Overview
Google assistant is AI (Artificial Intelligence) based voice command service. Using voice, we can interact with the google assistant and it can search on the internet, schedule events, set alarms, control appliances, etc.
This service is available on smartphones and Google Home devices.
We can control smart home devices including lights, switches, fans, and thermostats using our Google Assistant.
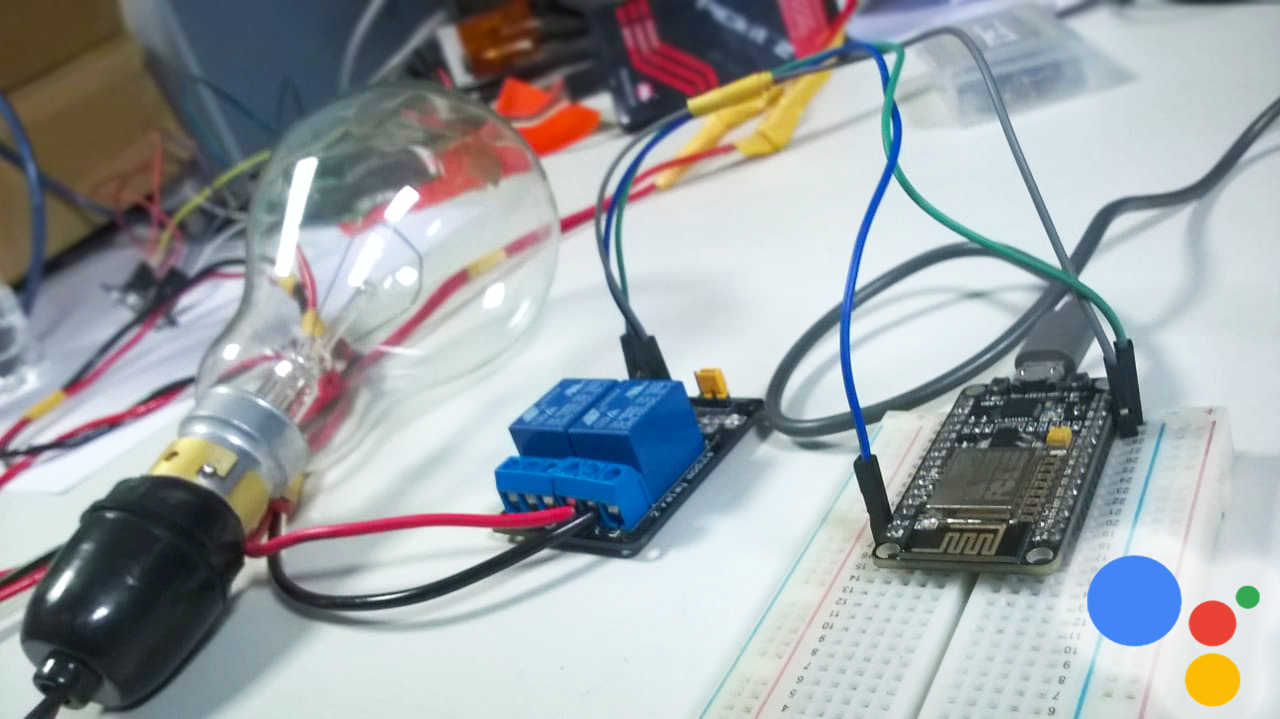
We will build an application that can control home appliances. Here, we will control a 60W bulb using Google Assistant service.
This application includes Google assistant along with Adafruit server and IFTTT service.
Hardware Used
- NodeMCU – 32-bit ESP8266 development board with Wi-Fi SoC.
- Relay module
- One 100 W Bulb
To build a home automation application, I used three different platforms
- Google Assistant
- Adafruit
- IFTTT
To use the above services we need to configure them.
Adafruit
First, created an account at www.Adafruit.io
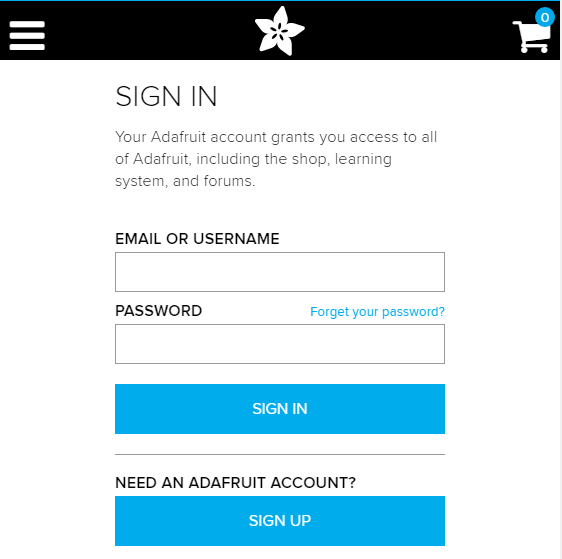
Now, create dashboard at Adafruit. This dashboard is a user interface to control things remotely.
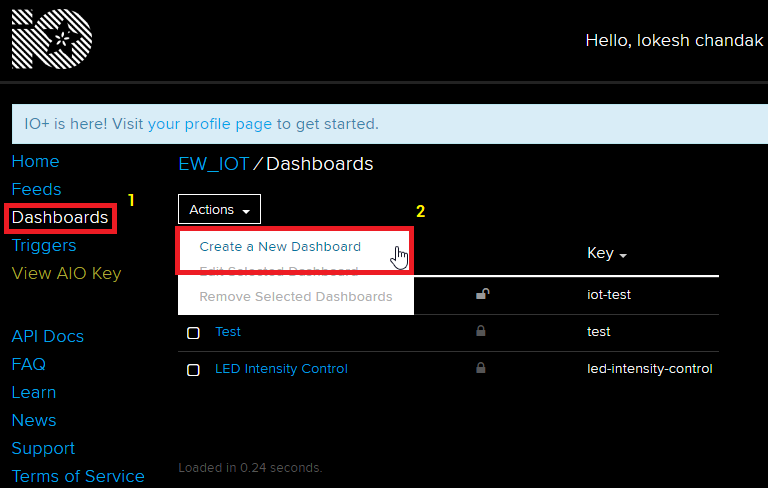
After following the above steps, provide a name to the dashboard and save it. We can see our dashboard as follows,
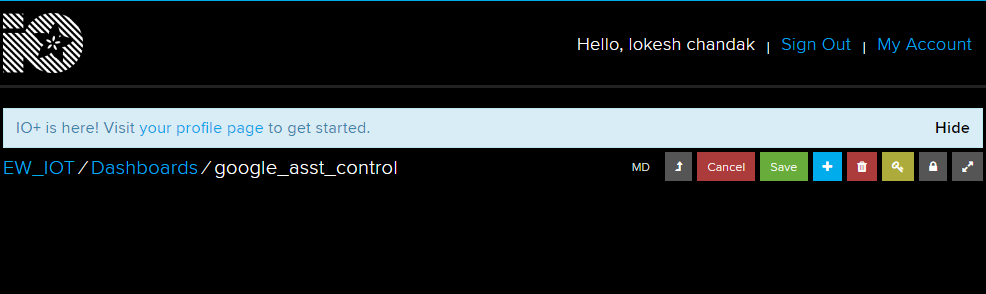
Now, create a feed (user interface) to control light On-Off. To create it, just click on the ‘+’ symbol and select the toggle feed shown below,
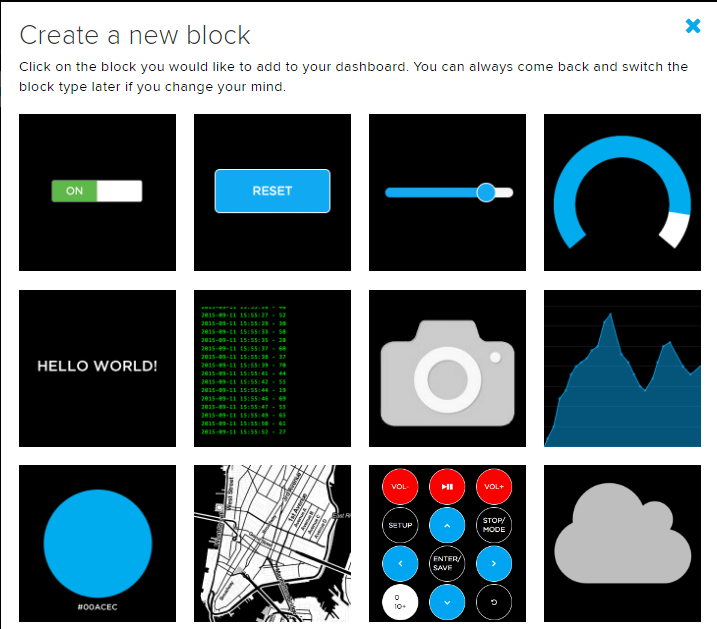
After selecting the toggle feed, a pop-up window appears as shown below.
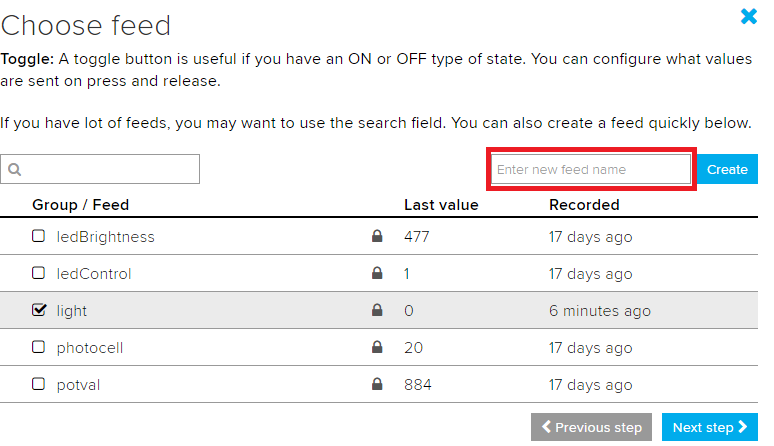
Enter the name of our feed (shown in the red box) and create it. After creation, select the created feed (here mine is light) and then click on the Next step.
In the next step configure the feed which is shown below,
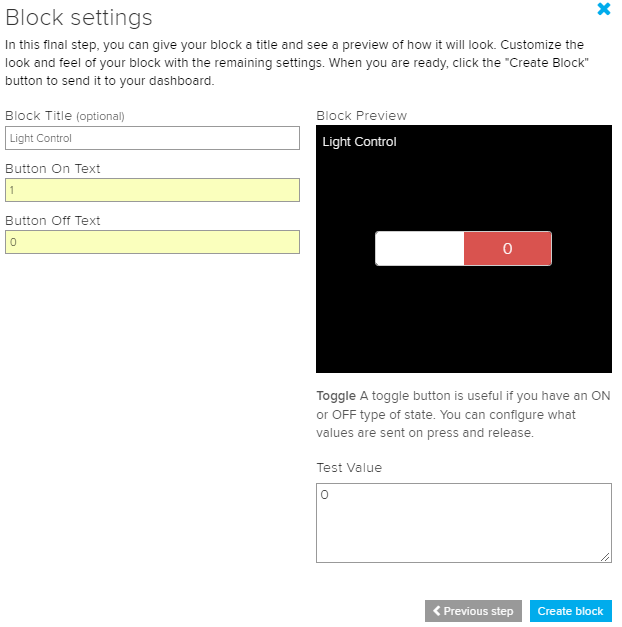
Here, I used 0(OFF) and 1(ON) text for button and then click on create. This will create a toggle button on your dashboard which can be used to control things remotely.
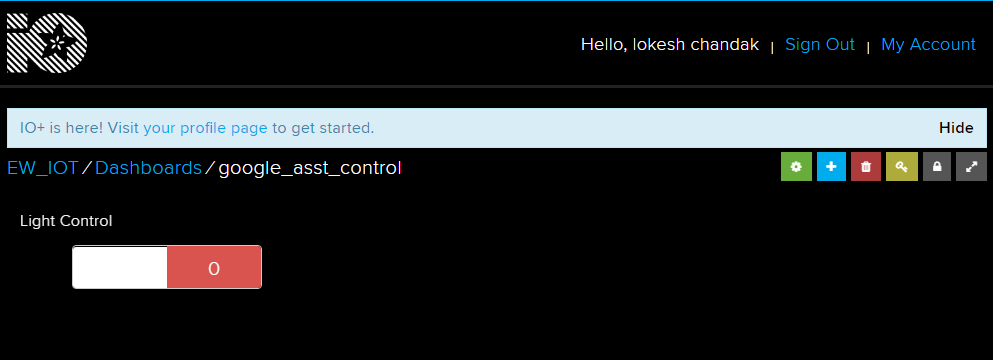
Now, my dashboard is ready for IoT applications like home automation.
IFTTT (If This Then That)
If This Then That, also known as IFTTT is a free web-based service to create chains of simple conditional statements, called applets. An applet is triggered by changes that occur within other web services such as Gmail, Facebook, Telegram, Instagram, or Pinterest.
For example, an applet may send an e-mail message if the user tweets using a hashtag or copy a photo on Facebook to a user's archive if someone tags a user in a photo.
Here, I used IFTTT to use google assistant service and Adafruit service in the chain. So, when I use google assistant to control the light of my home by saying Ok Google, turn the light ON or OFF. Then IFTTT interprets the message and can send it to Adafruit’s dashboard as an understandable command to the created feed.
Configure IFTTT
The first step is creating an account on IFTTT.
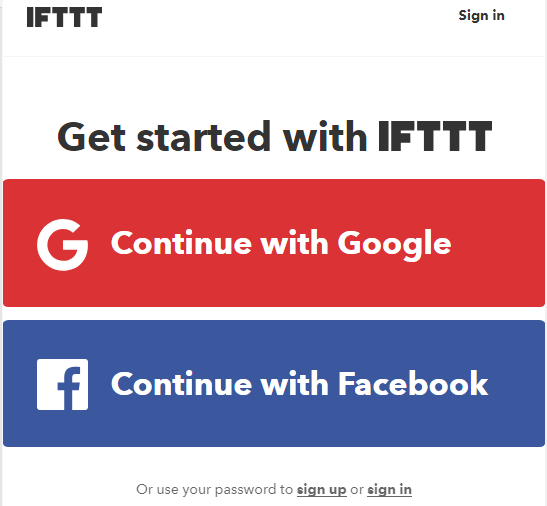
Note: Create an account on IFTTT by using the same e-mail id which you have used for Adafruit.
After account creation, click on My Applets and then select New Applet shown below,
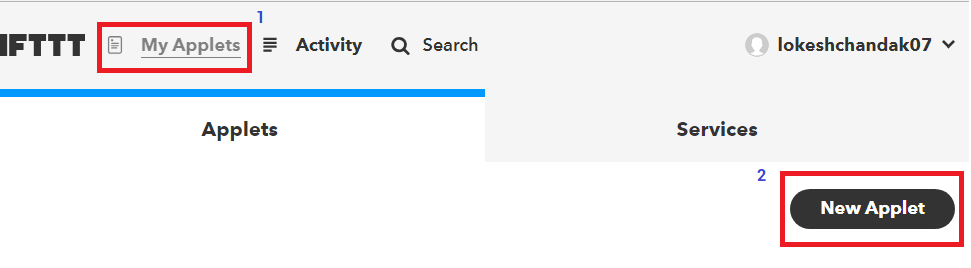
After selecting a new applet, we get a new page in which we should click on to This as shown in the below image.
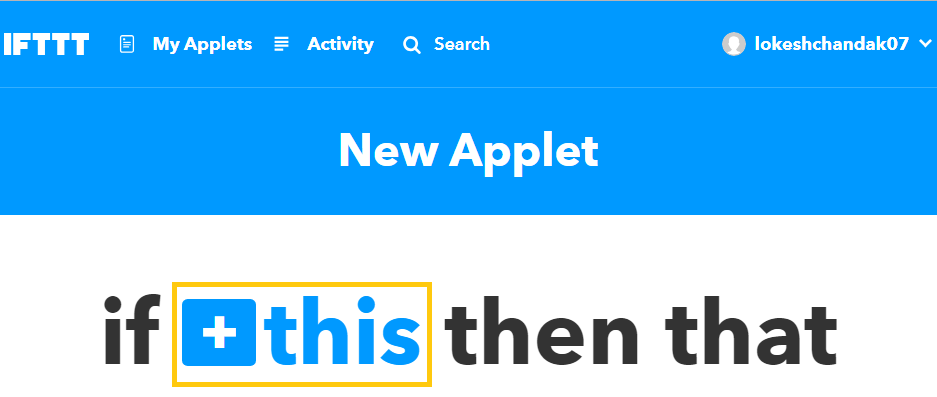
Then search for Google Assistant and select it.
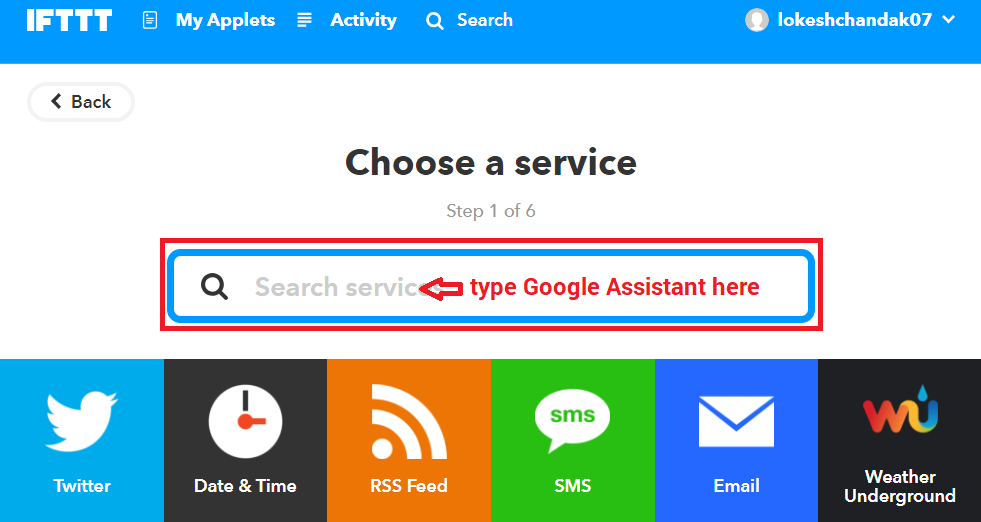
Now, enter voice phrases which we will use as a command for google assistant.
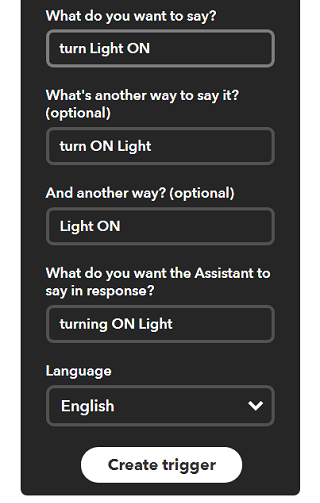
We can enter any phrase as per our application. As you can see, the phrases entered in the above fields is for making Light ON. For making Light OFF, we have to create another applet with different phrases.
Now, we get another page on which we have to click on that option which is used to connect Google Assistant with Adafruit.
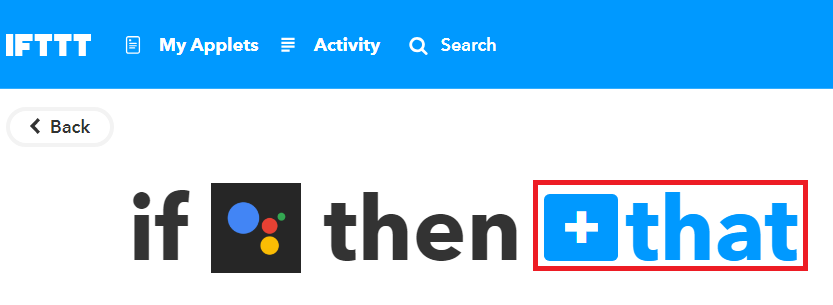
Then search for Adafruit and select it.
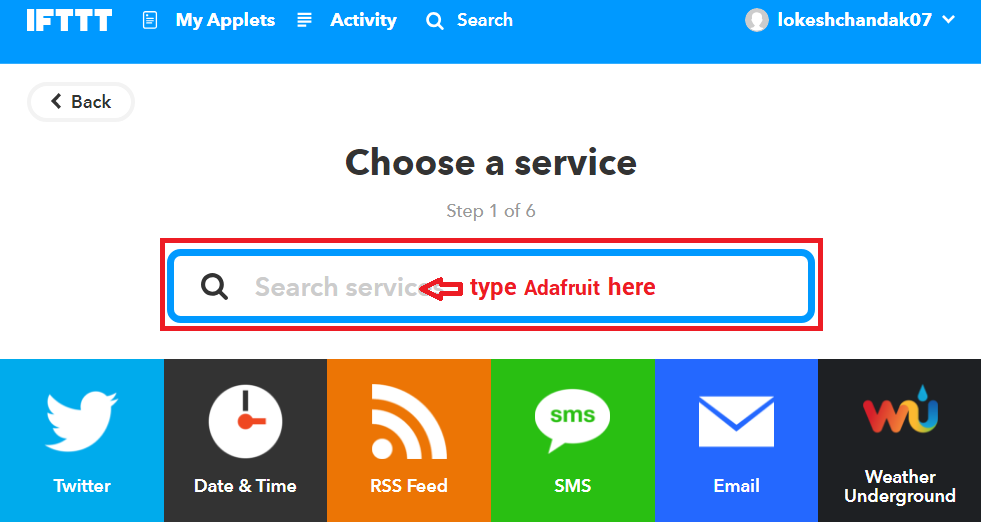
After selecting Adafruit, choose action as shown below,
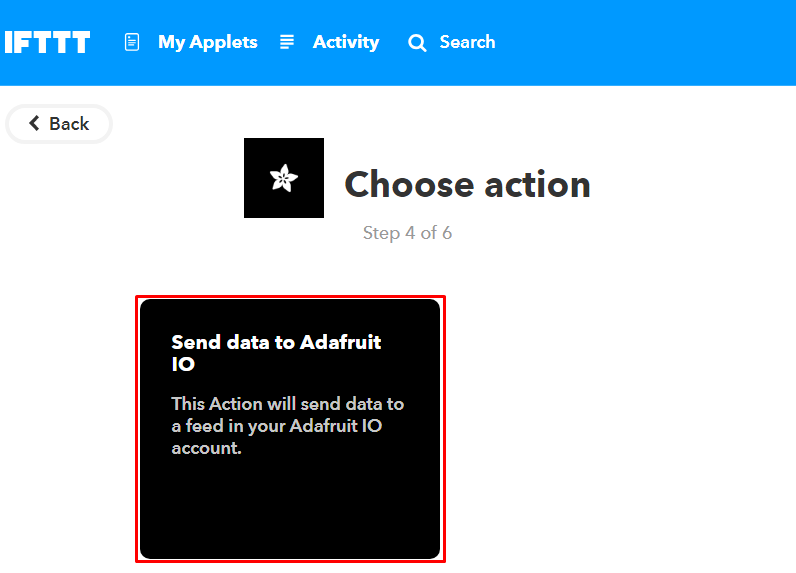
Now enter what data we need to send to which feed of Adafruit dashboard.
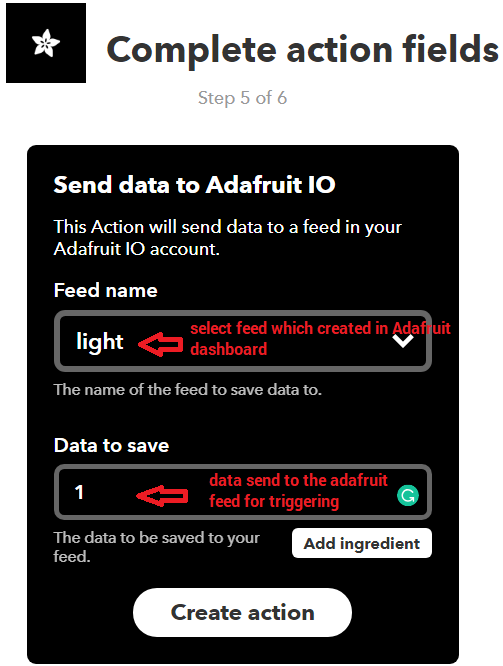
Click on Create Action.
So, when I use Google Assistant on my mobile and give a voice command as “Ok Google, Turn LED ON”, the applet created in IFTTT receives this command and will send data ‘1’ to the Adafruit feed. This will trigger the event on the Adafruit dashboard which is continuously monitored by the microcontroller (here NodeMCU). This microcontroller will take action as per the data change on the Adafruit dashboard.
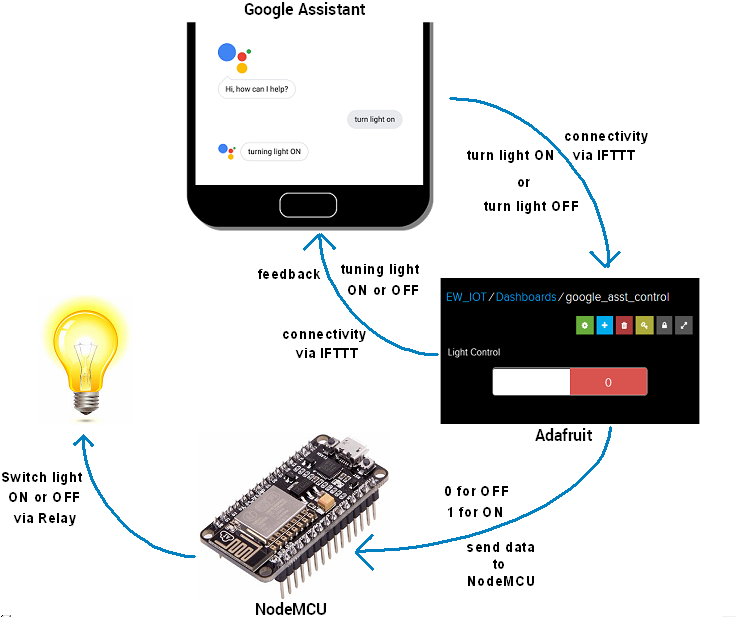
Connection Diagram of Relay Module with NodeMCU
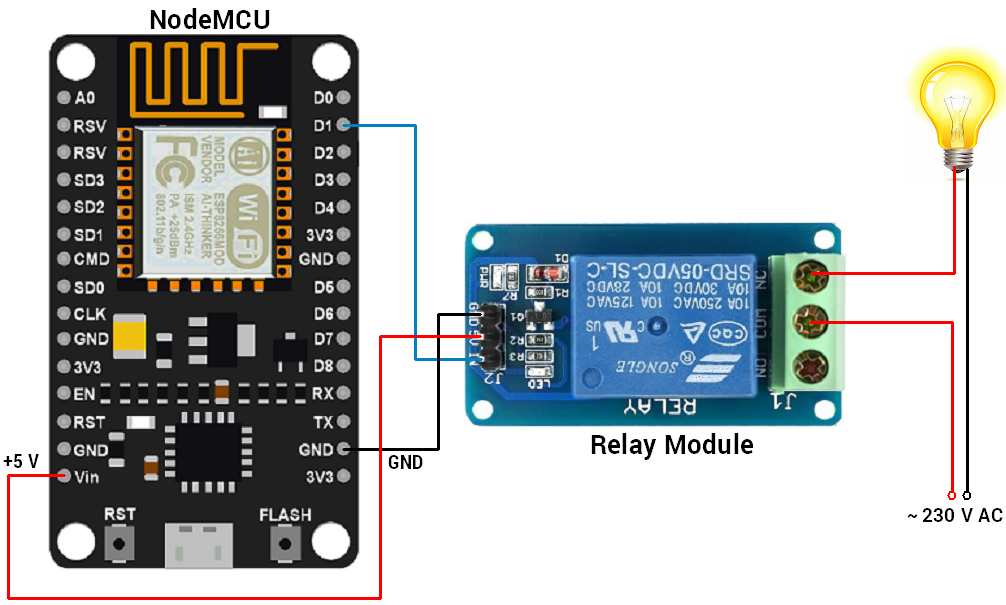
Library
Here, I used the Adafruit MQTT library for receiving data from the Adafruit server. To install this library, select option Sketch -> Include Library -> Manage Libraries.
In that library, search for Adafruit MQTT and installed it.
Control Home’s Light using Google Assistant and NodeMCU
I build an IoT based home automation application in which I control the 100 W bulb at remotely using AI-based Google Assistant.
Here, I used NodeMCU to read data from the Adafruit server and act accordingly. 100 W bulb connected to NodeMCU via relay for controlling its voice command using google assistant.
Program
#include <ESP8266WiFi.h>
#include "Adafruit_MQTT.h"
#include "Adafruit_MQTT_Client.h"
#define Relay1 D1
#define WLAN_SSID "Enter SSID NAME" // Your SSID
#define WLAN_PASS "SSID Password" // Your password
/************************* Adafruit.io Setup *********************************/
#define AIO_SERVER "io.adafruit.com"
#define AIO_SERVERPORT 1883 // use 8883 for SSL
#define AIO_USERNAME "Enter your UserName" // Replace it with your username
#define AIO_KEY "Enter you AIO_KEY" // Replace with your Project Auth Key
/************ Global State (you don't need to change this!) ******************/
// Create an ESP8266 WiFiClient class to connect to the MQTT server.
WiFiClient client;
// or... use WiFiFlientSecure for SSL
//WiFiClientSecure client;
// Setup the MQTT client class by passing in the WiFi client and MQTT server and login details.
Adafruit_MQTT_Client mqtt(&client, AIO_SERVER, AIO_SERVERPORT, AIO_USERNAME, AIO_KEY);
/****************************** Feeds ***************************************/
// Setup a feed called 'onoff' for subscribing to changes.
Adafruit_MQTT_Subscribe Light1 = Adafruit_MQTT_Subscribe(&mqtt, AIO_USERNAME"/feeds/Enter your Feed Name"); // FeedName
void MQTT_connect();
void setup() {
Serial.begin(115200);
pinMode(Relay1, OUTPUT);
// Connect to WiFi access point.
Serial.println(); Serial.println();
Serial.print("Connecting to ");
Serial.println(WLAN_SSID);
WiFi.begin(WLAN_SSID, WLAN_PASS);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println();
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
// Setup MQTT subscription for onoff feed.
mqtt.subscribe(&Light1);
}
void loop() {
MQTT_connect();
Adafruit_MQTT_Subscribe *subscription;
while ((subscription = mqtt.readSubscription(5000))) {
if (subscription == &Light1) {
Serial.print(F("Got: "));
Serial.println((char *)Light1.lastread);
int Light1_State = atoi((char *)Light1.lastread);
digitalWrite(Relay1, !(Light1_State));
}
}
}
void MQTT_connect() {
int8_t ret;
// Stop if already connected.
if (mqtt.connected()) {
return;
}
Serial.print("Connecting to MQTT... ");
uint8_t retries = 3;
while ((ret = mqtt.connect()) != 0) { // connect will return 0 for connected
Serial.println(mqtt.connectErrorString(ret));
Serial.println("Retrying MQTT connection in 5 seconds...");
mqtt.disconnect();
delay(5000); // wait 5 seconds
retries--;
if (retries == 0) {
// basically die and wait for WDT to reset me
while (1);
}
}
Serial.println("MQTT Connected!");
}
Components Used |
||
---|---|---|
Relay Module Single Channel Relay Module Single Channel |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 | |
ESP12F ESP12E |
X 1 |
Downloads |
||
---|---|---|
|
GoogleAssitance_Automation_ | Download |