Description
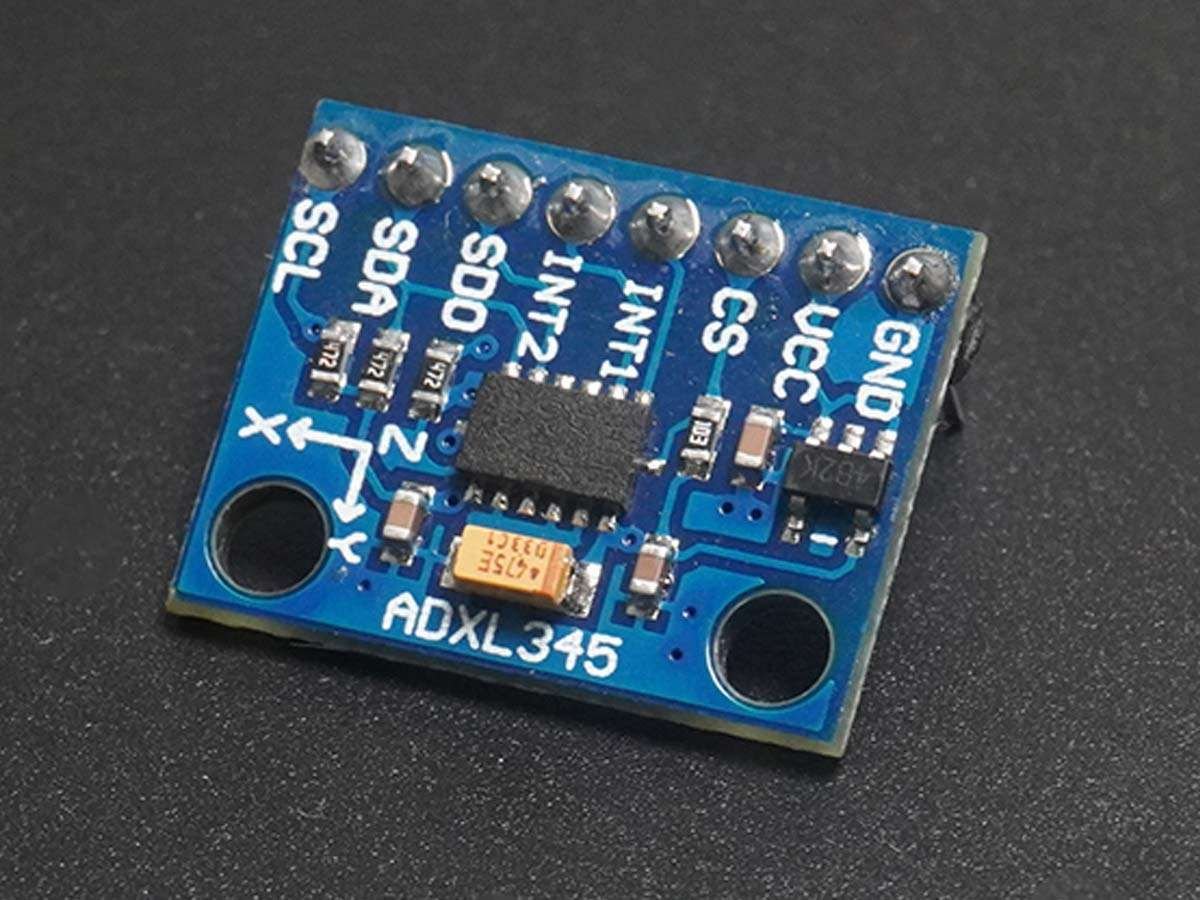
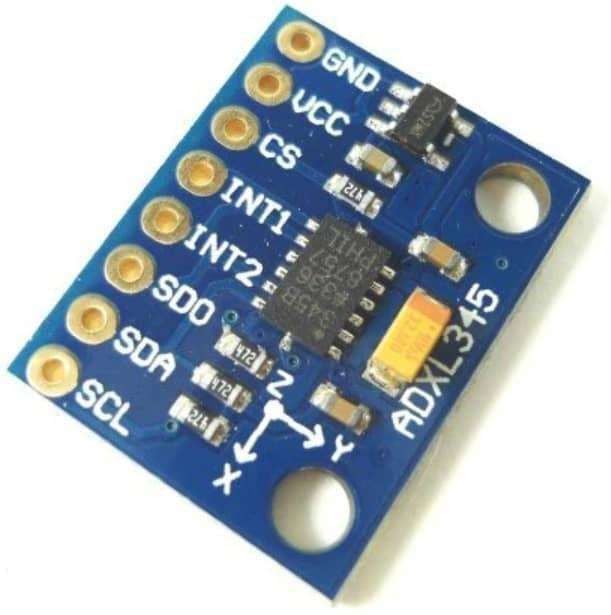
- Accelerometer is an electromechanical device that measures the force of acceleration caused by gravity in terms of g.
- On the surface of the earth, 1g corresponds to a 9.8 m/s2 acceleration. That is 1/6th on the moon and 1/3rd on Mars.
- The accelerometer is commonly used in gaming, robotics, tilt sensing, and dynamic motion sensing.
- ADXL345 has low power consumption and high sensitivity hence is ideal and portable
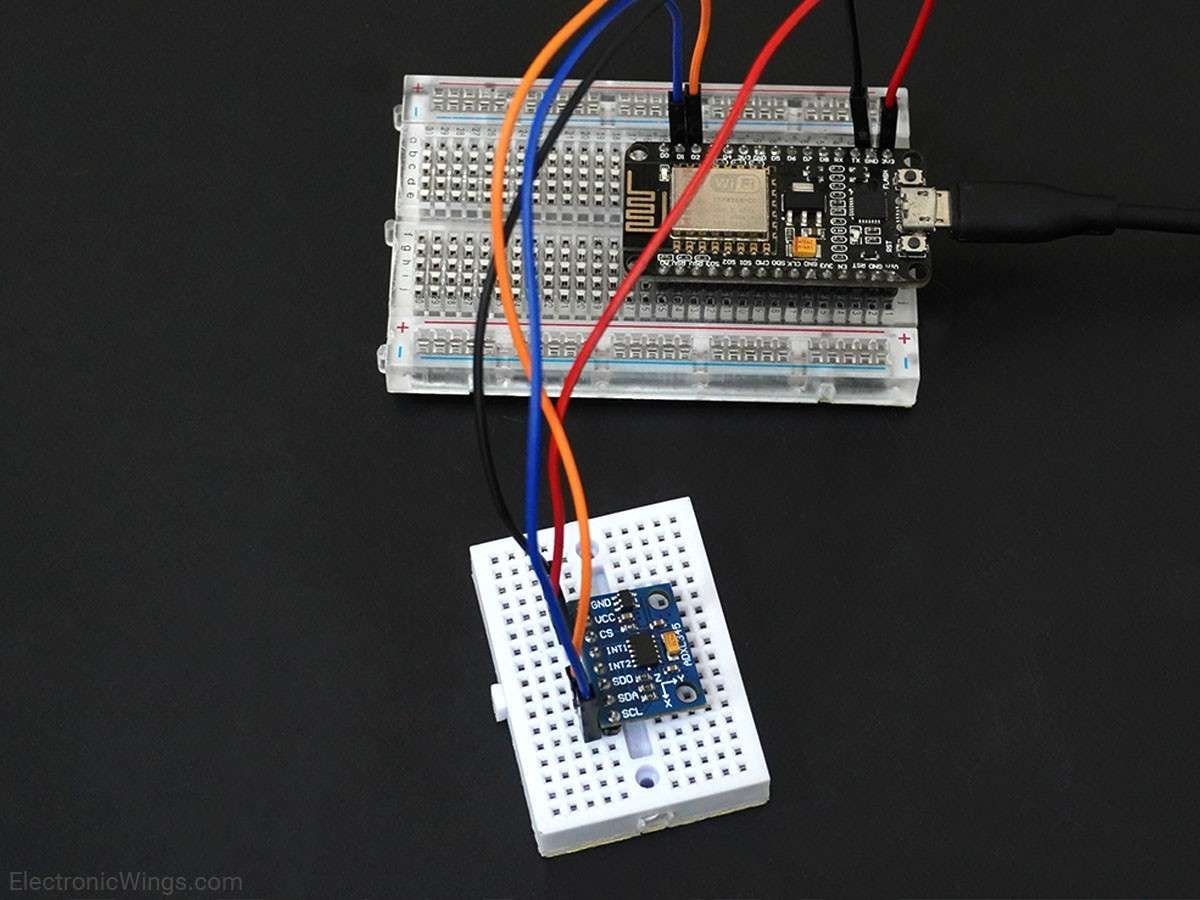
ADXL345 module Specification
- The ADXL345 is capable of measuring 3-axis acceleration in the range of
- X: -235 to +270
- Y: -240 to +260
- Z: -240 to +270
- The module gives output in I2C and SPI Digital form.
- This module can measure resolution up to 13-bit with the range of ±16 g in the x, y, and z-axis.
- The module consumes 40uA of current in the measurement mode and 0.1uA in standby mode.
- On-board Low drop-out (LDO) Voltage regulator
- The module has a bandwidth of 0.10Hz to 1600 Hz
- On-board MOSFET-based level shifter (Voltage level converter)
- It contains a polysilicon surface-micro machined sensor built on the top of a silicon wafer to provide resistance against forces due to applied acceleration.
ADXL345 Module Pinout
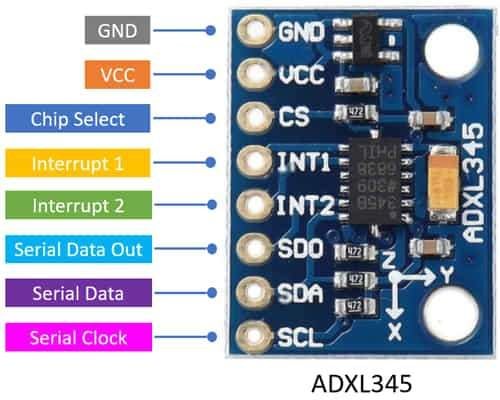
ADXL345 Pin Description
- VCC: Power supply pin connects in the range of 3 to 5.5V DC.
- GND: Connect to Supply ground.
- CS (Chip Select): Chip Select Pin.
- INT1 (Interrupt 1): Interrupt 1 Output Pin
- INT2 (Interrupt 2): Interrupt 2 Output Pin
- SDO (Serial Data Out): Serial Data Output (SPI 4-Wire)/Alternate I2C Address Select (I2C).
- SDA (Serial Data): Serial Data (I2C)/Serial Data Input (SPI 4-Wire)/Serial Data Input and Output (SPI 3-Wire).
- SCL (Serial Clock): Serial Communications Clock. SCL is the clock for I2C, and SCLK is the clock for SPI.
ADXL345 Hardware Connection with NodeMCU
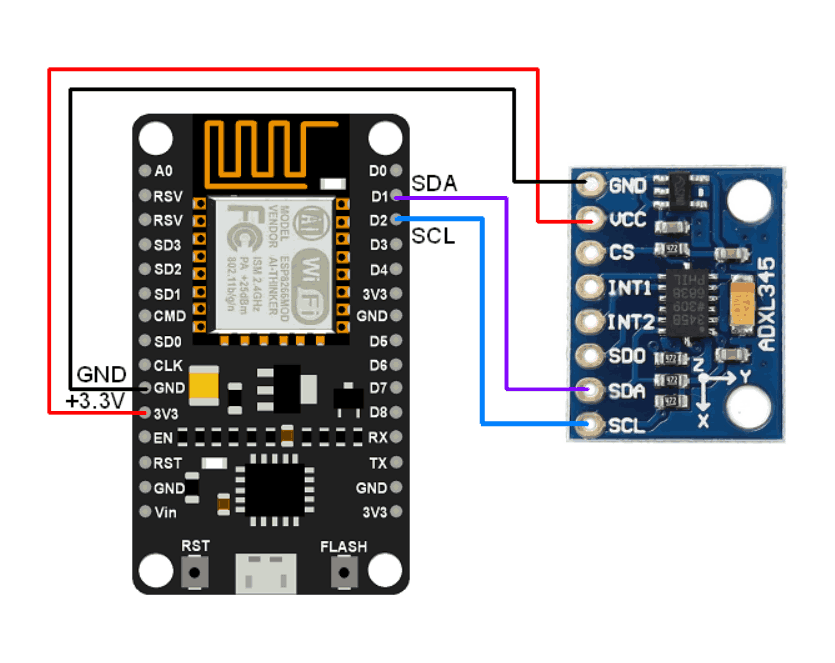
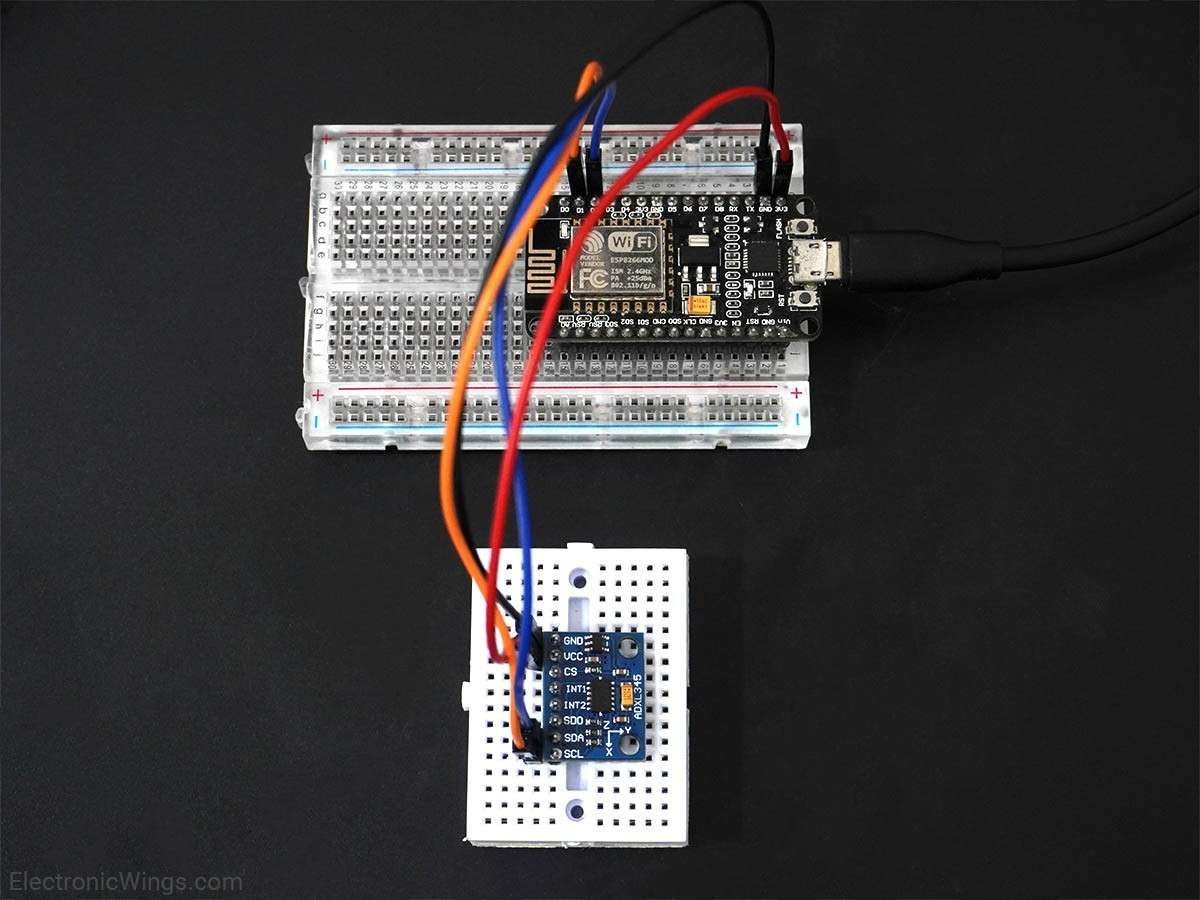
Read Accelerations using ADXL345 and NodeMCU
To find the roll and pitch of the device we will use the I2C interface of the accelerometer and display it on the serial monitor of Arduino IDE and a local host web server.
For the interface, we will use Adafruit libraries. We can install it from Arduino IDE Library Manager by searing Adafruit ADXL345 in the search box.
Open the Arduino IDE and navigate to Sketch ► Include Library ► Manage Libraries…
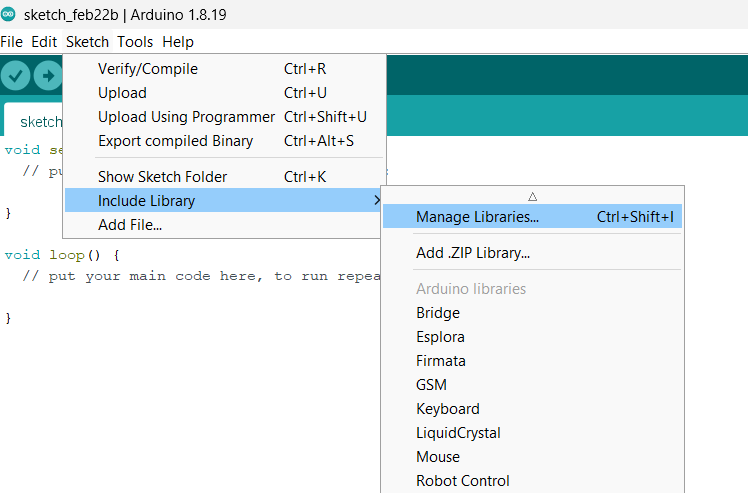
The library Manager window will pop up. Now type ADXL345 into the search box and click Install on the Adafruit ADXL345 option to install version 1.3.1 or higher.
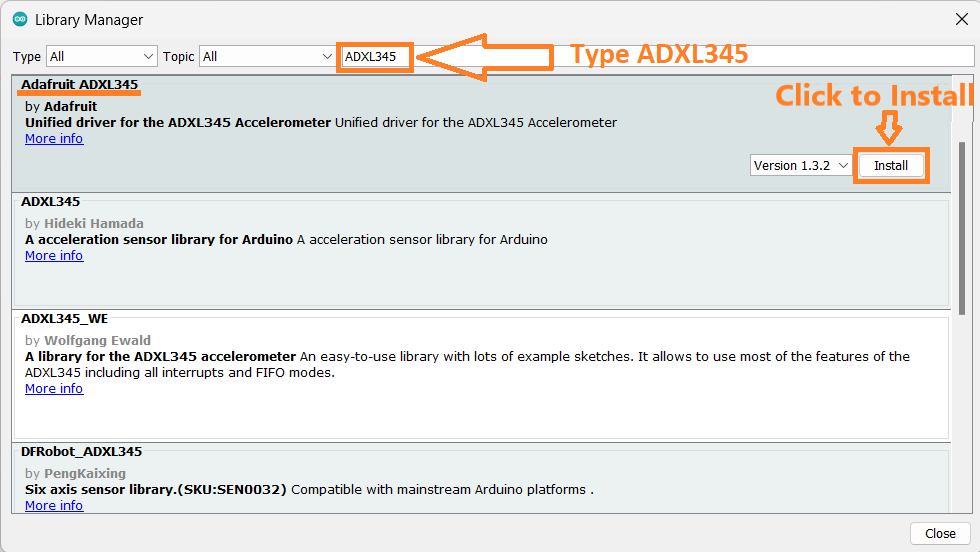
Now open an example of Adafruit ADXL345 io dashboard. To open it navigate to File ► Examples ► Adafruit ADXM345 ► sensortest
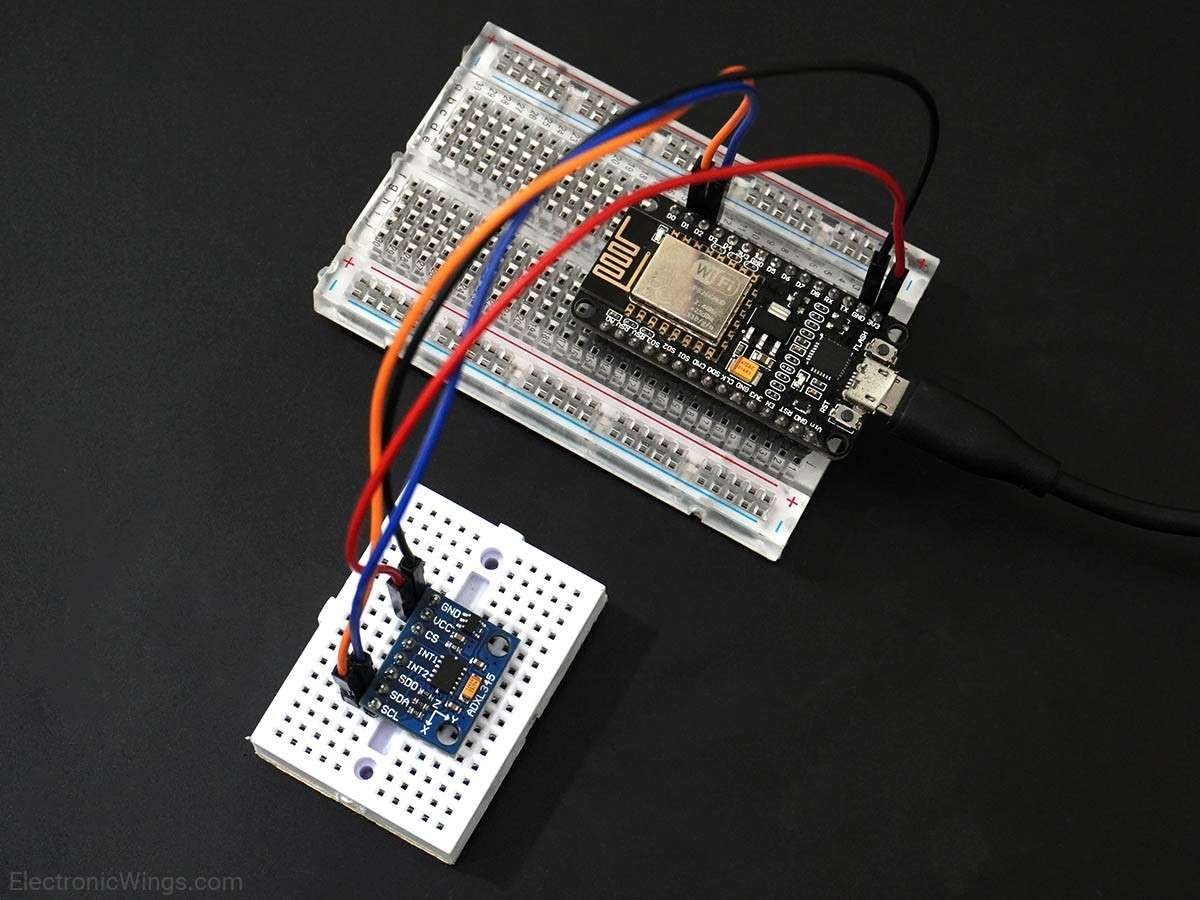
Here has used the same example below
ADXL345 Accelerometer Code for NodeMCU
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
/* Assign a unique ID to this sensor at the same time */
Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345);
void displaySensorDetails(void)
{
sensor_t sensor;
accel.getSensor(&sensor);
Serial.println("------------------------------------");
Serial.print ("Sensor: "); Serial.println(sensor.name);
Serial.print ("Driver Ver: "); Serial.println(sensor.version);
Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id);
Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" m/s^2");
Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" m/s^2");
Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" m/s^2");
Serial.println("------------------------------------");
Serial.println("");
delay(500);
}
void displayDataRate(void)
{
Serial.print ("Data Rate: ");
switch(accel.getDataRate())
{
case ADXL345_DATARATE_3200_HZ:
Serial.print ("3200 ");
break;
case ADXL345_DATARATE_1600_HZ:
Serial.print ("1600 ");
break;
case ADXL345_DATARATE_800_HZ:
Serial.print ("800 ");
break;
case ADXL345_DATARATE_400_HZ:
Serial.print ("400 ");
break;
case ADXL345_DATARATE_200_HZ:
Serial.print ("200 ");
break;
case ADXL345_DATARATE_100_HZ:
Serial.print ("100 ");
break;
case ADXL345_DATARATE_50_HZ:
Serial.print ("50 ");
break;
case ADXL345_DATARATE_25_HZ:
Serial.print ("25 ");
break;
case ADXL345_DATARATE_12_5_HZ:
Serial.print ("12.5 ");
break;
case ADXL345_DATARATE_6_25HZ:
Serial.print ("6.25 ");
break;
case ADXL345_DATARATE_3_13_HZ:
Serial.print ("3.13 ");
break;
case ADXL345_DATARATE_1_56_HZ:
Serial.print ("1.56 ");
break;
case ADXL345_DATARATE_0_78_HZ:
Serial.print ("0.78 ");
break;
case ADXL345_DATARATE_0_39_HZ:
Serial.print ("0.39 ");
break;
case ADXL345_DATARATE_0_20_HZ:
Serial.print ("0.20 ");
break;
case ADXL345_DATARATE_0_10_HZ:
Serial.print ("0.10 ");
break;
default:
Serial.print ("???? ");
break;
}
Serial.println(" Hz");
}
void displayRange(void)
{
Serial.print ("Range: +/- ");
switch(accel.getRange())
{
case ADXL345_RANGE_16_G:
Serial.print ("16 ");
break;
case ADXL345_RANGE_8_G:
Serial.print ("8 ");
break;
case ADXL345_RANGE_4_G:
Serial.print ("4 ");
break;
case ADXL345_RANGE_2_G:
Serial.print ("2 ");
break;
default:
Serial.print ("?? ");
break;
}
Serial.println(" g");
}
void setup(void)
{
//#ifndef ESP8266
// while (!Serial); // for Leonardo/Micro/Zero
//#endif
Serial.begin(9600);
Serial.println("Accelerometer Test"); Serial.println("");
/* Initialise the sensor */
if(!accel.begin())
{
/* There was a problem detecting the ADXL345 ... check your connections */
Serial.println("Ooops, no ADXL345 detected ... Check your wiring!");
while(1);
}
/* Set the range to whatever is appropriate for your project */
accel.setRange(ADXL345_RANGE_16_G);
// accel.setRange(ADXL345_RANGE_8_G);
// accel.setRange(ADXL345_RANGE_4_G);
// accel.setRange(ADXL345_RANGE_2_G);
/* Display some basic information on this sensor */
displaySensorDetails();
/* Display additional settings (outside the scope of sensor_t) */
displayDataRate();
displayRange();
Serial.println("");
}
void loop(void)
{
/* Get a new sensor event */
sensors_event_t event;
accel.getEvent(&event);
/* Display the results (acceleration is measured in m/s^2) */
Serial.print("X: "); Serial.print(event.acceleration.x); Serial.print(" ");
Serial.print("Y: "); Serial.print(event.acceleration.y); Serial.print(" ");
Serial.print("Z: "); Serial.print(event.acceleration.z); Serial.print(" ");Serial.println("m/s^2 ");
delay(500);
}
- Now upload the code on NodeMCU board.
- After uploading the code open the serial monitor and set the baud rate to 9600 to see the output.
Output on the serial monitor
.png)
Let’s understand the code
First we have to initialize the libraries.
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
Now we assign a unique ID to the sensor at the same time
Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345);
The displaySensorDetails(void)
is the function that gives the details about the sensor such as version, Unique ID, Max, Min value, etc.
displaySensorDetails(void)
The displayDataRate(void)
function returns the current data of the sensor.
Also, we can select the data rate from slowest to fastest which is 10Hz to 3200Hz. We can also select it as per the datasheet.
displayDataRate(void)
We can also change the data rate with the setDataRate(datarate)
function.
setRange(ADXL345_RANGE_16_G);
You can set the following data rate:
ADXL345_DATARATE_3200_HZ
ADXL345_DATARATE_1600_HZ
ADXL345_DATARATE_800_HZ
ADXL345_DATARATE_400_HZ
ADXL345_DATARATE_200_HZ
ADXL345_DATARATE_100_HZ
ADXL345_DATARATE_50_HZ
ADXL345_DATARATE_25_HZ
ADXL345_DATARATE_12_5_HZ
ADXL345_DATARATE_6_25HZ
ADXL345_DATARATE_3_13_HZ
ADXL345_DATARATE_1_56_HZ
ADXL345_DATARATE_0_78_HZ
ADXL345_DATARATE_0_39_HZ
ADXL345_DATARATE_0_20_HZ
ADXL345_DATARATE_0_10_HZ
This function is used to print the current range of the ADXL345 on the serial monitor. If we select ±8g
, then the accelerometer can detect acceleration up to ±78.4 m/s2 (8 * 9.8). The default range is ±16g.
displayRange(void)
In setup function,
We have initiated the serial communication with a 9600 Baud rate.
Serial.begin(9600);
Now we will begin with the I2C communication, detect the sensor and initialize it with the accel.begin()
function.
if(!accel.begin())
{
/* There was a problem detecting the ADXL345 ... check your connections */
Serial.println("Ooops, no ADXL345 detected ... Check your wiring!");
while(1);
}
We can set the range the of the sensor using accel.setRange(range);
function
accel.setRange(ADXL345_RANGE_16_G);
// accel.setRange(ADXL345_RANGE_8_G);
// accel.setRange(ADXL345_RANGE_4_G);
// accel.setRange(ADXL345_RANGE_2_G);
Printing the information about the sensor on the serial monitor.
displaySensorDetails();
Print the data rate of ADXL345 sensor on the serial monitor.
displayDataRate();
Print the current range of the ADXL345 on the serial monitor
displayRange();
In loop function
In the loop function first, we will read the acceleration and display them on serial monitor.
First, create an event object of sensors_event_t
sensors_event_t
is a user-defined datatype (Structures in C) that contains data from the ADXL345 sensor from a specific moment in time.
Then print the measured X, Y, and Z acceleration in m/s^2 on the serial monitor.
void loop(void)
{
/* Get a new sensor event */
sensors_event_t event;
accel.getEvent(&event);
/* Display the results (acceleration is measured in m/s^2) */
Serial.print("X: "); Serial.print(event.acceleration.x); Serial.print(" ");
Serial.print("Y: "); Serial.print(event.acceleration.y); Serial.print(" ");
Serial.print("Z: "); Serial.print(event.acceleration.z); Serial.print(" ");
Serialprintln("m/s^2 ");
delay(500);
}
Accelerometer ADXL345 Web Server using NodeMCU
Now let’s display the same readings on the web server using the Arduino IDE.
Here we have modified the above example to display the result on the web server.
Before uploading the code make sure you have added your SSID, and Password as follows.
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
ADXL345 Accelerometer Web Server Code for NodeMCU
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
#include "html.h"
ESP8266WebServer server(80);
const char* ssid = "*Your SSID*"; /*Enter Your SSID*/
const char* password = "*Your Password*"; /*Enter Your Password*/
long X, Y, Z;
/* Assign a unique ID to this sensor at the same time */
Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345);
void displaySensorDetails(void)
{
sensor_t sensor;
accel.getSensor(&sensor);
Serial.println("------------------------------------");
Serial.print ("Sensor: "); Serial.println(sensor.name);
Serial.print ("Driver Ver: "); Serial.println(sensor.version);
Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id);
Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" m/s^2");
Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" m/s^2");
Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" m/s^2");
Serial.println("------------------------------------");
Serial.println("");
delay(500);
}
void displayDataRate(void)
{
Serial.print ("Data Rate: ");
switch(accel.getDataRate())
{
case ADXL345_DATARATE_3200_HZ:
Serial.print ("3200 ");
break;
case ADXL345_DATARATE_1600_HZ:
Serial.print ("1600 ");
break;
case ADXL345_DATARATE_800_HZ:
Serial.print ("800 ");
break;
case ADXL345_DATARATE_400_HZ:
Serial.print ("400 ");
break;
case ADXL345_DATARATE_200_HZ:
Serial.print ("200 ");
break;
case ADXL345_DATARATE_100_HZ:
Serial.print ("100 ");
break;
case ADXL345_DATARATE_50_HZ:
Serial.print ("50 ");
break;
case ADXL345_DATARATE_25_HZ:
Serial.print ("25 ");
break;
case ADXL345_DATARATE_12_5_HZ:
Serial.print ("12.5 ");
break;
case ADXL345_DATARATE_6_25HZ:
Serial.print ("6.25 ");
break;
case ADXL345_DATARATE_3_13_HZ:
Serial.print ("3.13 ");
break;
case ADXL345_DATARATE_1_56_HZ:
Serial.print ("1.56 ");
break;
case ADXL345_DATARATE_0_78_HZ:
Serial.print ("0.78 ");
break;
case ADXL345_DATARATE_0_39_HZ:
Serial.print ("0.39 ");
break;
case ADXL345_DATARATE_0_20_HZ:
Serial.print ("0.20 ");
break;
case ADXL345_DATARATE_0_10_HZ:
Serial.print ("0.10 ");
break;
default:
Serial.print ("???? ");
break;
}
Serial.println(" Hz");
}
void displayRange(void)
{
Serial.print ("Range: +/- ");
switch(accel.getRange())
{
case ADXL345_RANGE_16_G:
Serial.print ("16 ");
break;
case ADXL345_RANGE_8_G:
Serial.print ("8 ");
break;
case ADXL345_RANGE_4_G:
Serial.print ("4 ");
break;
case ADXL345_RANGE_2_G:
Serial.print ("2 ");
break;
default:
Serial.print ("?? ");
break;
}
Serial.println(" g");
}
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send the code to the web server*/
}
void ADXL345html() {
String data = "[\""+String(X)+"\",\""+String(Y)+"\",\""+String(Z)+"\"]";
server.send(200, "text/plane", data);
}
void setup(void){
// #ifndef ESP8266
// while (!Serial); // for Leonardo/Micro/Zero
//#endif
Serial.begin(115200); /*Set the baudrate to 115200*/
Serial.println("Accelerometer Test"); Serial.println("");
/* Initialise the sensor */
if(!accel.begin())
{
/* There was a problem detecting the ADXL345 ... check your connections */
Serial.println("Ooops, no ADXL345 detected ... Check your wiring!");
while(1);
}
/* Set the range to whatever is appropriate for your project */
accel.setRange(ADXL345_RANGE_16_G);
// accel.setRange(ADXL345_RANGE_8_G);
// accel.setRange(ADXL345_RANGE_4_G);
// accel.setRange(ADXL345_RANGE_2_G);
/* Display some basic information on this sensor */
displaySensorDetails();
/* Display additional settings (outside the scope of sensor_t) */
displayDataRate();
displayRange();
Serial.println("");
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED){Serial.print(".");} /*Wait while connecting to WiFi*/
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
server.on("/", MainPage); /*Display the Web/HTML Page*/
server.on("/readADXL345", ADXL345html); /*Display the updated Distance value(CM and INCH)*/
server.begin(); /*Start Server*/
delay(1000); /*Wait for 1000mS*/
}
void loop(void){
/* Get a new sensor event */
sensors_event_t event;
accel.getEvent(&event);
X = event.acceleration.x;
Y = event.acceleration.y;
Z = event.acceleration.z;
/* Display the results (acceleration is measured in m/s^2) */
Serial.print("X: "); Serial.print(X); Serial.print(" ");
Serial.print("Y: "); Serial.print(Y); Serial.print(" ");
Serial.print("Z: "); Serial.print(Z); Serial.print(" ");Serial.println("m/s^2 ");
server.handleClient();
/*wait a second*/
delay(1000);
}
- Now upload the code in NodeMCU.
- Once you upload the code then open the serial monitor on IDE and set the baud rate to 115200. I necessary reset the NodeMCU and check the IP address as shown below:
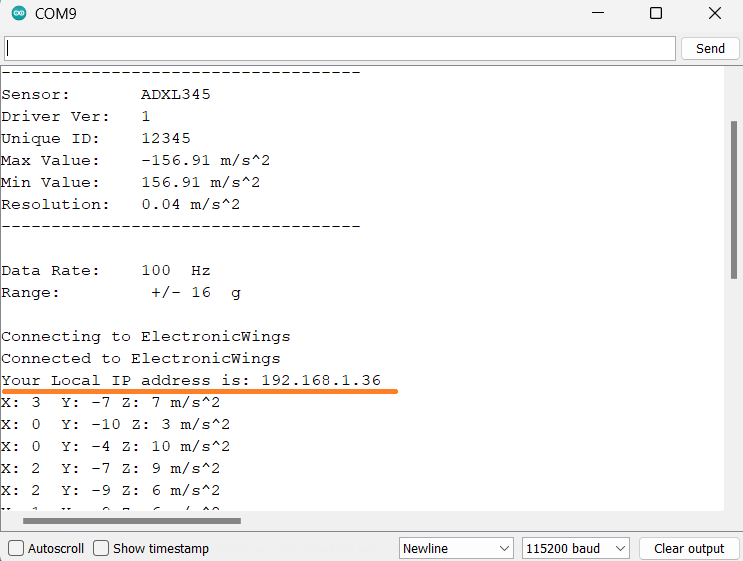
Output on the webserver:
- Open any internet browser on your mobile and type the IP address seen on the serial monitor and press enter button.
- If everything goes alright , then you see a web page showing the x y and z acceleration values on the web server like the below image
Note: make sure that both the devices NodeMCU and mobile are connected to the same wifi router/server, if they are connected to the same router then you will able to see the web page.
.jpg)
Let’s Understand the code.
This code starts with important header files and libraries. All NodeMCU(esp8266) Wi-Fi related definitions and functions are stored in the ESP8266WiFi.h
header file.
The ESP8266WebServer.h
header file has all the definitions that supports handling the HTTP GET and POST requests as well as setting up a local host server.
In the html.h
file contains all the web page code that is in html language.
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
#include "html.h"
Let’s define HTTP port i.e., Port 80 as follows
ESP8266WebServer server(80);
Define the variables for three axis
long X, Y, Z;
Setup Function:
In the setup function, we set the wifi of NodeMcu in STA mode and connect it the provided SSID and password
WiFi.mode(WIFI_STA); /*Set the WiFi in STA Mode*/
WiFi.begin(ssid, password);
Serial.print("Connecting to ");
Serial.println(ssid);
delay(1000); /*Wait for 1000mS*/
while(WiFi.waitForConnectResult() != WL_CONNECTED)
{
Serial.print(".");
}
After successfully connecting to the server we will print the IP address on the serial monitor of IDE.
Serial.print("Your Local IP address is: ");
Serial.println(WiFi.localIP()); /*Print the Local IP*/
Handling Client Requests and Serving the Page:
Server.on()
is the function used for handling the client request.
The function has two parameters to be passed that is the URL path and the function name that we want to execute.
So, when a client requests is root (/)
path, the function MainPage()
gets executed.
At the same time the client request "/readADXL345"
path , the function ADXL345html()
is executed.
server.on("/", MainPage); /*Client request handling:calls the function to serve HTML age */
server.on("/readADXL345", ADXL345html); /*Display the updated Distance value(CM and INCH)*/
Now start the server using server.begin()
function.
server.begin(); /*Start Server*/
Functions for Serving HTML
In the html.h
header file we have defined all the HTML page and added it to the header file list. Using server.send()
function, we send the complete html page to the client request.
In the server.send()
function we have passed three parameters. First is “200” which the status response code as OK (Standard response for successful HTTP request)
Second parameter is “text/html”
, which is the content type we are sending to the client and third is the “_html_page “
which is the html page code.
void MainPage() {
String _html_page = html_page; /*Read The HTML Page*/
server.send(200, "text/html", _html_page); /*Send HTM page to Client*/
}
In the ADXL345html()
function, we send the updated acceleration values to our web page.
void ADXL345html() {
String data = "[\""+String(X)+"\",\""+String(Y)+"\",\""+String(Z)+"\"]";
server.send(200, "text/plane", data);
}
Loop Function
Now we used handleClient()
function for handling the incoming client request and serve the HTML web page.
So, it continuously serves the client requests.
server.handleClient();
HTML Page Code
/*
NodeMCU HTML WebServer Page Code
http:://www.electronicwings.com
*/
const char html_page[] PROGMEM = R"RawString(
<!DOCTYPE html>
<html>
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
<body>
<h1>ADXL345 Interface with NodeMCU</h1><br>
<p>X : <span id="_X">0</span> m/s²</p>
<p>Y : <span id="_Y">0</span> m/s²</p>
<p>Z : <span id="_Z">0</span> m/s²</p>
<script>
setInterval(function() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
const text = this.responseText;
const myArr = JSON.parse(text);
document.getElementById("_X").innerHTML = myArr[0];
document.getElementById("_Y").innerHTML = myArr[1];
document.getElementById("_Z").innerHTML = myArr[2];
}
};
xhttp.open("GET", "readADXL345", true);
xhttp.send();
},100);
</script>
</body>
</html>
)RawString";
Let’s understand the code step by step.
<!DOCTYPE html>
is the standard declaration of all the html pages. It is just to inform the browser which type of document is expected.
<!DOCTYPE html>
The html tag is the container of the complete html page which represents on the top of the html code.
<html>
Now here we are defining the style information for a web page using the <style>
tag. Inside the style tag we have defined the font name, size, color, and test alignment.
<style>
body {font-family: sans-serif;}
h1 {text-align: center; font-size: 30px;}
p {text-align: center; color: #4CAF50; font-size: 40px;}
</style>
We define the document body inside the body tag. We have used headings and paragraphs if you need any other things such as images, tables, lists, etc you can add them.
We are displaying the heading of the page and the three readings of accelerations i.e. x,y,z on the web page.
Now accelerations value updates under the span id which is manipulated with JavaScript using the id attribute.
<body>
<h1>ADXL345 Interface with NodeMCU </h1><br>
<p>X : <span id="_X">0</span> m/s²</p>
<p>Y : <span id="_Y">0</span> m/s²</p>
<p>Z : <span id="_Z">0</span> m/s²</p>
This is the javascript code that comes under the <script>
tag, this is called as client-side script.
<script>
In setInterval()
method a delay of 50ms is generated and then the function is called.
setInterval(function() {},50);
here we are creating the html XMLHttpRequest
object
var xhttp = new XMLHttpRequest();
The ready state holds the status of XMLHttpRequest
. The event xhttp.onreadstatechange
is triggered every time the ready state changes.
The ready state in the code below is 4 that is the request is finished and the status is 200 which means OK.
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
const myArr = JSON.parse(this.responseText);
now here is the main thing, we are updating the acceleration values in html page using _X, _Y, and _Z id.
document.getElementById("_X").innerHTML = myArr[0];
document.getElementById("_Y").innerHTML = myArr[1];
document.getElementById("_Z").innerHTML = myArr[2];
here we used the AJAX method to send the updated values to the server without refreshing the page.
In the below function, we have used the GET method and sent the readADXL345
function which we defined in the main code asynchronously.
xhttp.open("GET", "readADXL345", true);
Send the request to the server using xhttp.send();function.
xhttp.send();
Close the script
</script>
Close the body
</body>
Close the html.
</html>
Components Used |
||
---|---|---|
ADXL345 Digital Accelerometer ADXL345 Digital Accelerometer ADXL345 Digital Accelerometer |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 |