Description
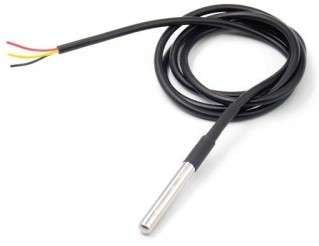
- DS18B20 is a Digital Temperature sensor which is developed by Maxim Integration.
- This sensor measures temperature in the range of -55°C to 125°C.
- It is a 3-terminal device that provides 9-bit to 10-bit Celsius temperature measurements.
- This is a 1-Wire temperature sensor.
DS18B20 Waterproof Sensor Pin details
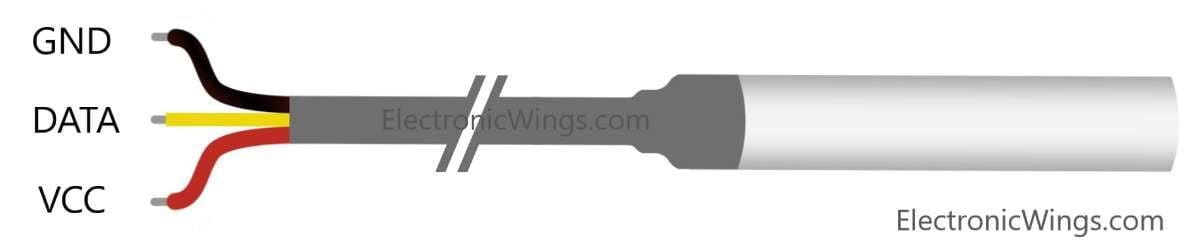
VCC: This is a supply pin that requires DC voltage in the range of 3.0V to 5.5V
DATA: This pin gives the output in digital format
GND: Connect to the supply ground
- DS18B20 consumes the 1mA in active mode and 750nA in standby mode.
- DS18B20 accuracy is ±0.5°C for the range of 10°C to the +85°C
- The DS18B20 is available in 8-Pin SO (150 mils), 8-Pin µSOP, and 3-Pin TO-92 Packages
Note: for the one wire communication we’ll need to add one 4.7k pull-up resistor between the DATA pin and VCC pin.
DS18B20 Interfacing Diagram with Nodemcu
.png)
Measure Temperature using DS18B20 and Nodemcu
Let’s interface the DS18B20 temperature sensor to Nodemcu and display the surrounding temperature on the webserver as well as on the serial monitor.
Here we are using DallasTemperature libraries for the above example. We need to install the DallasTemperature library using the Arduino Library Manager.
- Open the Arduino IDE
- Navigate to Sketch ► Include Library ► Manage Libraries…
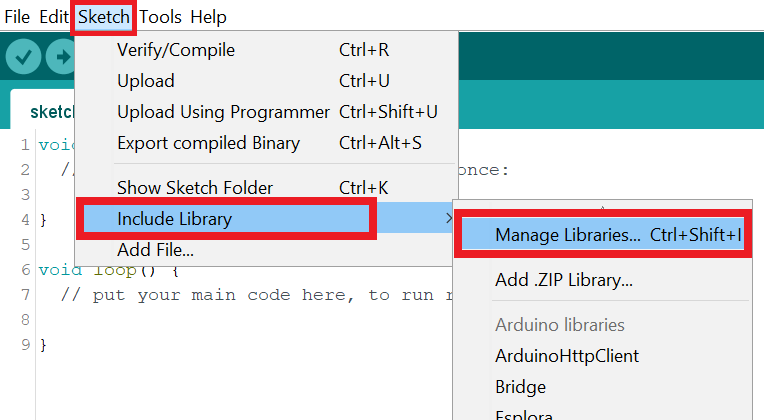
- The library Manager window will pop up. Now enter DS18B20 into the search box, and click Install on the DallasTemperature option to install version 3.9.0 or higher. As shown below image.
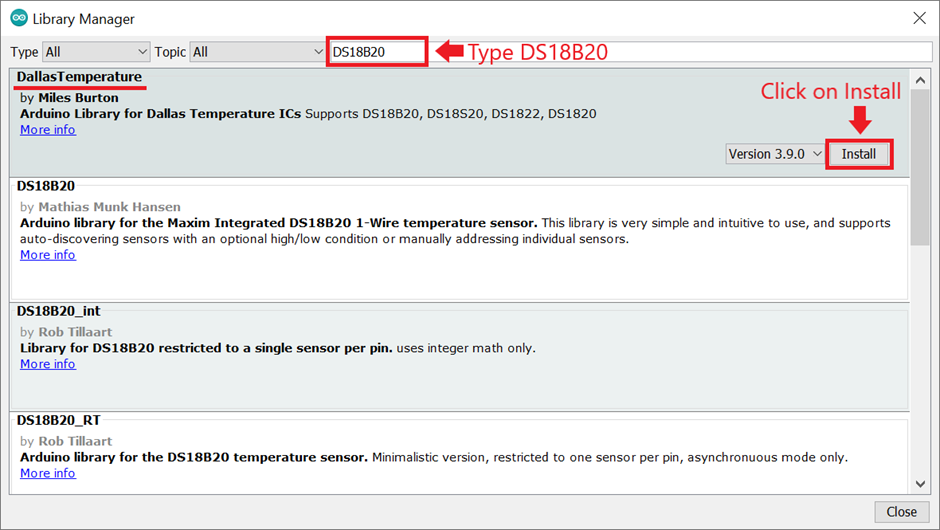
- If you don’t have the OneWire library, then this pop-up will come then click on Install all.
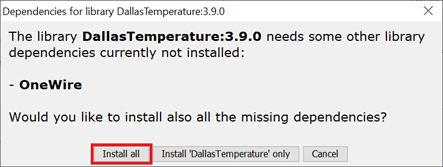
- Now open the simple example, to open it go to File ►Example ►DallasTemperature ► Simple
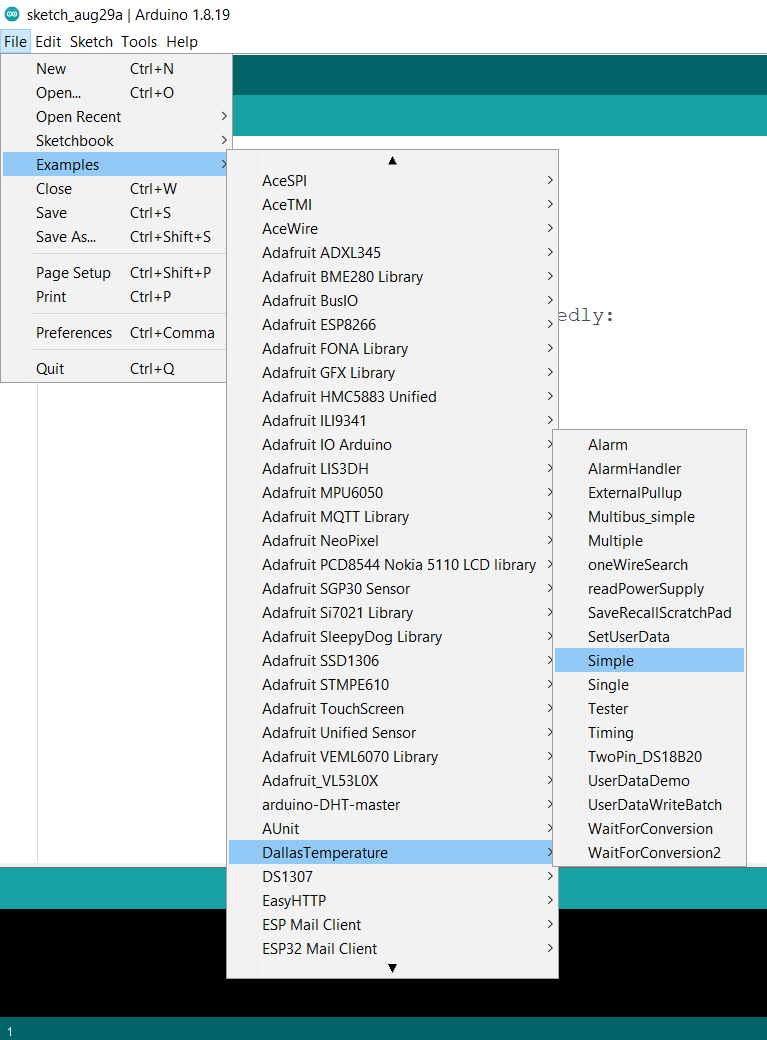
- Here we have modified the code as per the above example.
Code for DS18B20 Serial Monitor using Nodemcu
// Include the libraries we need
#include <OneWire.h>
#include <DallasTemperature.h>
// Data wire is plugged into port 2 on the Arduino
#define ONE_WIRE_BUS 2
// Setup a oneWire instance to communicate with any OneWire devices (not just Maxim/Dallas temperature ICs)
OneWire oneWire(ONE_WIRE_BUS);
// Pass our oneWire reference to Dallas Temperature.
DallasTemperature sensors(&oneWire);
/*
* The setup function. We only start the sensors here
*/
void setup(void)
{
// start serial port
Serial.begin(9600);
Serial.println("Dallas Temperature IC Control Library Demo");
// Start up the library
sensors.begin();
}
/*
* Main function, get and show the temperature
*/
void loop(void)
{
// call sensors.requestTemperatures() to issue a global temperature
// request to all devices on the bus
Serial.print("Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperatures
Serial.println("DONE");
// After we got the temperatures, we can print them here.
// We use the function ByIndex, and as an example get the temperature from the first sensor only.
float tempC = sensors.getTempCByIndex(0);
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
}
else
{
Serial.println("Error: Could not read temperature data");
}
}
Nodemcu serial monitor output for DS18B20
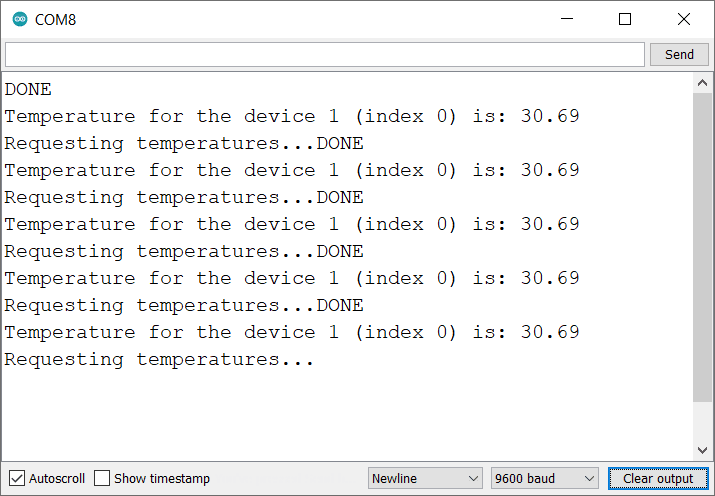
Let’s Understand the code
First initialize the DallasTemperature and OneWire Library.
#include <OneWire.h>
#include <DallasTemperature.h>
Set the pin number D2 for DS1307 of Nodemcu
#define ONE_WIRE_BUS D2
Setup a oneWire
instance to communicate with any OneWire
devices
OneWire oneWire(ONE_WIRE_BUS);
Pass oneWire reference to DallasTemperature
.
DallasTemperature sensors(&oneWire);
In setup function,
We have initiated the serial communication with a 9600 Baud rate and initialized the dallasTemperature object using sensors.begin() function.
void setup(void)
{
// start serial port
Serial.begin(9600);
Serial.println("Dallas Temperature IC Control Library Demo");
// Start up the library
sensors.begin();
}
In loop function
Call sensors.requestTemperatures()
to issue a global temperature request to all devices on the bus
sensors.requestTemperatures(); // Send the command to get temperatures
After getting the temperature, we saved it on the tempC
float variable.
Here we have connected only one temperature sensor if you have multiple sensors then add in ByIndex function.
float tempC = sensors.getTempCByIndex(0);
Now check the received temperature status reading and print on the serial monitor
// Check if reading was successful
if(tempC != DEVICE_DISCONNECTED_C)
{
Serial.print("Temperature for the device 1 (index 0) is: ");
Serial.println(tempC);
}
else
{
Serial.println("Error: Could not read temperature data");
}
Code for Temperature Monitoring on the web server using DS18B20 and Nodemcu
#include <OneWire.h>
#include <DallasTemperature.h>
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include "html.h"
#define DS18B20PIN D2 /* Connect DS18B20 to Pin No D2 of Nodemcu*/
OneWire oneWire(DS18B20PIN);
DallasTemperature sensor(&oneWire);
ESP8266WebServer server(80);
float _temperature;
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send the code to the web server*/
}
void Temp() {
String TempValue = String(_temperature); //Convert it into string
server.send(200, "text/plane", TempValue); //Send updated temperature value to the web server
}
void setup(void){
Serial.begin(115200); /*Set the baudrate to 115200*/
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");}
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
sensor.begin();
server.on("/", MainPage); /*Display the Web/HTML Page*/
server.on("/readTemp", Temp); /*Display the updated Temperature and Humidity value*/
server.begin(); /*Start Server*/
delay(1000); /*Wait for 1000mS*/
}
void loop(void){
sensor.requestTemperatures();
_temperature = sensor.getTempCByIndex(0); /* Read the temperature */
Serial.print("Temperature = ");
Serial.print(_temperature); /* Print Temperature on the serial window */
Serial.println("ºC");
server.handleClient();
delay(1000); /* Wait for 1000mS */
}
- Now upload the code.
- After uploading the code open the serial monitor and set the baud rate to 115200 then reset the Nodemcu board and check the IP address as shown in the below image
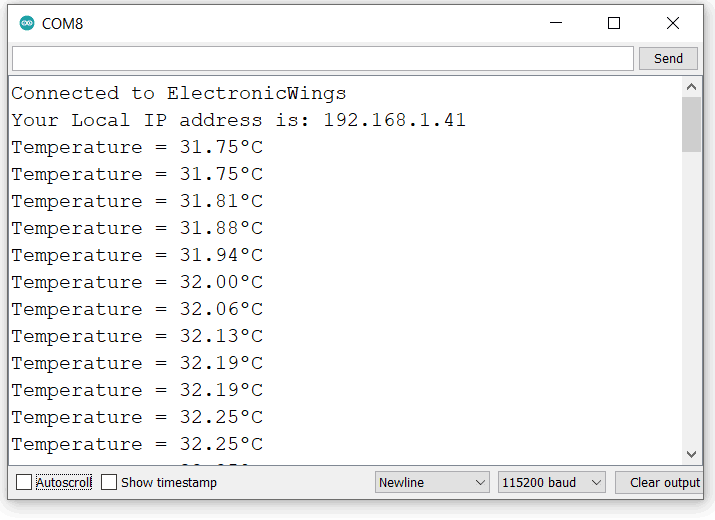
- Now open any mobile browser type the IP address that is shown in the serial monitor and hit the enter button.
- If all is okay, then the web page will start the showing current temperature and humidity on the web server like in the below image.
Note: make sure your Nodemcu and mobile are connected to the same router/server, if they are connected to the same router or server then only you will be able to visible the web page.
Final Output on the webserver
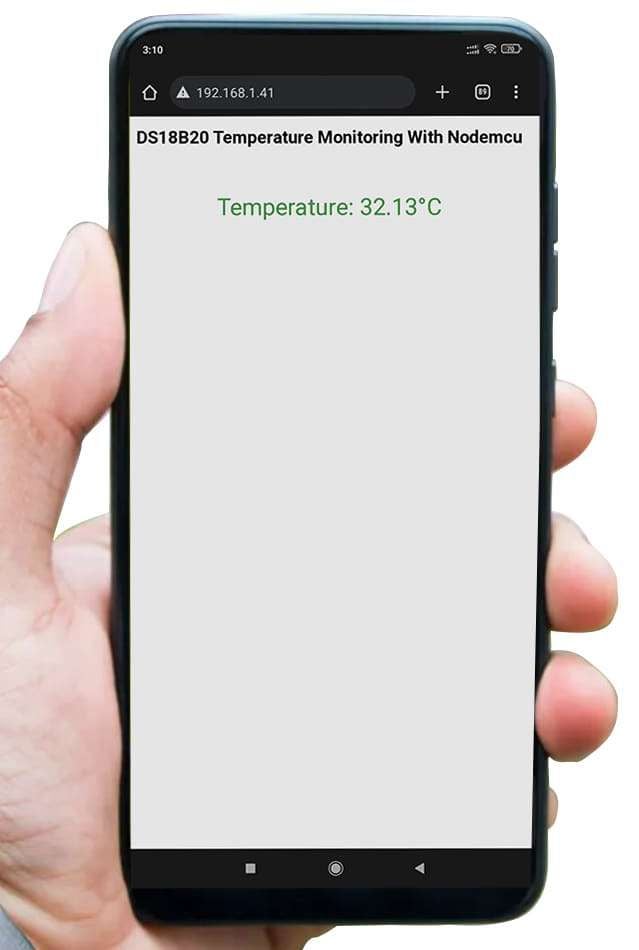
Let’s Understand the code.
To understand this code, please refer to the basics guide of “How to create the ESP32 Server”.
Once you get the basics of Nodemcu server creation, it will be very simple to understand the code.
This code starts with important header files and libraries, In WiFi.h
file contains all Nodemcu WiFi related definitions, here we have used them for network connection purposes.
The WebServer.h
file supports handling the HTTP GET and POST requests as well as setting up a server. In the html.h file contains all the web page code, In one wire library contains the I2C related functions and then add the DallasTemperature.h library file.
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include "html.h"
#include <OneWire.h>
#include <DallasTemperature.h>
Define the pin number for DS18B20 temperature sensor
#define DS18B20PIN D2 /* Connect DS18B20 to Pin No D2 of Nodemcu*/
Let’s define HTTP port i.e., Port 80 as follows
ESP8266WebServer server(80);
Setup Function:
In setup function, first we set the WiFi as an STA mode and connect to the given SSID and password
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");}
After successfully connecting to the server print the local IP address on the serial window.
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
Handling Client Requests and Serving the Page:
To handle the client request, we use server.on()
function.
It takes two parameters, The first is the requested URL path, and the second is the function name, which we want to execute.
As per the below code, when a client requests the root (/)
path, the “MainPage()
” function executes.
Also, when a client requests the “/readTemp
” path, The Temp()
function will be called.
server.on("/", MainPage); /*Client request handling: calls the function to serve HTML page */
server.on("/readTemp", Temp);/*Display the updated Temperature and Humidity value*/
Now start the server using server.begin() function.
server.begin(); /*Start Server*/
Functions for Serving HTML
We have defined the complete HTML page in a file named “html.h
” and added it in the header file. With the following function, we are sending the complete page to the client via server.send()
function.
While sending, we are passing the first parameter “200” which is the status response code as OK (Standard response for successful HTTP requests).
The second parameter is content type as “text/html
“, and the third parameter is HTML page code.
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send HTM page to Client*/
}
Now in the below function only we are sending the updated temperature values to the web page.
void Temp() {
String TempValue = String(_temperature); //Convert it into string
server.send(200, "text/plane", TempValue); //Send updated temperature value to the web server
}
In Loop Function,
Now to handle the incoming client requests and serve the relevant HTML page, we can use handleClient()
function. It executing relevant server.on()
as a callback function although it is defined in void setup().
So, it continuously serves the client requests.
server.handleClient();
HTML Web Page Code
This is a code for the web page that shows the measured temperature using a K-type thermocouple using MAX6675 and Nodemcu.
/*
Nodemcu HTML WebServer Page Code
http:://www.electronicwings.com
*/
const char html_page[] PROGMEM = R"rawSrting(
<!DOCTYPE html>
<html>
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
<body>
<h1>DS18B20 Temperature Monitoring With Nodemcu</h1><br>
<p>Temperature: <span id="TempValue">0</span>°C</p><br>
<script>
setInterval(function() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("TempValue").innerHTML = this.responseText;
}
};
xhttp.open("GET", "readTemp", true);
xhttp.send();
},50);
</script>
</body>
</html>
)rawSrting";
Let’s understand the code step by step.
All html pages start with the <!DOCTYPE html>
declaration, it is just information to the browser about what type of document is expected.
<!DOCTYPE html>
The html tag is the container of the complete html page which represents on the top of the html code.
<html>
Now here we are defining the style information for a web page using the <style>
tag. Inside the <style> tag we have defined the font name, size, color, and test alignment.
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
Inside the body, we are defining the document body, in below we have used headings, and paragraphs if you want you can add images, hyperlinks, tables, lists, etc. also.
On the web page, we are displaying the heading of the page, and inside a paragraph temperature values.
Now temperature value updates under the span id which is manipulated with JavaScript using the id attribute.
<body>
<h1>DS18B20 Temperature Monitoring With Nodemcu</h1><br>
<p>Temperature: <span id="TempValue">0</span>°C</p><br>
Now, this is the javascript that comes under the <script>
tag, this is also called a client-side script.
<script>
In setInterval()
method we are calling the function at every 50mS intervals.
setInterval(function() {},50);
here we are creating the html XMLHttpRequest
object
var xhttp = new XMLHttpRequest();
The xhttp.onreadystatechange
event is triggered every time the readyState changes and the readyState holds the status of the XMLHttpRequest.
Now in the below code, the ready state is 4 means the request finished and response is ready and the status is 200 which means OK.
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
const myArr = JSON.parse(this.responseText);
now here is the main thing, we are updating the Temperature values in html page using TempValue id.
document.getElementById("TempValue").innerHTML = this.responseText;
here we used the AJAX method to send the updated values to the server without refreshing the page.
In the below function, we have used the GET method and sent the readTemp function which we defined in the main code asynchronously.
xhttp.open("GET", "readTemp", true);
Send the request to the server using xhttp.send();
function.
xhttp.send();
Close the script
</script>
Close the body
</body>
Close the html.
</html>
Components Used |
||
---|---|---|
NodeMCU NodeMCUNodeMCU |
X 1 | |
DS18B20 Waterproof temperature sensor DS18B20 Waterproof temperature sensor |
X 1 |