Overview of GSM
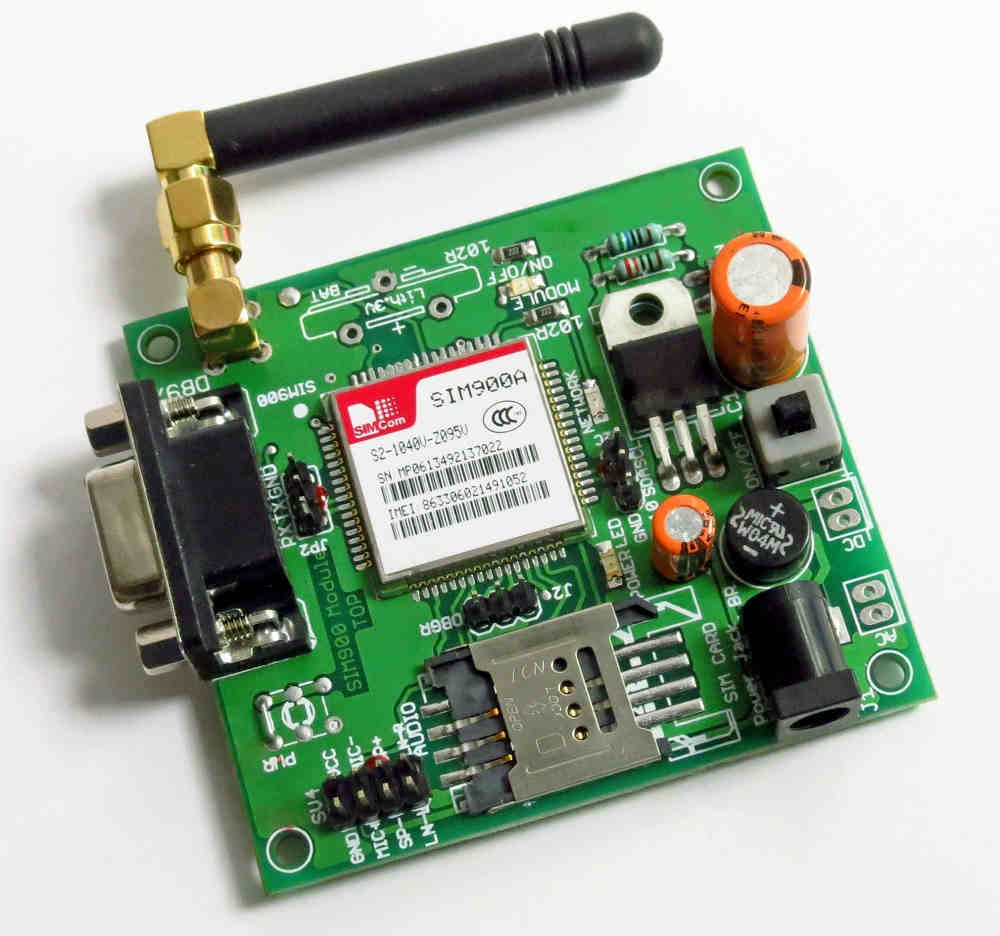
Global System for Mobile communication (GSM) is a digital cellular system used for mobile devices. It is an international standard for mobile which is widely used for long-distance communication.
There are various GSM modules available in markets like SIM900, SIM700, SIM800, SIM808, SIM5320, etc.
SIM900A module allows users to send/receive data over GPRS, send/receive SMS, and make/receive voice calls.
The GSM/GPRS module uses USART communication to communicate with a microcontroller or PC terminal. AT commands are used to configure the module in different modes and to perform various functions like calling, posting data to a site, etc.
For more information about Sim900A and how to use it, refer to the topic Sim900A GSM/GPRS Module in the sensors and modules section.
Connection Diagram of SIM900A with MSP-EXP430G2 TI Launchpad
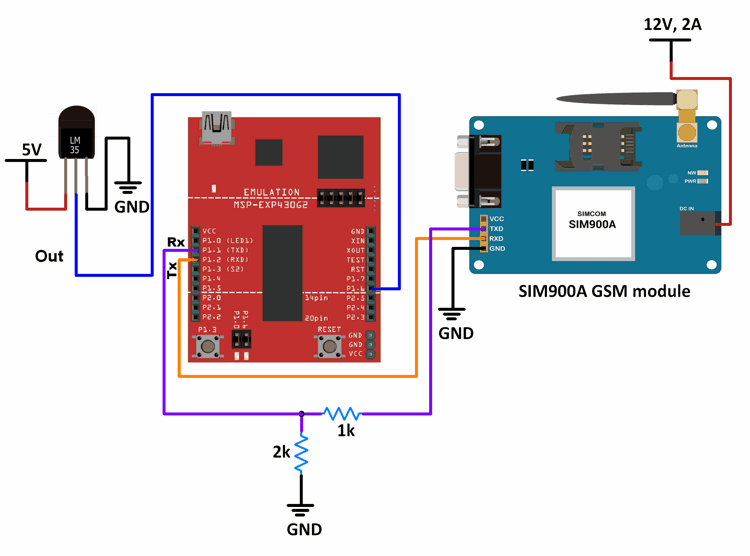
Note: For the TI Launchpad board, P1.1 is the Rx pin and P1.2 is the Tx pin when the jumpers are positioned for using Hardware Serial. Here, we are using, Hardware Serial, hence P1.1 is the Rx pin and P1.2 is the Tx pin.
When jumpers are positioned for software serial, P1.1 acts as the Tx pin, and P1.2 acts as the Rx pin.
Temperature Monitoring and control using SIM900A GSM Module using MSP-EXP430G2 Launchpad
Monitoring temperature and calling a specific number if the temperature increases beyond a certain value. If a certain message is received within a specified time after the call is placed, then, a fan is turned on (LED turned on for demonstration).
Here, an LM35 is used for measuring the temperature. If the temperature measured is more than 35° C, a call is placed to the number specified and the call is cut after 25 seconds. If the user responds with a “Cooldown” message, the fan is turned on (LED turned on for demonstration).
Tread Carefully: MSP-EXP430G2 TI Launchpad board has a RAM of 512 bytes which is easily filled, especially while using different libraries. There are times when you need the Serial buffer to be large enough to contain the data you want and you will have to modify the buffer size for the Serial library. While doing such things, we must ensure that the code does not utilize more than 70% RAM. This could lead to the code working in an erratic manner, working well at times, and failing miserably at others.
There are times when the RAM usage may exceed 70% and the codes will work absolutely fine, and times when the code will not work even when the RAM usage is 65%.
In such cases, a bit of trial and error with the buffer size and/or variables may be necessary.
SIM900 GSM Module Code for MSP-EXP430G2 Launchpad
char data[40];
void flush_rx_buffer(void) /* Flush out the data in the receive buffer*/
{
char c;
while(Serial.available()!=0)
{
c = Serial.read();
}
}
char UnreadSMS(void)
{
int8_t i=0;
char *s;
char rx_buff1[100];
memset(rx_buff1,0,100);
flush_rx_buffer();
Serial.println("AT+CMGF=1"); /* Select message format as text */
delay(700);
flush_rx_buffer();
Serial.println("AT+CMGL=\"REC UNREAD\",1"); /* List received messages without changing their status */
delay(700);
while(Serial.available()!=0) /* If data is available on serial port */
{
rx_buff1[i] = Serial.read(); /* Copy data to a buffer for later use */
i++;
}
if(strstr(rx_buff1,"+CMGL:")!=0) /* If buffer contains +CMGL: */
{
s = strstr(rx_buff1,":"); /* Pointer to : */
return atoi(s+1); /* Return message index extracted, in int form */
}
return 0;
}
void readSMS(int messageIndex, char *data)
{
int8_t k = 0;
char *st;
char index[4];
char rx_buff2[100];
memset(rx_buff2,0,100);
flush_rx_buffer();
Serial.println("AT+CMGF=1"); /* Select message format as text */
delay(700);
flush_rx_buffer();
Serial.print("AT+CMGR="); /* Read message at specified index */
itoa(messageIndex, index, 10);
Serial.println(index);
delay(700);
while(Serial.available()!=0) /* If data is available on serial port */
{
rx_buff2[k] = Serial.read(); /* Copy data to a buffer for later use */
k++;
}
if( (st = strstr(rx_buff2,"+CMGR:")) !=0 ) /* If buffer contains +CMGR: */
{
if( (st = strstr(st,"\r\n"))!=0 ) /* If buffer contains \r\n */
{
Serial.println(st); /* Print message received */
for(int8_t j = 0; j<40 ; j++)
{
*(data + j) = *(st++); /* Store message received in data buffer */
}
}
}
}
void setup() {
Serial.begin(9600); /* Define baud rate for serial communication */
pinMode(2, OUTPUT); /* LED to show whether fan is on or off */
digitalWrite(2, LOW);
}
void loop() {
int16_t temp_adc_val;
float temp_val;
temp_adc_val = analogRead(A6); /* Read temperature from LM35 */
temp_val = (temp_adc_val * 3.22);
temp_val = (temp_val/10);
//Serial.print("Temperature = ");
//Serial.print(temp_val);
//Serial.print(" Degree Celsius\n");
if(temp_val>35)
{
char number[11] = "98xxxxxx09"; /* Mobile number to be called */
memset(data, 0, 40);
//Serial.println("Too hot");
//Serial.println("Calling..");
sprintf(data,"ATD%s;",number);
Serial.println(data);
memset(data,0,40);
delay(25000);
Serial.println("ATH");
delay(700);
int8_t messageIndex,count = 0;
messageIndex = UnreadSMS(); /* Check if new message available */
Serial.print(messageIndex);
while( (messageIndex < 1) && !strstr( data,"Cool down") ) /* No new unread message */
{
if(count == 5)
{
messageIndex = UnreadSMS();
break;
}
count++;
delay(5000);
messageIndex = UnreadSMS();
}
while(messageIndex > 0 ) /* New unread message available */
{
memset(data,0, 40);
delay(1000);
flush_rx_buffer();
readSMS(messageIndex, data);
if(strstr(data,"Cool down")) /* If message received has Cool down */
{
//Serial.print("Fan ON");
digitalWrite(2, HIGH); /* Turn on LED to show fan is on */
memset(data, 0, 40);
}
messageIndex = UnreadSMS();
}
delay(10000);
}
else
{
//Serial.print("\n");
//Serial.print("Everything is fine\n");
digitalWrite(2, LOW); /* Turn off LED to show fan is off */
}
delay(3000);
}
Video of Temperature Monitoring and control using SIM900A GSM Module using MSP-EXP430G2 Launchpad
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
SIM900A GSM GPRS Module SIM900A is dual band GSM/GPRS 900/1800MHz module board used to utilize GSM and GPRS services around the globe. It is used to make/receive voice calls, send/receive text messages, connect to and access the internet over GPRS. |
X 1 |
Downloads |
||
---|---|---|
|
Sim900_GSM_Interfacing_With_TI_Launchpad_INO | Download |