Introduction to Watchdog
- Watchdog Timer (WDT) can be helpful to automatically reset the system whenever a timeout occurs.
- A system reset is required for preventing the failure of the system in a situation of a hardware fault or program error.
- There are countless applications where the system cannot afford to get stuck at a point (not even for a small duration of time). For example, in a radar system, if the system hangs for 5 minutes, it can result in serious repercussions (an enemy plane or missile may go undetected resulting in huge losses).
- The system should be robust enough to automatically detect the failures quickly and reset itself in order to recover from the failures and function normally without errors.
- One can manually reset the system to recover from errors. But it is not always feasible to manually reset the system, especially once it has been deployed.
- To overcome such problems, a watchdog timer is necessary to automatically reset the system without human intervention.
How does Watchdog Timer work?
- The watchdog timer is loaded with a timeout period which is dependent on the application.
- The watchdog timer starts its counting independent of the system clock i.e. it has a separate internal oscillator to work independently of a system clock.
- The watchdog timer cleared through software each time before the timeout period occurs.
- Whenever software failed to clear the watchdog timer before its timeout period, then watchdog timer resets the system.
- For this purpose, the watchdog timer is used to overcome software failures in real-time applications.
- The watchdog timer is also used to wake up the microcontroller from sleep mode.
To enable, disable, and check the status of the watchdog timer following registers are used.
MCU Control and Status Register (MCUCSR):
7 | 6 | 5 | 4 | 3 | 2 | 1 | 0 |
JTD | ISC2 | - | JTRF | WDRF | BORF | EXTRF | PORF |
WDRF: Watchdog Reset Flag
- This bit is used to check the status of the watchdog timer.
- WDRF is set if a watchdog reset occurs.
Watchdog Timer Control Register (WDTCR):
7 | 6 | 5 | 4 | 3 | 2 | 1 | 0 |
- | - | - | WDTOE | WDE | WDP2 | WDP1 | WDP0 |
WDTOE: (Watchdog Turn-off Enable)
- This bit is used to disable the watchdog timer, this bit sets only when the WDE bit is written to logic 0. Otherwise, the watchdog timer is not disabled.
WDE: (Watchdog Enable)
- This bit is used to enable the watchdog timer. Watchdog timer enables when this bit is logic 1 and to disable set to logic 0.
1: Enable the watchdog timer
0: Disable the watchdog timer
To disable the watchdog timer –
- First, write logic 1 to WDTOE and WDE.
- Within the next four clock cycles, write a logic 0 to WDE. This disables the
Watchdog timer.
WDP2, WDP1 & WDP0
- WDP2, WDP1 & WDP0 bits represent the watchdog timer pre-scaling, the different pre-scaling timeout period is shown in the below table.
WDP2 | WDP1 | WDP0 | Typical Time-out at VCC = 5.0V |
---|---|---|---|
0 | 0 | 0 | 16.3ms |
0 | 0 | 1 | 32.5ms |
0 | 1 | 0 | 65ms |
0 | 1 | 1 | 0.13s |
1 | 0 | 0 | 0.26s |
1 | 0 | 1 | 0.52s |
1 | 1 | 0 | 1.0s |
1 | 1 | 1 | 2.1s |
Example
- Here, we are going to design a simple application that demonstrates the use of watchdog timer in ATmega16/ATmega32 based on AVR.
- In this application, the watchdog timer resets the main program after a 2.1-sec timeout. LED turns ON and OFF after every 2.1-sec due Watchdog timer.
Circuit diagram
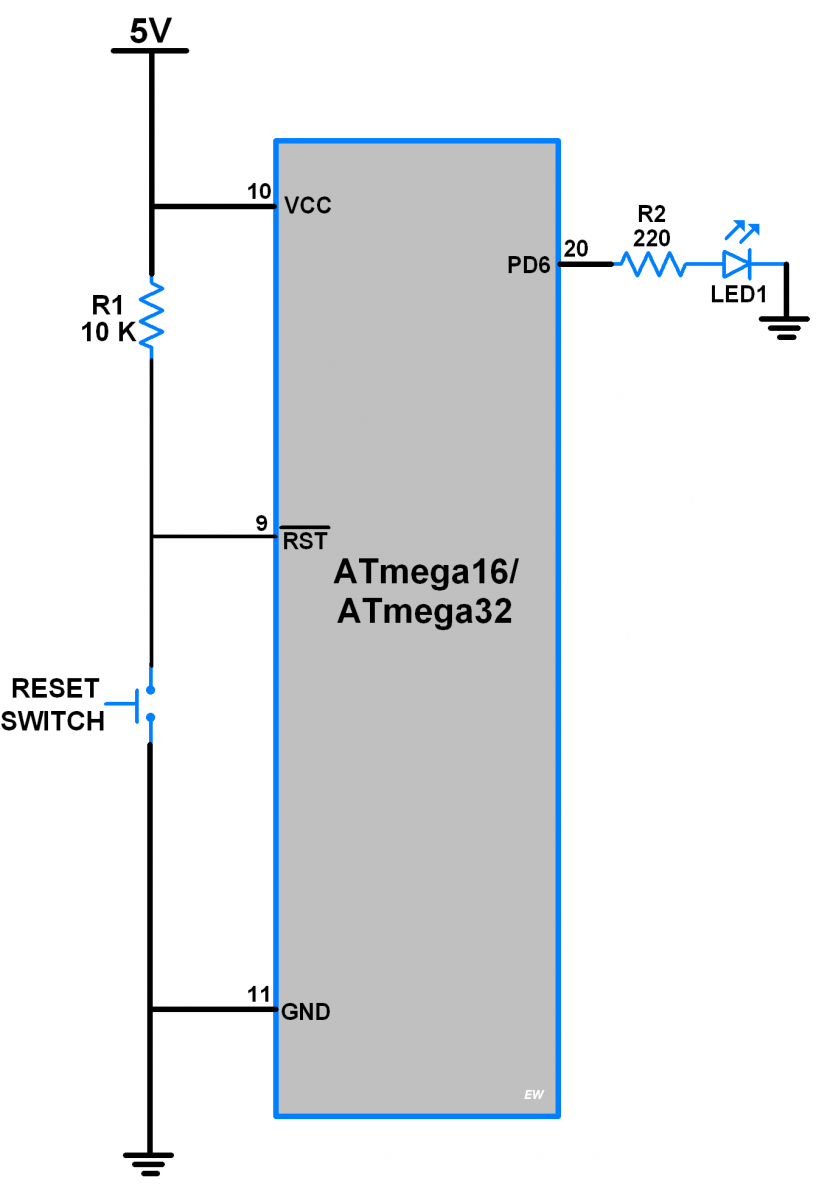
ATmega16/32 Watchdog timer program
/*
* ATmega16_watchdog_timer.c
*
* http://www.electronicwings.com
*/
#define F_CPU 8000000UL
#include <avr/io.h>
#include <util/delay.h>
#define LED_DDR DDRD
#define LED_PORT PORTD
void WDT_ON()
{
/*
Watchdog timer enables with typical timeout period 2.1
second.
*/
WDTCR = (1<<WDE)|(1<<WDP2)|(1<<WDP1)|(1<<WDP0);
}
void WDT_OFF()
{
/*
This function use for disable the watchdog timer.
*/
WDTCR = (1<<WDTOE)|(1<<WDE);
WDTCR = 0x00;
}
int main(void)
{
WDT_ON(); /* Enable the watchdog timer */
LED_DDR |= 0xC0;
LED_PORT |= (1<<6); /* Set PD6 pin to logic high */
_delay_ms(1000); /* Wait for 1 second */
LED_PORT &= ~(1<<6); /* Clear PD6 pin */
while(1);
}
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
LED 5mm LED 5mm |
X 1 |
Downloads |
||
---|---|---|
|
ATmega16 watchdog timer project file | Download |