Introduction
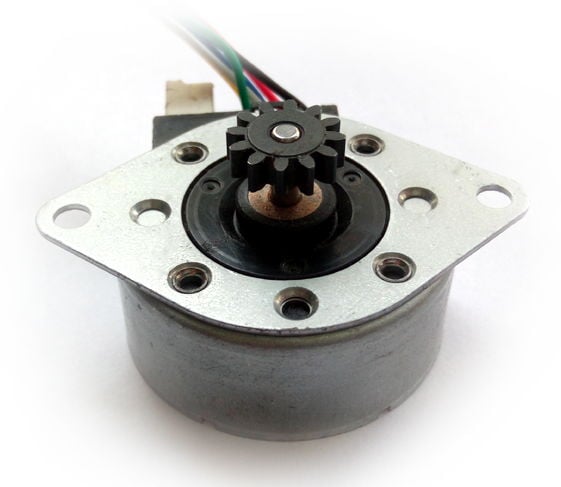
The Stepper motor is a brushless DC motor that divides the full rotation angle of 360° into the number of equal steps.
- The motor is rotated by applying a certain sequence of control signals. The speed of rotation can be changed by changing the rate at which the control signals are applied.
- Various stepper motors with different step angles and torque ratings are available in the market.
- A microcontroller can be used to apply different control signals to the motor to make it rotate according to the need of an application.
For more information about Stepper Motor and how to use it, refer to the topic Stepper Motor in the sensors and modules section.
Interfacing of Stepper Motor with PIC18F4550
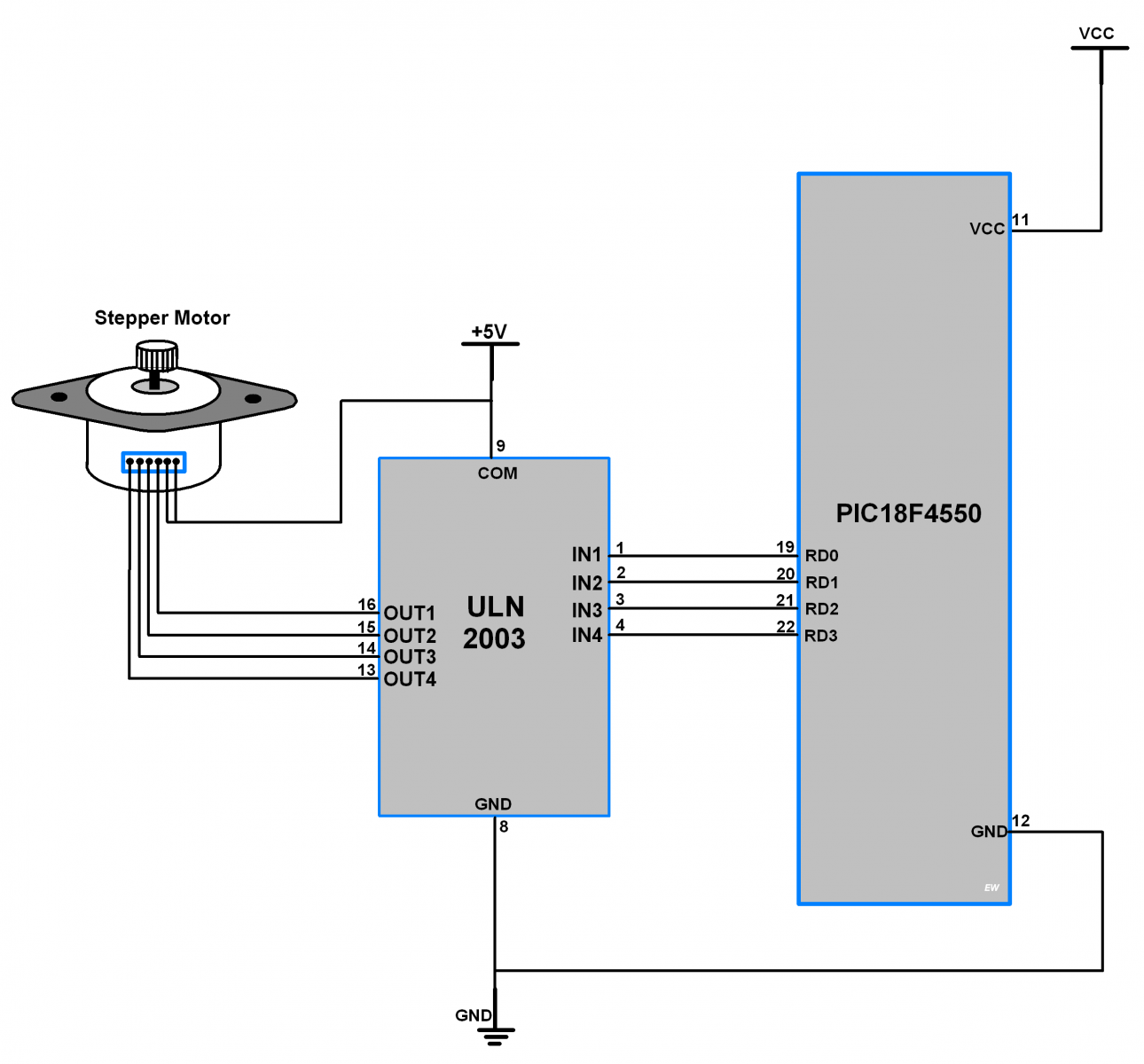
- Here we are going to interface 6 wires Unipolar Stepper Motor with PIC18F4550 controller.
- Only four wires are required to control a stepper motor.
- Two common wires of stepper motor connected to the 5V supply.
- ULN2003 driver is used to driving a stepper motor.
- Note that to know the winding coil and their center tap leads measure resistance in between leads. From center leads, we will get half the resistance value of that winding.
Example
Let’s program PIC18F4550 to rotate the stepper motor 360° clockwise by half step sequence and 360° anticlockwise by full step sequence.
Stepper Motor Control Code for PIC18F4550
/*
* Stepper Motor Control using PIC
* http://www.electronicwings.com
*/
#include <pic18f4550.h>
#include "Configuration_header_file.h"
void MSdelay(unsigned int val)
{
unsigned int i,j;
for(i=0;i<val;i++)
for(j=0;j<165;j++);
}
void main(void)
{
int period;
OSCCON = 0x72; /* Use Internal Oscillator 8MHz */
TRISD = 0x00; /* Make PORTD as output */
period = 100; /* Set period in between two steps */
while (1)
{
/* Rotate Stepper Motor clockwise with Half step sequence */
for(int i=0;i<12;i++)
{
LATD = 0x09;
MSdelay(period);
LATD = 0x08;
MSdelay(period);
LATD = 0x0C;
MSdelay(period);
LATD = 0x04;
MSdelay(period);
LATD = 0x06;
MSdelay(period);
LATD = 0x02;
MSdelay(period);
LATD = 0x03;
MSdelay(period);
LATD = 0x01;
MSdelay(period);
}
LATD = 0x09; /* Last step to initial position */
MSdelay(period);
MSdelay(1000);
/* Rotate Stepper Motor Anticlockwise with Full step sequence */
for(int i=0;i<12;i++)
{
LATD = 0x09;
MSdelay(period);
LATD = 0x03;
MSdelay(period);
LATD = 0x06;
MSdelay(period);
LATD = 0x0C;
MSdelay(period);
}
LATD = 0x09;
MSdelay(period);
MSdelay(1000);
}
}
Video
Components Used |
||
---|---|---|
ULN2003 Motor Driver ULN2003A is a high-voltage, high-current Darlington transistor array. |
X 1 | |
PICKit 4 MPLAB PICKit 4 MPLAB |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 |
Downloads |
||
---|---|---|
|
PIC18F4550 Stepper Motor Interface Project File | Download |