Introduction
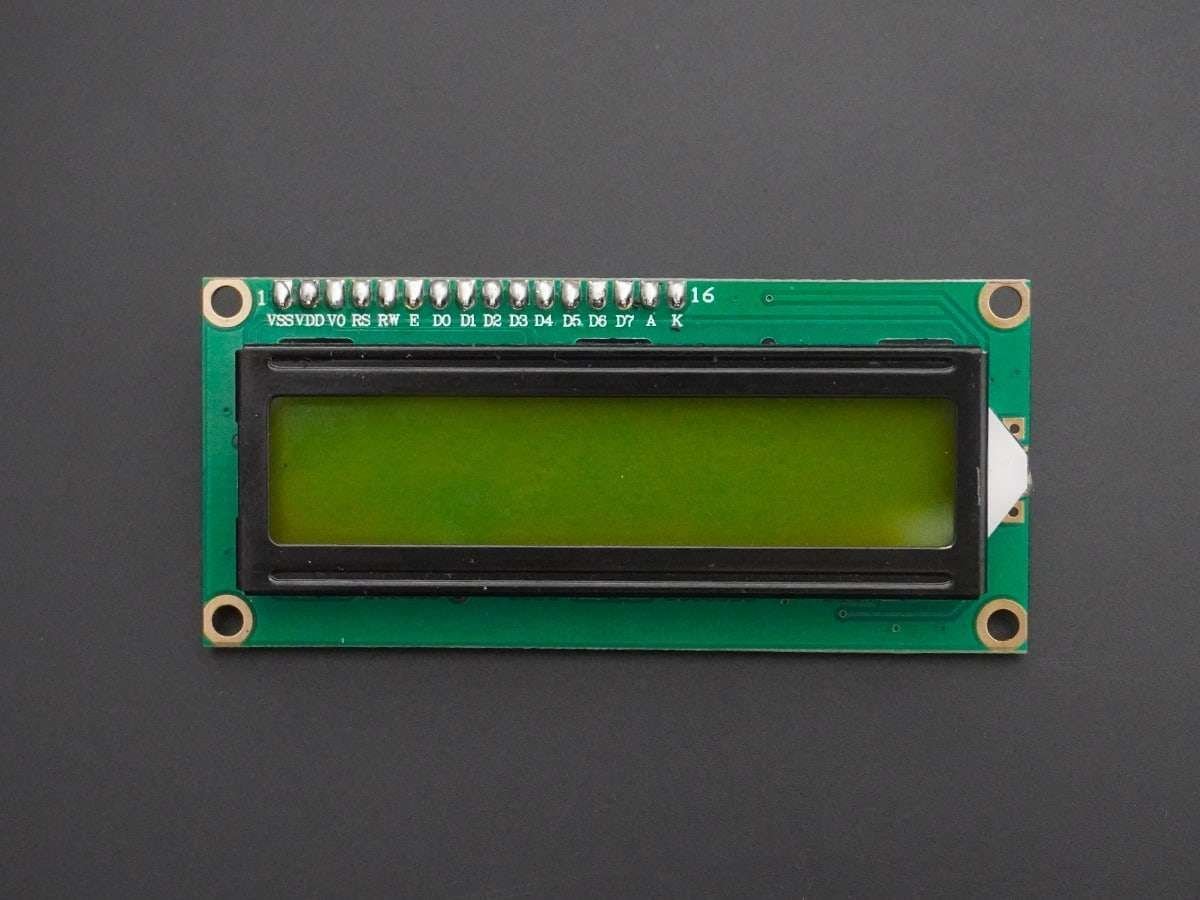
LCDs (Liquid Crystal Displays) are used for displaying status or parameters in embedded systems.
LCD 16x2 is a 16 pin device which has 8 data pins (D0-D7) and 3 control pins (RS, RW, EN). The remaining 5 pins are for supply and backlight for the LCD.
The control pins help us configure the LCD in command mode or data mode. They also help configure read mode or write mode and also when to read or write.
LCD 16x2 can be used in 4-bit mode or 8-bit mode depending on the requirement of the application. In order to use it, we need to send certain commands to the LCD in command mode and once the LCD is configured according to our need, we can send the required data in data mode.
For more information about LCD 16x2 and how to use it, refer to the topic LCD 16x2 display module in the sensors and modules section.
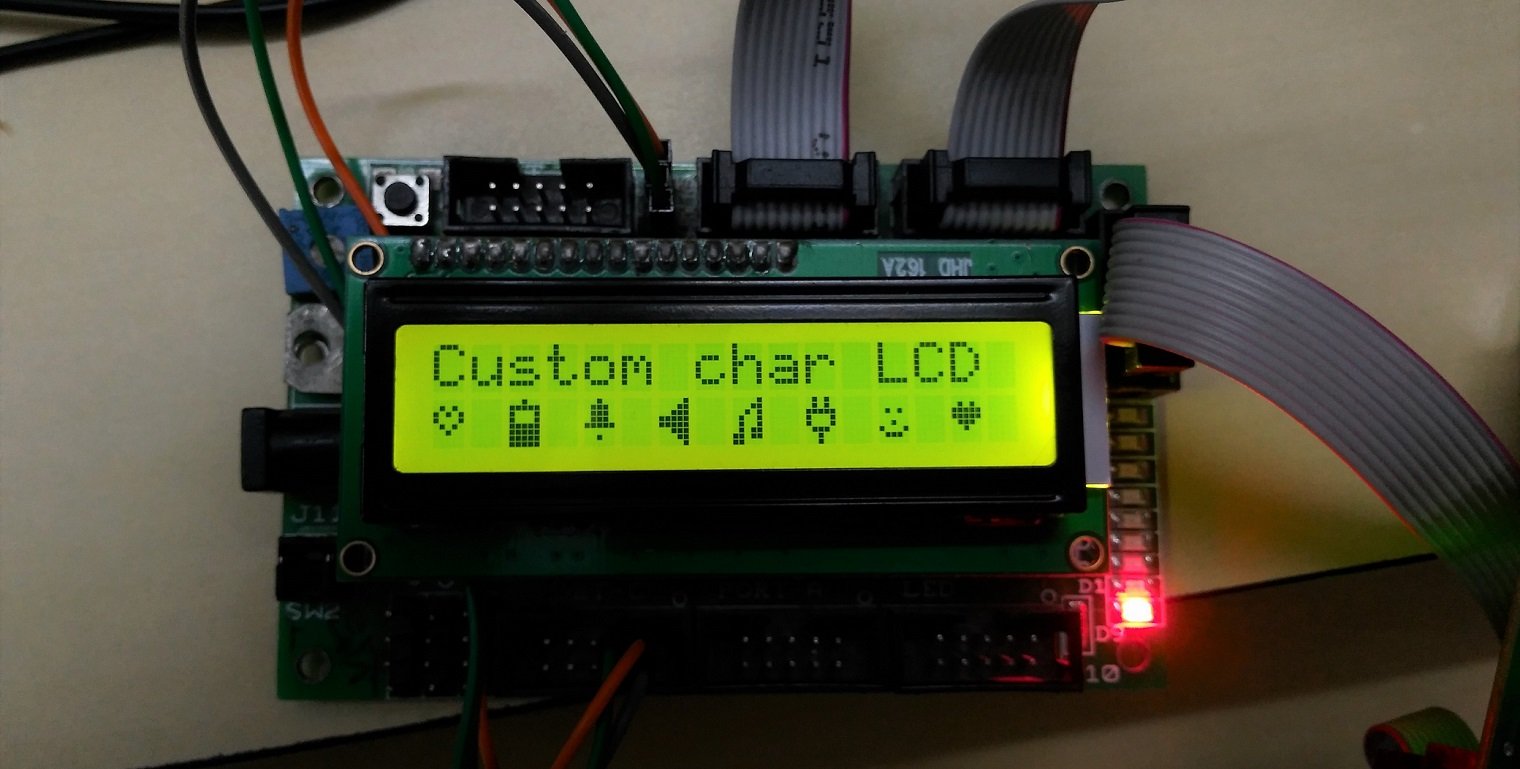
Connection Diagram of LCD16x2 with ATmega16/32
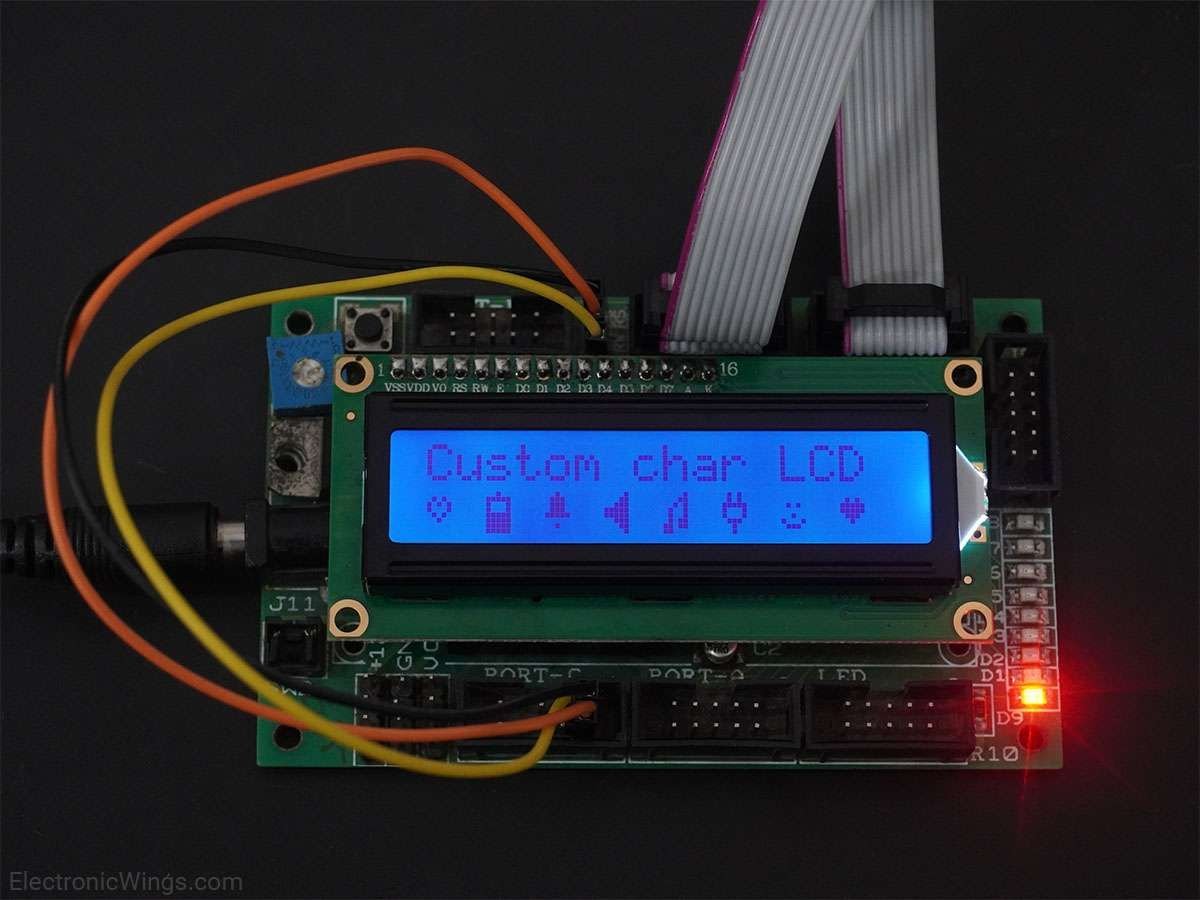
Suppose, we decide to put “Bell” shape custom character at pattern number 1 then to store them in CGRAM following function is used.
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char i;
if(loc<8)
{
LCD_Command (0x40 + (loc*8)); /* Command 0x40 and onwards forces
the device to point CGRAM address */
for(i=0;i<8;i++) /* Write 8 byte for generation of 1 character */
LCD_Char(msg[i]);
}
}
The above function will be used to store the custom characters in CGRAM.
Display Custom Characters on LCD16x2 Using ATmega16/32
After storing all custom characters in CGRAM, we can display it on LCD16x2.
To display custom characters, simply provide custom character number (from 0 to 7) as a data to LCD16x2.
LCD16x2 Code for ATmega16/32
Printing custom characters on lcd16x2.
/*
LCD16x2 8 bit ATmega16 interface
http://www.electronicwings.com
*/
#define F_CPU 8000000UL /* Define CPU Frequency e.g. here its 8MHz */
#include <avr/io.h> /* Include AVR std. library file */
#include <util/delay.h> /* Include inbuilt defined Delay header file */
#define LCD_Data_Dir DDRB /* Define LCD data port direction */
#define LCD_Command_Dir DDRC /* Define LCD command port direction register */
#define LCD_Data_Port PORTB /* Define LCD data port */
#define LCD_Command_Port PORTC /* Define LCD data port */
#define RS PC0 /* Define Register Select signal pin */
#define RW PC1 /* Define Read/Write signal pin */
#define EN PC2 /* Define Enable signal pin */
void LCD_Command(unsigned char cmnd)
{
LCD_Data_Port= cmnd;
LCD_Command_Port &= ~(1<<RS); /* RS=0 command reg. */
LCD_Command_Port &= ~(1<<RW); /* RW=0 Write operation */
LCD_Command_Port |= (1<<EN); /* Enable pulse */
_delay_us(1);
LCD_Command_Port &= ~(1<<EN);
_delay_ms(2);
}
void LCD_Char (unsigned char char_data) /* LCD data write function */
{
LCD_Data_Port= char_data;
LCD_Command_Port |= (1<<RS); /* RS=1 Data reg. */
LCD_Command_Port &= ~(1<<RW); /* RW=0 write operation */
LCD_Command_Port |= (1<<EN); /* Enable Pulse */
_delay_us(1);
LCD_Command_Port &= ~(1<<EN);
_delay_ms(2); /* Data write delay */
}
void LCD_Init (void) /* LCD Initialize function */
{
LCD_Command_Dir = 0xFF; /* Make LCD command port direction as o/p */
LCD_Data_Dir = 0xFF; /* Make LCD data port direction as o/p */
_delay_ms(20); /* LCD Power ON delay always >15ms */
LCD_Command (0x38); /* Initialization of 16X2 LCD in 8bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* clear display */
_delay_ms(2); /* Clear display command delay> 1.63 ms */
LCD_Command (0x80); /* Cursor at home position */
}
void LCD_String (char *str) /* Send string to LCD function */
{
int i;
for(i=0;str[i]!=0;i++) /* Send each char of string till the NULL */
{
LCD_Char (str[i]);
}
}
void LCD_String_xy (char row, char pos, char *str) /* Send string to LCD with xy position */
{
if (row == 0 && pos<16)
LCD_Command((pos & 0x0F)|0x80); /* Command of first row and required position<16 */
else if (row == 1 && pos<16)
LCD_Command((pos & 0x0F)|0xC0); /* Command of first row and required position<16 */
LCD_String(str); /* Call LCD string function */
}
void LCD_Clear()
{
LCD_Command (0x01); /* clear display */
LCD_Command (0x80); /* cursor at home position */
}
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char i;
if(loc<8)
{
LCD_Command (0x40 + (loc*8)); /* Command 0x40 and onwards forces the device to point CGRAM address */
for(i=0;i<8;i++) /* Write 8 byte for generation of 1 character */
LCD_Char(msg[i]);
}
}
int main()
{
char i;
unsigned char Character1[8] = { 0x00, 0x0A, 0x15, 0x11, 0x0A, 0x04, 0x00, 0x00 }; /* Custom char set for alphanumeric LCD Module */
unsigned char Character2[8] = { 0x04, 0x1F, 0x11, 0x11, 0x1F, 0x1F, 0x1F, 0x1F };
unsigned char Character3[8] = { 0x04, 0x0E, 0x0E, 0x0E, 0x1F, 0x00, 0x04, 0x00 };
unsigned char Character4[8] = { 0x01, 0x03, 0x07, 0x1F, 0x1F, 0x07, 0x03, 0x01 };
unsigned char Character5[8] = { 0x01, 0x03, 0x05, 0x09, 0x09, 0x0B, 0x1B, 0x18 };
unsigned char Character6[8] = { 0x0A, 0x0A, 0x1F, 0x11, 0x11, 0x0E, 0x04, 0x04 };
unsigned char Character7[8] = { 0x00, 0x00, 0x0A, 0x00, 0x04, 0x11, 0x0E, 0x00 };
unsigned char Character8[8] = { 0x00, 0x0A, 0x1F, 0x1F, 0x0E, 0x04, 0x00, 0x00 };
LCD_Init();
LCD_Custom_Char(0, Character1); /* Build Character1 at position 0 */
LCD_Custom_Char(1, Character2); /* Build Character2 at position 1 */
LCD_Custom_Char(2, Character3); /* Build Character3 at position 2 */
LCD_Custom_Char(3, Character4); /* Build Character4 at position 3 */
LCD_Custom_Char(4, Character5); /* Build Character5 at position 4 */
LCD_Custom_Char(5, Character6); /* Build Character6 at position 5 */
LCD_Custom_Char(6, Character7); /* Build Character6 at position 6 */
LCD_Custom_Char(7, Character8); /* Build Character6 at position 7 */
LCD_Command(0x80); /*cursor at home position */
LCD_String("Custom char LCD");
LCD_Command(0xc0);
for(i=0;i<8;i++) /* function will send data 1 to 8 to lcd */
{
LCD_Char(i); /* char at 'i'th position will display on lcd */
LCD_Char(' '); /* space between each custom char. */
}
while(1);
}
Video of LCD16x2 Custom Charactor Display using ATmega16/32
Example 2
Animation: Pacman chasing Dot
/*
LCD16x2 8 bit ATmega16 interface
http://www.electronicwings.com
*/
#define F_CPU 8000000UL /* Define CPU Frequency e.g. here its 8MHz */
#include <avr/io.h> /* Include AVR std. library file */
#include <util/delay.h> /* Include inbuilt defined Delay header file */
#define LCD_Data_Dir DDRB /* Define LCD data port direction */
#define LCD_Command_Dir DDRC /* Define LCD command port direction register */
#define LCD_Data_Port PORTB /* Define LCD data port */
#define LCD_Command_Port PORTC /* Define LCD data port */
#define RS PC0 /* Define Register Select signal pin */
#define RW PC1 /* Define Read/Write signal pin */
#define EN PC2 /* Define Enable signal pin */
void LCD_Command(unsigned char cmnd)
{
LCD_Data_Port= cmnd;
LCD_Command_Port &= ~(1<<RS); /* RS=0 command reg. */
LCD_Command_Port &= ~(1<<RW); /* RW=0 Write operation */
LCD_Command_Port |= (1<<EN); /* Enable pulse */
_delay_us(1);
LCD_Command_Port &= ~(1<<EN);
_delay_ms(2);
}
void LCD_Char (unsigned char char_data) /* LCD data write function */
{
LCD_Data_Port= char_data;
LCD_Command_Port |= (1<<RS); /* RS=1 Data reg. */
LCD_Command_Port &= ~(1<<RW); /* RW=0 write operation */
LCD_Command_Port |= (1<<EN); /* Enable Pulse */
_delay_us(1);
LCD_Command_Port &= ~(1<<EN);
_delay_ms(2); /* Data write delay */
}
void LCD_Init (void) /* LCD Initialize function */
{
LCD_Command_Dir = 0xFF; /* Make LCD command port direction as o/p */
LCD_Data_Dir = 0xFF; /* Make LCD data port direction as o/p */
_delay_ms(20); /* LCD Power ON delay always >15ms */
LCD_Command (0x38); /* Initialization of 16X2 LCD in 8bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* clear display */
_delay_ms(2); /* Clear display command delay> 1.63 ms */
LCD_Command (0x80); /* Cursor at home position */
}
void LCD_String (char *str) /* Send string to LCD function */
{
int i;
for(i=0;str[i]!=0;i++) /* Send each char of string till the NULL */
{
LCD_Char (str[i]);
}
}
void LCD_String_xy (char row, char pos, char *str) /* Send string to LCD with xy position */
{
if (row == 0 && pos<16)
LCD_Command((pos & 0x0F)|0x80); /* Command of first row and required position<16 */
else if (row == 1 && pos<16)
LCD_Command((pos & 0x0F)|0xC0); /* Command of first row and required position<16 */
LCD_String(str); /* Call LCD string function */
}
void LCD_Clear()
{
LCD_Command (0x01); /* clear display */
LCD_Command (0x80); /* cursor at home position */
}
void LCD_Custom_Char (unsigned char loc, unsigned char *msg)
{
unsigned char j;
if(loc<8)
{
LCD_Command (0x40 + (loc*8)); /* Command 0x40 and onwards forces the device to point CGRAM address */
for(j=0;j<8;j++) /* Write 8 byte for generation of 1 character */
LCD_Char(msg[j]);
}
}
void show_set1()
{
LCD_Char(0x0);
LCD_Char(0x01);
}
void show_set2()
{
LCD_Char(0x2);
LCD_Char(0x3);
}
void show_dots(char j)
{
LCD_Command(0x80|(addr&0x0f));
LCD_Char(j);
}
int main(void)
{
LCD_Init();
LCD_String("Ewings");
LCD_Command(0xc3);
LCD_String("Animation");
_delay_ms(500);
lcd_built();
addr=0xc1;
while(1)
{
addr=0xc1;
i=4;
do{ /* showing set 1 left to right rolling */
LCD_Command(addr++);
show_set1();
show_dots(i);
if(i<7)
i++;
else
i=4;
_delay_ms(200);
LCD_Clear();
LCD_Command(addr++);
show_set2();
show_dots(i);
if(i<7)
i++;
else
i=7;
_delay_ms(200);
LCD_Clear();
}while(addr<0xce);
do{ /* showing set 2 right to left rolling */
LCD_Command(addr--);
show_set1();
show_dots(i);
if(i<7)
i++;
else
i=4;
_delay_ms(200);
LCD_Clear();
LCD_Command(addr--);
show_set2();
show_dots(i);
if(i<7)
i++;
else
i=7;
_delay_ms(200);
LCD_Clear();
}while(addr>0xc2);
}
}
void lcd_built(void)
{
unsigned char Character1[8] = { 0x01, 0x03, 0x07, 0x0D, 0x0F, 0x02, 0x05, 0x0A };
unsigned char Character2[8] = { 0x10, 0x18, 0x1C, 0x16, 0x1E, 0x08, 0x14, 0x0A };
unsigned char Character3[8] = { 0x01, 0x03, 0x07, 0x0D, 0x0F, 0x05, 0x08, 0x04 };
unsigned char Character4[8] = { 0x10, 0x18, 0x1C, 0x16, 0x1E, 0x14, 0x02, 0x04 };
unsigned char Character5[8] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x18, 0x18 };
unsigned char Character6[8] = { 0x00, 0x00, 0x00, 0x00, 0x06, 0x06, 0x00, 0x00 };
unsigned char Character7[8] = { 0x00, 0x00, 0x03, 0x03, 0x00, 0x00, 0x00, 0x00 };
unsigned char Character8[8] = { 0x00, 0x18, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00 };
/* ---------- Build Custom Characters -----------------*/
LCD_Custom_Char(0, Character1); /* Build Character1 at position 0 */
LCD_Custom_Char(1, Character2); /* Build Character2 at position 1 */
LCD_Custom_Char(2, Character3); /* Build Character3 at position 2 */
LCD_Custom_Char(3, Character4); /* Build Character4 at position 3 */
LCD_Custom_Char(4, Character5); /* Build Character5 at position 4 */
LCD_Custom_Char(5, Character6); /* Build Character6 at position 5 */
LCD_Custom_Char(6, Character7); /* Build Character6 at position 6 */
LCD_Custom_Char(7, Character8); /* Build Character6 at position 7 */
}
Components Used |
||
---|---|---|
LCD16x2 Display LCD16x2 Display |
X 1 | |
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 |