ADC (Analog to Digital converter) is most widely used device in embedded systems which is designed especially to convert analog signal into digital signal. ARM MBED LPC1768 has in-built 12-bit ADC.
MBED LPC1768 has on-board 6 ADC channels (analog inputs) i.e. p15 – p20 which is shown in below image,
ARM MBED ADC Pins
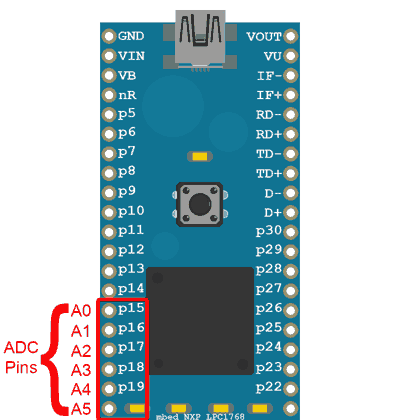
Note: p18 pin can be used as analog output too.
In the MBED board, there are total 12-bit 6 Analog Input available from p15 to p20 and 1 Analog output pin is available on p18. The function for analog input is as follows.
Analog Input:
AnalogIN (pin)
Create an AnalogIN Object for pin that we are using as analog input.
pin: give the pin name which we read as an input (from p15 to p20).
e.g.
AnalogIn sensor_one(p15)
ADC.read()
This statement is used for to read the analog input voltage in the float value from 0.0 to 1.0 volt.
e.g.
sensor_one.read();
ADC.read_u16()
This statement is used for to read the analog input voltage in the unsigned value from 0x0 to 0xFFFF.
e.g.
sensor_one.read_u16();
Note:
AnalogIn()
reads the voltage as a fraction of the system voltage The value is a floating point from 0.0 (VSS) to 1.0 (VCC). For example, if you have a 3.3V system and the applied voltage is 1.65V, then AnalogIn() reads 0.5 as the value.
Interfacing Diagram
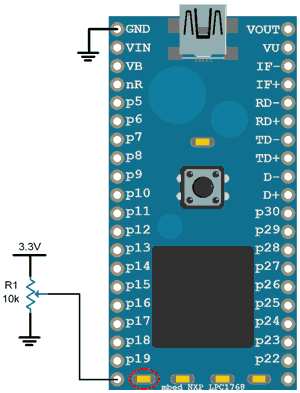
Connect a potentiometer to the p20 pin of ARM mbed board as shown in above figure and monitor the on-board LED1.
Example
- ADC read’s the changeable potentiometer values and comparing it, as well as print these values on serial monitor.
- When ADC value is greater than 0.3 volt then LED1 will turn ON.
- And when ADC value is less than 0.3 volt then LED1 will turn OFF.
Program
#include "mbed.h"
// Initialize a pins to perform analog input and digital output functions
AnalogIn ain(A5);
DigitalOut dout(LED1);
int main(void)
{
while (1) {
// test the voltage on the initialized analog pin
// and if greater than 0.3 * VCC set the digital pin
// to a logic 1 otherwise a logic 0
if(ain > 0.3f) {
dout = 1;
} else {
dout = 0;
}
// print the percentage and 16 bit normalized values
printf("percentage: %3.3f%%\n", ain.read()*100.0f);
printf("normalized: 0x%04X \n", ain.read_u16());
wait(0.2f);
}
}
Components Used |
||
---|---|---|
ARM mbed 1768 LPC1768 Board ARM mbed 1768 Board |
X 1 |