Overview of DS1307 RTC
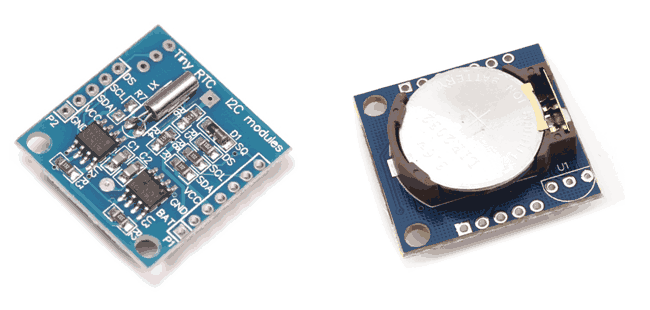
- Real-Time Clock (RTC) is used for monitoring time and maintaining a calendar.
- In order to use an RTC, we need to first program it with the current date and time. Once this is done, the RTC registers can be read at any time to know the time and date.
- DS1307 is an RTC that works on I2C protocol. Data from various registers can be read by accessing their addresses for reading using I2C communication.
For information on DS1307 and how to use it, refer to the topic RTC (Real Time Clock) DS1307 Module in the sensors and modules section.
Connection Diagram of DS1307 with MSP-EXP430G2 TI Launchpad
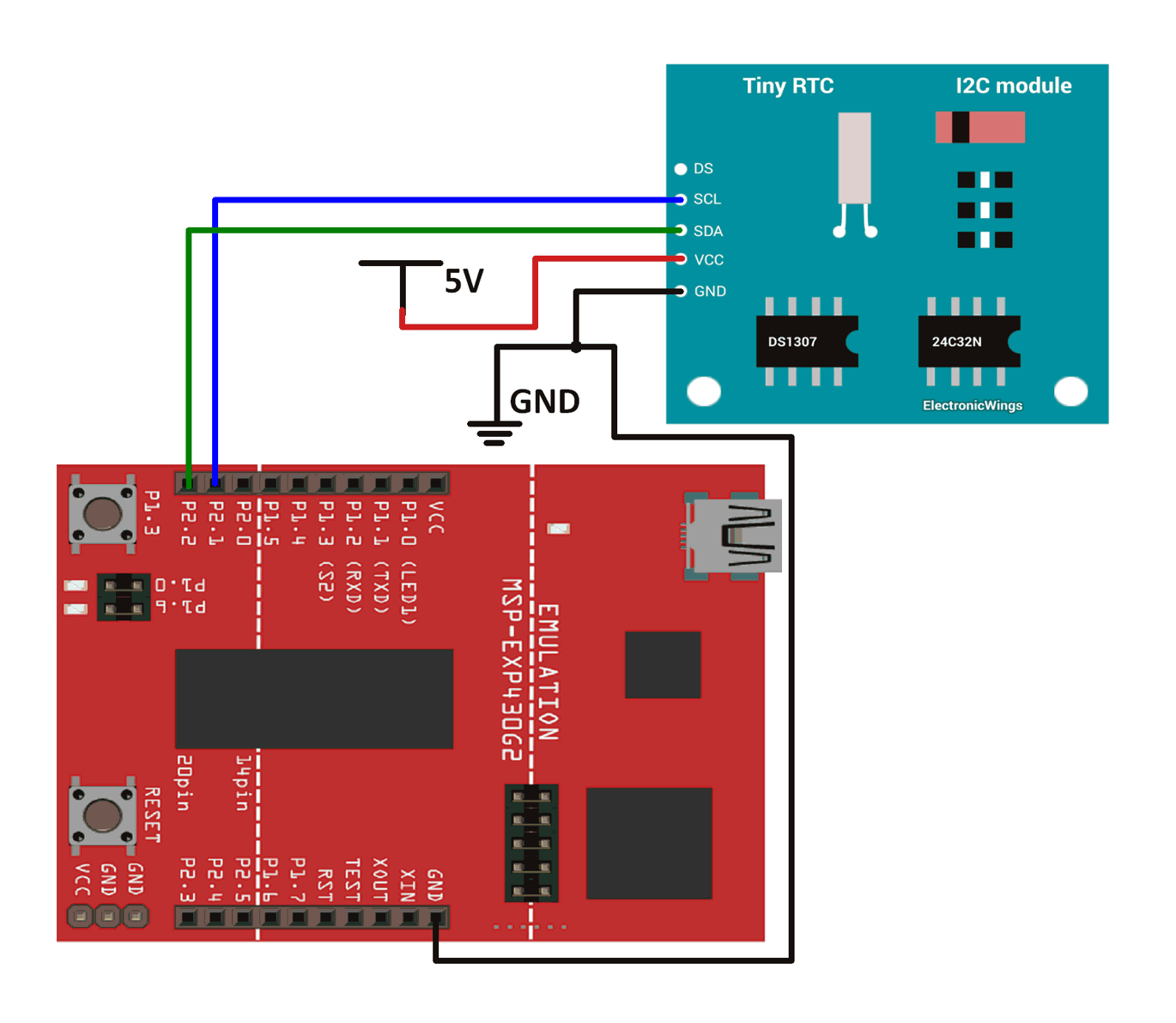
Example
Programming RTC to current date and time; and reading the date and time from the RTC.
Here, we will be using the DS1307 library by Watterott from GitHub. This library is for Arduino but works for MSP-EXP430G2 TI Launchpad as well.
Download this library from here.
Extract the library and add the folder named DS1307 to the libraries folder path of Energia IDE.
For information about how to add a custom library to the Energia IDE and use examples from it, refer to Adding Library To Energia IDE in the Basics section.
Once the library has been added to the Energia IDE, open the IDE and open the example sketch named Example from the DS1307 library added.
Important: The SCL and SDA for MSP-EXP430G2 TI Launchpad are on pins 14 (P1_6) and 15 (P1_7) respectively. The Energia IDE makes use of software-based I2C implementation (twi_sw) for MSP-EXP430G2 TI Launchpad. This software-based I2C implementation is defined with pins 9 (P2_1) and 10 (P2_2) as SCL and SDA pins. Hence, we need to use these pins for the I2C functions (that are used in most of the libraries) of Energia to work on the MSP-EXP430G2 TI Launchpad board. This has been done on Energia 17 and 18 (Have not checked earlier version of the IDE).
This is not the case for all the Launchpad boards. This has been done so that the booster packs provided by TI work on all the launchpads.
Word Of Caution : In the example sketch, in setup loop, rtc.set() function is used. Pass the current date and time arguments as mentioned to this function. In the example sketch, this statement will be commented. Uncomment it and upload the sketch. Once the sketch is uploaded, uncomment the statement again and upload the sketch. If this is not done, each time the MSP-EXP430G2 TI Launchpad board resets or is powered on after power off, the date and time you set will be set over and over again and you will not be able to read the exact current time and date.
Tread Carefully: MSP-EXP430G2 TI Launchpad board has a RAM of 512 bytes which is easily filled, especially while using different libraries. There are times when you need the Serial buffer to be large enough to contain the data you want and you will have to modify the buffer size for the Serial library. While doing such things, we must ensure that the code does not utilize more than 70% RAM. This could lead to the code working in an erratic manner, working well at times and failing miserably at others.
There are times when the RAM usage may exceed 70% and the codes will work absolutely fine, and times when the code will not work even when the RAM usage is 65%.
In such cases, a bit of trial and error with the buffer size and/or variables may be necessary.
Setting And Reading Time And Date In DS1307 using MSP-EXP430G2 TI Launchpad
/*
DS1307 RTC (Real-Time-Clock) Example
Uno A4 (SDA), A5 (SCL)
Mega 20 (SDA), 21 (SCL)
Leonardo 2 (SDA), 3 (SCL)
*/
#include <Wire.h>
#include <DS1307.h>
DS1307 rtc;
void setup()
{
//init Serial port
Serial.begin(9600);
while(!Serial); //wait for serial port to connect - needed for Leonardo only
//init RTC
Serial.println("Init RTC...");
//only set the date+time one time
rtc.set(0, 0, 8, 24, 12, 2014); //08:00:00 24.12.2014 //sec, min, hour, day, month, year
//stop/pause RTC
// rtc.stop();
//start RTC
rtc.start();
}
void loop()
{
uint8_t sec, min, hour, day, month;
uint16_t year;
//get time from RTC
rtc.get(&sec, &min, &hour, &day, &month, &year);
//serial output
Serial.print("\nTime: ");
Serial.print(hour, DEC);
Serial.print(":");
Serial.print(min, DEC);
Serial.print(":");
Serial.print(sec, DEC);
Serial.print("\nDate: ");
Serial.print(day, DEC);
Serial.print(".");
Serial.print(month, DEC);
Serial.print(".");
Serial.print(year, DEC);
//wait a second
delay(1000);
}
Video of DS1307 using MSP-EXP430G2 TI Launchpad
DS1307 Functions Used
DS1307 rtc
- This defines an object named rtc of the class DS1307.
rtc.set(uint8_t sec, uint8_t min, uint8_t hour, uint8_t day, uint8_t month, uint16_t year)
- This function is used to set the current time and date into the DS1307 RTC IC.
rtc.start()
- This function is used to start I2C communication with DS1307.
- It fetches the SEC and CH byte from the DS1307 Timekeeper registers.
rtc.get(uint8_t *sec, uint8_t *min, uint8_t *hour, uint8_t *day, uint8_t *month, uint16_t *year)
- This function is used to get the time and date stored in DS1307 IC.
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
DS1307 RTC DS1307 RTC DS1307 RTC DS1307 RTC |
X 1 |
Downloads |
||
---|---|---|
|
DS1307 Library | Download |