Overview of MPU6050
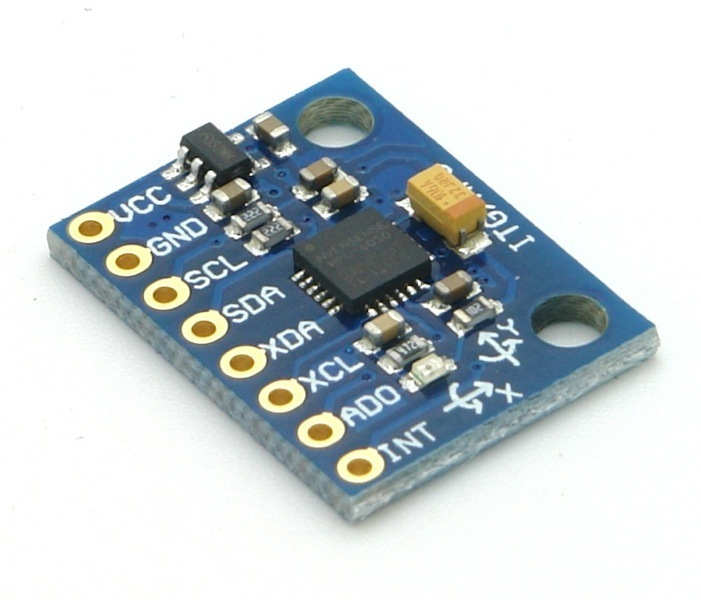
The MPU6050 sensor module is an integrated 6-axis Motion tracking device.
It has a 3-axis Gyroscope, 3-axis Accelerometer, Digital Motion Processor, and a Temperature sensor, all in a single IC.
It can accept inputs from other sensors like a 3-axis magnetometer or pressure sensor using its Auxiliary I2C bus.
If the external 3-axis magnetometer is connected, it can provide complete 9-axis Motion Fusion output.
A microcontroller can communicate with this module using the I2C communication protocol. Various parameters can be found by reading values from addresses of certain registers using I2C communication.
Gyroscope and accelerometer reading along X, Y, and Z axes are available in 2’s complement form. The temperature reading is also available in a signed integer form.
Gyroscope readings are in degrees per second (DPS) unit; Accelerometer readings are in g unit, and Temperature reading is in degrees Celsius.
For more information about the MPU6050 Sensor Module and how to use it, refer to the topic MPU6050 Sensor Module in the sensors and modules section.
Connection Diagram of HMC5883L with MSP-EXP430G2 TI Launchpad
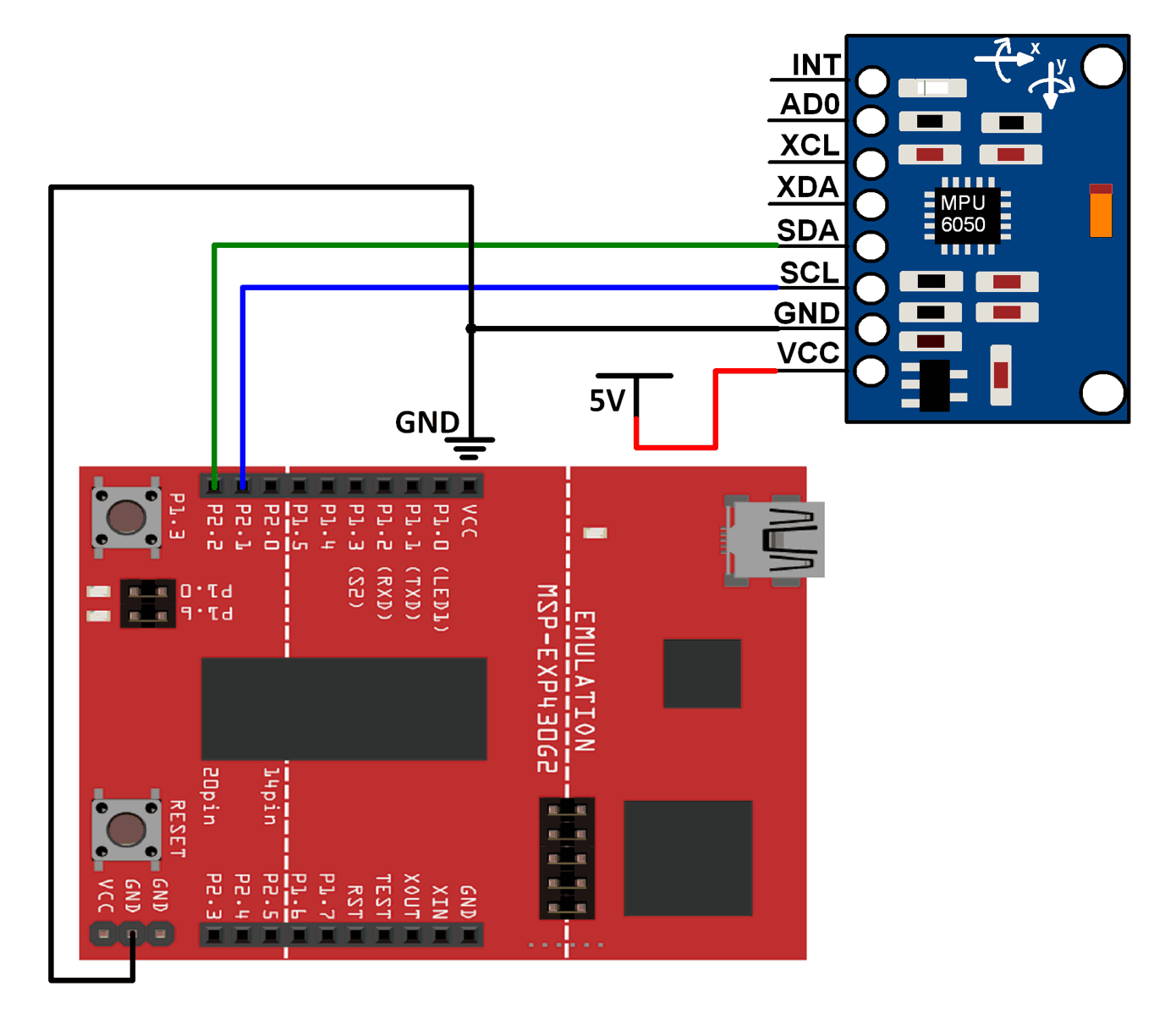
Example
Reading Accelerometer, Gyroscope, and Temperature parameters from the MPU6050 module and displaying them on Energia Serial Monitor.
Here, we will be using Korneliusz Jarzebski’s MPU6050 library from GitHub. This library is for Arduino but works for MSP -EXP430G2 TI Launchpad as well.
Download this library from here.
Extract the library and add it to the libraries folder path of Energia IDE.
For information about how to add a custom library to the Energia IDE and use examples from it, refer to Adding Library To Energia IDE in the Basics section.
Once the library has been added to the Energia IDE, open the IDE and open the example sketch you want from the list of examples from the library added.
Important: The SCL and SDA for MSP-EXP430G2 TI Launchpad are on pins 14 (P1_6) and 15 (P1_7) respectively. The Energia IDE makes use of software-based I2C implementation (twi_sw) for MSP-EXP430G2 TI Launchpad. This software-based I2C implementation is defined with pins 9 (P2_1) and 10 (P2_2) as SCL and SDA pins. Hence, we need to use these pins for the I2C functions (that are used in most of the libraries) of Energia to work on the MSP-EXP430G2 TI Launchpad board. This has been done on Energia 17 and 18 (We have not checked the earlier version of the IDE).
This is not the case for all the Launchpad boards. This has been done so that the booster packs provided by TI work on all the launchpads.
Tread Carefully: MSP-EXP430G2 TI Launchpad board has a RAM of 512 bytes which is easily filled, especially while using different libraries. There are times when you need the Serial buffer to be large enough to contain the data you want and you will have to modify the buffer size for the Serial library. While doing such things, we must ensure that the code does not utilize more than 70% RAM. This could lead to the code working in an erratic manner, working well at times, and failing miserably at others.
There are times when the RAM usage may exceed 70% and the codes will work absolutely fine, and times when the code will not work even when the RAM usage is 65%.
In such cases, a bit of trial and error with the buffer size and/or variables may be necessary.
MPU6050 Accelerometer Measurements Code for MSP-EXP430G2 TI Launchpad
/*
MPU6050 Triple Axis Gyroscope & Accelerometer. Simple Accelerometer Example.
Read more: http://www.jarzebski.pl/arduino/czujniki-i-sensory/3-osiowy-zyroskop-i-akcelerometr-mpu6050.html
GIT: https://github.com/jarzebski/Arduino-MPU6050
Web: http://www.jarzebski.pl
(c) 2014 by Korneliusz Jarzebski
*/
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup()
{
Serial.begin(115200);
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
// If you want, you can set accelerometer offsets
// mpu.setAccelOffsetX();
// mpu.setAccelOffsetY();
// mpu.setAccelOffsetZ();
checkSettings();
}
void checkSettings()
{
Serial.println();
Serial.print(" * Sleep Mode: ");
Serial.println(mpu.getSleepEnabled() ? "Enabled" : "Disabled");
Serial.print(" * Clock Source: ");
switch(mpu.getClockSource())
{
case MPU6050_CLOCK_KEEP_RESET: Serial.println("Stops the clock and keeps the timing generator in reset"); break;
case MPU6050_CLOCK_EXTERNAL_19MHZ: Serial.println("PLL with external 19.2MHz reference"); break;
case MPU6050_CLOCK_EXTERNAL_32KHZ: Serial.println("PLL with external 32.768kHz reference"); break;
case MPU6050_CLOCK_PLL_ZGYRO: Serial.println("PLL with Z axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_YGYRO: Serial.println("PLL with Y axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_XGYRO: Serial.println("PLL with X axis gyroscope reference"); break;
case MPU6050_CLOCK_INTERNAL_8MHZ: Serial.println("Internal 8MHz oscillator"); break;
}
Serial.print(" * Accelerometer: ");
switch(mpu.getRange())
{
case MPU6050_RANGE_16G: Serial.println("+/- 16 g"); break;
case MPU6050_RANGE_8G: Serial.println("+/- 8 g"); break;
case MPU6050_RANGE_4G: Serial.println("+/- 4 g"); break;
case MPU6050_RANGE_2G: Serial.println("+/- 2 g"); break;
}
Serial.print(" * Accelerometer offsets: ");
Serial.print(mpu.getAccelOffsetX());
Serial.print(" / ");
Serial.print(mpu.getAccelOffsetY());
Serial.print(" / ");
Serial.println(mpu.getAccelOffsetZ());
Serial.println();
}
void loop()
{
Vector rawAccel = mpu.readRawAccel();
Vector normAccel = mpu.readNormalizeAccel();
Serial.print(" Xraw = ");
Serial.print(rawAccel.XAxis);
Serial.print(" Yraw = ");
Serial.print(rawAccel.YAxis);
Serial.print(" Zraw = ");
Serial.println(rawAccel.ZAxis);
Serial.print(" Xnorm = ");
Serial.print(normAccel.XAxis);
Serial.print(" Ynorm = ");
Serial.print(normAccel.YAxis);
Serial.print(" Znorm = ");
Serial.println(normAccel.ZAxis);
delay(10);
}
Video of MPU6050 Accelerometer Measurements using MSP-EXP430G2 TI Launchpad
Functions Used
1. mpu.readRawAccel()
- This function gives the decimal equivalent of the 16-bit values of the acceleration in the X, Y, and Z directions.
- This does not give the acceleration values in g units.
2. mpu.readNormalizeAccel()
- This function gives the values of the acceleration in the X, Y, and Z directions in g units.
- It takes into consideration the range setting chosen for the accelerometer and the corresponding sensitivity. Using this it gives acceleration in g units which can vary from 0 to the range chosen.
3. mpu.begin(gyro_scale,accelo_range)
- This function is used to set the range of accelerometer and the scale of gyroscope.
- gyro_scale can be
MPU6050_SCALE_250DPS
,MPU6050_SCALE_500DPS
, MPU6050_SCALE_1000DPS orMPU6050_SCALE_2000DPS
for 250 dps, 500 dps, 1000 dps or 2000 dps respectively. - aceelo_range can be
MPU6050_RANGE_2G
,MPU6050_RANGE_4G
,MPU6050_RANGE_8G
orMPU6050_RANGE_16G
for measurement range of 2g, 4g, 8g or 16g respectively.
MPU6050 Roll and Pitch Measurement Code for MSP-EXP430G2 TI Launchpad
/*
MPU6050 Triple Axis Gyroscope & Accelerometer. Pitch & Roll Accelerometer Example.
Read more: http://www.jarzebski.pl/arduino/czujniki-i-sensory/3-osiowy-zyroskop-i-akcelerometr-mpu6050.html
GIT: https://github.com/jarzebski/Arduino-MPU6050
Web: http://www.jarzebski.pl
(c) 2014 by Korneliusz Jarzebski
*/
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup()
{
Serial.begin(115200);
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
}
void loop()
{
// Read normalized values
Vector normAccel = mpu.readNormalizeAccel();
// Calculate Pitch & Roll
int pitch = -(atan2(normAccel.XAxis, sqrt(normAccel.YAxis*normAccel.YAxis + normAccel.ZAxis*normAccel.ZAxis))*180.0)/M_PI;
int roll = (atan2(normAccel.YAxis, normAccel.ZAxis)*180.0)/M_PI;
// Output
Serial.print(" Pitch = ");
Serial.print(pitch);
Serial.print(" Roll = ");
Serial.print(roll);
Serial.println();
delay(10);
}
Video of MPU6050 Roll and Pitch Measurement MSP-EXP430G2 TI Launchpad
MPU6050 Simple Gyroscope Measurements Code for MSP-EXP430G2 TI Launchpad
/*
MPU6050 Triple Axis Gyroscope & Accelerometer. Simple Gyroscope Example.
Read more: http://www.jarzebski.pl/arduino/czujniki-i-sensory/3-osiowy-zyroskop-i-akcelerometr-mpu6050.html
GIT: https://github.com/jarzebski/Arduino-MPU6050
Web: http://www.jarzebski.pl
(c) 2014 by Korneliusz Jarzebski
*/
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup()
{
Serial.begin(115200);
// Initialize MPU6050
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
// If you want, you can set gyroscope offsets
// mpu.setGyroOffsetX(155);
// mpu.setGyroOffsetY(15);
// mpu.setGyroOffsetZ(15);
// Calibrate gyroscope. The calibration must be at rest.
// If you don't want calibrate, comment this line.
mpu.calibrateGyro();
// Set threshold sensivty. Default 3.
// If you don't want use threshold, comment this line or set 0.
// mpu.setThreshold(3);
// Check settings
checkSettings();
}
void checkSettings()
{
Serial.println();
Serial.print(" * Sleep Mode: ");
Serial.println(mpu.getSleepEnabled() ? "Enabled" : "Disabled");
Serial.print(" * Clock Source: ");
switch(mpu.getClockSource())
{
case MPU6050_CLOCK_KEEP_RESET: Serial.println("Stops the clock and keeps the timing generator in reset"); break;
case MPU6050_CLOCK_EXTERNAL_19MHZ: Serial.println("PLL with external 19.2MHz reference"); break;
case MPU6050_CLOCK_EXTERNAL_32KHZ: Serial.println("PLL with external 32.768kHz reference"); break;
case MPU6050_CLOCK_PLL_ZGYRO: Serial.println("PLL with Z axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_YGYRO: Serial.println("PLL with Y axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_XGYRO: Serial.println("PLL with X axis gyroscope reference"); break;
case MPU6050_CLOCK_INTERNAL_8MHZ: Serial.println("Internal 8MHz oscillator"); break;
}
Serial.print(" * Gyroscope: ");
switch(mpu.getScale())
{
case MPU6050_SCALE_2000DPS: Serial.println("2000 dps"); break;
case MPU6050_SCALE_1000DPS: Serial.println("1000 dps"); break;
case MPU6050_SCALE_500DPS: Serial.println("500 dps"); break;
case MPU6050_SCALE_250DPS: Serial.println("250 dps"); break;
}
Serial.print(" * Gyroscope offsets: ");
Serial.print(mpu.getGyroOffsetX());
Serial.print(" / ");
Serial.print(mpu.getGyroOffsetY());
Serial.print(" / ");
Serial.println(mpu.getGyroOffsetZ());
Serial.println();
}
void loop()
{
Vector rawGyro = mpu.readRawGyro();
Vector normGyro = mpu.readNormalizeGyro();
Serial.print(" Xraw = ");
Serial.print(rawGyro.XAxis);
Serial.print(" Yraw = ");
Serial.print(rawGyro.YAxis);
Serial.print(" Zraw = ");
Serial.println(rawGyro.ZAxis);
Serial.print(" Xnorm = ");
Serial.print(normGyro.XAxis);
Serial.print(" Ynorm = ");
Serial.print(normGyro.YAxis);
Serial.print(" Znorm = ");
Serial.println(normGyro.ZAxis);
delay(10);
}
Video of MPU6050 Gyroscope Measurements using MSP-EXP430G2 TI Launchpad
Functions Used
1. mpu.readRawGyro()
- This function gives the decimal equivalent of the 16-bit values of the gyroscope rotation in the X, Y, and Z directions.
- This does not give the value of gyroscope parameters in DPS (degrees per second) units.
2. mpu.readNormalizeGyro()
- This function gives the value of the gyroscope parameters in the X, Y, and Z directions in DPS units.
- It takes into consideration the scale setting chosen for the gyroscope and the corresponding sensitivity. Using this it gives gyroscope rotation in DPS units.
MPU6050 Roll, Pitch, and Yaw Measurement Using Gyroscope Code for MSP-EXP430G2 TI Launchpad
/*
MPU6050 Triple Axis Gyroscope & Accelerometer. Pitch & Roll & Yaw Gyroscope Example.
Read more: http://www.jarzebski.pl/arduino/czujniki-i-sensory/3-osiowy-zyroskop-i-akcelerometr-mpu6050.html
GIT: https://github.com/jarzebski/Arduino-MPU6050
Web: http://www.jarzebski.pl
(c) 2014 by Korneliusz Jarzebski
*/
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
// Timers
unsigned long timer = 0;
float timeStep = 0.01;
// Pitch, Roll and Yaw values
float pitch = 0;
float roll = 0;
float yaw = 0;
void setup()
{
Serial.begin(115200);
// Initialize MPU6050
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
// Calibrate gyroscope. The calibration must be at rest.
// If you don't want calibrate, comment this line.
mpu.calibrateGyro();
// Set threshold sensivty. Default 3.
// If you don't want use threshold, comment this line or set 0.
// mpu.setThreshold(3);
}
void loop()
{
timer = millis();
// Read normalized values
Vector norm = mpu.readNormalizeGyro();
// Calculate Pitch, Roll and Yaw
pitch = pitch + norm.YAxis * timeStep;
roll = roll + norm.XAxis * timeStep;
yaw = yaw + norm.ZAxis * timeStep;
// Output raw
Serial.print(" Pitch = ");
Serial.print(pitch);
Serial.print(" Roll = ");
Serial.print(roll);
Serial.print(" Yaw = ");
Serial.println(yaw);
// Wait to full timeStep period
delay((timeStep*1000) - (millis() - timer));
}
MPU6050 Temperature Measurement Code for MSP-EXP430G2 TI Launchpad
/*
MPU6050 Triple Axis Gyroscope & Accelerometer. Temperature Example.
Read more: http://www.jarzebski.pl/arduino/czujniki-i-sensory/3-osiowy-zyroskop-i-akcelerometr-mpu6050.html
GIT: https://github.com/jarzebski/Arduino-MPU6050
Web: http://www.jarzebski.pl
(c) 2014 by Korneliusz Jarzebski
*/
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup()
{
Serial.begin(115200);
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
}
void loop()
{
float temp = mpu.readTemperature();
Serial.print(" Temp = ");
Serial.print(temp);
Serial.println(" *C");
delay(500);
}
Video of MPU6050 Temperature Measurement using MSP-EXP430G2 TI Launchpad
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
MPU6050 Gyroscope and Accelerometer MPU6050 (Gyroscope + Accelerometer + Temperature) is a combination of 3-axis Gyroscope, 3-axis Accelerometer and Temperature sensor with on-chip Digital Motion Processor (DMP). It is used in mobile devices, motion enabled games, 3D mice, Gesture (motion command) technology etc |
X 1 |
Downloads |
||
---|---|---|
|
MPU6050 Library | Download |