Overview of DHT11
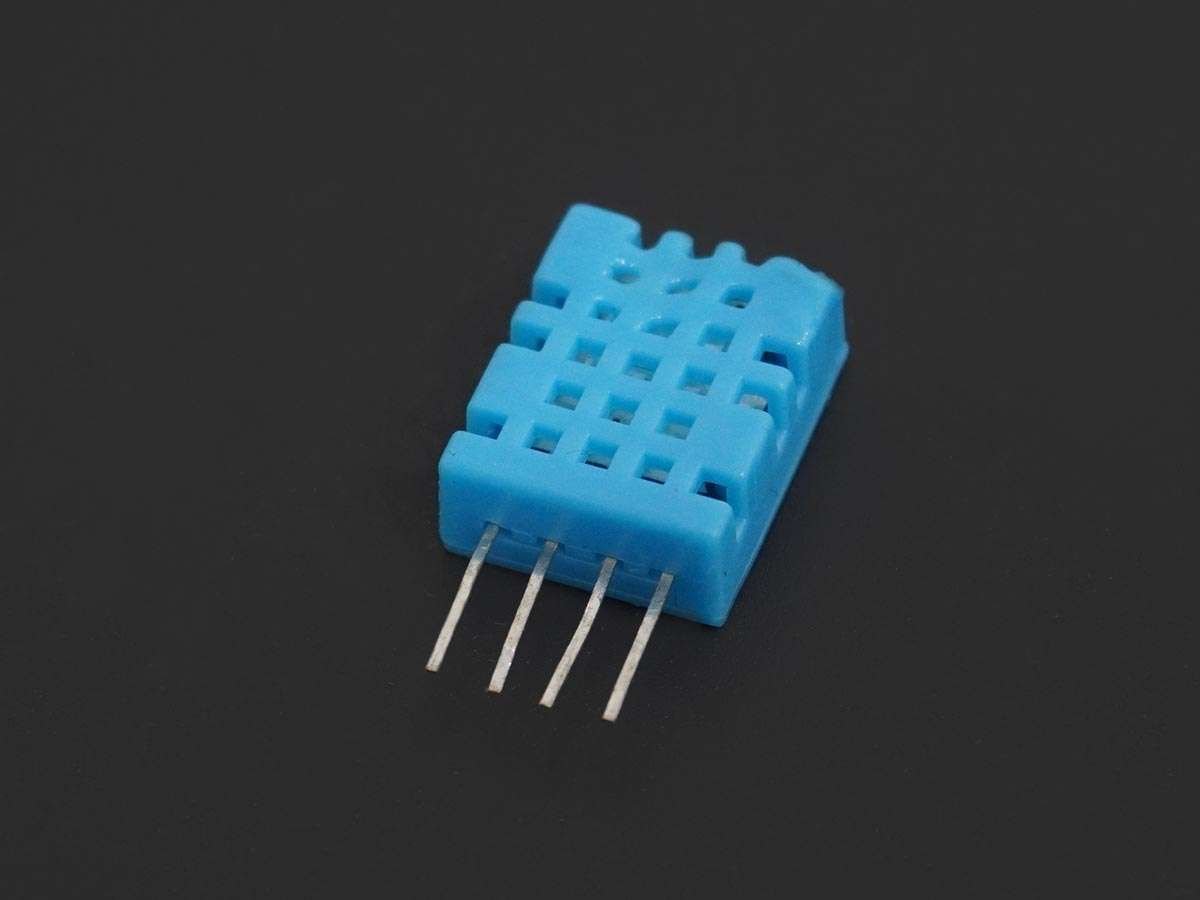
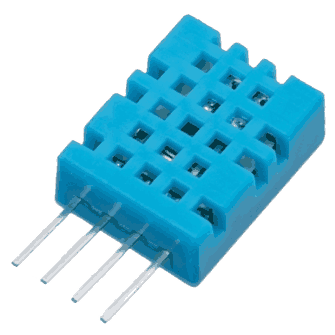
DHT11 sensor measures and provides humidity and temperature values serially over a single wire.
It can measure the relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
It has 4 pins; one of which is used for data communication in serial form.
Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of the frame.
For more information about the DHT11 sensor and how to use it, refer to the topic DHT11 sensor in the sensors and modules topic.
Connection Diagram DHT11 with NodeMCU
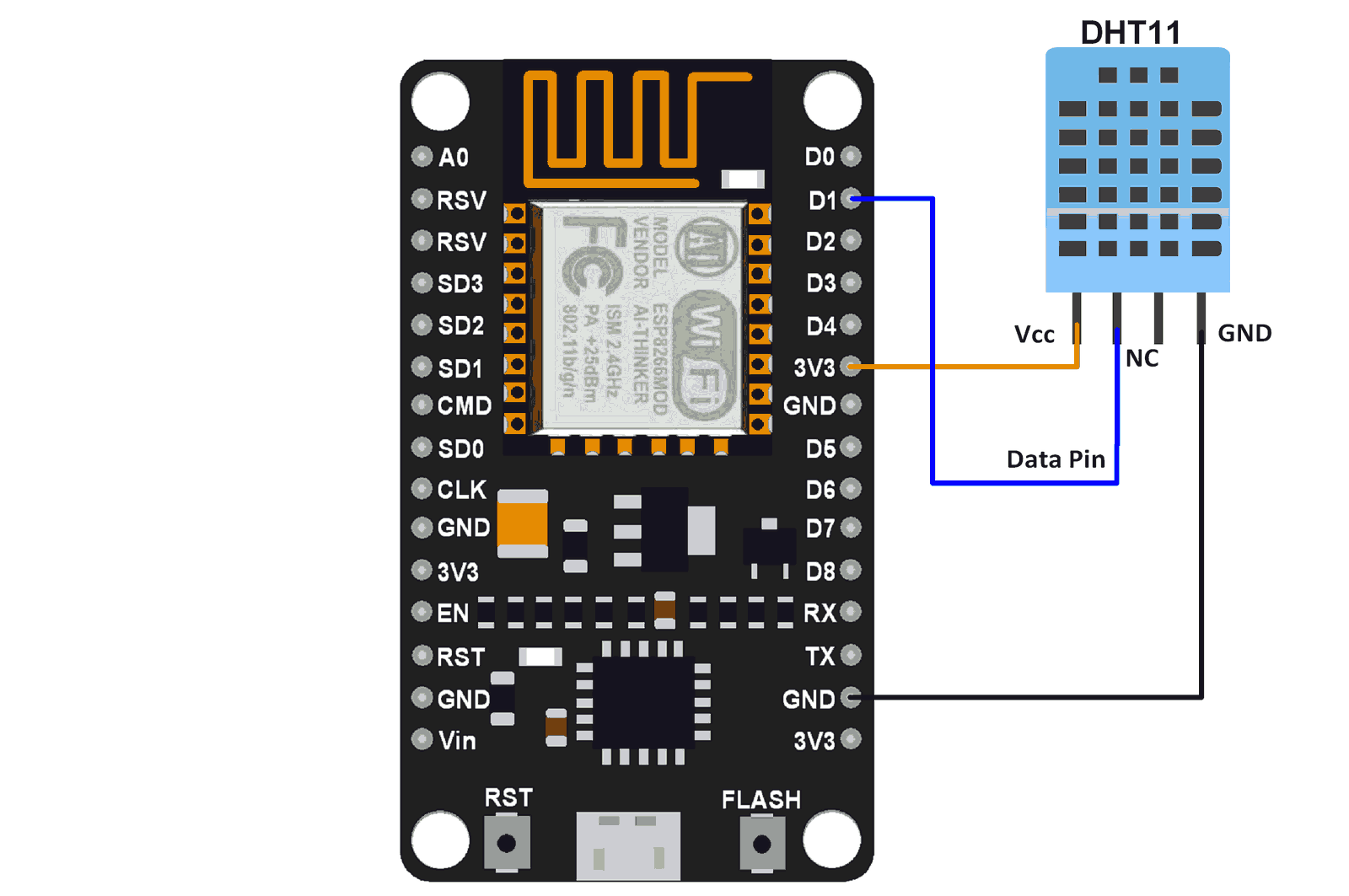
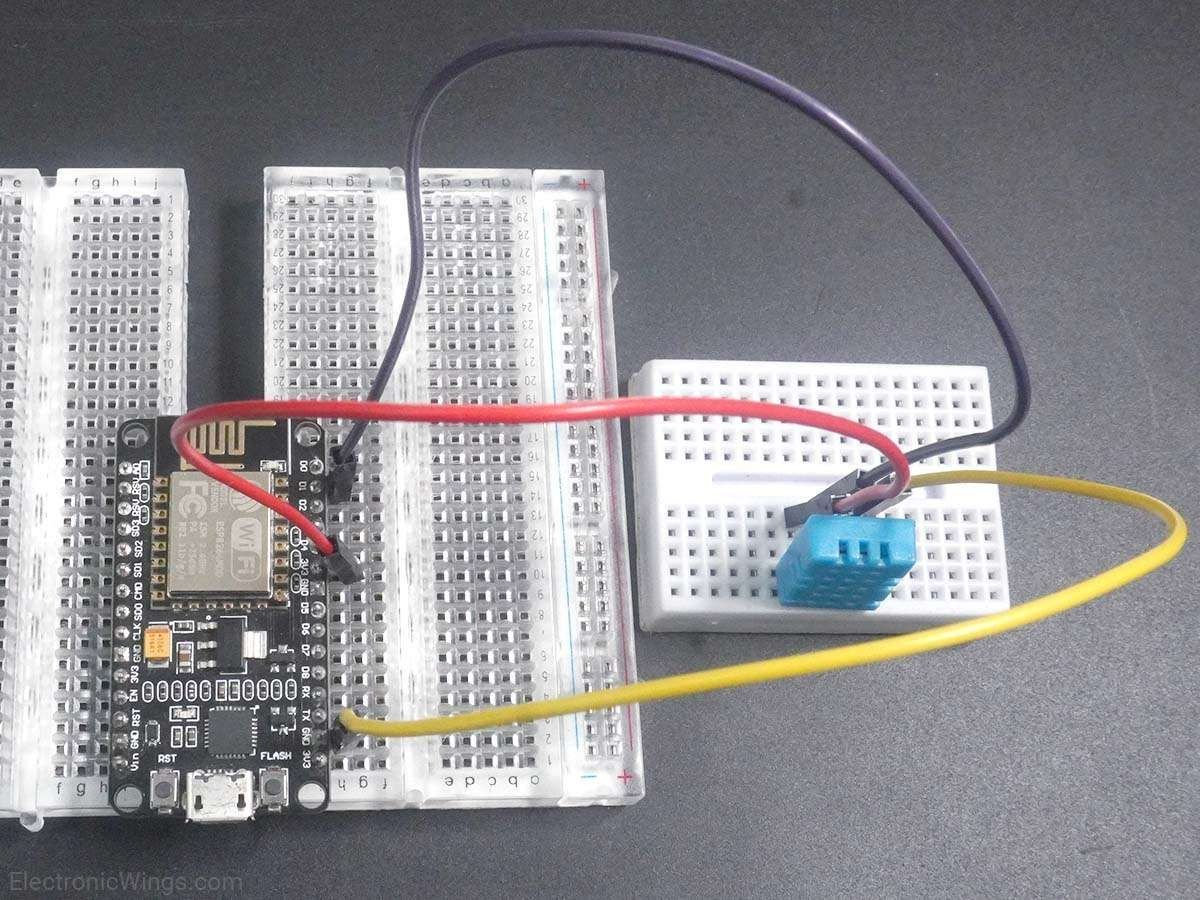
Read temperature and humidity using DHT11 and NodeMCU
Let’s interface DHT11 with NodeMCU and read the value of Temperature and Humidity from DHT11.
We can write codes for NodeMCU DevKit in either Lua Script or C/C++ language. We are using ESPlorer IDE for writing code in Lua scripts and Arduino IDE for writing code in C/C++. To know more refer to Getting started with NodeMCU using ESPlorer IDE (which uses Lua scripting for NodeMCU) and Getting started with NodeMCU using Arduino IDE (which uses C language-based Arduino sketches for NodeMCU).
NodeMCU LUA based functions for DHT module
Let’s see the LUA based functions of NodeMCU that can be used for DHT modules to read the Temperature and Humidity.
dht.read()
This function is used to read data from all kinds of DHT sensors, including DHT11, 21, 22, 33, 44 humidity temperature sensors.
Syntax:
dht.read(pin)
Parameters:
pin
: pin number from which output of the DHT sensor is to be read. 0 pin number (D0) is not supported.
Returns:
status
: it returns one status out of dht.OK, dht.ERROR_CHECKSUM, dht.ERROR_TIMEOUT.
temp
: temperature
humi
: humidity
temp_dec
: temperature decimal
humi_dec
: humidity decimal
Note: the data type of return variables depends on firmware supported data types. i.e. if firmware supports floating then it will return float values otherwise it will return an integer.
Also, we can use dht11.read()
the function to read output from only dht11 or dht.readxx()
function to read output from DHT modules other than dht11 i.e. xx will be 22, 33, etc. These functions have the same syntax, parameters, and return values.
Let’s write Lua script to read temperature and humidity from dht11
Lua Script for DHT11
pin = 1
status, temp, humi, temp_dec, humi_dec = dht.read(pin)--read dht11 from pin
if status == dht.OK then --check status is ok and print temperature and humidity
print(string.format("DHT Temperature:%d.%03d;Humidity:%d.%03d",
math.floor(temp),
temp_dec,
math.floor(humi),
humi_dec
))
elseif status == dht.ERROR_CHECKSUM then --else print either status
print( "DHT Checksum error." )
elseif status == dht.ERROR_TIMEOUT then
print( "DHT timed out." )
end
ESPlorer Serial Output Window
ESPlorer Serial monitor output window for DHT11 temperature & humidity measure
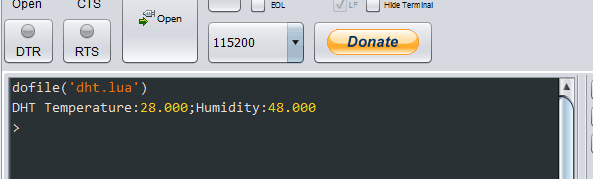
Arduino Sketch for DHT11
Here, we will be using the DHT11 library by Mark Ruys from GitHub.
Download this library from here.
Extract the library and add the folder to the libraries folder path of Arduino IDE.
For information about how to add a custom library to the Arduino IDE and use examples from it, referring Library to Arduino IDE in the Basics section.
Once the library has been added to the Arduino IDE, open the IDE and open the example sketch named DHT_Test from the library added.
Change the DHT11 pin in the sketch. You can refer to the sketch given below for the change to be made.
DHT11 Code for NodeMCU using Arduino IDE
#include "DHT.h"
DHT dht;
void setup()
{
Serial.begin(9600);
Serial.println();
Serial.println("Status\tHumidity (%)\tTemperature (C)\t(F)");
dht.setup(D1); /* D1 is used for data communication */
}
void loop()
{
delay(dht.getMinimumSamplingPeriod()); /* Delay of amount equal to sampling period */
float humidity = dht.getHumidity();/* Get humidity value */
float temperature = dht.getTemperature();/* Get temperature value */
Serial.print(dht.getStatusString());/* Print status of communication */
Serial.print("\t");
Serial.print(humidity, 1);
Serial.print("\t\t");
Serial.print(temperature, 1);
Serial.print("\t\t");
Serial.println(dht.toFahrenheit(temperature), 1);/* Convert temperature to Fahrenheit units */
}
Arduino Serial Output Window
Arduino Serial monitor output window DHT11 temperature & humidity measure
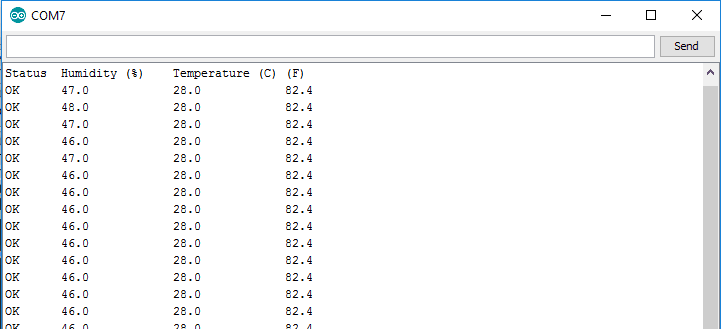
Components Used |
||
---|---|---|
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 | |
ESP12F ESP12E |
X 1 |