Overview of Micro SD Card
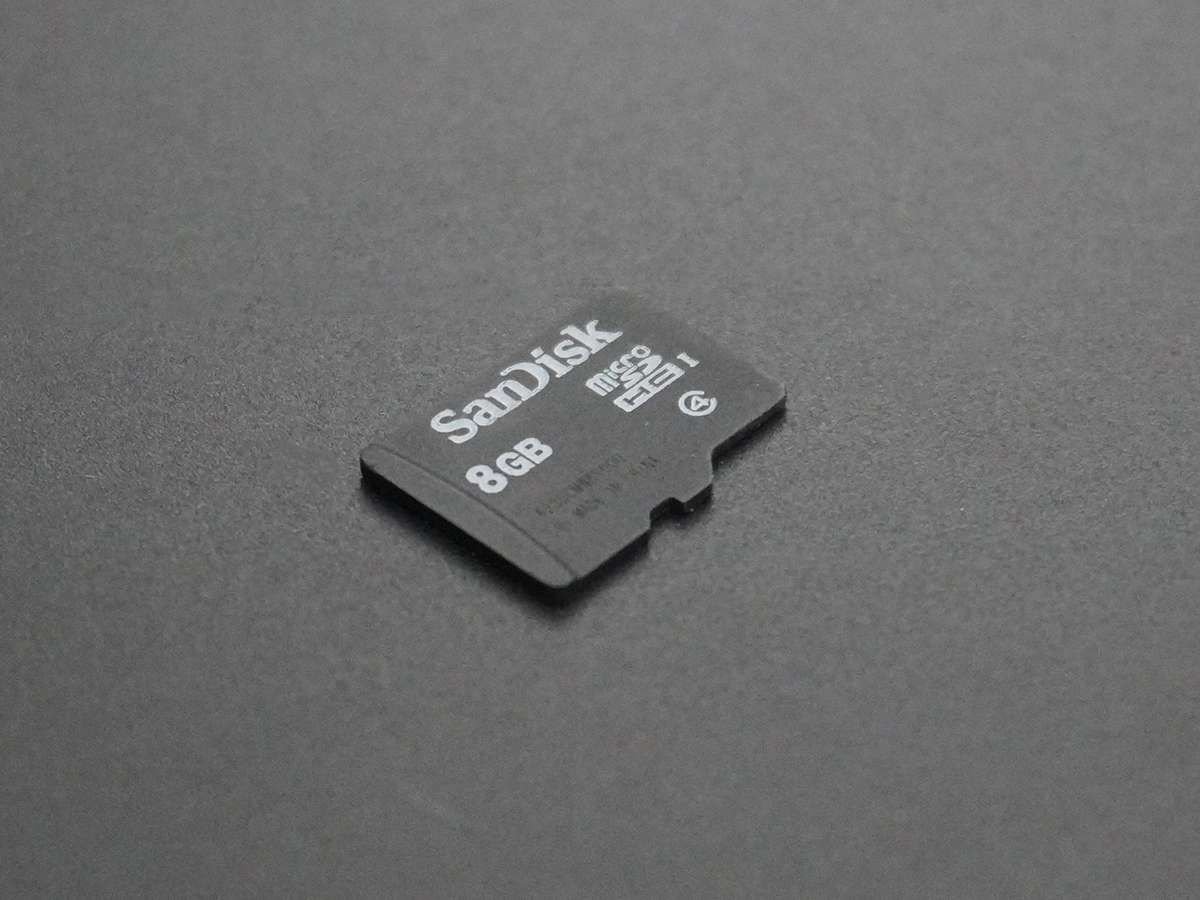
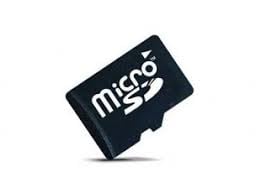
Secure Digital (SD) is a non-volatile memory card format developed by the SD Card Association (SDA) for use in portable devices like mobile phones, cameras etc. Secure Digital includes four card families as follows-
1. SDSC: Standard Capacity SD. It has storage capacity of 2GB uses FAT- 12 and FAT-16 file systems.
2. SDHC: High Capacity SD. It has storage capacity ranging from 2GB to 32GB uses FAT-32 file system.
3. SDXC: eXtended Capacity SD. It has storage capacity ranging from 32GB to 2TB uses exFAT file system.
4. SDIO: It combines INPUT/OUTPUT functions with data storage.
SD cards come in different sizes-
- Regular SD - 1.25 by 0.95 inches
- Mini SD - 0.87 by 0.79 inches
- Micro SD - 0.43 by 0.59 inches
Here we use the micro SD card for interfacing.
File System
SD card is a block-addressable storage device, in which the host device can read or write fixed-size blocks by specifying their block number. Most SD cards are preformatted with one or more MBR (Master Boot Record) partitions in which the first partition contains the file system which enables it to work like hard disks.
1. For SDSC card
- Capacity smaller than 16 MB- FAT 12
- Capacity between 16 MB and 32 MB- FAT 16
- Capacity larger than 32 MB- FAT 16B
2. For SDHC cards
- Capacity smaller than 7.8 GB- FAT 32
- Capacity greater than 7.8 GB- FAT 32
3. For SDXC cards
- exFAT
Notes
1. Arduino library supports only FAT16 and FAT32 file systems. Make sure that your SD card is formatted with these two only otherwise you will get an initialization error.
2. For formatting your SD card you can download SD formatter on following link - https://www.sdcard.org/downloads/formatter_4/
Micro SD Card Module
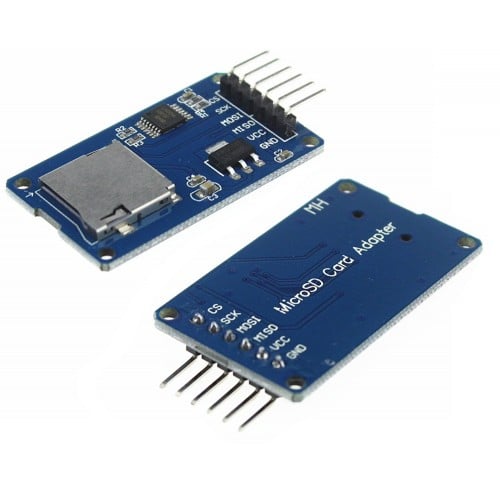
The micro- SD Card Module is a simple solution for transferring data to and from a standard SD card. This module has SPI interface which is compatible with any SD card and it uses 5V or 3.3V power supply which is compatible with Arduino UNO/Mega.
MicroSD Pinout
Pin Name | Description |
CS | Chip Select |
SCK | Clock |
MISO | Master In Slave Out |
MOSI | Master Out Slave In |
Connection Diagram of MicroSD with Arduino
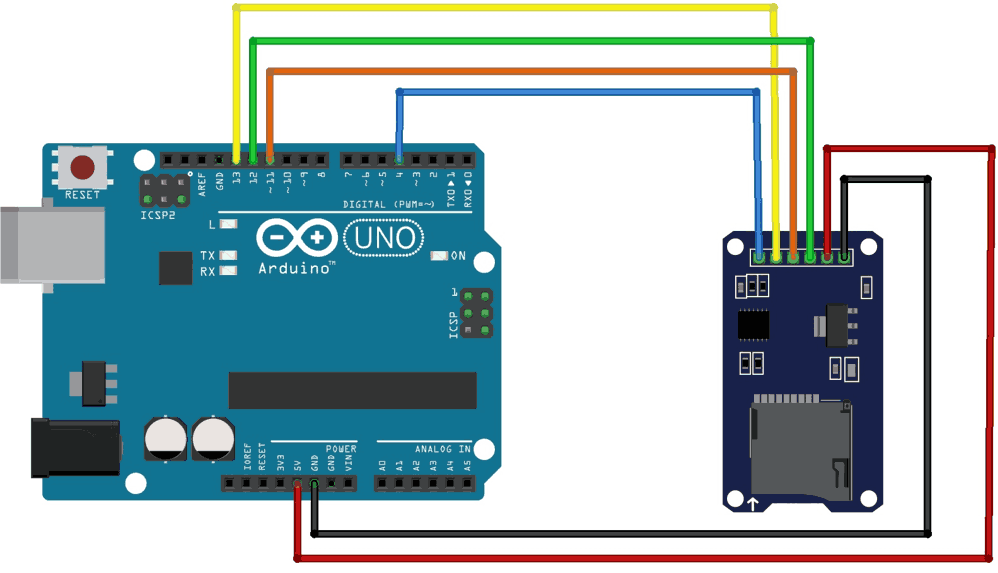
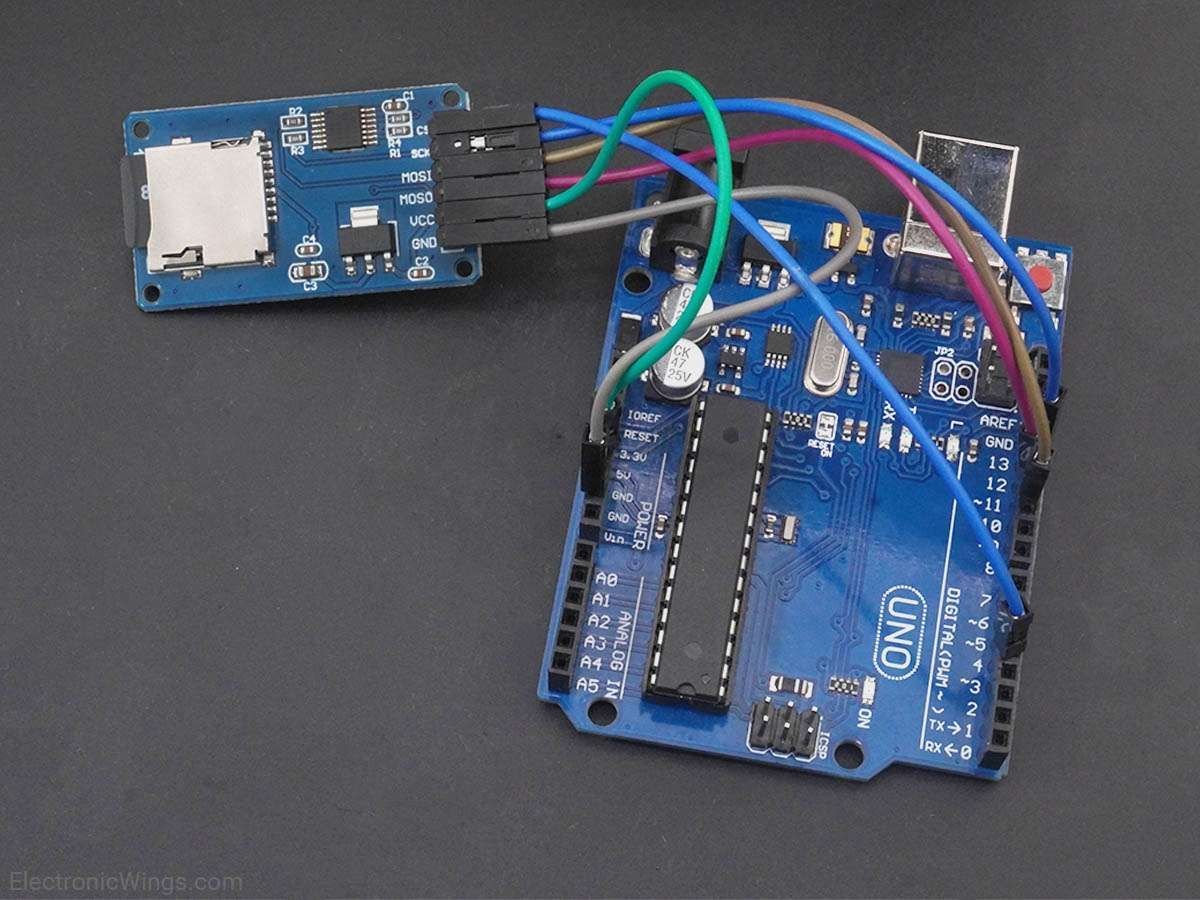
The diagram shows the detail connections of SD module with Arduino.
- MOSI - pin 11
- MISO - pin 12
- CLK - pin 13
- CS - pin 4
Note You can connect VCC of the module to either 5v or 3.3v.
Read and Write on SD Card using Arduino
We are going to interface the micro SD card with Arduino and perform the read-write operation on it.
Here we will be using the inbuilt code provided by Arduino IDE.
The code in the Arduino IDE uses the two library files –
- SPI.h
- SD.h
These library files direct contain function definitions which are used for SD card read-write operations.
For accessing code in Arduino IDE you can follow the following mention steps-
Open IDE -> Click on Files -> Click on examples -> Select SD -> Open ReadWrite
For your simplicity, we have provided the same code over here.
Micro SD Card Read and Write Code using Arduino
#include <SPI.h>
#include <SD.h>
File myFile;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
Serial.print("Initializing SD card...");
if (!SD.begin(4)) {
Serial.println("initialization failed!");
return;
}
Serial.println("initialization done.");
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
myFile = SD.open("test.txt", FILE_WRITE);
// if the file opened okay, write to it:
if (myFile) {
Serial.print("Writing to test.txt...");
myFile.println("testing 1, 2, 3.");
// close the file:
myFile.close();
Serial.println("done.");
} else {
// if the file didn't open, print an error:
Serial.println("error opening test.txt");
}
// re-open the file for reading:
myFile = SD.open("test.txt");
if (myFile) {
Serial.println("test.txt:");
// read from the file until there's nothing else in it:
while (myFile.available()) {
Serial.write(myFile.read());
}
// close the file:
myFile.close();
} else {
// if the file didn't open, print an error:
Serial.println("error opening test.txt");
}
}
void loop() {
// nothing happens after setup
}
Components Used |
||
---|---|---|
Arduino UNO Arduino UNO |
X 1 | |
Arduino Nano Arduino Nano |
X 1 | |
SD Card SD (Secure Digital) is a non-volatile memory card format developed by the SD Card Association (SDA) for use in portable devices. |
X 1 | |
SD Card Module SD Card |
X 1 |