Overview of DTMF
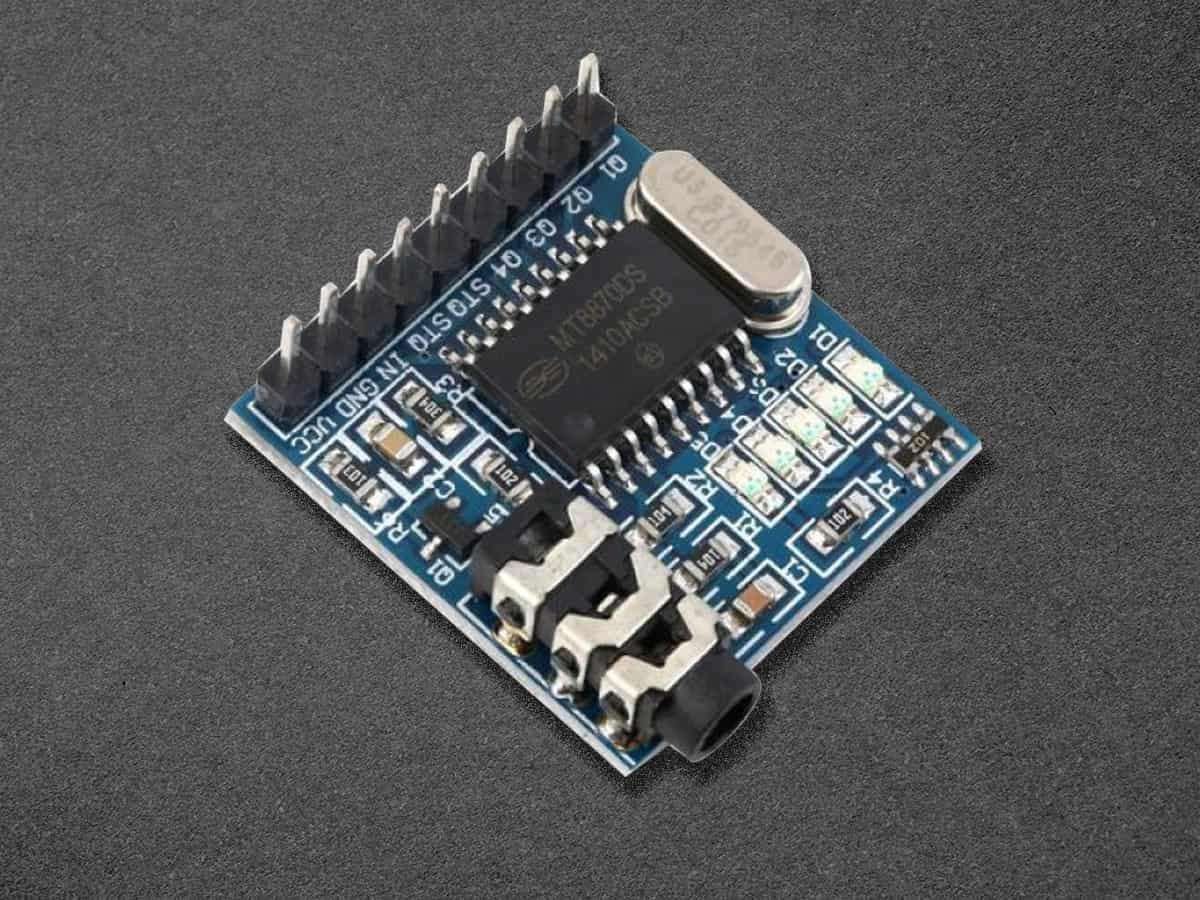
DTMF (Dual Tone Multi-Frequency) is a telecommunication signaling technique that uses a mixture of two pure tones (pure sine waves) sounds. It is used in phones to generate dial tones.
Whenever a key is pressed, a combination of 2 sine waves (one of lower frequency and one of higher frequency) is transmitted. There are 8 different frequencies, 4 from the higher frequency range and 4 from the lower frequency range. This gives us 16 different combinations of lower and higher frequencies to transmit for 16 keys.
MT8870 is a DTMF decoder; it helps decodes the key pressed.
It consists of a band split filter section that helps separate the input signal into a lower frequency and a higher frequency and in turn, helps identify the key pressed.
It gives a 4-bit digital output. This gives 16 possible outputs for 16 different keys.
Microcontroller can read these 4 bits to detect which key has been pressed.
For more information about the MT8870 DTMF decoder and how to use it, refer to the topic MT8870 DTMF Decoder in the sensors and modules section.
Connection Diagram of MT8870 with ATmega16/32
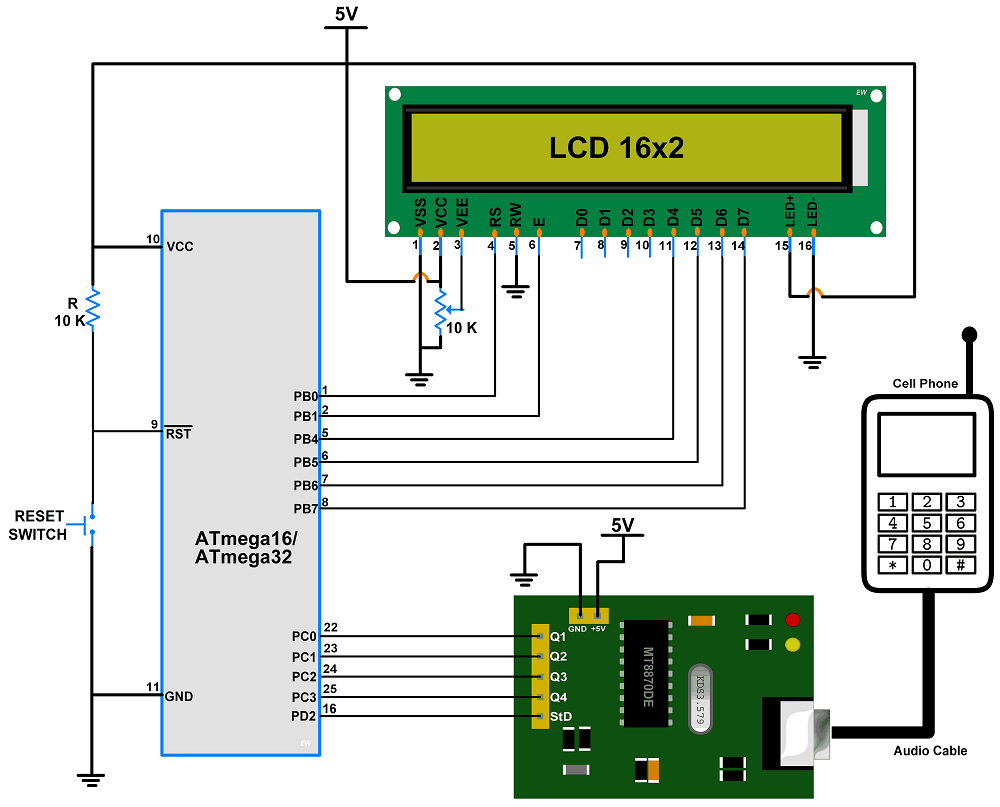
MT8870 DTMF Tone Detection Using ATmega16/32
Here, we are going to interface the MT8870 DTMF receiver module to AVR ATmega16/ATmega32 which will receive key pressed input over a telephone line and display that decoded key on LCD16x2.
MT8870 DTMF Tone Detection Code for ATmega16/32
/*
* ATmega16_DFMF_interfacing.c
*
* http://www.electronicwings.com
*/
#define F_CPU 8000000UL
#include <avr/io.h>
#include <util/delay.h>
#include <avr/interrupt.h>
#include <stdbool.h>
#include "LCD16x2_4bit.h"
bool keydetect = false;
ISR(INT0_vect)
{
keydetect = true;
_delay_ms(50); /* Software debouncing control delay */
}
int main(void)
{
LCD_Init(); /* initialize the LCD */
DDRC = 0x00; /* PORTC define as a input port */
LCD_String("DTMF"); /* write string on LCD */
GICR = 1<<INT0; /* Enable INT0*/
MCUCR = 1<<ISC01 | 1<<ISC00; /* Trigger INT0 on rising edge */
sei(); /* Enable Global Interrupt */
while(1)
{
_delay_ms(5);
if (keydetect)
{
keydetect = false;
switch (PINC & 0x0F)
{
case (0x01):
LCD_String_xy(1, 0, "1");
break;
case (0x02):
LCD_String_xy(1, 0, "2");
break;
case (0x03):
LCD_String_xy(1, 0, "3");
break;
case (0x04):
LCD_String_xy(1, 0, "4");
break;
case (0x05):
LCD_String_xy(1, 0, "5");
break;
case (0x06):
LCD_String_xy(1, 0, "6");
break;
case (0x07):
LCD_String_xy(1, 0, "7");
break;
case (0x08):
LCD_String_xy(1, 0, "8");
break;
case (0x09):
LCD_String_xy(1, 0, "9");
break;
case (0x0A):
LCD_String_xy(1, 0, "0");
break;
case (0x0B):
LCD_String_xy(1, 0, "*");
break;
case (0x0C):
LCD_String_xy(1, 0, "#");
break;
default:
break;
}
}
}
}
Video of DTMF Tone Detection using ATmega16/32 and MT8870
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
MT8870 DTMF Decoder MT8870 is a DTMF (Dual Tone Multi-Frequency) receiver, which decodes the dial tone generated from telephone keypad. It is used in interactive voice response systems (IVRS), Remote control, Credit card systems etc. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 |