Introduction
An interrupt is an event that occurs randomly in the flow of continuity. It is just like a call you have when you are busy with some work and depending upon call priority you decide whether to attend or neglect it.
NodeMCU based ESP8266 has an interrupt feature on its GPIO pins. This function is available on D0-D8 pins of NodeMCU Dev Kit.
GPIO pin interrupts are supported through Arduino interrupt functions i.e. attachInterrupt, detachInterrupt.
Interrupts can be attached to any GPIO pin, except the D0/GPIO16 pin. Standard Arduino interrupt types are supported i.e. CHANGE, RISING, FALLING.
Arduino Interrupt functions for NodeMCU
Let’s see the functions that are used to set interrupts for the NodeMCU kit in Arduino IDE.
attachInterrupt()
This function is used to attach an interrupt on the specified pin.
Syntax: attachInterrupt(pin, ISR(callback function), interrupt type/mode);
Parameters:
pin
: The pin number for which interrupt is to be set.
ISR (callback function)
: The ISR to call when the interrupt occurs; this function does not take any parameters and returns nothing.
Interrupt type/mode
: It defines when the interrupt should be triggered.
CHANGE: Used to trigger the interrupt whenever pin value changes.
RISING: Used to trigger an interrupt when the pin goes from low to high.
FALLING: Used to trigger an interrupt when the pin goes from high to low.
Returns: null
detachInterrupt()
This function is used to disable the interrupt on the specified GPIO pin.
Syntax: detachInterrupt(pin)
Parameters:
pin
: The GPIO pin of the interrupt to disable.
Returns: null
Example
Let’s write an Arduino sketch to set a rising edge-interrupt on the 2nd GPIO pin of NodeMCU. Here we connect a switch on the 2nd GPIO pin to generate a rising edge interrupt on it. Also, we are going to print the time (in milliseconds) of interrupt occurred on the serial monitor window.
Interfacing Diagram
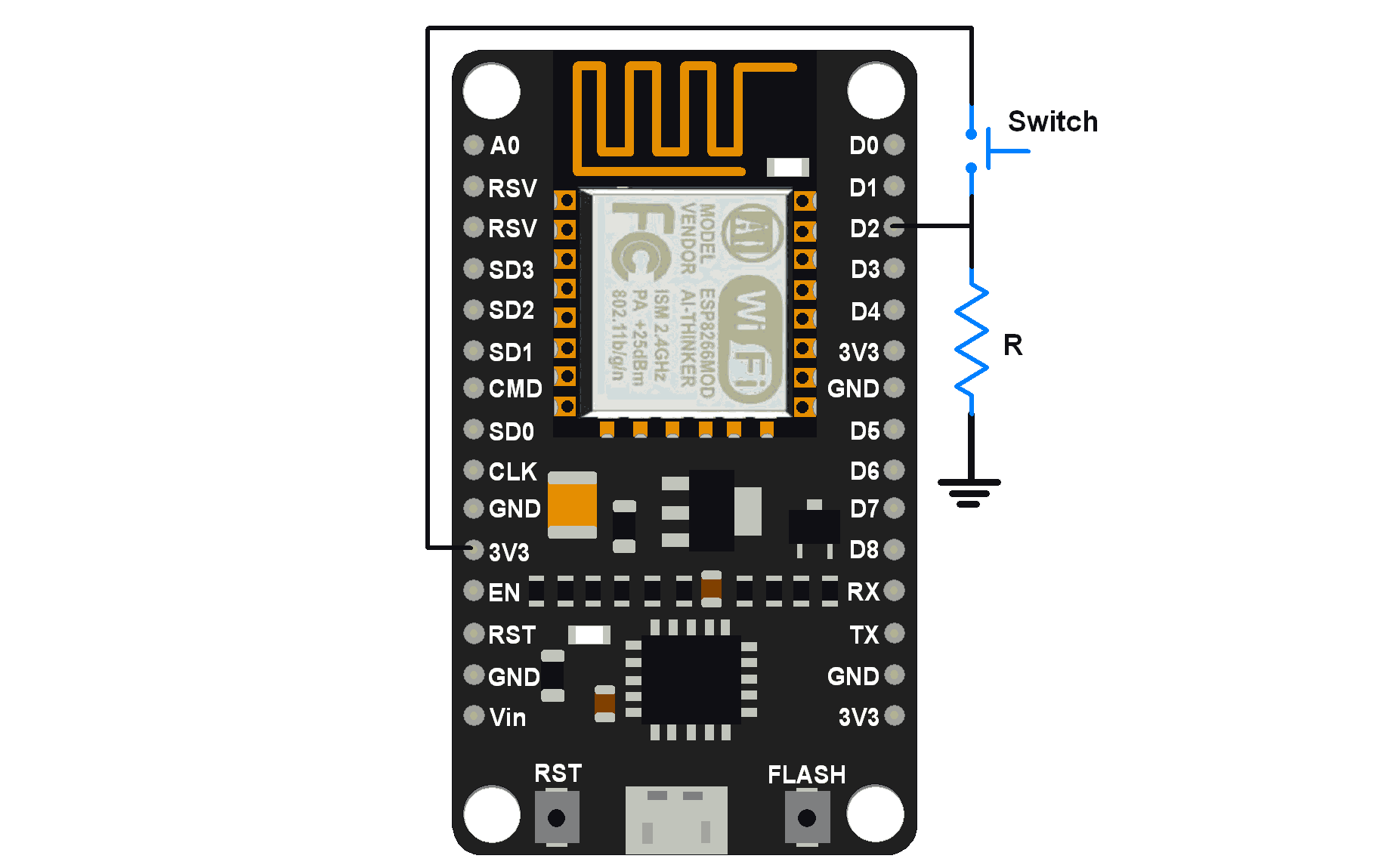
Arduino Sketch for GPIO Interrupt
uint8_t GPIO_Pin = D2;
void setup() {
Serial.begin(9600);
attachInterrupt(digitalPinToInterrupt(GPIO_Pin), IntCallback, RISING);
}
void loop() {
}
void IntCallback(){
Serial.print("Stamp(ms): ");
Serial.println(millis());
}
Output Window
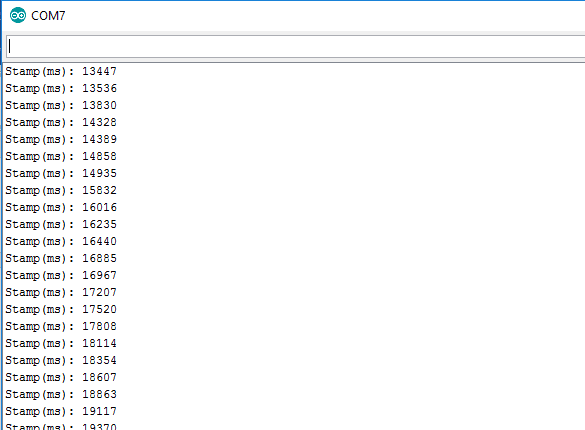
Components Used |
||
---|---|---|
NodeMCU NodeMCUNodeMCU |
X 1 | |
ESP12F ESP12E |
X 1 |