I2C (Inter Integrated Circuit) is a serial bus interface connection protocol. It is also called as TWI (two wire interface) since it uses only two wires for communication. Those two wires are SDA (serial data) and SCL (serial clock).
I2C is acknowledgement-based communication protocol i.e. transmitter waits for acknowledgement from receiver after transmitting data to know whether data is received by receiver successfully.
I2C works in two modes namely,
- Master mode
- Slave mode
SDA (serial data) wire is used for data exchange in between master and slave device. SCL (serial clock) is used for synchronous clock in between master and slave device.
Master device initiates communication with slave device. Master device requires slave device address to initiate conversation with slave device. Slave device responds to master device when it is addressed by master device.
In MBED board has two I2C functionality support on its GPIO pins.
ARM MBED I2C Pins
.png)
Note: Remember that you need a pull-up resistor on SDA and SCL line.
I2C Functions for ARM MBED LPC1768
The basic required function to implement I2C communication are given below,
I2C (sda, scl)
Create an I2C Object first connected to the specified I2C pins.
sda : I2C data line
scl : I2C clock line
e.g.
I2C i2c (p9 , p10)
frequency (int hz)
This function is used to set the I2C clock frequency in Hz.
hz : To set the I2C clock frequency
e.g.
i2c.frequency(1000000);
start (void)
This function is used to start the I2C communication.
e.g.
i2c.start();
stop (void)
This function is used to stop the I2C communication.
e.g.
i2c.stop();
write (int address, const char * data, int length, bool repeated = false)
This function is used to write data to the slave.
address: 8-bit address of slave
data: Pointer to the byte-array data to send
length: Number of bytes to send
repeated: Repeated start, true - do not send stop at end
e.g.
i2c.write(0x05, 0x56, 5, false);
write (int data)
This function is used to write a single byte on I2C bus.
data: write the data you want to send.
Returns
'0' - NAK was received, '1' - ACK was received, '2' - timeout
e.g.
i2c.write(0x05);
read (int address, char *data, int length, bool repeated = false)
This function is used to read data from an I2C slave.
address: 8-bit address of slave
data: Pointer to the byte-array data to send
length: Number of bytes to send
repeated: Repeated start, true - do not send stop at end
Returns
0 on success (ack), non-0 on failure (nack)
e.g.
i2c.read(0x05, 0x56, 5, false);
read (int ack)
This function is used to read a single byte from I2C slave.
ack: indicates if the byte is to be acknowledged (1 = acknowledge)
Returns
the byte read
e.g.
i2c.read();
Example
Let’s build I2C communication between ARM MBED LPC1768 and Arduino. Here, we will send string (data) from ARM MBED (act as Master) to Arduino Uno (act as Slave) and display received data on Arduino Serial window.
To know about Arduino I2C functions you can refer link.
ARM MBED I2C Interfacing Diagram
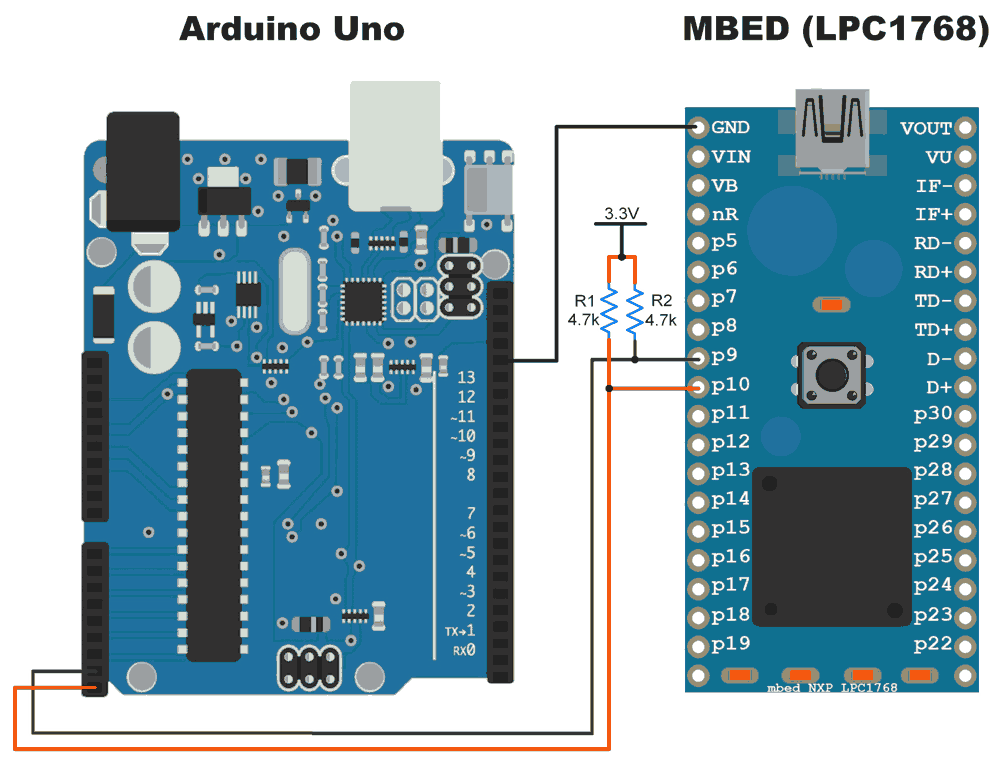
Program
ARM MBED I2C Program (I2C Master)
#include "mbed.h"
I2C i2c(p9 , p10); /* PinName sda, PinName scl */
const int addr8bit = 0x90; /* 8bit I2C address, 0x90 */
const char *cmd = "Hello Slave"; /* Send string "Hello Slave" to slave */
int main() {
while (1) {
i2c.write(addr8bit, cmd, 11); /* address, data, int length */
wait(0.5); /* wait for 500mS */
}
}
Arduino Uno I2C Program (I2C Slave)
#include <Wire.h>
void setup() {
Wire.begin(8); /* join i2c bus with address 8 */
Wire.onReceive(receiveEvent); /* register receive event */
Wire.onRequest(requestEvent); /* register request event */
Serial.begin(9600); /* start serial for debug */
}
void loop() {
delay(100);
}
/* function that executes whenever data is received from master */
void receiveEvent(inthowMany) {
while (0 <Wire.available()) {
char c = Wire.read(); /* receive byte as a character */
Serial.print(c); /* print the character */
}
Serial.println(); /* to newline */
}
/* function that executes whenever data is requested from master */
void requestEvent() {
Wire.write("Hello NodeMCU"); /* send string on request */
}
Output Window
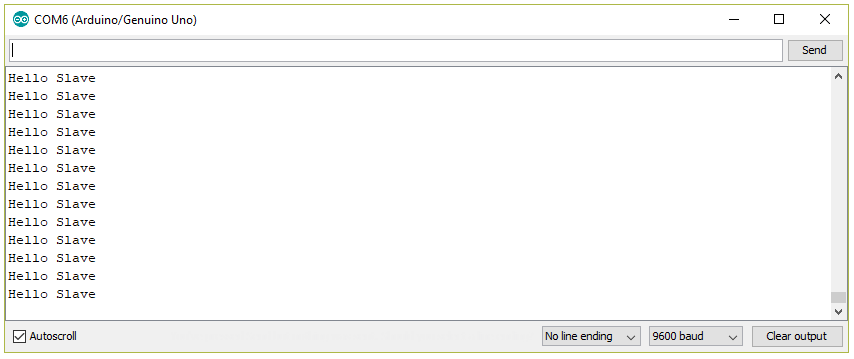
Components Used |
||
---|---|---|
ARM mbed 1768 LPC1768 Board ARM mbed 1768 Board |
X 1 | |
Arduino UNO Arduino UNO |
X 1 |