Overview of DHT11
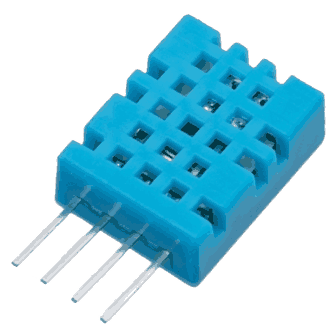
The DHT11 sensor measures and provides humidity and temperature values serially over a single wire.
It can measure the relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
It has 4 pins; one of which is used for data communication in serial form.
Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of the frame.
For more information about the DHT11 sensor and how to use it, refer to the topic DHT11 sensor in the sensors and modules topic.
Connection Diagram of DHT11 with MSP-EXP430G2 TI Launchpad
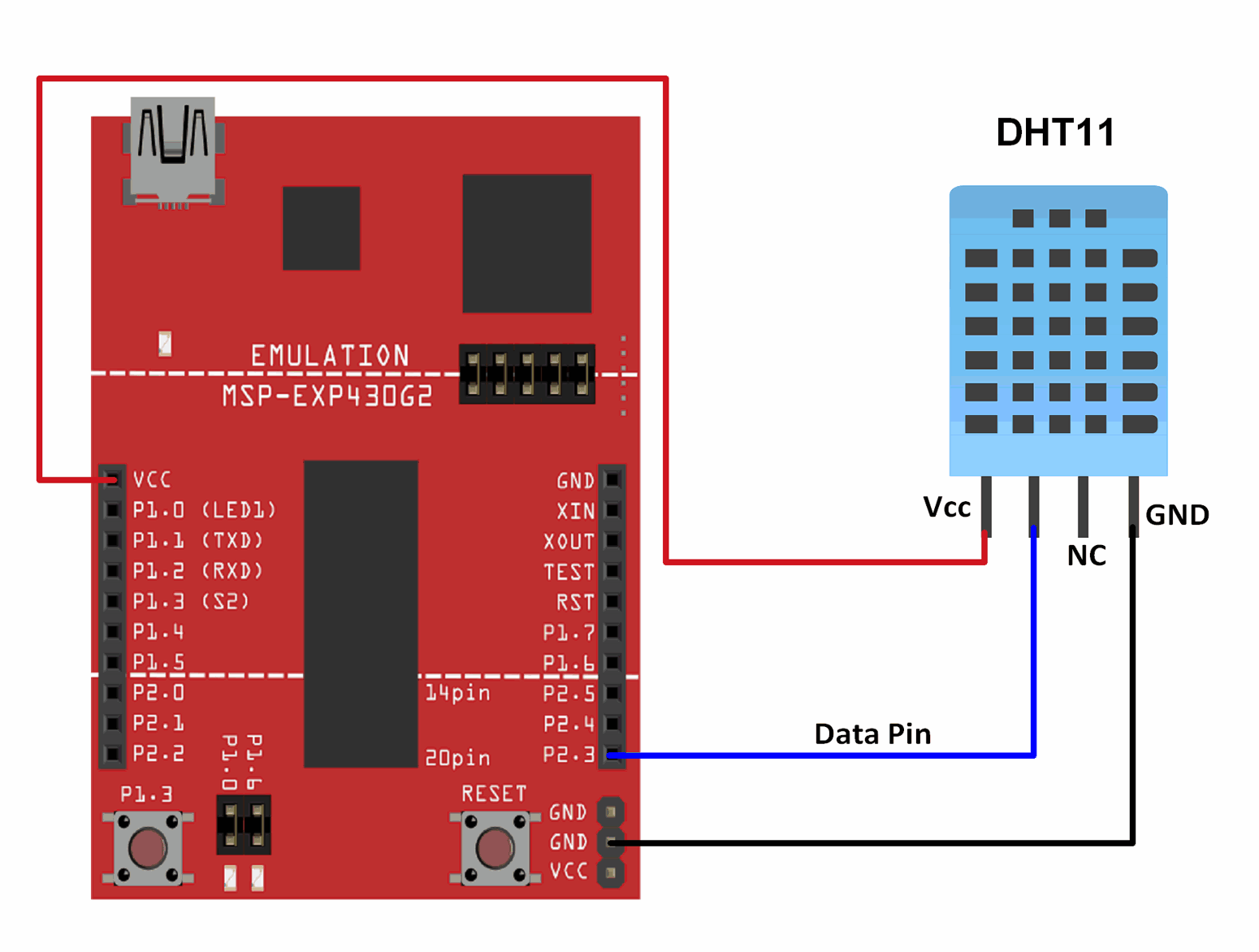
Read temperature and humidity from the DHT11 sensor using MSP-EXP430G2 TI Launchpad
Here, we will be using the DHT11 library by Adafruit that is available GitHub.
Download this library from here.
Extract the library and add the folder to the libraries folder path of Energia IDE.
This library is designed to work with the Adafruit Unified Sensor library.
Download the Unified Sensor library from here.
Extract the file named Adafruit_Sensors.h and add it to the DHT11 library folder that was added to the libraries as told above.
For information about how to add a custom library to the Energia IDE and use examples from it, refer to Adding Library To Energia IDE in the Basics section.
We have modified and used the DHT_Unified_Sensor example sketch according to our requirements.
Word of Caution: In order for the library mentioned above to work in Energia IDE, we need to change the value of float variable f in the definition of read temperature and read humidity functions, in the DHT.cpp file in the library, from NAN to 0 (zero). The links above will give you the original library files. We have provided the modified library and sketch for Energia IDE in the attachments and source code section given below.
Tread Carefully: MSP-EXP430G2 TI Launchpad board has a RAM of 512 bytes which is easily filled, especially while using different libraries. There are times when you need the Serial buffer to be large enough to contain the data you want and you will have to modify the buffer size for the Serial library. While doing such things, we must ensure that the code does not utilize more than 70% RAM. This could lead to the code working in an erratic manner, working well at times, and failing miserably at others.
There are times when the RAM usage may exceed 70% and the codes will work absolutely fine, and times when the code will not work even when the RAM usage is 65%.
In such cases, a bit of trial and error with the buffer size and/or variables may be necessary.
DHT11 code for MSP-EXP430G2 TI Launchpad
// DHT Temperature & Humidity Sensor
// Unified Sensor Library Example
// Written by Tony DiCola for Adafruit Industries
// Released under an MIT license.
// Depends on the following Arduino libraries:
// - Adafruit Unified Sensor Library: https://github.com/adafruit/Adafruit_Sensor
// - DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library
#include <Adafruit_Sensor.h>
#include <DHT.h>
#include <DHT_U.h>
#define DHTPIN 11 // Pin which is connected to the DHT sensor.
// Uncomment the type of sensor in use:
#define DHTTYPE DHT11 // DHT 11
//#define DHTTYPE DHT22 // DHT 22 (AM2302)
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
// See guide for details on sensor wiring and usage:
// https://learn.adafruit.com/dht/overview
DHT_Unified dht(DHTPIN, DHTTYPE);
uint32_t delayMS;
void setup() {
Serial.begin(9600);
// Initialize device.
dht.begin();
Serial.println("DHTxx Unified Sensor Example");
// Print temperature sensor details.
sensor_t sensor;
dht.temperature().getSensor(&sensor);
Serial.println("------------------------------------");
Serial.println("Temperature");
Serial.print ("Sensor: "); Serial.println(sensor.name);
Serial.print ("Driver Ver: "); Serial.println(sensor.version);
Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id);
Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" *C");
Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" *C");
Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" *C");
Serial.println("------------------------------------");
// Print humidity sensor details.
dht.humidity().getSensor(&sensor);
Serial.println("------------------------------------");
Serial.println("Humidity");
Serial.print ("Sensor: "); Serial.println(sensor.name);
Serial.print ("Driver Ver: "); Serial.println(sensor.version);
Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id);
Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println("%");
Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println("%");
Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println("%");
Serial.println("------------------------------------");
// Set delay between sensor readings based on sensor details.
delayMS = sensor.min_delay / 1000;
}
void loop() {
delay(delayMS);
sensors_event_t event;
dht.temperature().getEvent(&event); /* Read temperature value */
if (isnan(event.temperature)) { /* If temperature value is not a number */
Serial.println("Error reading temperature!");
}
else {
Serial.print("Temperature : ");
Serial.print(event.temperature);
Serial.println(" *C");
}
dht.humidity().getEvent(&event); /* Read humidity value */
if (isnan(event.relative_humidity)) { /* If humidity value is not a number */
Serial.println("Error reading humidity!");
}
else {
Serial.print("Humidity : ");
Serial.print(event.relative_humidity);
Serial.println("%");
}
}
Components Used |
||
---|---|---|
TI Launchpad MSP-EXP430G2 TI Launchpad MSP-EXP430G2 |
X 1 | |
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
Breadboard Breadboard |
X 1 |