Overview of DHT11
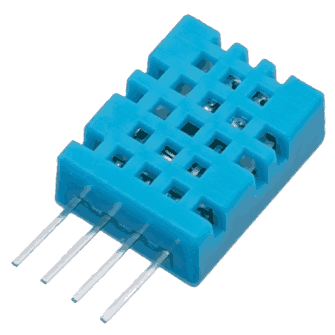
The DHT11 is a basic, low cost digital temperature and humidity sensor.
- DHT11 is a single wire digital humidity and temperature sensor, which provides humidity and temperature values serially with one-wire protocol.
- DHT11 sensor provides relative humidity value in percentage (20 to 90% RH) and temperature values in degree Celsius (0 to 50 °C).
- DHT11 sensor uses resistive humidity measurement component, and NTC temperature measurement component.
DHT11 Pinout:
- DHT11 is a 4-pin sensor, these pins are VCC, DATA, GND and one pin is not in use shown in fig below.
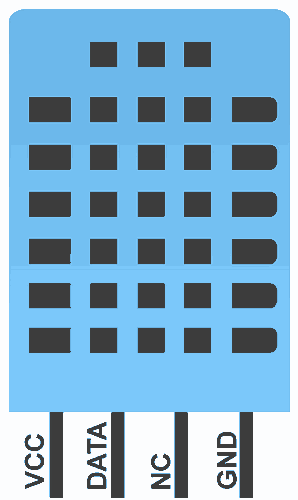
Pin Description:
Pin No. | Pin Name | Pin Description |
1 | VCC | Power supply 3.3 to 5.5 Volt DC |
2 | DATA | Digital output pin |
3 | NC | Not in use |
4 | GND | Ground |
Specification of the DHT11 sensor
- Power supply: 3.3 to 5V DC
- Current consumption: max 2.5mA
- Operating range: 20-80% RH, 0-50°C
- Humidity measurement range: 20-90% RH
- Humidity measurement accuracy: ±5% RH
- Temperature measurement range: 0-50°C
- Temperature measurement accuracy: ±2°C
- Response time: 1s
- Sampling rate: 1Hz (1 sample per second)
- Data output format: single-bus digital signal
- Data transmission distance: 20-30m (at open air)
- Dimensions: 15mm x 12mm x 5.5mm
- Weight: 2.5g
- Digital signal transmission protocol: 1 start signal + 40bit data + 1 checksum
Communication with Microcontroller
- DHT11 uses only one wire for communication. The voltage levels with certain time value defines the logic one or logic zero on this pin.
- The communication process is divided in three steps, first is to send request to DHT11 sensor then sensor will send response pulse and then it starts sending data of total 40 bits to the microcontroller.
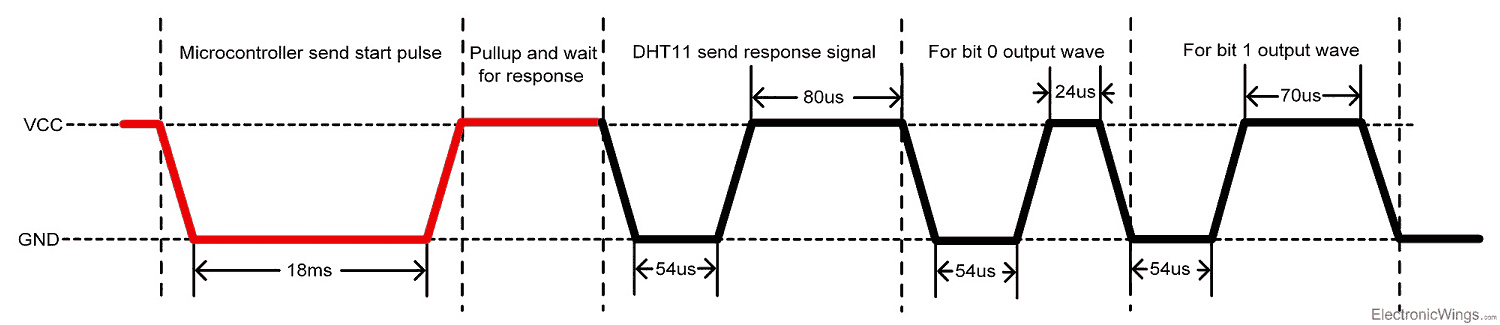
Start pulse (Request)
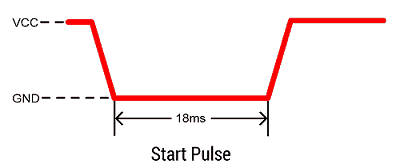
- To start communication with DHT11, first we should send the start pulse to the DHT11 sensor.
- To provide start pulse, pull down (low) the data pin minimum 18ms and then pull up, as shown in diag.
Response
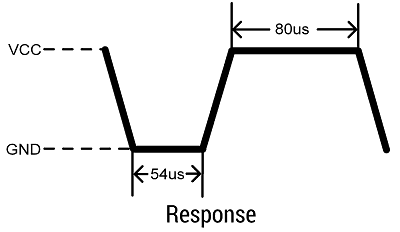
- After getting start pulse from, DHT11 sensor sends the response pulse which indicates that DHT11 received start pulse.
- The response pulse is low for 54us and then goes high for 80us.
Data
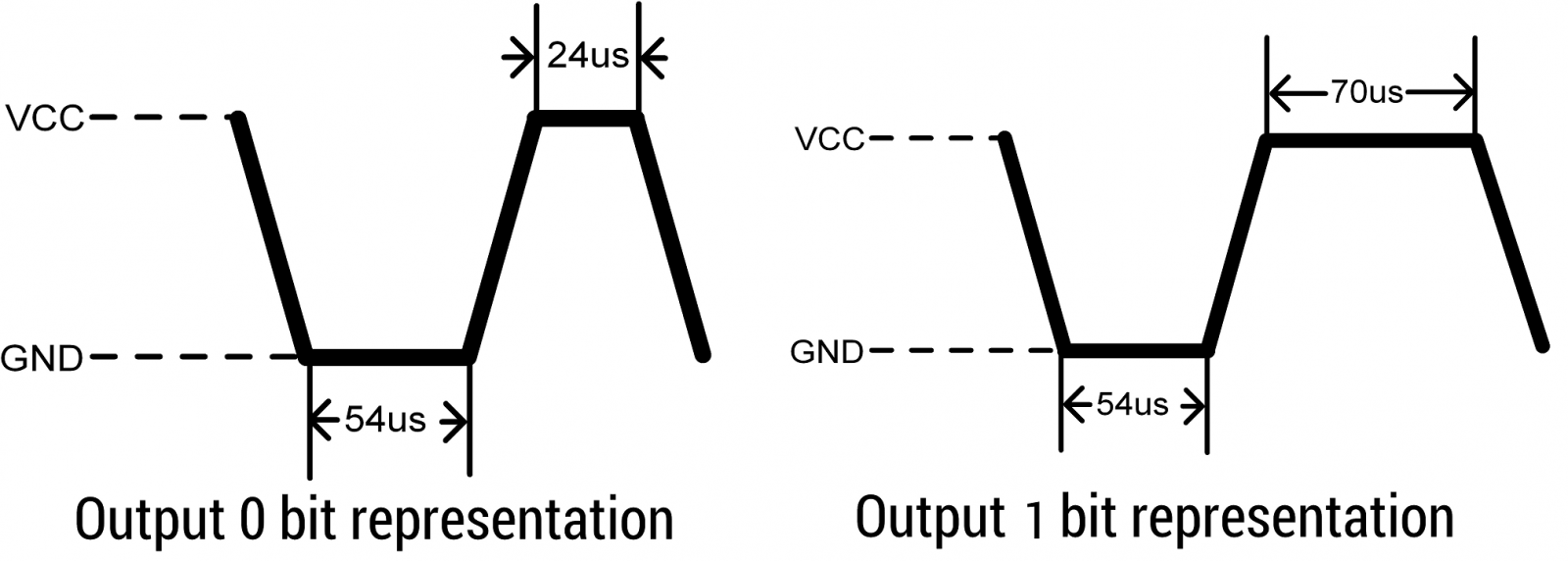
- After sending the response pulse, DHT11 sensor sends the data, which contains humidity and temperature value along with checksum.
- The data frame is of total 40 bits long, it contains 5 segments (byte) and each segment is 8-bit long.
- In these 5 segments, first two segments contain humidity value in decimal integer form. This value gives us Relative Percentage Humidity. 1st 8-bits are integer part and next 8 bits are fractional part.
- Next two segments contain temperature value in decimal integer form. This value gives us temperature in Celsius form.
- Last segment is the checksum which holds checksum of first four segments.
- Here checksum byte is direct addition of humidity and temperature value. And we can verify it, whether it is same as checksum value or not. If it is not equal, then there is some error in the received data.
- Once data received, DHT11 pin goes in low power consumption mode till next start pulse.
End of frame
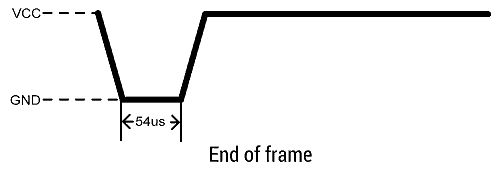
- After sending 40-bit data, DHT11 sensor sends 54us low level and then goes high. After this DHT11 goes in sleep mode.
DHT11 vs DHT22
Two versions of the DHT sensor, they look a bit similar and have the same pinout, but have different characteristics and specifications:
- Ultra-low cost
- 3 to 5V power and I/O
- 2.5mA max current use during conversion (while requesting data)
- Good for 20-80% humidity readings with 5% accuracy
- Good for 0-50°C temperature readings ±2°C accuracy
- No more than 1 Hz sampling rate (once every second)
- Body size 15.5mm x 12mm x 5.5mm
- 4 pins with 0.1" spacing
- Low cost
- 3 to 5V power and I/O
- 2.5mA max current use during conversion (while requesting data)
- Good for 0-100% humidity readings with 2-5% accuracy
- Good for -40 to 125°C temperature readings ±0.5°C accuracy
- No more than 0.5 Hz sampling rate (once every 2 seconds)
- Body size 15.1mm x 25mm x 7.7mm
- 4 pins with 0.1" spacing
Alternate options for DHT11
- DHT22 or AM2302
- SHT71
DHT11 Interfacing With Arduino
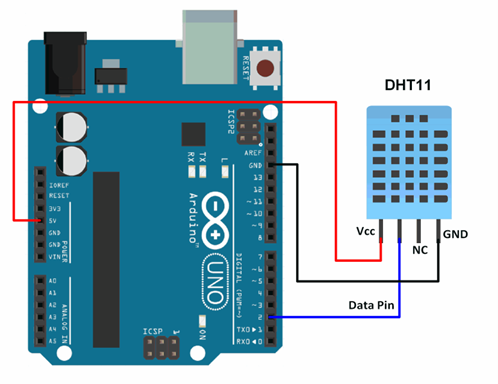
Temperature and Humidity Measurement Code using DHT11 for Arduino
#include"DHT.h"
DHT dht;
voidsetup()
{
Serial.begin(9600);
Serial.println();
Serial.println("Status\tHumidity (%)\tTemperature (C)\t(F)");
dht.setup(2); /* set pin for data communication */
}
voidloop()
{
delay(dht.getMinimumSamplingPeriod()); /* Delay of amount equal to sampling period */
float humidity = dht.getHumidity(); /* Get humidity value */
float temperature = dht.getTemperature(); /* Get temperature value */
Serial.print(dht.getStatusString()); /* Print status of communication */
Serial.print("\t");
Serial.print(humidity, 1);
Serial.print("\t\t");
Serial.print(temperature, 1);
Serial.print("\t\t");
Serial.println(dht.toFahrenheit(temperature), 1); /* Convert temperature to Fahrenheit units */
}
The output will display the status of communication, humidity (%), the temperature in Celsius, and temperature in Fahrenheit. The humidity and temperature values will be displayed with one decimal point precision on the serial monitor.
To know more about DHT11 Sensor using Arduino refer to this link
Examples of DHT11 interfacing
Components Used |
||
---|---|---|
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
DHT-22 sensor DHT-22 sensor |
X 1 |