Overview of Analog Joystick
Analog Joystick is used as an input device for changing the position of a cursor in correspondence to the movement of the joystick.
It gives analog voltages in X and Y directions corresponding to the position of the joystick.
These voltages can be processed to find the position of a cursor or for moving the cursor according to the position of the joystick.
For more information about Analog Joystick and how to use it, refer to the topic Analog Joystick in the sensors and modules section.
In order to process the analog signals, we need to use ADC on the microcontroller.
For information about ADC in PIC18F4550 and how to use it, refer to the topic ADC in PIC18F4550 in the PIC inside section.
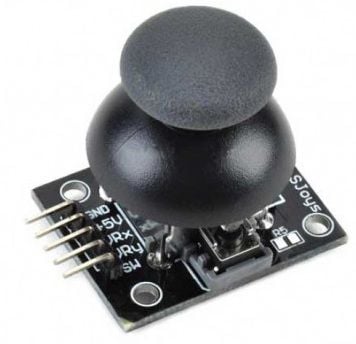
Connection Diagram of Analog Joystick to PIC18F4550
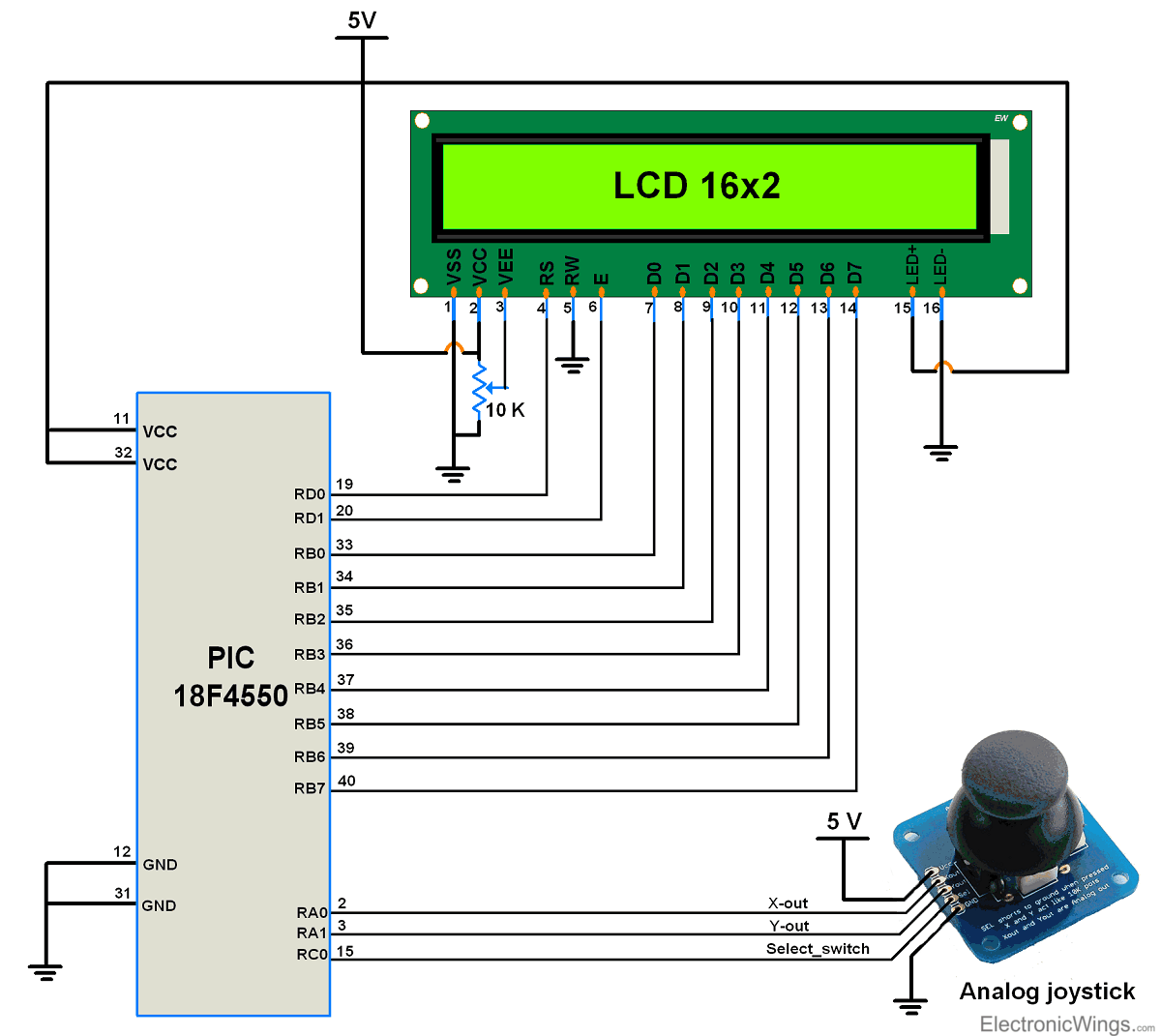
Read Analog Joystick using PIC18F4550
Here we are going to interface Analog Joystick with PIC18F4550. In this, we will display digital value for the XY movement on LCD16x2.
While interfacing the Joystick with PIC18F4550, we have to connect the X and Y output pin of a joystick to the ADC channel of the microcontroller. By taking the ADC value of X and Y pins, we can determine the position of the Joystick.
Also, we show the Joystick movement on PC using Matlab. In Matlab, we read Serial Port which accepts value send by the PIC18F4550 microcontroller serially.
Analog Joystick Code for PIC18F4550
/*
Analog Joystick Interfacing with PIC18f4550
http://www.electronicwings.com
*/
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <p18f4550.h>
#include "Configuration_Header_File.h" /* Header File for Configuration bits */
#include "LCD_16x2_8-bit_Header_File.h" /* Header File for LCD Functions */
#include "PIC18F4550_ADC_Header_File.h" /* Header File for ADC Functions */
#include "USART_Header_File.h" /* Header File for USART Functions */
void main()
{
char data[10],data1[10];
int x,y;
OSCCON=0x72; /*Set internal Oscillator frequency to 8 MHz*/
LCD_Init(); /*Initialize 16x2 LCD*/
ADC_Init(); /*Initialize 10-bit ADC*/
USART_Init(9600);
while(1)
{
x=ADC_Read(0);
sprintf(data,"%d ",x); /* it is used to convert integer value to ASCII string */
LCD_String_xy(0,0,data); /* send string data for printing */
USART_SendString(data);
MSdelay(90);
y=ADC_Read(1);
sprintf(data1,"%d ",y); /* it is used to convert integer value to ASCII string*/
LCD_String_xy(0,6,data1); /* send string data for printing*/
USART_SendString(data1);
MSdelay(90);
USART_TxChar(0x0A);
USART_TxChar(0x0D);
memset(data,0,10);
memset(data1,0,10);
}
}
Video of Reading Analog Joystick using PIC18F4550
Components Used |
||
---|---|---|
Analog Joystick Joystick is an input device used to control the pointer movement in 2-dimension axis. It is mostly used in Video games. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 | |
PICKit 4 MPLAB PICKit 4 MPLAB |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 |
Downloads |
||
---|---|---|
|
PIC18f4550 Joystick with Matlab Project File | Download |