Overview of GPS
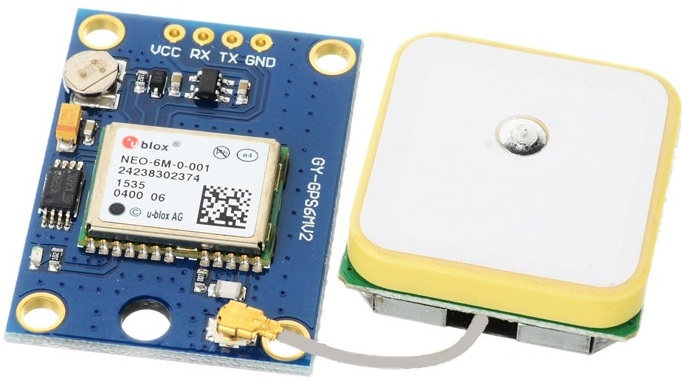
- Global Positioning System (GPS) makes use of signals sent by satellites in space and ground stations on Earth to accurately determine its position on Earth.
- The NEO-6M GPS receiver module uses USART communication to communicate with the microcontroller or PC terminal.
- It receives information like latitude, longitude, altitude, UTC time, etc. from the satellites in the form of an NMEA string. This string needs to be parsed to extract the information that we want to use.
For more information about GPS and how to use it, refer the topic GPS Receiver Module in the sensors and modules section.
Connection Diagram of GPS Module with ARM MBED (LPC1768)
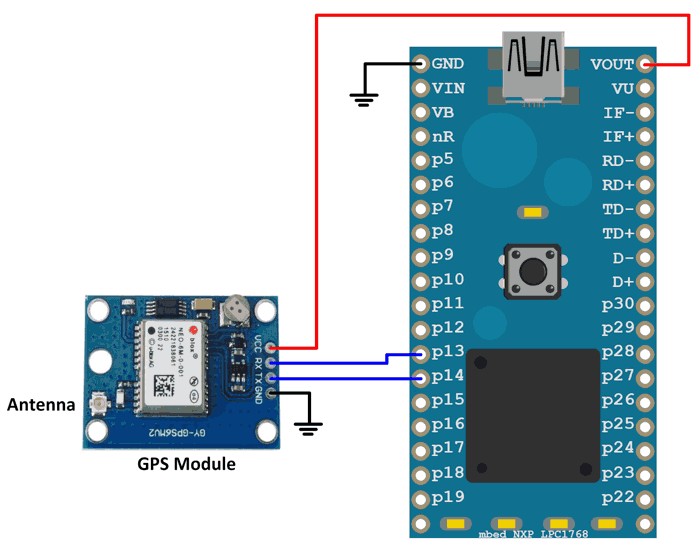
Get all GPS data using GPS Module and ARM MBED LPC1768
We are going to display data (latitude, longitude, altitude, speed, date, number of satellite, and time) received by the GPS receiver module on the serial monitor.
Here, we will be using Edoardo De Marchi’s GPS_U-blox_NEO-6M_Test_Code library and code from MBED.
Download or import this library from here.
Note: The GPS may require some time to lock on to satellites. Give it around 20-30 seconds so that it can start giving you correct data. It usually takes no more than 5 seconds to lock on to satellites if you are in an open space; but occasionally it may take more time (for example if 3 or more satellites are not visible to the GPS receiver).
GPS Code for ARM MBED (LPC1768)
/*
* Author: Edoardo De Marchi
* Date: 22-08-14
* Notes: Firmware for GPS U-Blox NEO-6M
*/
#include "main.h"
void Init()
{
gps.baud(9600);
pc.baud(115200);
pc.printf("Init OK\n");
}
int main()
{
Init();
char c;
while(true)
{
if(gps.readable())
{
if(gps.getc() == '$'); // wait a $
{
for(int i=0; i<sizeof(cDataBuffer); i++)
{
c = gps.getc();
if( c == '\r' )
{
//pc.printf("%s\n", cDataBuffer);
parse(cDataBuffer, i);
i = sizeof(cDataBuffer);
}
else
{
cDataBuffer[i] = c;
}
}
}
}
}
}
void parse(char *cmd, int n)
{
char ns, ew, tf, status;
int fq, nst, fix, date; // fix quality, Number of satellites being tracked, 3D fix
float latitude, longitude, timefix, speed, altitude;
// Global Positioning System Fix Data
if(strncmp(cmd,"$GPGGA", 6) == 0)
{
sscanf(cmd, "$GPGGA,%f,%f,%c,%f,%c,%d,%d,%*f,%f", &timefix, &latitude, &ns, &longitude, &ew, &fq, &nst, &altitude);
pc.printf("GPGGA Fix taken at: %f, Latitude: %f %c, Longitude: %f %c, Fix quality: %d, Number of sat: %d, Altitude: %f M\n", timefix, latitude, ns, longitude, ew, fq, nst, altitude);
}
// Satellite status
if(strncmp(cmd,"$GPGSA", 6) == 0)
{
sscanf(cmd, "$GPGSA,%c,%d,%d", &tf, &fix, &nst);
pc.printf("GPGSA Type fix: %c, 3D fix: %d, number of sat: %d\r\n", tf, fix, nst);
}
// Geographic position, Latitude and Longitude
if(strncmp(cmd,"$GPGLL", 6) == 0)
{
sscanf(cmd, "$GPGLL,%f,%c,%f,%c,%f", &latitude, &ns, &longitude, &ew, &timefix);
pc.printf("GPGLL Latitude: %f %c, Longitude: %f %c, Fix taken at: %f\n", latitude, ns, longitude, ew, timefix);
}
// Geographic position, Latitude and Longitude
if(strncmp(cmd,"$GPRMC", 6) == 0)
{
sscanf(cmd, "$GPRMC,%f,%c,%f,%c,%f,%c,%f,,%d", &timefix, &status, &latitude, &ns, &longitude, &ew, &speed, &date);
pc.printf("GPRMC Fix taken at: %f, Status: %c, Latitude: %f %c, Longitude: %f %c, Speed: %f, Date: %d\n", timefix, status, latitude, ns, longitude, ew, speed, date);
}
}
Output of Serial Monitor
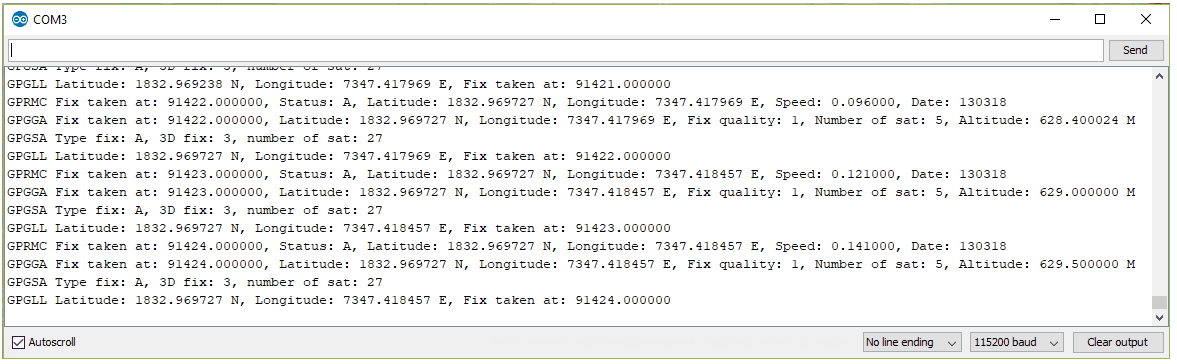
Components Used |
||
---|---|---|
ARM mbed 1768 LPC1768 Board ARM mbed 1768 Board |
X 1 | |
Ublox NEO-6m GPS Ublox Neo 6m GPS |
X 1 |