Overview of Soil Moisture
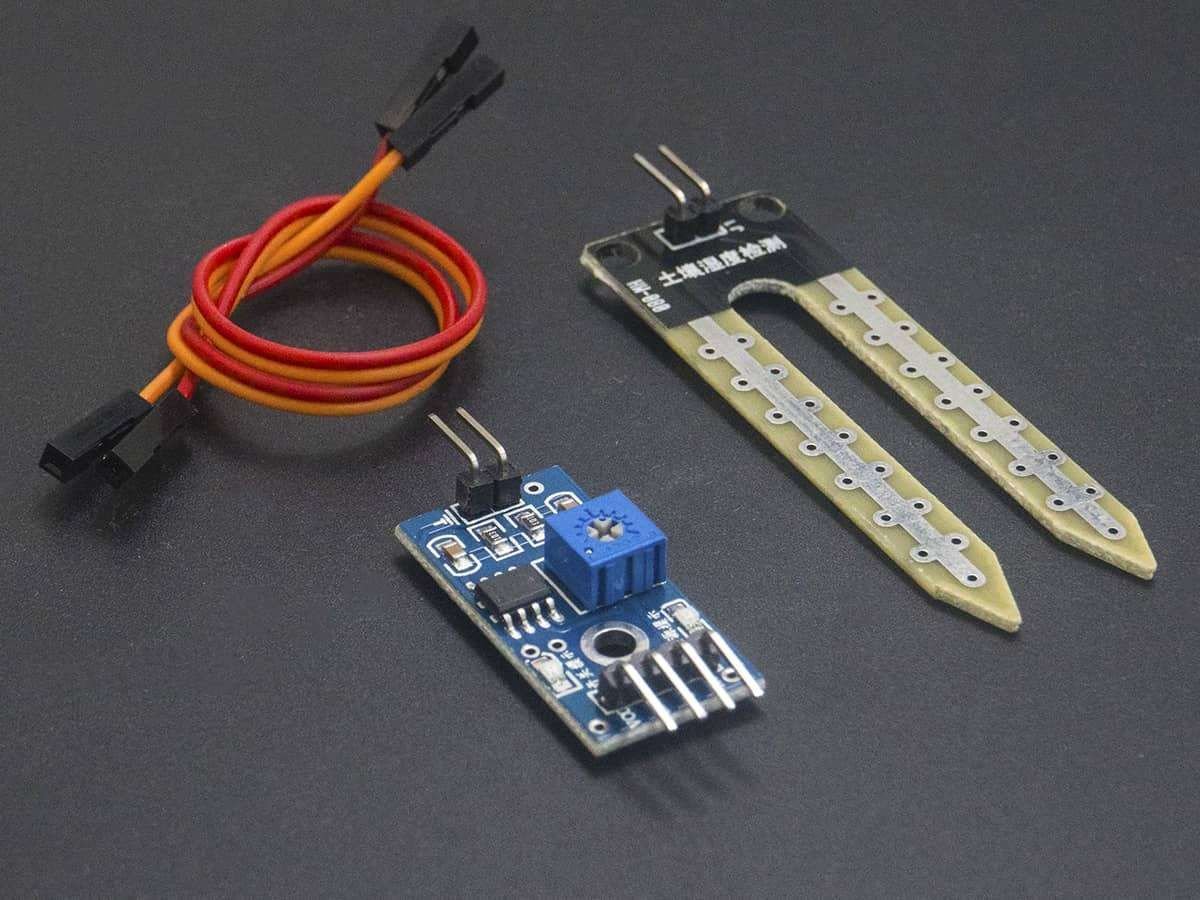
A soil moisture sensor is a simple sensor consisting of two conducting plates that act as a probe. It measures the moisture content present in the soil.
The resistance between the two conducting plates varies inversely with the moisture content present in the soil.
This change in resistance can be used as a measure of the moisture content in the soil.
The sensor is used in series with a fixed resistance to form a voltage divider network whose output varies with the moisture content in the soil.
The output voltage can be processed through an ADC and used according to the need of the application.
For more information about soil moisture sensor and how to use it, refer to the topic Soil Moisture Sensor in the sensors and modules section.
For information about ADC in ATmega16 and how to use it, refer to the topic ADC in AVR ATmega16/ATmega32 in the ATmega inside section.
.png)
Connection Diagram of Soil Moisture Sensor with ATmega16/32
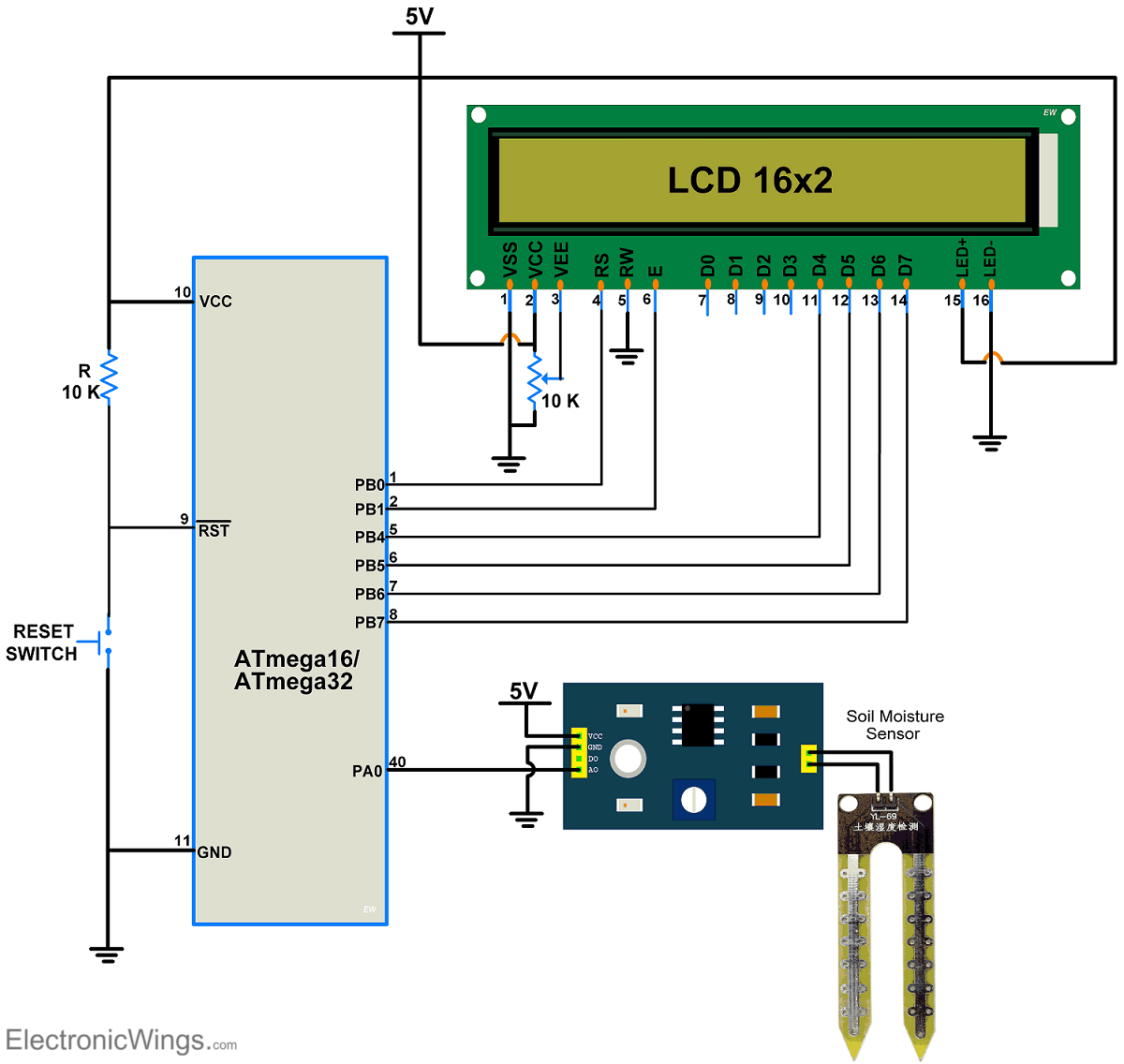
- The complete interfacing diagram of the soil moisture sensor is shown in the above figure.
- Here we use the soil moisture sensor module, which gives output analog as well as digital. Here, we will take the analog output from this module.
- The output of the soil moisture sensor changes in the range of ADC value is 0 – 1023, and to show in percentage from the given formula,
Analog output = (ADC Value / 1023)
Moisture in percentage = 100 – (Analog output * 100)
- For zero moisture, we get a 10-bit ADC value maximum i.e. 1023 which in turn gives 0% moisture.
Soil Moisture Sensor Code for ATmega16/32
/*
* ATmega16_soil_moisture.c
* http://www.electronicwings.com
*/
#include <avr/io.h>
#include "LCD16x2_4bit.h"
#include <util/delay.h>
#include <stdlib.h>
#include <string.h>
void ADC_Init()
{
DDRA=0x0; /* Make ADC port as input */
ADCSRA = 0x87; /* Enable ADC, fr/128 */
}
int ADC_Read()
{
ADMUX = 0x40; /* Vref: Avcc, ADC channel: 0 */
ADCSRA |= (1<<ADSC); /* start conversion */
while ((ADCSRA &(1<<ADIF))==0); /* monitor end of conversion interrupt flag */
ADCSRA |=(1<<ADIF); /* set the ADIF bit of ADCSRA register */
return(ADCW); /* return the ADCW */
}
int main(void)
{
lcdinit(); /* initialize the 16x2 LCD */
lcd_clear(); /* clear the LCD */
ADC_Init(); /* initialize the ADC */
char array[10];
int adc_value;
float moisture;
while(1)
{
adc_value = ADC_Read(); /* Copy the ADC value */
moisture = 100-(adc_value*100.00)/1023.00; /* Calculate moisture in % */
lcd_gotoxy(0,0);
lcd_print("Moisture: ");
dtostrf(moisture,3,2,array);
strcat(array,"% "); /* Concatenate unit of % */
lcd_gotoxy(0,1); /* set column and row */
lcd_print(array); /* Print moisture on second row */
memset(array,0,10);
_delay_ms(500);
}
}
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
Soil Moisture Sensor Soil moisture sensor is used to measure the water content (moisture) in soil. It is used in agriculture applications, irrigation and gardening systems, etc. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 |
Downloads |
||
---|---|---|
|
ATmega16 soil moisture Project File | Download |