Overview of XBee S2 Module
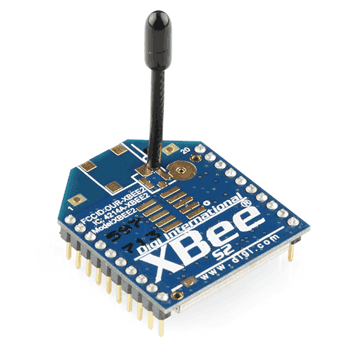
XBee (ZigBee) radios are based on IEEE 802.15.4 (technical standard which defines the operation of low-rate wireless personal area networks (LR-WPANs)) standard and it is designed for point to point, star, etc. communication over the air.
ZigBee is an IEEE 802.15.4 based specification for high-level communication protocols used to create personal area networks with low-power digital radios.
Following are the major features of XBee radio devices,
- They work on 2.4 GHz (Unlicensed Radio Band) radiofrequency.
- Low data rate (≈250Kbps).
- Low power consumption (1mW, 6mW, 250mW etc.).
- Wireless communication over short distances (90m, 750m, 1mile, etc.).
Hence they are used in Home Automation, Wireless Sensor Networks, Industrial Control, Medical Data Collection, Building Automation, etc.
To know more about how Xbee modules work, refer to the topic XBee Module in the sensors and modules section.
Connection Diagram of Xbee S2 with MSP-EXP430G2 TI Launchpad
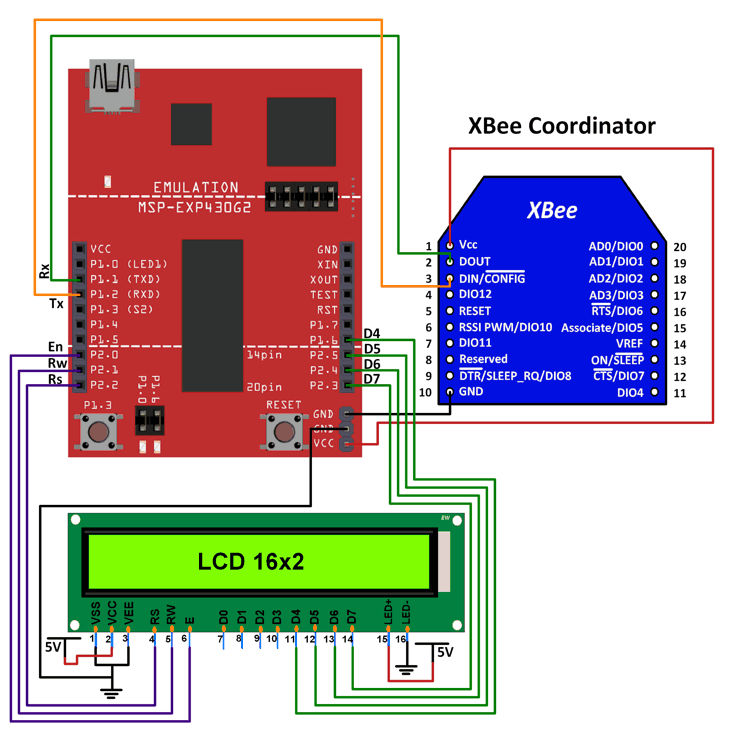
Xbee Connections at Receiver Side
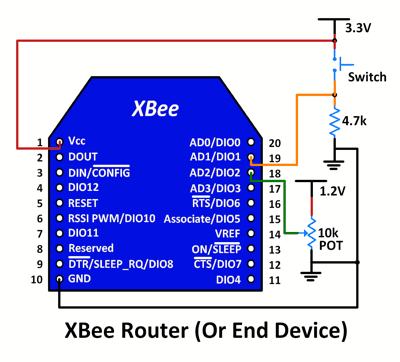
Xbee Communication using MSP-EXP430G2 TI Launchpad
Here, we have connected an XBee S2 to MSP-EXP430G2. This XBee is configured as a Coordinator in API mode with API enable set to 1(you can use API enable set to 2 as well, it will use escape characters along with the data).
Data received by the Coordinator is displayed on a 16x2 LCD which is interfaced with the MSP-EXP430G2.
Another XBee device is configured as a Router in API mode with API enable set to 1(you can use API enable set to 2 as well, it will use escape characters along with the data). Use the same API enable setting for both XBee devices. (You can also configure this device as an End Device in API mode).
A switch is connected to pin DIO1 (pin 19 on the module) of the Router (or End Device) XBee module and the pin is configured as a Digital Input.
A potentiometer is given 1.2V and ground to its fixed terminals and the variable terminal of the potentiometer is connected to pin AD2 (pin 18on the module) of the Router (or End Device) XBee module and the pin is configured as an Analog Input.
IO Sampling (IR) rate can be set according to the requirement of the application, 100 msec for example.
All the configurations and settings are done using X-CTU software provided by Digi International.
If you don’t know how to configure XBee modules using X-CTU, refer to the sub-topic XBee Configuration in the topic XBee Module in the sensors and modules section.
The Router (or End Device) XBee module sends the IO samples of the potentiometer and the switch periodically depending on the IR setting. This data is received by the coordinator and the Sketch uploaded in the MSP-EXP430G2 TI Launchpad parses the data received and extracts the IO samples information from it.
This is displayed on the 16x2 LCD so that working can be verified.
Here, we will be using Andrew Rapp’s XBee library for Arduino from GitHub. Although the library is for Arduino, it works well for MSP-EXP430G2 TI Launchpad as well.
Download this library from here.
Extract the library and add it to the libraries folder path of Energia IDE.
For information about how to add a custom library to the Energia IDE and use examples from it, refer Adding Library To Energia IDE in the Basics section.
Here, we have created a sketch by modifying the example sketch given by the Author for receiving the IO sample for S2 modules. The modifications basically replace a Serial Port with an LCD for debugging since only one Serial port is available and it cannot be used while using Software Serial.
XBee S2 code for MSP-EXP430G2 TI Launchpad
#include <XBee.h>
#include <LiquidCrystal.h>
/* Create object named lcd of the class LiquidCrystal */
LiquidCrystal lcd(10, 9, 8, 14, 13, 12, 11); /* For 4-bit mode */
XBee xbee = XBee(); /* Create an object named xbee(any name of your choice) of the class XBee */
ZBRxIoSampleResponse ioSamples = ZBRxIoSampleResponse();
/* Create an object named ioSamples(any name of your choice) of the class ZBRxIoSampleResponse */
bool lcd_clear = 0;
bool xbee_sample = 1;
void setup() {
Serial.begin(9600); /* Define baud rate for serial communication */
xbee.setSerial(Serial); /* Define serial communication to be used for communication with xbee */
lcd.begin(16,2); /* Initialize 16x2 LCD */
lcd.clear(); /* Clear the LCD */
lcd.setCursor(0,0); /* Set cursor to column 0 row 0 */
lcd.print("XBee Demo"); /* Print data on display */
delay(1000);
lcd.clear();
}
void loop() {
bool analog, digital;
if(lcd_clear)
{
lcd.clear();
lcd_clear = 0;
xbee_sample = 1;
}
if(xbee_sample)
{
lcd.setCursor(0,0);
lcd.print("POT : ");
lcd.setCursor(0,1);
lcd.print("Switch : ");
xbee_sample = 0;
}
xbee.readPacket(); /* Read until a packet is received or an error occurs */
if(xbee.getResponse().isAvailable()) /* True if response has been successfully parsed and is complete */
{
if(xbee.getResponse().getApiId()==ZB_IO_SAMPLE_RESPONSE) /* If response is of IO_Sample_response type */
{
xbee.getResponse().getZBRxIoSampleResponse(ioSamples); /* Get the IO Sample Response */
if (ioSamples.containsAnalog()) { /* If Analog samples present in the response */
analog = 1;
}
else
{
analog = 0;
}
if (ioSamples.containsDigital()) { /* If Digital samples present in the response */
digital = 1;
}
else
{
digital = 0;
}
/* Loop for identifying the analog samples present in the received sample data and to print it */
if(analog)
{
for (int i = 0; i <= 4; i++) { /* Only 4 Analog channels */
if (ioSamples.isAnalogEnabled(i)) { /* Check Analog channel mask to see if the any pin is enabled for analog input sampling */
lcd.setCursor(6,0);
lcd.print(ioSamples.getAnalog(i),DEC);
lcd.print(" ");
}
}
}
else
{
lcd.setCursor(6,0);
lcd.print("No Data");
}
if(digital)
{
for (int i = 0; i <= 12; i++) { /* 12 Digital IO lines */
if (ioSamples.isDigitalEnabled(i)) { /* Check Digital channel mask to see if any pin is enabled for digital IO sampling */
lcd.setCursor(9,1);
lcd.print(ioSamples.isDigitalOn(i), DEC);
lcd.print(" ");
}
}
}
else
{
lcd.setCursor(9,1);
lcd.print("No Data");
}
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Response is : ");
lcd.setCursor(0,1);
lcd.print(xbee.getResponse().getApiId(), HEX); /* Print response received instead of IO_Sample_Response */
lcd_clear = 1;
delay(1000);
}
}
else if (xbee.getResponse().isError()) { /* If error detected, print the error code */
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Error code is : ");
lcd.setCursor(0,1);
lcd.print(xbee.getResponse().getErrorCode(), DEC);
lcd_clear = 1;
delay(1000);
}
}
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
XBee S2 Module XBee is a radio module developed by Digi International. It is popular wireless transceiver used to send or receive data. It is used for low data rate, low power over short distance wireless communication applications such as Home Automation, Wireless sensor n/w, Industrial control, Medical data collection, Building automation etc. |
X 1 |