Introduction
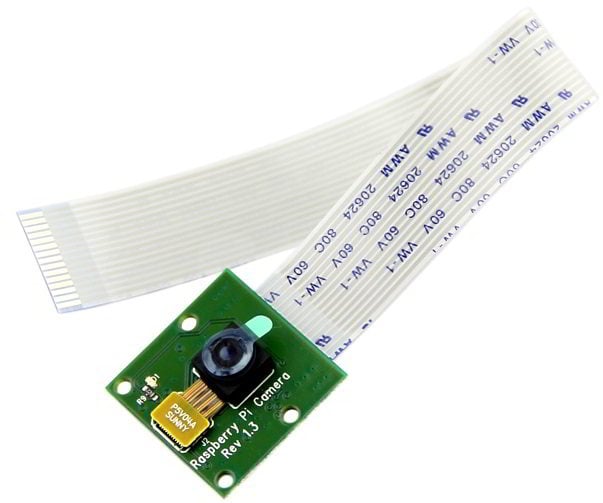
The pi Camera module is a camera that can be used to take pictures and high definition video.
Raspberry Pi Board has CSI (Camera Serial Interface) interface to which we can attach the PiCamera module directly.
This Pi Camera module can attach to the Raspberry Pi’s CSI port using a 15-pin ribbon cable.
Features of Pi Camera
Here, we have used Pi camera v1.3. Its features are listed below,
- Resolution – 5 MP
- HD Video recording – 1080p @30fps, 720p @60fps, 960p @45fps and so on.
- It Can capture wide, still (motionless) images of a resolution 2592x1944 pixels
- CSI Interface enabled.
How to attach Pi Camera to Raspberry Pi?
Connect Pi Camera to the CSI interface of the Raspberry Pi board as shown below,
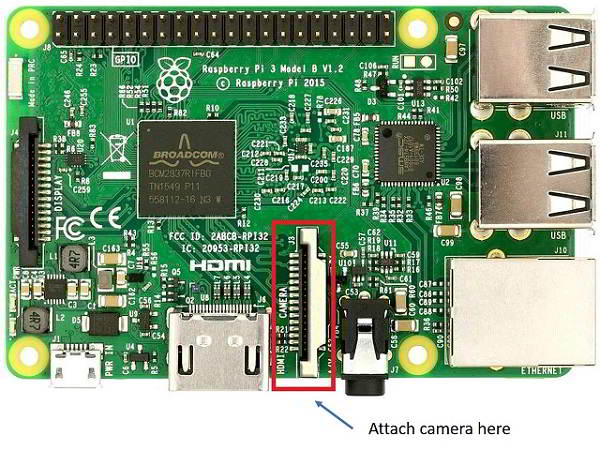
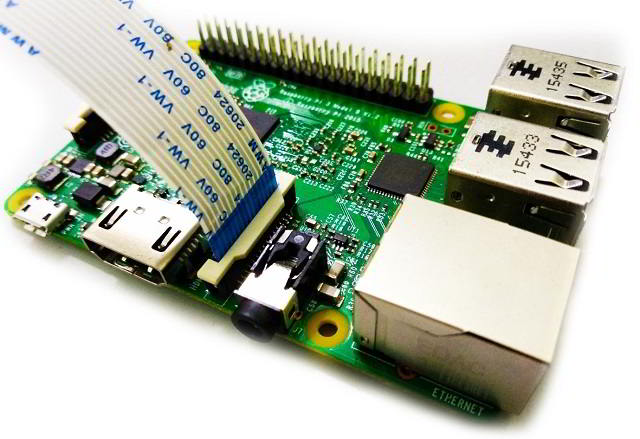
Now, we can use Pi Camera for capturing images and videos using Raspberry Pi.
Before using Pi Camera, we need to enable camera for its working.
How to Enable Camera functionality on Raspberry Pi
For enabling the camera in Raspberry Pi, open the raspberry pi configuration using the following command,
sudo raspi-config
then select Interfacing options in which select the camera option to enable its functionality.
reboot Raspberry Pi.
Now we can access the camera on Raspberry Pi.
Now we can capture images and videos using Pi Camera on Raspberry Pi.
Example
Capture images and save it to the specified directory.
We can capture images using Python. Here, we will write a Python program to capture images using Pi Camera on Raspberry Pi.
Here, we have used picamera
package(library) which provides different classes for Raspberry Pi. Out of which we are mainly interested in PiCamera
class which is for camera module.
Pi Camera Python Program for Image Capture
'''
capture images on Raspberry Pi using Pi Camera
http://www.electronicwings.com
'''
import picamera
from time import sleep
#create object for PiCamera class
camera = picamera.PiCamera()
#set resolution
camera.resolution = (1024, 768)
camera.brightness = 60
camera.start_preview()
#add text on image
camera.annotate_text = 'Hi Pi User'
sleep(5)
#store image
camera.capture('image1.jpeg')
camera.stop_preview()
Functions Used
To use picamera
python based library we have to include it in our program as given below
import picamera
This picamera
library has PiCamera
class for the camera module. So, we have to create an object for PiCamera class.
PiCamera Class
To use Pi Camera in Python on Raspberry Pi, we can use PiCamera class which has different APIs for camera functionality. We need to create object for PiCamera class.
E.g.
Camera = picamera.PiCamera()
The above PiCamera class has different member variables and functions which we can access by simply inserting a dot (.) in between object name and member name.
E.g.
Camera.resolution = (1080, 648)
capture()
It is used to capture images using Pi Camera.
E.g.
Camera.capture(“/home/pi/image.jpeg”)
The capture()
function has different parameters which we can pass for different operations like resize, format, use_video_port, etc.
E.g.
Camera.capture(“/home/pi/image.jpeg”, resize=(720, 480))
resolution= (width,height)
It sets the resolution of the camera at which image captures, video records, and previews will display. The resolution can be specified as (width, height) tuple, as a string formatted WIDTHxHEIGHT, or as a string containing commonly recognized display resolution names e.g. “HD”, “VGA”, “1080p”, etc.
E.g.
Camera.resolution = (720, 480)
Camera.resolution = “720 x 480”
Camera.resolution = “720p”
Camera.resolution = “HD”
Annotate_text = “Text”
It is used to add text on images, videos, etc.
E.g.
Camera.annotate_text = “Hi Pi User”
start_preview()
It displays the preview overlay of the default or specified resolution.
E.g.
Camera.start_preview()
stop_preview()
It is used to close the preview overlay.
E.g.
Camera.stop_preview()
Note: There are various APIs of PiCamera class. So, to know more API in detail you can refer
Pi Camera Python Program for Video Recording
'''
Record video on Raspberry Pi using pi Camera
http://www.electronicwings.com
'''
import picamera
from time import sleep
camera = picamera.PiCamera()
camera.resolution = (640, 480)
print()
#start recording using pi camera
camera.start_recording("/home/pi/demo.h264")
#wait for video to record
camera.wait_recording(20)
#stop recording
camera.stop_recording()
camera.close()
print("video recording stopped")
Functions used
We have to create an object for PiCamera class. Here, we have create objects as camera.
start_recording()
It is used to start video recording and store it.
E.g.
Camera.start_recording(‘demo.h264’)
It records video named demo of h264 format.
wait_recording(timeout)
Wait on the video encoder for specified timeout seconds.
E.g.
Camera.wait_recording(60)
stop_recording()
It is used to stop video recording.
E.g.
Camera.stop_recording()
Play Recorded Video
To open a video, we can use omxplayer by using the following command,
omxplayer video_name
Components Used |
||
---|---|---|
Raspberry Pi 4B Raspberry Pi 4BRaspberry Pi 4B |
X 1 | |
Raspberry Pi Camera V2 Raspberry Pi Camera V2 |
X 1 |