Overview of Nokia5110 Display
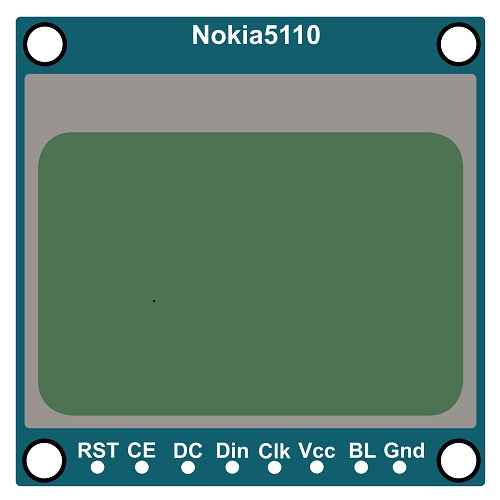
- Nokia 5110 is a graphical display that can display text, images, and various patterns.
- It has a resolution of 48x84 and comes with a backlight.
- It uses SPI communication to communicate with a microcontroller.
- Data and commands can be sent through a microcontroller to the display to control the display output.
- It has 8 pins.
For more information about the Nokia5110 display and how to use it, refer to the topic Nokia5110 Graphical Display in the sensors and modules section.
Connection Diagram of Nokia5110 Module with MSP-EXP430G2 TI Launchpad
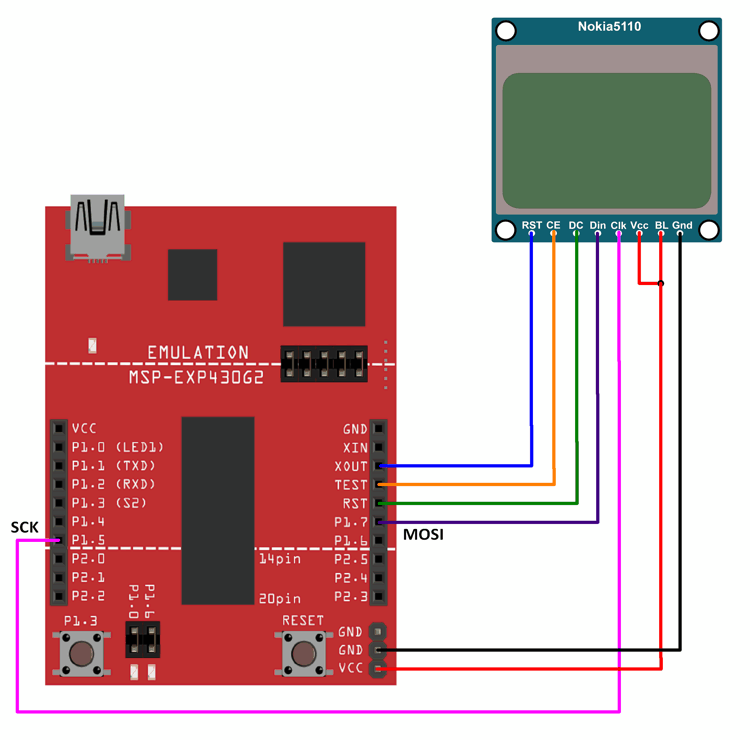
Programming for Nokia5110 Display
Initialization of Nokia5110
- In the initialization of the Nokia5110, the first step is to reset the display by sending low to high pulse to the reset pin.
- Send command 0x21H to set the command in additional mode (H=1).
- Then set VOP = 5V by sending command 0xC0H.
- Set the temperature coefficient to 3 by sending 0x07H.
- Set the voltage bias system using the 0x13 command, it is recommended for n=4 and 1:48 mux rate.
- Send the command 0x20 for basic mode operation (H=0).
- And then send 0x0C for normal mode operation.
void N5110_init(void)
{
N5110_Reset(); /* reset the display */
N5110_Cmnd(0x21); /* command set in addition mode */
N5110_Cmnd(0xC0); /* set the voltage by sending C0 means VOP = 5V */
N5110_Cmnd(0x07); /* set the temp. coefficient to 3 */
N5110_Cmnd(0x13); /* set value of Voltage Bias System */
N5110_Cmnd(0x20); /* command set in basic mode */
N5110_Cmnd(0x0C); /* display result in normal mode */
}
Command write function
- For command, operation makes DC pin low.
- Enable (make low) the slave select pin.
- Send the command on the SPI data register.
- Enable (make high) the DC pin for data operation.
- Disable (make high) the slave select pin.
void N5110_Cmnd(char DATA)
{
digitalWrite(DC, LOW);
digitalWrite(chipSelectPin, LOW);
SPI.transfer(DATA);
digitalWrite(chipSelectPin, HIGH);
digitalWrite(DC, HIGH);
}
Data write function
- For data, operation makes DC pin high.
- Enable(make low) the slave select pin.
- Send the data to the SPI data register.
- Enable (make low) the DC pin for command operation.
- Disable (make high) the slave select pin after sending the data.
void N5110_Data(char *DATA)
{
digitalWrite(DC, HIGH);
digitalWrite(chipSelectPin, LOW);
int len = strlen(DATA);
for (int g=0; g<len; g++)
{
for (int index=0; index<5; index++)
{
SPI.transfer(ASCII[DATA[g] - 0x20][index]);
}
SPI.transfer(0x00);
}
digitalWrite(chipSelectPin, HIGH);
digitalWrite(DC, LOW);
}
Image Display function
void N5110_image(const unsigned char *image_data) /* clear the Display */
{
digitalWrite(DC, HIGH);
digitalWrite(chipSelectPin, LOW);
for (int k=0; k<504; k++)
{
SPI.transfer(image_data[k]);
}
digitalWrite(chipSelectPin, HIGH);
digitalWrite(DC, LOW);
}
Example Code
Displaying text and images on the Nokia5110 display.
Here, we will display simple text on the Nokia5110 display followed by a few images that will be displayed in a loop.
Nokia5110 Display Code for MSP-EXP430G2 TI Launchpad
#include <SPI.h>
#include "Font.h"
#include <string.h>
#include "images.h"
const int chipSelectPin = 17; /* Chip Select Pin */
const int DC = 16; /* Data/Command Pin */
const int RST = 18; /* Reset Pin */
void N5110_Cmnd(char DATA) /* Function for sending command to Nokia5110 display */
{
digitalWrite(DC, LOW); /* DC = 0, display in command mode */
digitalWrite(chipSelectPin, LOW); /* Make chip select pin low to enable SPI commuincation */
SPI.transfer(DATA); /* Send data(command) */
digitalWrite(chipSelectPin, HIGH); /* Make chip select pin high to disable SPI commuincation */
digitalWrite(DC, HIGH); /* DC = 1, display in data mode */
}
void N5110_Data(char *DATA) /* Function for sending data to Nokia5110 display */
{
digitalWrite(DC, HIGH); /* DC = 1, display in data mode */
digitalWrite(chipSelectPin, LOW); /* Make chip select pin low to enable SPI commuincation */
int len = strlen(DATA);
for (int g=0; g<len; g++)
{
for (int index=0; index<5; index++)
{
SPI.transfer(ASCII[DATA[g] - 0x20][index]); /* Send data */
}
SPI.transfer(0x00);
}
digitalWrite(chipSelectPin, HIGH); /* Make chip select pin high to disable SPI commuincation */
digitalWrite(DC, LOW); /* DC = 0, display in command mode */
}
void N5110_Reset(void) /* Function for resetting Nokia5110 display */
{
digitalWrite(RST, LOW); /* Make reset pin low for resetting the Nokia5110 display */
delay(100); /* Reset pin needs to be low for at least 100nsec, we are keeping it low for 100msec */
digitalWrite(RST, HIGH); /* Make reset pin high to bring Nokia5110 display out of reset */
}
void N5110_init(void) /* Function for initializing Nokia5110 display */
{
N5110_Reset(); /* reset the display */
N5110_Cmnd(0x21); /* command set in addition mode */
N5110_Cmnd(0xC0); /* set the voltage by sending C0 means VOP = 5V */
N5110_Cmnd(0x07); /* set the temp. coefficient to 3 */
N5110_Cmnd(0x13); /* set value of Voltage Bias System */
N5110_Cmnd(0x20); /* command set in basic mode */
N5110_Cmnd(0x0C); /* display result in normal mode */
}
void lcd_setXY(char x, char y) /* Function for setting cursor to (x,y) co-ordinates */
{
N5110_Cmnd(x); /* x co-ordinate can be 0x80-0xD3 for position 0-83 */
N5110_Cmnd(y); /* y co-ordinate can be 0x40-0x45 for position 0-5 */
}
void N5110_clear(void) /* Function for clearing Nokia5110 display */
{
digitalWrite(DC, HIGH); /* DC = 1, display in data mode */
digitalWrite(chipSelectPin, LOW); /* Make chip select pin low to enable SPI commuincation */
for (int k=0; k<=503; k++)
{
SPI.transfer(0x00); /* Send data 0x00 to clear the Nokia5110 display */
}
digitalWrite(chipSelectPin, HIGH); /* Make chip select pin high to disable SPI commuincation */
digitalWrite(DC, LOW); /* DC = 0, display in command mode */
}
void N5110_image(const unsigned char *image_data) /* Function for sending image data to Nokia5110 display */
{
digitalWrite(DC, HIGH); /* DC = 1, display in data mode */
digitalWrite(chipSelectPin, LOW); /* Make chip select pin low to enable SPI commuincation */
for (int k=0; k<504; k++)
{
SPI.transfer(image_data[k]); /* Send image data to be displayed */
}
digitalWrite(chipSelectPin, HIGH); /* Make chip select pin high to disable SPI commuincation */
digitalWrite(DC, LOW); /* DC = 0, display in command mode */
}
void setup() {
SPI.begin(); /* Initialize the SPI bus */
pinMode(chipSelectPin, OUTPUT);
pinMode(DC, OUTPUT);
pinMode(RST, OUTPUT);
delay(1000);
N5110_init(); /* Initialize the Nokia5110 display */
delay(100);
N5110_clear(); /* Clear the Nokia5110 display */
delay(1000);
lcd_setXY(0x80,0x40);
N5110_image(Launchpad_Logo);
delay(3000);
N5110_clear();
lcd_setXY(0x9B,0x42);
N5110_Data("Smiley");
lcd_setXY(0xA0,0x43);
N5110_Data("Demo");
delay(3000);
lcd_setXY(0x80,0x40);
}
void loop() {
N5110_clear();
N5110_image(Smiley_1);
delay(300);
N5110_clear();
N5110_image(Smiley_2);
delay(300);
N5110_clear();
N5110_image(Smiley_3);
delay(300);
N5110_clear();
N5110_image(Smiley_4);
delay(300);
}
Output
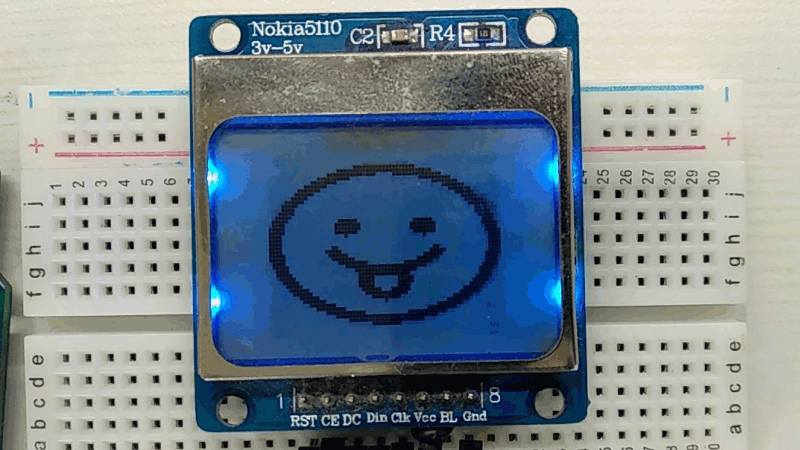
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
Nokia5110 Graphical Display Nokia5110 is 48x84 dot LCD display with Serial Peripheral Interface (SPI) Connectivity. It was designed for cell phones. It is also used in embedded applications. |
X 1 |
Downloads |
||
---|---|---|
|
Nokia5110_Interfacing_With_TI_Launchpad_INO | Download |