memcmp(macStr, dhtSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&DHT11_data, incomingData, sizeof(DHT11_data));
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.print("°C, Humidity: ");
Serial.print(DHT11_data.hum);
Serial.println("%");
Serial.println("");
}
else if (memcmp(macStr, bmpSenderMac, 17) == 0) {
Serial.print("Data received from: ");
Serial.println(macStr);
memcpy(&myData, incomingData, sizeof(myData));
Serial.print("X: ");
Serial.print(myData.x);
Serial.print(", Y: ");
Serial.println(myData.y);
Serial.println("");
}
}
In the setup function,
Initialize the serial monitor
Serial.begin(115200);
Set ESP32 in a Wi-Fi station Mode:
WiFi.mode(WIFI_STA);
Initialize ESP-NOW:
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
Set the ESP8266 role as a controller, to send data to other devices in the network
esp_now_set_self_role(ESP_NOW_ROLE_SLAVE);
Now, register the callback function that will be called when a message is sent.
esp_now_register_send_cb(OnDataSent);
Output
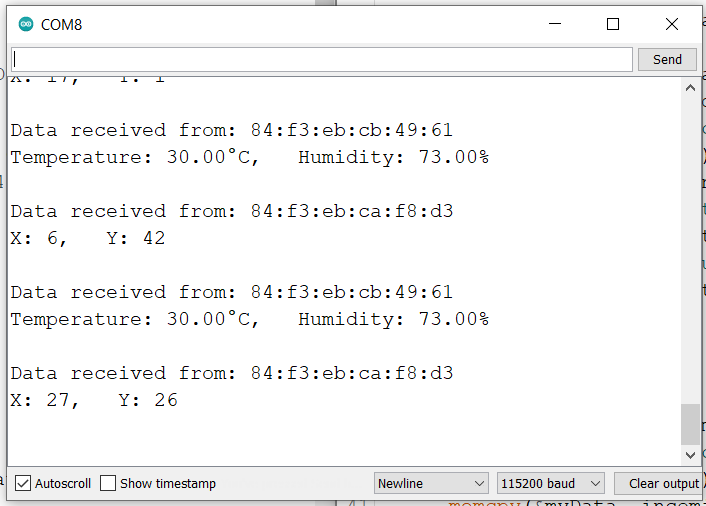
Components Used |
||
---|---|---|
NodeMCU NodeMCUNodeMCU |
X 3 | |
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 |