Introduction to ADC
- When we interface sensors to the microcontroller, the output of the sensor many of the times is analog in nature. But the microcontroller processes digital signals.
- Hence, we use ADC in between the sensor and microcontroller. It converts an analog signal into a digital and gives it to the microcontroller.
- There are many applications of ADC like in a biometric application, Environment monitoring, Gas leakage detection etc.
Arduino Uno has 6 0n-board ADC channels which can be used to read analog signal in the range 0-5V.
It has 10-bit ADC means it will give digital value in the range of 0 – 1023 (2^10). This is called as a resolution which indicates the number of discrete values it can produce over the range of analog values.
Digital Output value Calculation
ADC Resolution = Vref / ((2^n) - 1)
Digital Output = Vin / Resolution
Where,
Vref - The reference voltage is the maximum value that the ADC can convert.
To keep things simple, let us consider that Vref is 5V,
For 0 Vin, digital o/p value = 0
For 5 Vin, digital o/p value = 1023 (10-bit)
For 2.5 Vin, digital o/p value = 512 (10-bit)
ADC Pins of Arduino Uno
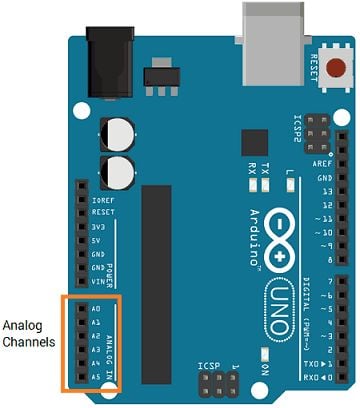
Analog Functions for Arduino ADC
analogRead (pin)
This function is used to read analog value from specified analog pin.
pin
- number of analog pin which we want to read
returns
- digital value 0 – 1023
e.g. analogRead(A0) //read analog value at A0 channel
analogReference (type)
This function is used for configuring the reference voltage used for analog input.
let's see How to Read Analog values using Arduino
Let’s write a program to read varying analog value generated using potentiometer which is connected to A0 analog channel. Display the digital value on Serial monitor which we got from the Arduino ADC.
Potentiometer Interfacing with Arduino Uno
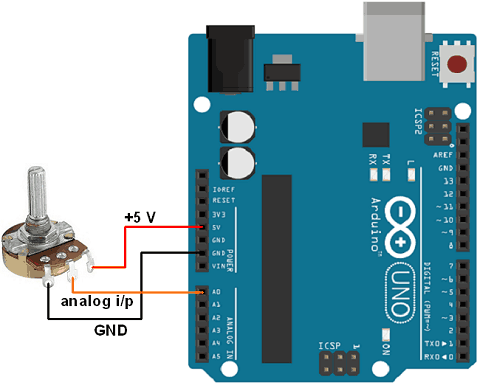
Arduino Code for reading analog value
int sensorPin = A0; // input pin for the potentiometer
int digitalValue = 0;// variable to store the value coming from the sensor
void setup() {
Serial.begin(9600);
}
void loop() {
digitalValue = analogRead(sensorPin);// read the value from the analog channel
Serial.print("digital value = ");
Serial.println(digitalValue); //print digital value on serial monitor
delay(1000);
}
Output on Serial Monitor
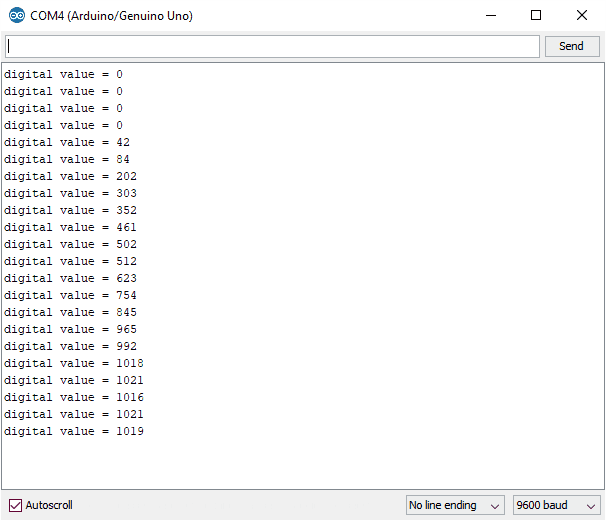
Note: If nothing is connected to analog input channel then the analogRead () function return the noisy fluctuating value.
Read Analog Voltage using Arduino Uno
As ADC provide digital output which is proportional to analog value. To know what is input analog value, we need to convert this digital value back to analog value through program. To convert this digital value to analog input voltage,
Aout = digital value * (Vref/2^n – 1)
e.g. digital value = 512 and ADC is 10-bit with 5V Vref. But, we want to know that for what analog voltage it is giving respective digital value. Then,
Aout = 512 * (5 V / 1023)
= 2.5 V
Code for Read Analog Voltage using Arduino
int sensorPin = A0; // select the input pin for the potentiometer
int digitalValue = 0; // variable to store the value coming from the sensor
float analogVoltage = 0.00;
void setup() {
Serial.begin(9600);
}
void loop() {
digitalValue = analogRead(sensorPin);// read the value from the analog channel
Serial.print("digital value = ");
Serial.print(digitalValue); //print digital value on serial monitor
//convert digital value to analog voltage
analogVoltage = (digitalValue * 5.00)/1023.00;
Serial.print(" analog voltage = ");
Serial.println(analogVoltage);
delay(1000);
}
Output on Serial Window
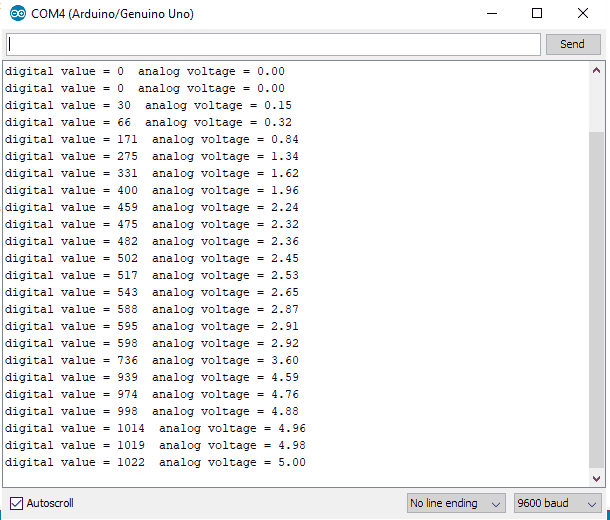
For more implementation, you can visit
Components Used |
||
---|---|---|
Arduino UNO Arduino UNO |
X 1 | |
Arduino Nano Arduino Nano |
X 1 |