Introduction
The keypad is used as an input device to read the key pressed by the user and to process it.
4x4 keypad consists of 4 rows and 4 columns. Switches are placed between the rows and columns. A keypress establishes a connection between the corresponding row and column between which the switch is placed.
In order to read the keypress, we need to configure the rows as outputs and columns as inputs.
Columns are read after applying signals to the rows in order to determine whether or not a key is pressed and if pressed, which key is pressed.
For more information about the keypad and how to use it, refer to the topic 4x4 Keypad in the sensors and modules section.
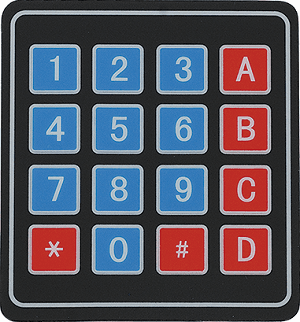
Interfacing Diagram
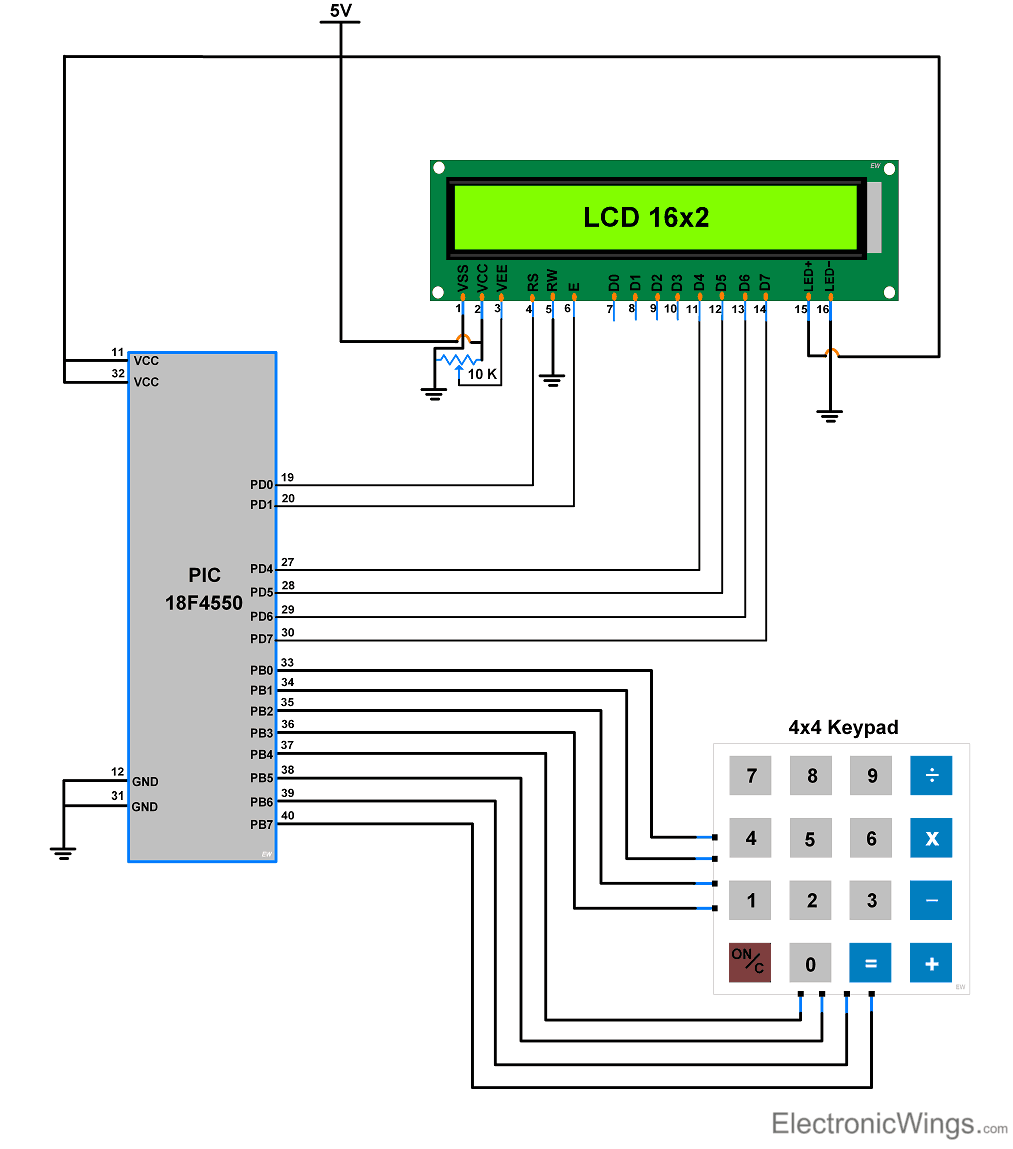
4x4 Matrix Keypad Example using PIC18F4550
Here, we are going to interface the 4x4 keypad with PIC18F4550 and will display the pressed a key on LCD16x2.
LCD16x2 used here in 4-bit mode.
4x4 Keypad Code for PIC18F4550
/*
* Keypad Interfacing with PIC18F4550
* http://www.electronicwings.com
*
*/
#include <pic18f4550.h>
#include "Configuration_Header_File.h"
#include "16x2_LCD_4bit_File.h"
unsigned char keyfind(); /* function to find pressed key */
#define write_port LATB /* latch register to write data on port */
#define read_port PORTB /* PORT register to read data of port */
#define Direction_Port TRISB
unsigned char col_loc,rowloc,temp_col;
unsigned char keypad[4][4]= {'7','8','9','/',
'4','5','6','*',
'1','2','3','-',
' ','0','=','+'};
void main()
{
char key;
OSCCON = 0x72;
RBPU=0; /* activate pull-up resistor */
LCD_Init(); /* initialize LCD16x2 in 4-bit mode */
LCD_String_xy(0,0,"Press a Key");
LCD_Command(0xC0); /* display pressed key on 2nd line of LCD */
while(1)
{
key = keyfind(); /* find a pressed key */
LCD_Char(key); /* display pressed key on LCD16x2 */
}
}
unsigned char keyfind()
{
Direction_Port = 0xf0; /* PORTD.0-PORTD.3 as a Output Port and PORTD.4-PORTD.7 as a Input Port*/
unsigned char temp1;
write_port = 0xf0; /* Make lower nibble as low(Gnd) and Higher nibble as High(Vcc) */
do
{
do
{
col_loc = read_port & 0xf0; /* mask port with f0 and copy it to col_loc variable */
}while(col_loc!=0xf0); /* Check initially at the start there is any key pressed*/
col_loc = read_port & 0xf0; /* mask port with f0 and copy it to col_loc variable */
}while(col_loc!=0xf0);
MSdelay(50);
write_port = 0xf0; /* Make lower nibble as low(Gnd) and Higher nibble as High(Vcc) */
do
{ do
{
col_loc = read_port & 0xf0;
}while(col_loc==0xf0); /* Wait for key press */
col_loc = read_port & 0xf0;
}while(col_loc==0xf0); /* Wait for key press */
MSdelay(20);
col_loc = read_port & 0xf0;
while(1)
{
write_port = 0xfe; /* make Row0(D0) Gnd and keep other row(D1-D3) high */
col_loc = read_port & 0xf0; /* Read Status of PORT for finding Row */
temp_col=col_loc;
if(col_loc!=0xf0)
{
rowloc=0; /* If condition satisfied get Row no. of key pressed */
while(temp_col!=0xf0) /* Monitor the status of Port and Wait for key to release */
{
temp_col = read_port & 0xf0; /* Read Status of PORT for checking key release or not */
}
break;
}
write_port = 0xfd; /* make Row1(D1) Gnd and keep other row(D0-D2-D3) high */
col_loc = read_port & 0xf0; /* Read Status of PORT for finding Row */
temp_col=col_loc;
if(col_loc!=0xf0)
{
rowloc=1; /* If condition satisfied get Row no. of key pressed*/
while(temp_col!=0xf0) /* Monitor the status of Port and Wait for key to release */
{
temp_col = read_port & 0xf0; /* Read Status of PORT for checking key release or not */
}
break;
}
write_port = 0xfb; /* make Row0(D2) Gnd and keep other row(D0-D1-D3) high */
col_loc = read_port & 0xf0; /* Read Status of PORT for finding Row*/
temp_col=col_loc;
if(col_loc!=0xf0)
{
rowloc=2; /* If condition satisfied get Row no. of key pressed */
while(temp_col!=0xf0) /* Wait for key to release */
{
temp_col = read_port & 0xf0; /* Read Status of PORT for checking key release or not */
}
break;
}
write_port = 0xf7; /* make Row0(D3) Gnd and keep other row(D0-D2) high */
col_loc = read_port & 0xf0; /* Read Status of PORT for finding Row */
temp_col=col_loc;
if(col_loc!=0xf0)
{
rowloc=3; /* If condition satisfied get Row no. of key pressed */
while(temp_col!=0xf0) /* Wait for key to release */
{
temp_col = read_port & 0xf0; /* Read Status of PORT for checking key release or not */
}
break;
}
}
while(1)
{
if(col_loc==0xe0)
{
return keypad[rowloc][0]; /* Return key pressed value to calling function */
}
else
if(col_loc==0xd0)
{
return keypad[rowloc][1]; /* Return key pressed value to calling function */
}
else
if(col_loc==0xb0)
{
return keypad[rowloc][2]; /* Return key pressed value to calling function */
}
else
{
return keypad[rowloc][3]; /* Return key pressed value to calling function */
}
}
MSdelay(300);
}
Components Used |
||
---|---|---|
4x4 Keypad Module Keypad is an input device which is generally used in applications such as calculator, ATM machines, computer etc. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 | |
PICKit 4 MPLAB PICKit 4 MPLAB |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 |
Downloads |
||
---|---|---|
|
Keypad interfacing with PIC18F4550 Project File | Download |