Overview of RTC
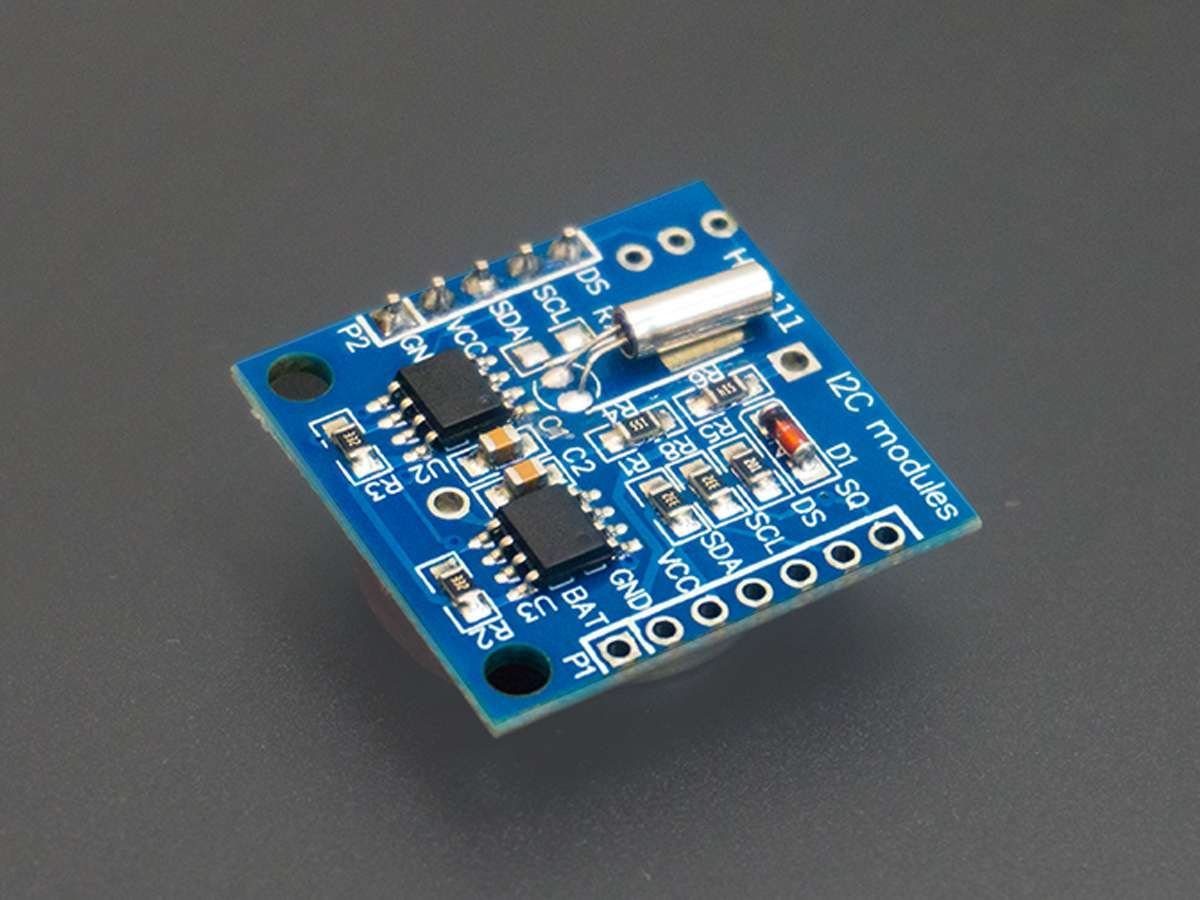
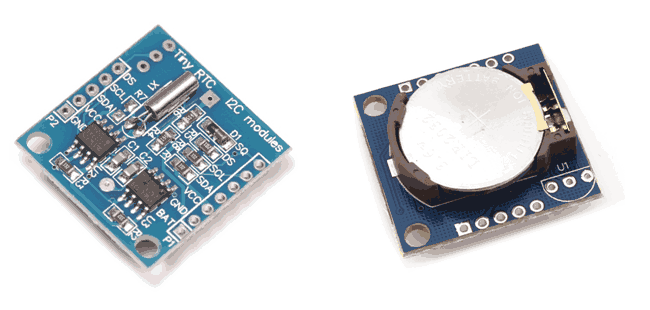
- Real Time Clock (RTC) is used for monitoring time and maintaining a calendar.
- In order to use an RTC, we need to first program it with the current date and time. Once this is done, the RTC registers can be read any time to know the time and date.
- DS1307 is an RTC which works on I2C protocol. Data from various registers can be read by accessing their addresses for reading using I2C communication.
For information on DS1307 and how to use it, refer the topic RTC (Real Time Clock ) DS1307 Module in the sensors and modules section.
Connection Diagram of DS1307 RTC Module with Arduino
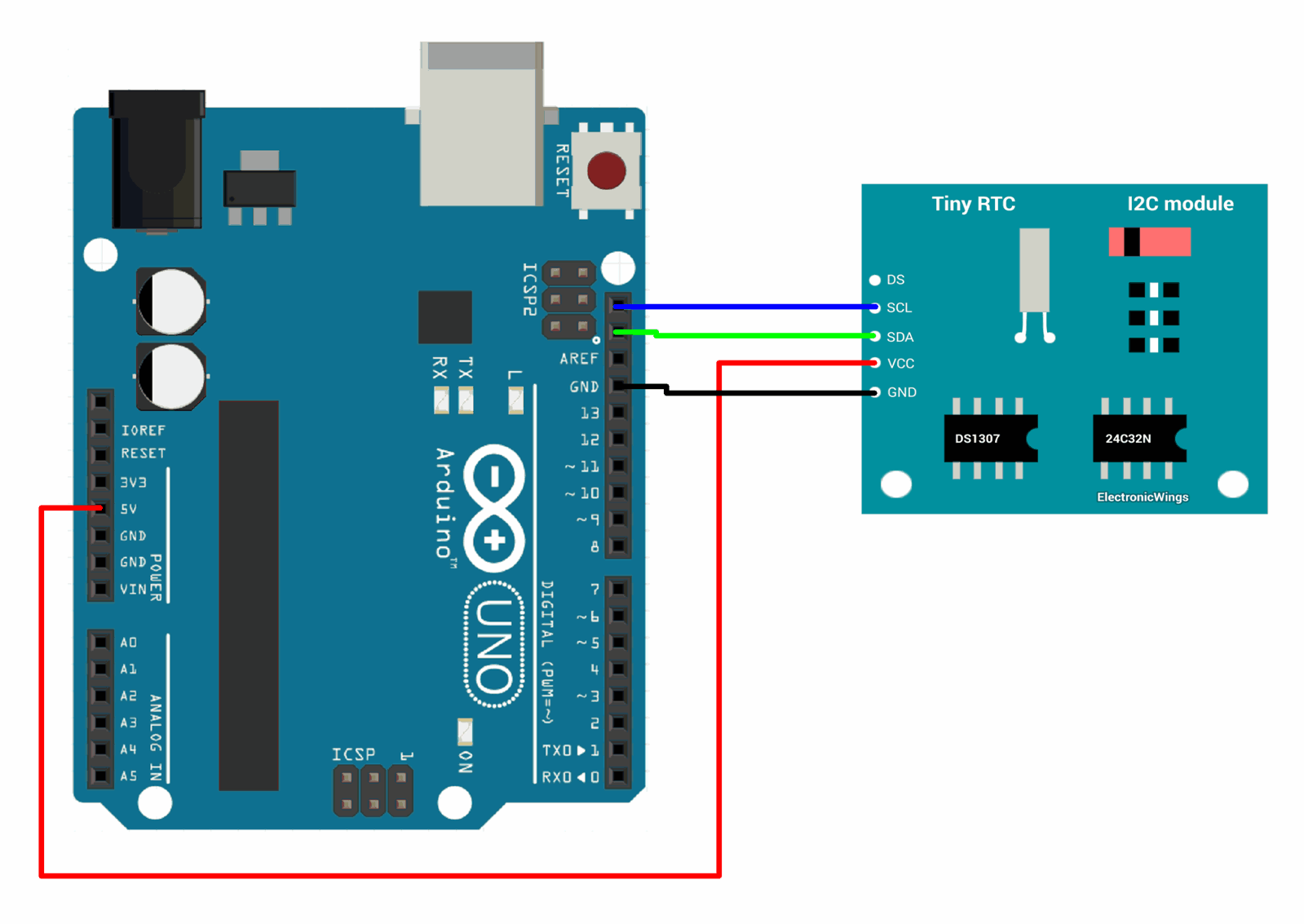
Set Date and Time in DS1307 RTC and Read Using Arduino Uno
Programming Arduino to feed RTC with current date and time, and reading the date and time from the RTC.
Here, we will be using DS1307 library by Watterott from GitHub.
Download this library from here.
Extract the library and add the folder named DS1307 to the libraries folder path of Arduino IDE.
For information about how to add a custom library to the Arduino IDE and use examples from it, refer Adding Library To Arduino IDE in the Basics section.
Once the library has been added to the Arduino IDE, open the IDE and open the example sketch named Example from the DS1307 library added.
Word Of Caution : In the example sketch, in setup loop, rtc.set() function is used. Pass the current date and time arguments as mentioned to this function. In the example sketch, this statement will be commented. Uncomment it and upload the sketch. Once the sketch is uploaded, uncomment the statement again and upload the sketch. If this is not done, each time the Arduino UNO board resets or is powered on after power off, the date and time you set will be set over and over again and you will not be able to read the exact current time and date.
Code for Set and Read Time and Date In DS1307 using Arduino
/*
DS1307 RTC (Real-Time-Clock) Example
Uno A4 (SDA), A5 (SCL)
Mega 20 (SDA), 21 (SCL)
Leonardo 2 (SDA), 3 (SCL)
*/
#include <Wire.h>
#include <DS1307.h>
DS1307 rtc;
void setup()
{
/*init Serial port*/
Serial.begin(9600);
while(!Serial); /*wait for serial port to connect - needed for Leonardo only*/
rtc.begin();
/*init RTC*/
Serial.println("Init RTC...");
/*only set the date+time one time*/
rtc.set(0, 0, 8, 24, 12, 2014); /*08:00:00 24.12.2014 //sec, min, hour, day, month, year*/
/*stop/pause RTC*/
// rtc.stop();
/*start RTC*/
rtc.start();
delay(1000);
}
void loop()
{
uint8_t sec, min, hour, day, month;
uint16_t year;
/*get time from RTC*/
rtc.get(&sec, &min, &hour, &day, &month, &year);
/*serial output*/
Serial.print("\nTime: ");
Serial.print(hour, DEC);
Serial.print(":");
Serial.print(min, DEC);
Serial.print(":");
Serial.print(sec, DEC);
Serial.print("\nDate: ");
Serial.print(day, DEC);
Serial.print(".");
Serial.print(month, DEC);
Serial.print(".");
Serial.print(year, DEC);
/*wait a second*/
delay(1000);
}
Video of RTC DS1307 with Arduino Uno
Functions Used
DS1307 rtc
- This defines an object named rtc of the class DS1307.
rtc.set(uint8_t sec, uint8_t min, uint8_t hour, uint8_t day, uint8_t month, uint16_t year)
- This function is used to set the current time and date into DS1307 RTC IC.
rtc.start()
- This function is used to start I2C communication with DS1307. It fetches the SEC and CH byte from the DS1307 Timekeeper registers.
rtc.get(uint8_t *sec, uint8_t *min, uint8_t *hour, uint8_t *day, uint8_t *month, uint16_t *year)
- This function is used to get the time and date stored in DS1307 IC.
Components Used |
||
---|---|---|
Arduino UNO Arduino UNO |
X 1 | |
Arduino Nano Arduino Nano |
X 1 | |
DS1307 RTC DS1307 RTC DS1307 RTC DS1307 RTC |
X 1 |
Downloads |
||
---|---|---|
|
DS1307 RTC Project File | Download |