Overview of Thermistor
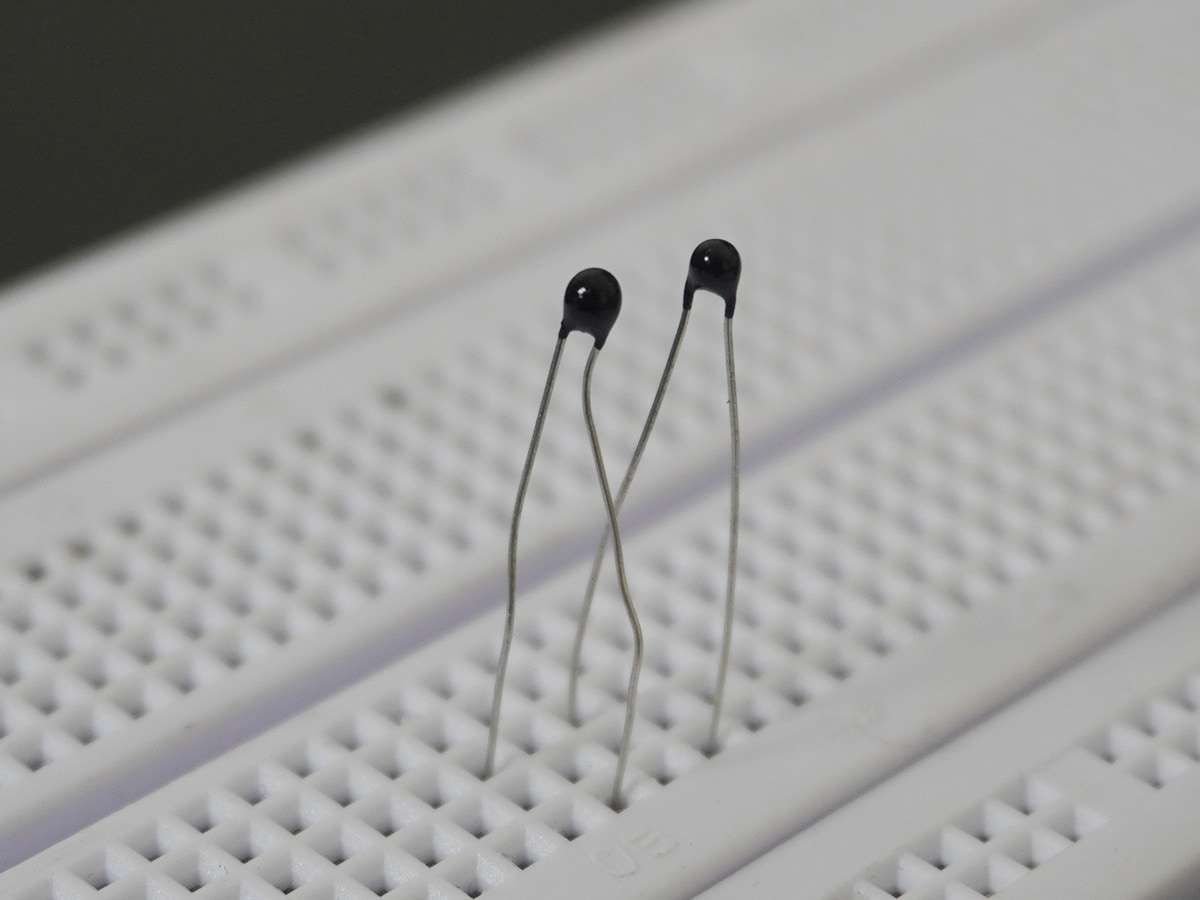
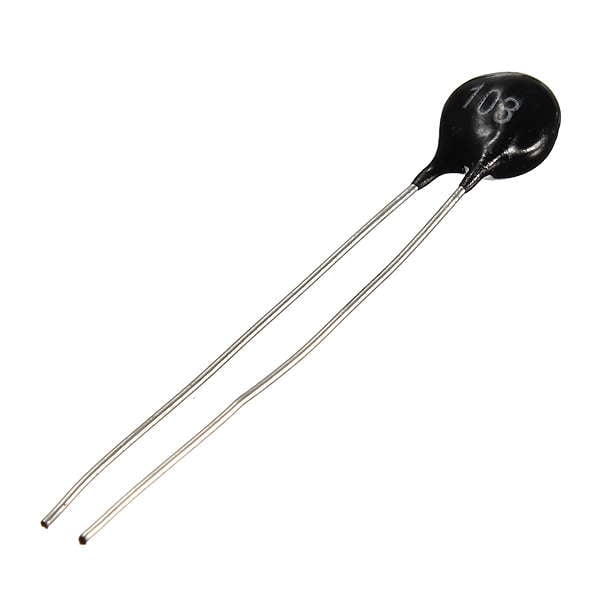
A thermistor is a variable resistance element; whose resistance varies with change in temperature. The change in resistance value is a measure of the temperature. Thermistors are classified as PTC (Positive Temperature Coefficient) or NTC (Negative Temperature Coefficient).
They can be used as current limiters, temperature sensors, overcurrent protectors, etc. For more information about the thermistor and how to use it, refer to the topic NTC Thermistor in the sensors and modules section.
Connection Diagram of NTC Thermistor with NodeMCU
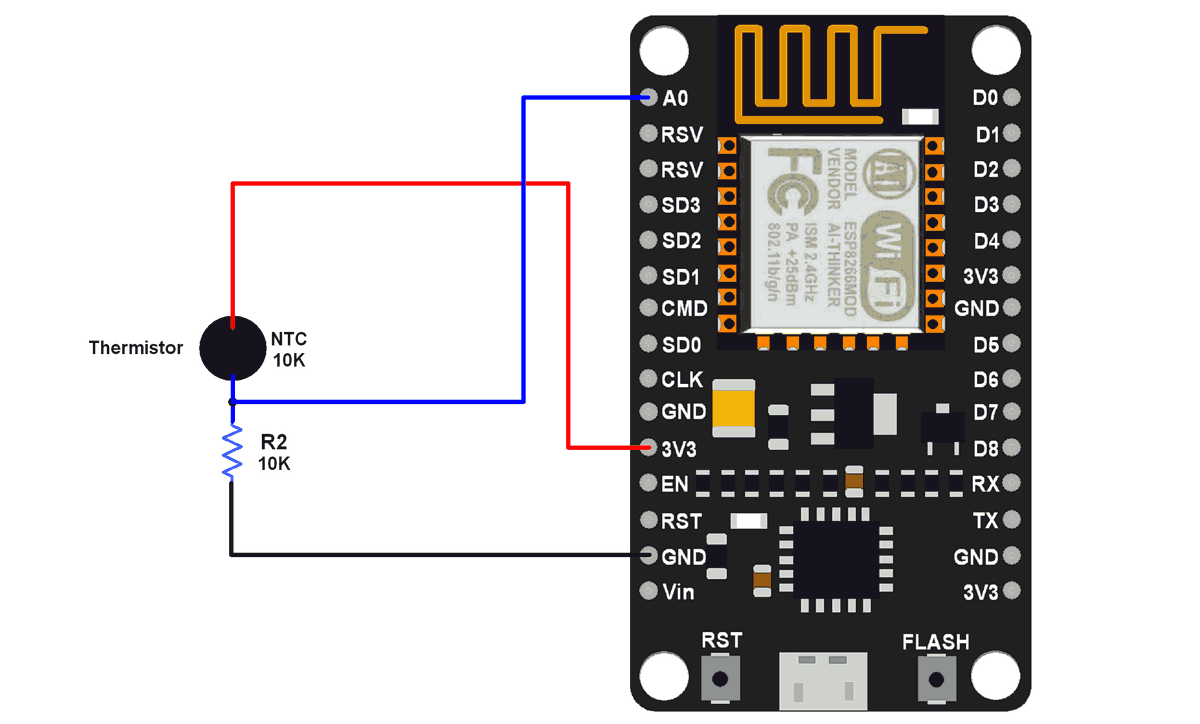
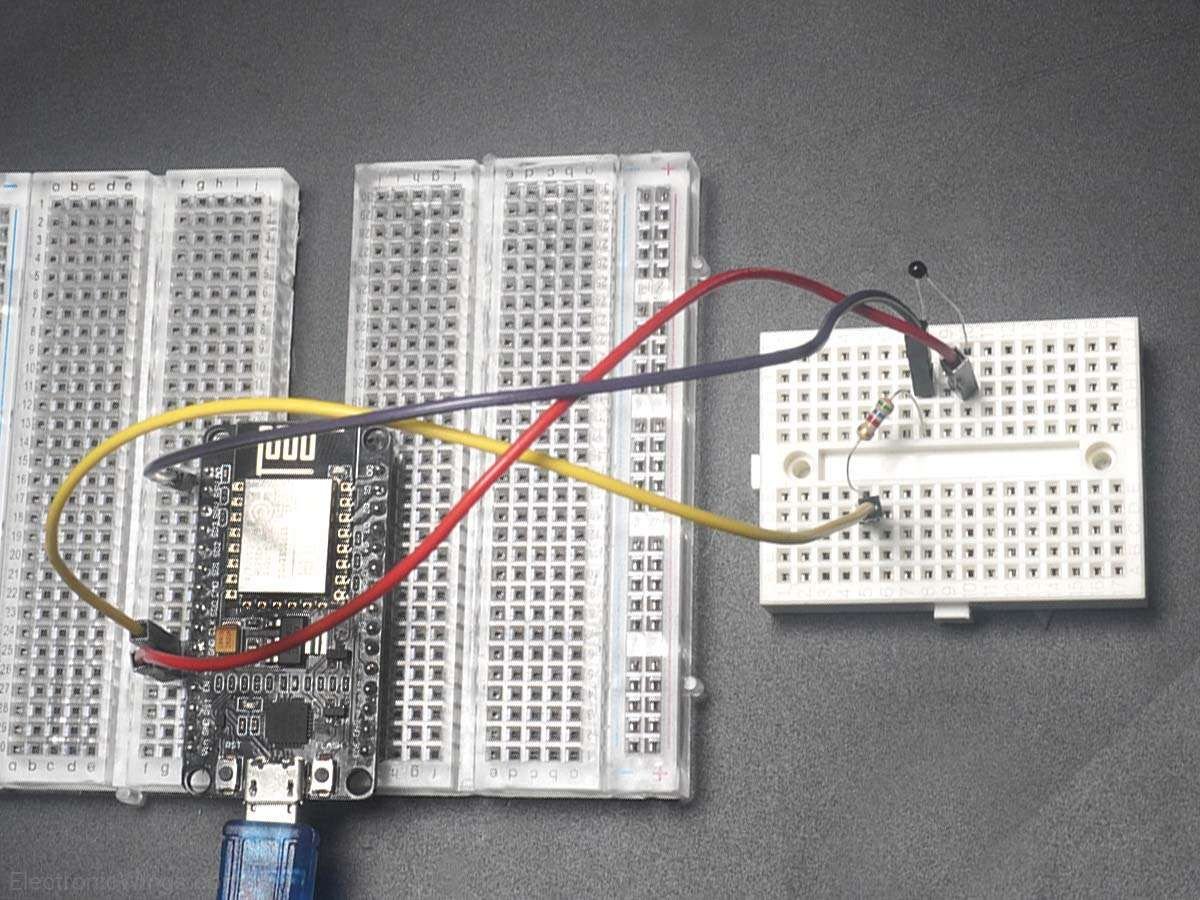
Measure Temperature using NTC Thermistor and NodeMCU
Measuring temperature using a thermistor.
Here, an NTC type thermistor of 10kΩ (thermistor resistance) is used. NTC of 10kΩ means that this thermistor has a resistance of 10kΩ at 25°C. The voltage across the 10kΩ resistor is given to the ADC of NodeMCU.
We can calculate the voltage across a series of 10kΩ resistor (R2 in the above figure) as,
Vout = VCC * ADC_Value / ADC_Resolution
Where Vout is the voltage measured by the ADC of NodeMCU
The temperature can be found out from thermistor resistance using the Steinhart-Hart equation.
Temperature(in kelvin) = 1 / (A + B[ln(Rth)] + C[ln(Rth)]^3)
where, A = 0.001129148,
B = 0.000234125,
C = 8.76741*10^-8 and
Rth is the thermistor resistance.
The thermistor resistance (Rth) can be found out using a simple voltage divider network formula.
Rth + 10k = VCC * 10k / Vout
Where Rth is the thermal resistance
NodeMCU ADC can be used to measure the analog voltage across the 10kΩ resistor. To know about ADC of NodeMCU refer to NodeMCU ADC with ESPlorer IDE and NodeMCU ADC with Arduino IDE.
We can write codes for NodeMCU Dev Kit in either Lua Script or C/C++ language. We are using ESPlorer IDE for writing Lua scripts and Arduino IDE for writing code in C/C++. To know more refer to Getting started with NodeMCU using ESPlorer IDE (which uses Lua scripting for NodeMCU) and Getting started with NodeMCU using Arduino IDE (which uses C language-based Arduino sketches for NodeMCU).
Let’s write Lua script for NodeMCU to measure temperature using a thermistor.
Lua Script for thermistor
VCC = 3.3 -- NodeMCU on board 3.3v vcc
R2 = 10000 -- 10k ohm series resistor
adc_resolution = 1023 -- 10-bit adc
-- thermistor equation parameters
A = 0.001129148
B = 0.000234125
C = 8.76741*10^-8
function ln(x) --natural logarithm function for x>0 real values
local y = (x-1)/(x+1)
local sum = 1
local val = 1
if(x == nil) then
return 0
end
-- we are using limited iterations to acquire reliable accuracy.
-- here its upto 10000 and increased by 2
for i = 3, 10000, 2 do
val = val*(y*y)
sum = sum + (val/i)
end
return 2*y*sum
end
while true do
local Vout, Rth, temperature
local adc_value = adc.read(0)
Vout = (adc_value * VCC) / adc_resolution
Rth = (VCC * R2 / Vout) - R2
temperature = (1 / (A + (B * ln(Rth)) + (C * (ln(Rth))^3))) -- Temperature in kelvin
temperature = temperature - 273.15 -- Temperature in degree celsius
print(string.format("Temperature = %0.3g °C",temperature))
tmr.delay(100000)
end
ESPlorer Serial Output Window
ESPlorer Serial monitor output window for temperature using thermistor
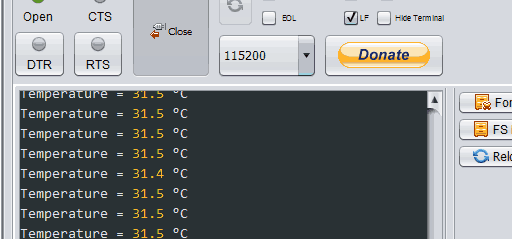
Now let’s write a program of the thermistor for NodeMCU using Arduino IDE
NTC thermistor Code for NodeMCU using Arduino IDE
const double VCC = 3.3; // NodeMCU on board 3.3v vcc
const double R2 = 10000; // 10k ohm series resistor
const double adc_resolution = 1023; // 10-bit adc
const double A = 0.001129148; // thermistor equation parameters
const double B = 0.000234125;
const double C = 0.0000000876741;
void setup() {
Serial.begin(9600); /* Define baud rate for serial communication */
}
void loop() {
double Vout, Rth, temperature, adc_value;
adc_value = analogRead(A0);
Vout = (adc_value * VCC) / adc_resolution;
Rth = (VCC * R2 / Vout) - R2;
/* Steinhart-Hart Thermistor Equation:
* Temperature in Kelvin = 1 / (A + B[ln(R)] + C[ln(R)]^3)
* where A = 0.001129148, B = 0.000234125 and C = 8.76741*10^-8 */
temperature = (1 / (A + (B * log(Rth)) + (C * pow((log(Rth)),3)))); // Temperature in kelvin
temperature = temperature - 273.15; // Temperature in degree celsius
Serial.print("Temperature = ");
Serial.print(temperature);
Serial.println(" degree celsius");
delay(500);
}
Arduino Serial Output Window
Arduino Serial monitor output window for temperature using thermistor
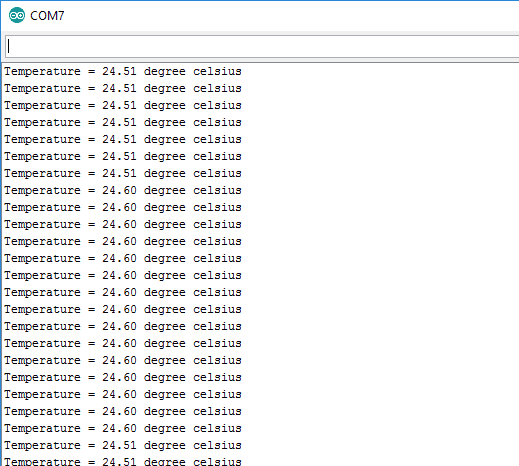
Video of Temperature Measurement using NTC Thermistor and NodemCU
Components Used |
||
---|---|---|
Thermistor Thermistor is a type of resistor whose resistance changes in accordance with change in temperature. It is used to measure the temperature over small range typically -100 °C to 300 °C. |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 | |
ESP12F ESP12E |
X 1 |
Downloads |
||
---|---|---|
|
NodeMCU Thermistor Source files | Download |