LCD 16x2
LCDs (Liquid Crystal Displays) are used for displaying status or parameters in embedded systems.
LCD 16x2 is 16 pin device that has 8 data pins (D0-D7) and 3 control pins (RS, RW, EN). The remaining 5 pins are for the supply and the backlight for the LCD.
The control pins help us configure the LCD in command mode or data mode. They also help configure read mode or write mode and also when to read or write.
LCD 16x2 can be used in 4-bit mode or 8-bit mode depending on the requirement of the application. In order to use it, we need to send certain commands to the LCD in command mode and once the LCD is configured according to our needs, we can send the required data in data mode.
For more information about LCD 16x2 and how to use it, refer to the topic LCD 16x2 display module in the sensors and modules section.
LCD16x2 Pinout
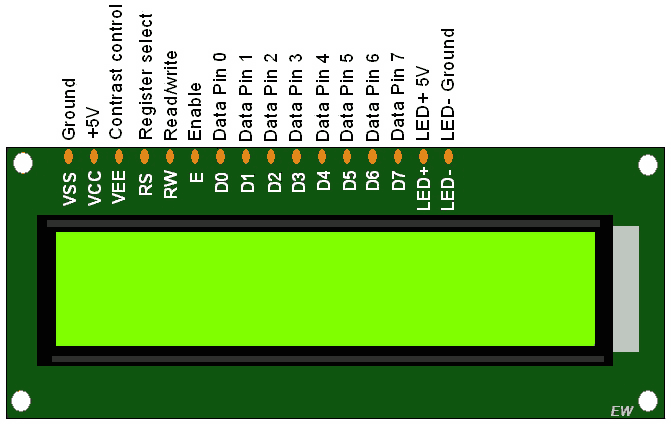
LCD 16x2 4-bit Mode
- In 4-bit mode, data/command is sent in a 4-bit (nibble) format.
- To do this 1st send a Higher 4-bit and then send a lower 4-bit of data/command.
- Only 4 data (D4 - D7) pins of 16x2 of LCD are connected to the microcontroller and other control pins RS (Register select), RW (Read/write), and E (Enable) is connected to other GPIO Pins of the controller.
- Therefore, due to such connections, we can save four GPIO pins which can be used for another application.
LCD16x2 4-bit mode connection with 8051
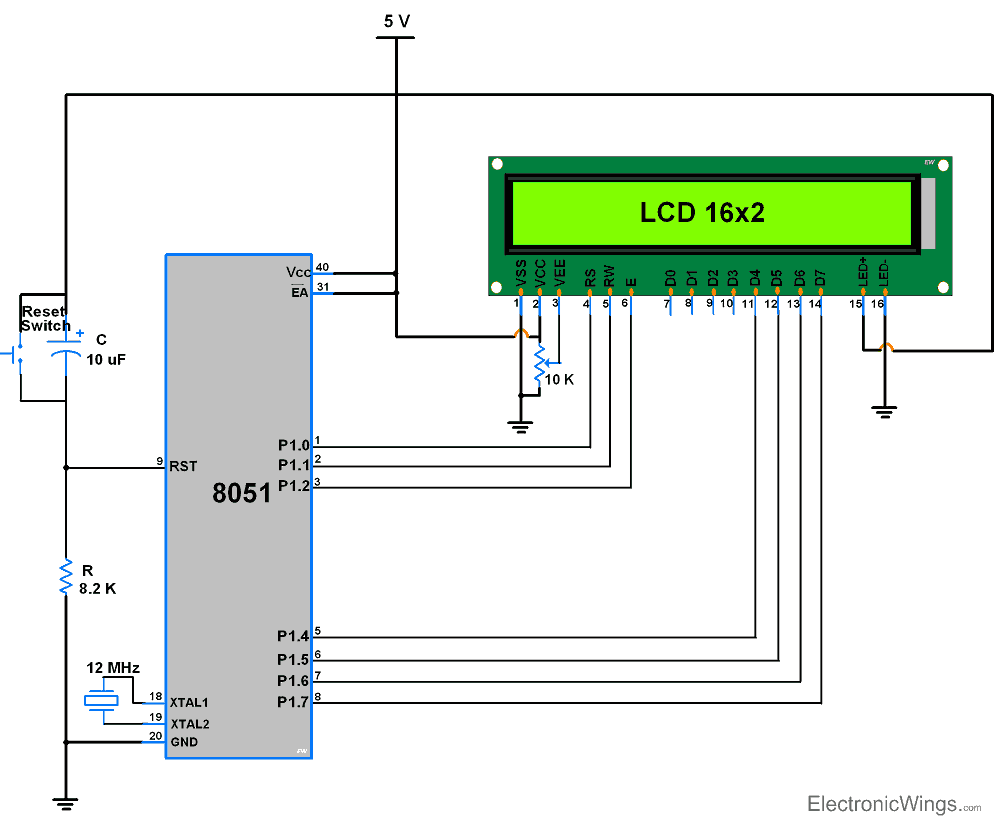
Programming LCD16x2 4-bit mode
Initialization
- Wait for 15ms, Power-on initialization time for LCD16x2.
- Send 0x02 command which initializes LCD 16x2 in 4-bit mode.
- Send 0x28 command which configures LCD in 2-line, 4-bit mode, and 5x8 dots.
- Send any Display ON command (0x0E, 0x0C)
- Send 0x06 command (increment cursor)
void LCD_Init (void) /* LCD Initialize function */
{
delay(20); /* LCD Power ON Initialization time >15ms */
LCD_Command (0x02); /* 4bit mode */
LCD_Command (0x28); /* Initialization of 16X2 LCD in 4bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* Clear display */
LCD_Command (0x80); /* Cursor at home position */
}
Now we successfully initialized LCD & it is ready to accept data in 4-bit mode to display.
To send command/data to 16x2 LCD we have to send a higher nibble followed by a lower nibble. As 16x2 LCD’s D4 - D7 pins are connected as data pins, we have to shift the lower nibble to the right by 4 before transmitting.
LCD 16x2 4bit mode Command write function
- First, send a Higher nibble of command.
- Make RS pin low, RS=0 (command reg.)
- Make RW pin low, RW=0 (write operation) or connect it to ground.
- Give High to Low pulse at Enable (E).
- Send lower nibble of command.
- Give High to Low pulse at Enable (E).
void LCD_Command (char cmnd) /* LCD16x2 command funtion */
{
LCD_Port =(LCD_Port & 0x0F) | (cmnd & 0xF0);/* Send upper nibble */
rs=0; /* Command reg. */
rw=0; /* Write operation */
en=1;
delay(1);
en=0;
delay(2);
LCD_Port = (LCD_Port & 0x0F) | (cmnd << 4);/* Send lower nibble */
en=1; /* Enable pulse */
delay(1);
en=0;
delay(5);
}
Data write function for LCD 16x2 4bit mode
- First, send a Higher nibble of data.
- Make RS pin high, RS=1 (data reg.)
- Make RW pin low, RW=0 (write operation) or connect it to ground.
- Give High to Low pulse at Enable (E).
- Send lower nibble of data.
- Give High to Low pulse at Enable (E).
void LCD_Char (char char_data) /* LCD data write function */
{
LCD_Port =(LCD_Port & 0x0F) | (char_data & 0xF0);/* Send upper nibble */
rs=1; /* Data reg.*/
rw=0; /* Write operation*/
en=1;
delay(1);
en=0;
delay(2);
LCD_Port = (LCD_Port & 0x0F) | (char_data << 4);/* Send lower nibble */
en=1; /* Enable pulse */
delay(1);
en=0;
delay(5);
}
Code 8051 LCD16x2 4 bit mode
/*
LCD16x2 4 bit 8051 interface
http://www.electronicwings.com
*/
#include<reg51.h>
sfr LCD_Port=0x90; /* P1 port as data port */
sbit rs=P1^0; /* Register select pin */
sbit rw=P1^1; /* Read/Write pin */
sbit en=P1^2; /* Enable pin */
/* Function to provide delay Approx 1ms with 11.0592 Mhz crystal*/
void delay(unsigned int count)
{
int i,j;
for(i=0;i<count;i++)
for(j=0;j<112;j++);
}
void LCD_Command (char cmnd) /* LCD16x2 command funtion */
{
LCD_Port =(LCD_Port & 0x0F) | (cmnd & 0xF0);/* Send upper nibble */
rs=0; /* Command reg. */
rw=0; /* Write operation */
en=1;
delay(1);
en=0;
delay(2);
LCD_Port = (LCD_Port & 0x0F) | (cmnd << 4);/* Send lower nibble */
en=1; /* Enable pulse */
delay(1);
en=0;
delay(5);
}
void LCD_Char (char char_data) /* LCD data write function */
{
LCD_Port =(LCD_Port & 0x0F) | (char_data & 0xF0);/* Send upper nibble */
rs=1; /*Data reg.*/
rw=0; /*Write operation*/
en=1;
delay(1);
en=0;
delay(2);
LCD_Port = (LCD_Port & 0x0F) | (char_data << 4);/* Send lower nibble */
en=1; /* Enable pulse */
delay(1);
en=0;
delay(5);
}
void LCD_String (char *str) /* Send string to LCD function */
{
int i;
for(i=0;str[i]!=0;i++) /* Send each char of string till the NULL */
{
LCD_Char (str[i]); /* Call LCD data write */
}
}
void LCD_String_xy (char row, char pos, char *str) /* Send string to LCD function */
{
if (row == 0)
LCD_Command((pos & 0x0F)|0x80);
else if (row == 1)
LCD_Command((pos & 0x0F)|0xC0);
LCD_String(str); /* Call LCD string function */
}
void LCD_Init (void) /* LCD Initialize function */
{
delay(20); /* LCD Power ON Initialization time >15ms */
LCD_Command (0x02); /* 4bit mode */
LCD_Command (0x28); /* Initialization of 16X2 LCD in 4bit mode */
LCD_Command (0x0C); /* Display ON Cursor OFF */
LCD_Command (0x06); /* Auto Increment cursor */
LCD_Command (0x01); /* clear display */
LCD_Command (0x80); /* cursor at home position */
}
void main()
{
LCD_Init(); /* Initialization of LCD*/
LCD_String("ElectronicWINGS"); /* write string on 1st line of LCD*/
LCD_Command(0xC0); /* Go to 2nd line*/
LCD_String_xy(1,0,"Hello World"); /*write string on 2nd line*/
while(1); /* Infinite loop. */
}
LCD 16x2 4bit mode output with 8051
.jpg)
Components Used |
||
---|---|---|
LCD16x2 Display LCD16x2 Display |
X 1 | |
8051 AT89c51 8051 AT89c51 |
X 1 |