Overview of Bluetooth
HC-05 is a Bluetooth device used for wireless communication. It works on serial communication (USART).
- It is a 6 pin module.
- The device can be used in 2 modes; data mode and command mode.
- The data mode is used for data transfer between devices whereas command mode is used for changing the settings of the Bluetooth module.
- AT commands are required in command mode.
- The module works on 5V or 3.3V. It has an onboard 5V to 3.3V regulator.
As the HC-05 Bluetooth module has a 3.3 V level for RX/TX and the microcontroller can detect 3.3 V level, so, no need to shift the transmit level of the HC-05 module. But we need to shift the transmit voltage level from the microcontroller to RX of the HC-05 module.
For more information about the HC-05 Bluetooth module and how to use it, refer to the topic Bluetooth module HC-05 in the sensors and modules section.
For information on USART in AVR ATmega16/ATmega32 and how to use it, refer the topic on USART in AVR ATmega16/ATmega32 in the ATmega basics section.
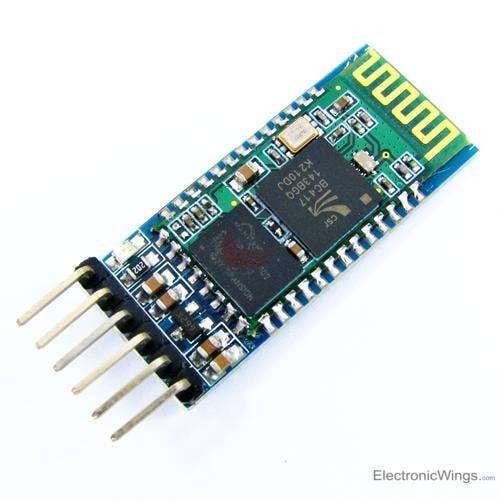
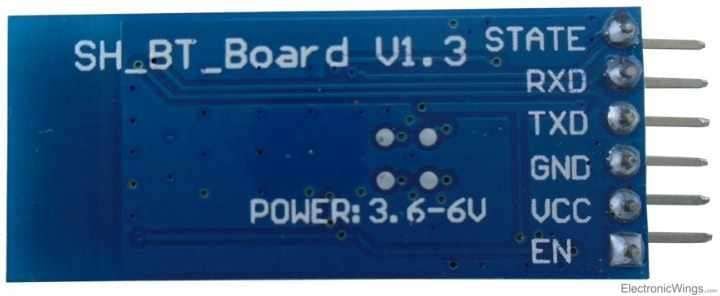
- Key: It is used to bring Bluetooth module in AT commands mode. If key pin is set to high, then this module will work in command mode. Otherwise by default it is in data mode. The default baud rate of HC-05 in command mode is 38400 bps.
- VCC: 5 V or 3.3 V.
- GND: Ground.
- TXD: Transmit Serial data (wirelessly received data by Bluetooth module transmitted out serially on TXD pin)
- RXD: Receive data serially (received data will be transmitted wirelessly by Bluetooth module).
- State: It tells that module is connected or not (here not used).
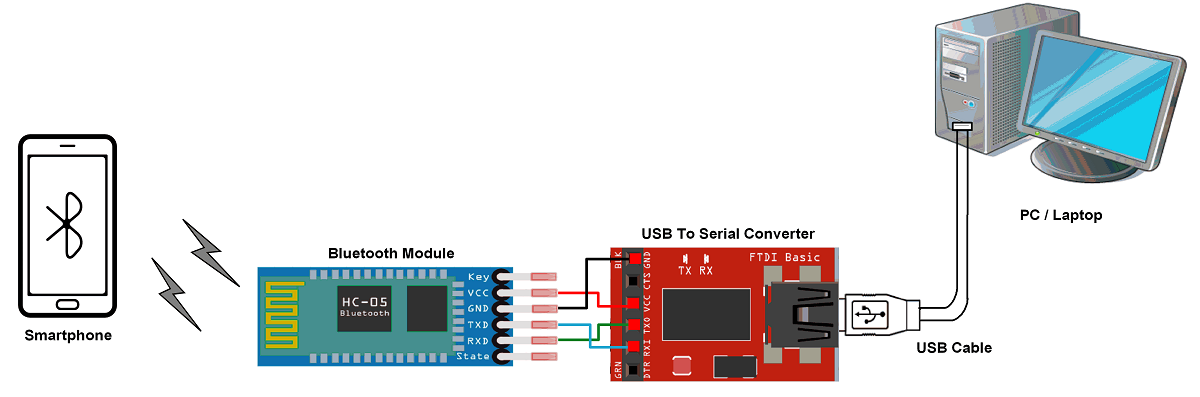
Following are some AT command generally used to change setting, customising of Bluetooth module.
To send these commands, we have to connect HC-05 Bluetooth module to the PC via serial to USB converter and transmit these commands through serial terminal of PC.
However, without changing any settings, we can also use bluetooth module HC-05 with it's default settings.
Command | Description | Response |
AT | Checking communication | OK |
AT+PSWD=XXXX | Set Password e.g. AT+PSWD=4567 | OK |
AT+NAME=XXXX | Set Bluetooth Device Name e.g. AT+NAME=MyHC-05 | OK |
AT+UART=Baud rate, stop bit, parity bit | Change Baud rate e.g. AT+UART=9600,1,0 | OK |
AT+VERSION? | Respond version no. of Bluetooth module | +Version: XX OK e.g. +Version: 2.0 20130107 OK |
AT+ORGL | Send detail of setting done by manufacturer | Parameters: device type, module mode, serial parameter, passkey, etc. |
Connection Diagram of HC-05 Bluetooth Module With ATmega16/32
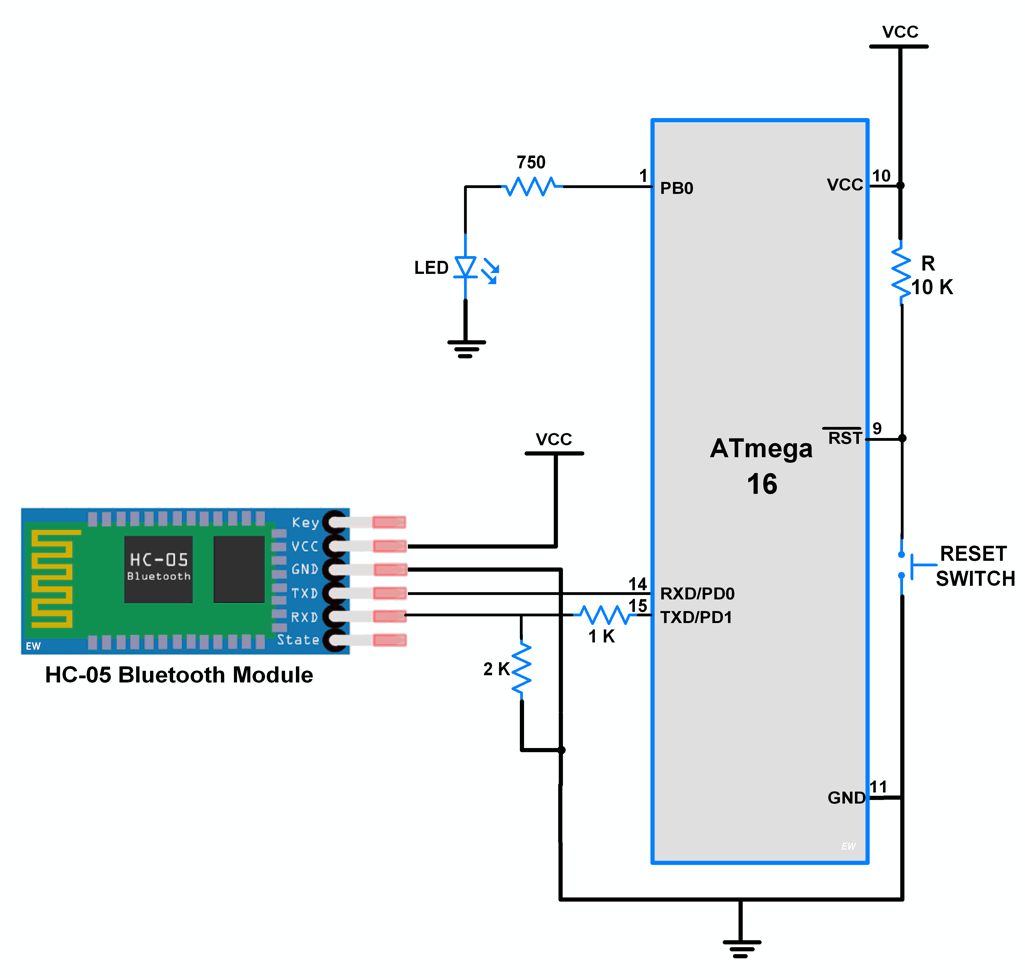
Controle the LED using HC-05 Bluetooth and ATmega16/32
Here let’s develop a small application in which we can control LED ON-OFF through a smartphone.
This is done by interfacing AVR-based ATmega16/ATmega32 with the HC-05 Bluetooth module. Data from HC-05 is received/ transmitted serially by ATmega16/32.
In this application, when 1 is sent from the smartphone, LED will Turn ON and if 2 is sent LED will get Turned OFF. If received data is other than 1 or 2, it will return a message to mobile that select the proper option.
Programming steps
- Initialize ATmega16/ATmega32 USART communication.
- Receive data from the HC-05 Bluetooth module.
- Check whether it is ‘1’ or ‘2’ and take respective controlling action on the LED.
HC-05 Bluetooth Code for ATmega16/32
/*
Bluetooth_Interface with ATmega16 to Control LED via smartphone
http://www.electronicwings.com
*/
#include <avr/io.h>
#include "USART_RS232_H_file.h" /* include USART library */
#define LED PORTB /* connected LED on PORT pin */
int main(void)
{
char Data_in;
DDRB = 0xff; /* make PORT as output port */
USART_Init(9600); /* initialize USART with 9600 baud rate */
LED = 0;
while(1)
{
Data_in = USART_RxChar(); /* receive data from Bluetooth device*/
if(Data_in =='1')
{
LED |= (1<<PB0); /* Turn ON LED */
USART_SendString("LED_ON");/* send status of LED i.e. LED ON */
}
else if(Data_in =='2')
{
LED &= ~(1<<PB0); /* Turn OFF LED */
USART_SendString("LED_OFF"); /* send status of LED i.e. LED OFF */
}
else
USART_SendString("Select proper option"); /* send message for selecting proper option */
}
}
Video of Bluetooth Communication using ATmega16
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
Bluetooth Module HC-05 Bluetooth is a wireless communication protocol used to communicate over short distances. It is used for low power, low cost wireless data transmission applications over 2.4 – 2.485 GHz (unlicensed) frequency band. |
X 1 | |
LED 5mm LED 5mm |
X 1 | |
Breadboard Breadboard |
X 1 |
Downloads |
||
---|---|---|
|
ATMEGA16/32 HC-05 Interfacing Project File | Download |