Overview of Nokia5110 Display
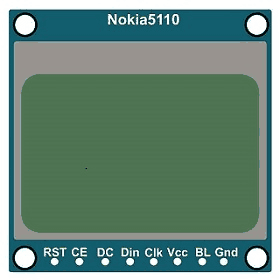
- Nokia5110 is a graphical display that can display text, images, and various patterns.
- It has a resolution of 48x84 and comes with a backlight.
- It uses SPI communication to communicate with a microcontroller.
- Data and commands can be sent through the microcontroller to the display to control the display output.
- It has 8 pins.
For more information about the Nokia5110 display and how to use it, refer the topic Nokia5110 Graphical Display in the sensors and modules section.
Connection Diagram of Nokia5110 with ARM MBED (LPC1768)
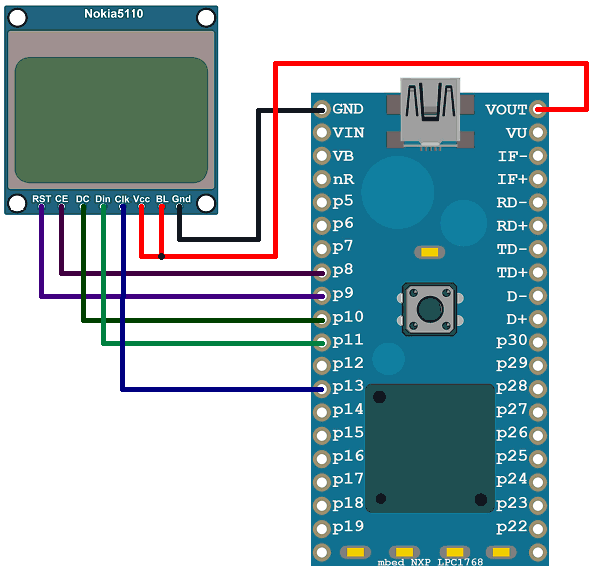
Example
Here, we display the NXP logo and Tesla logo on Nokia 5110 GLCD.
Nokia5110 Code for ARM MBED (LPC1768)
// Project: Nokia5110 - Controlling a NK5110 display from an NXP LPC1768
// File: main.cpp
// Author: Chris Yan
// Created: January, 2012
// Revised:
// Desc: A basic LCD output test which uses the NXP LPC1768's SPI interface to
// display pixels, characters, and numbers on the Nokia 5110 LCD.
// Created using a sparkfun breakout board with integrated Phillips 8544 driver
// for 48x84 LCDs.
//https://os.mbed.com/users/Fuzball/code/Nokia5110/file/41063eb2a040/main.cpp/
#include "mbed.h"
#include "NOKIA_5110.h"
#include "NXP_logo.h"
#include "Tesla_Logo.h"
void N5110_image(const unsigned char *image_data, NokiaLcd* image)
{
for (int k=0; k<=503; k++)
{
image->SendDrawData(image_data[k]);
}
}
int main()
{
// Init the data structures and NokiaLcd class
LcdPins myPins;
myPins.sce = p8;
myPins.rst = p9;
myPins.dc = p10;
myPins.mosi = p11;
myPins.miso = NC;
myPins.sclk = p13;
NokiaLcd myLcd( myPins );
// Start the LCD
myLcd.InitLcd();
// Draw a test pattern on the LCD and stall for 15 seconds
myLcd.TestLcd( 0xAA );
wait( 1 );
myLcd.ClearLcdMem();
myLcd.SetXY(0,10);
myLcd.DrawString("Welcome");
wait( 1 );
myLcd.ClearLcdMem();
while( 1 ) {
myLcd.SetXY(0,0);
N5110_image(NXP_logo, &myLcd);
wait(2);
myLcd.ClearLcdMem();
myLcd.SetXY(0,0);
N5110_image(Tesla_Logo, &myLcd);
wait(2);
myLcd.ClearLcdMem();
}
}
Components Used |
||
---|---|---|
ARM mbed 1768 LPC1768 Board ARM mbed 1768 Board |
X 1 | |
Nokia5110 Graphical Display Nokia5110 is 48x84 dot LCD display with Serial Peripheral Interface (SPI) Connectivity. It was designed for cell phones. It is also used in embedded applications. |
X 1 |