Overview of LM35
.jpg)
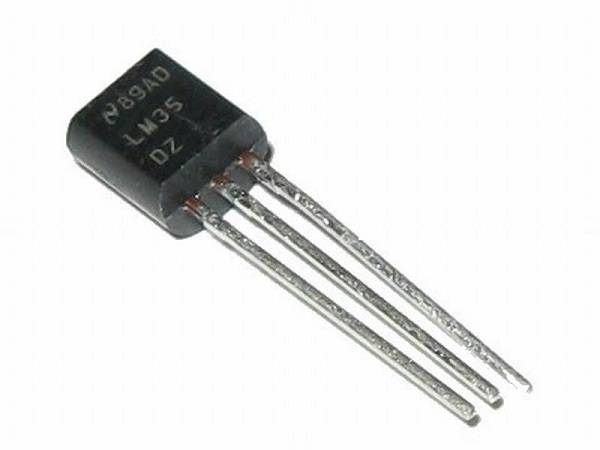
LM35 is a temperature sensor that can measure temperature in the range of -55°C to 150°C.
It is a 3-terminal device that provides an analog voltage proportional to the temperature. The higher the temperature, the higher is the output voltage.
The output analog voltage can be converted to digital form using ADC so that a microcontroller can process it.
For more information about LM35 and how to use it, refer to the topic LM35 Temperature Sensor in the sensors and modules section.
For information about ADC in AVR ATmega16/ATmega32 and how to use it, refer the topic ADC in AVR ATmega16/ATmega32 in the ATmega inside section.
Measure Temperature using LM35 With Atmega16/32 Microcontroller
Let’s interface the LM35 temperature sensor with ATmega16 and display the surrounding temperature on the LCD16x2 display.
LM35 gives output in the analog form so connect out pin of a sensor to one of the ADC channels of ATmega16/ATmega32.
Connection Diagram of LM35 with ATmega16/32
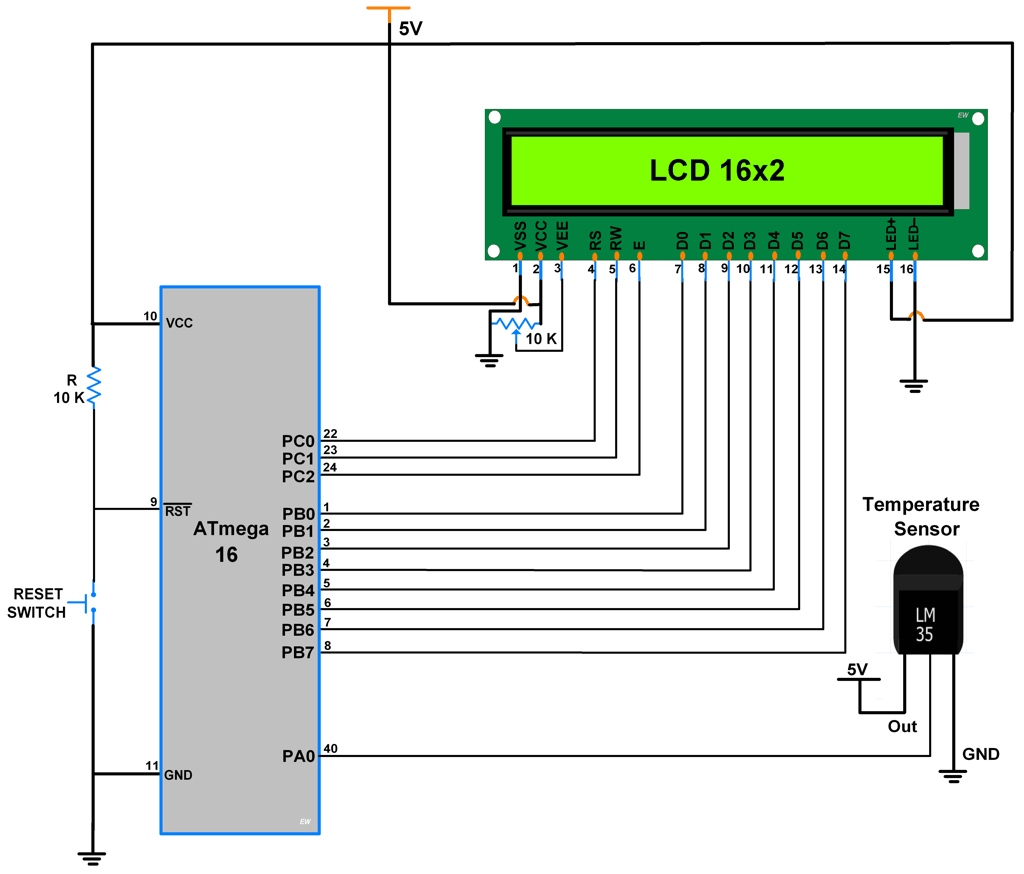
LM35 Code for ATmega16/32
/*
LM35 Interfacing with ATmega16/32
http://www.electronicwings.com
*/
#define F_CPU 8000000UL
#include <avr/io.h>
#include <util/delay.h>
#include <string.h>
#include <stdio.h>
#include "LCD_16x2_H_file.h"
#define degree_sysmbol 0xdf
void ADC_Init(){
DDRA = 0x00; /* Make ADC port as input */
ADCSRA = 0x87; /* Enable ADC, with freq/128 */
ADMUX = 0x40; /* Vref: Avcc, ADC channel: 0 */
}
int ADC_Read(char channel)
{
ADMUX = 0x40 | (channel & 0x07); /* set input channel to read */
ADCSRA |= (1<<ADSC); /* Start ADC conversion */
while (!(ADCSRA & (1<<ADIF))); /* Wait until end of conversion by polling ADC interrupt flag */
ADCSRA |= (1<<ADIF); /* Clear interrupt flag */
_delay_ms(1); /* Wait a little bit */
return ADCW; /* Return ADC word */
}
int main()
{
char Temperature[10];
float celsius;
LCD_Init(); /* initialize 16x2 LCD*/
ADC_Init(); /* initialize ADC*/
while(1)
{
LCD_String_xy(1,0,"Temperature");
celsius = (ADC_Read(0)*4.88);
celsius = (celsius/10.00);
sprintf(Temperature,"%d%cC ", (int)celsius, degree_sysmbol);/* convert integer value to ASCII string */
LCD_String_xy(2,0,Temperature);/* send string data for printing */
_delay_ms(1000);
memset(Temperature,0,10);
}
}
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 | |
LM35 Temperature Sensor LM35 is a sensor which is used to measure temperature. It provides electrical output proportional to the temperature (in Celsius). |
X 1 |