Overview of Nokia5110 Display
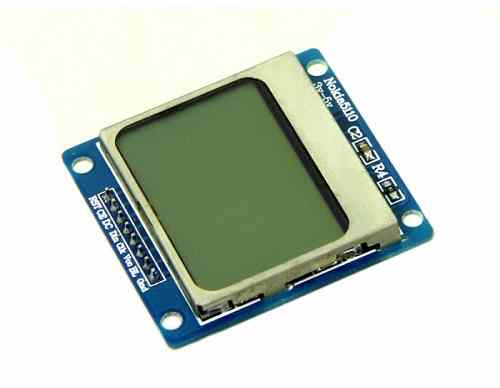
Nokia5110 is a graphical display that can display text, images, and various patterns.
It has a resolution of 48x84 and comes with a backlight.
It uses SPI communication to communicate with a microcontroller/microprocessor.
Data and commands can be sent through the processor to the display to control the display output.
It has 8 pins.
For more information about the Nokia5110 display and how to use it, refer the topic Nokia5110 Graphical Display in the sensors and modules section.
As Nokia5110 uses SPI communication, we need to make sure that the SPI interface of Raspberry Pi is enabled. If it is not enabled then using we have to make it enable.
How to enable SPI?
To enable SPI on Raspberry Pi, enter the following command
sudo raspi-config
then, a window will pop up in which select Interface Options
After selecting the Interface option, enable the SPI interface. And then reboot Raspberry Pi.
Now, we can establish SPI communication.
Connection Diagram of Nokia5110 Display with Raspberry Pi
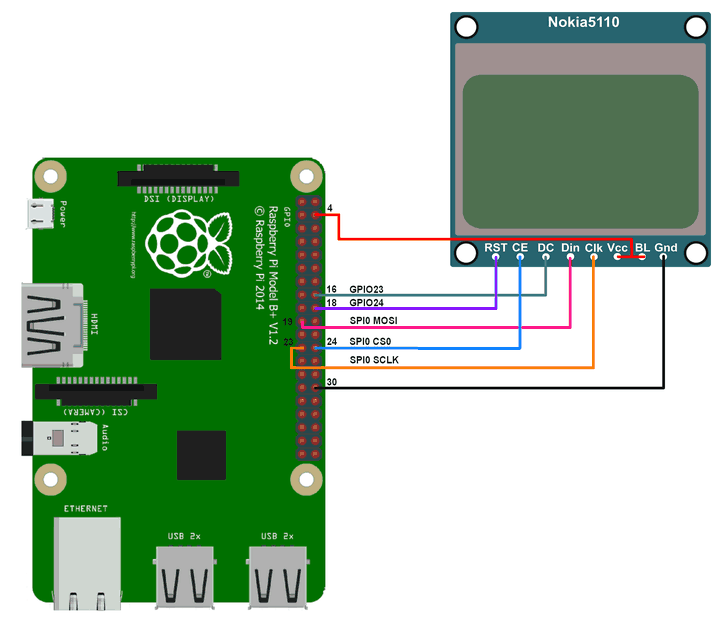
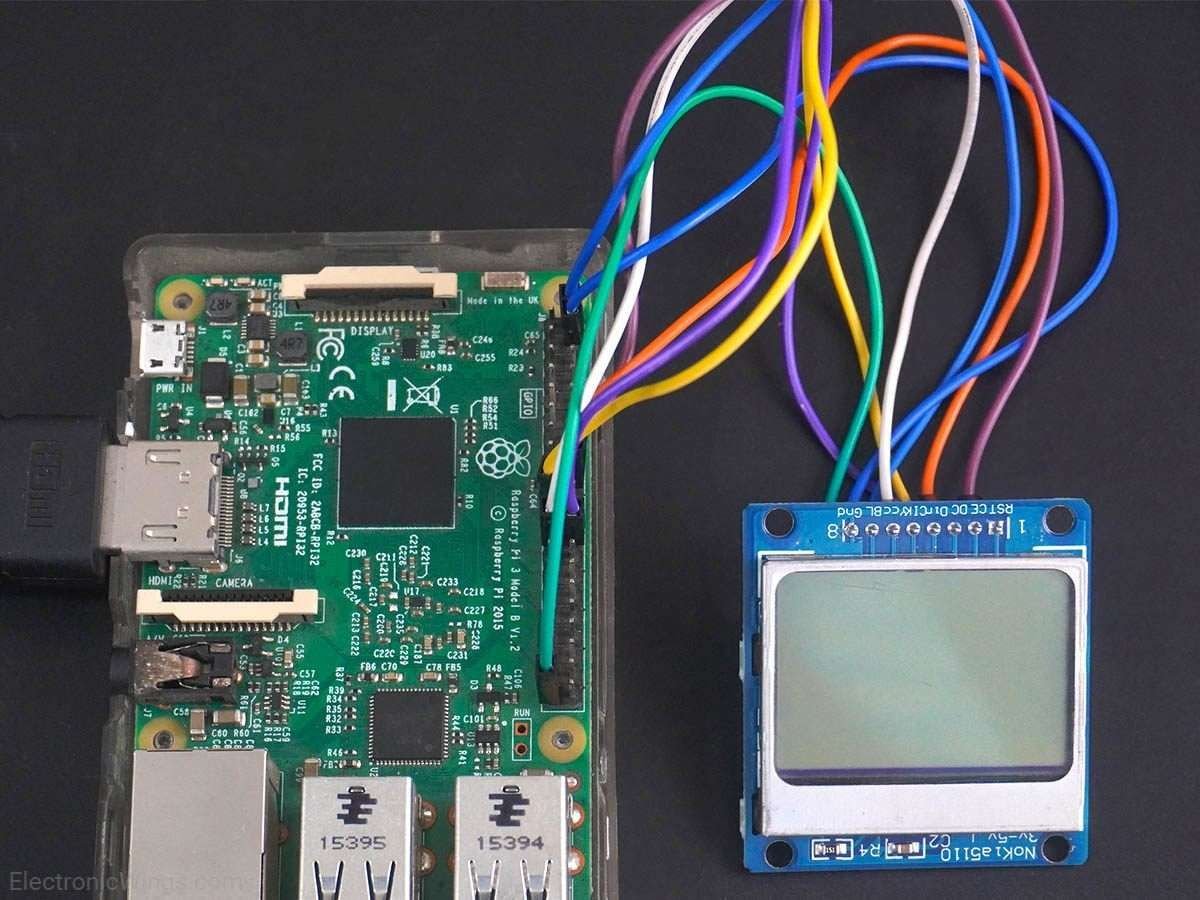
Display Text and Images on Nokia5110 Display using Raspberry Pi
Here, we are going to interface the Nokia5110 display with Raspberry Pi 3
We will be using the theNokia5110 Python library by Adafruit from GitHub.
Download the Adafruit Nokia LCD Python library.
Extract the above library and install it in the directory of the above library by executing the following command,
sudo python setup.py install
Also, we need to install python imaging library as given below,
sudo apt-get install python-imaging
Once the library has been added, we can test the Nokia LCD interfacing with Raspberry Pi by executing existing examples kept in examples
folder of the library.
Here, we are going to create a small python program in which we will display text, image,s and flying bird animation. This python program file along with sample images is kept in the “example” folder of the library provided in the attachment.
Note: While displaying image on Nokia5110 display, we have to provide image to the program. This image should be of display size (84x48 here). If we provide image with other resolution it will give error.
Nokia5110 Python Program for Raspberry Pi
'''
Nokia5110 LCD Display Interface with Raspberry Pi
http://www.electronicwings.com
'''
import time
import Adafruit_Nokia_LCD as LCD
import Adafruit_GPIO.SPI as SPI
from PIL import ImageDraw
from PIL import Image
from PIL import ImageFont
# Raspberry Pi hardware SPI config:
DC = 23
RST = 24
SPI_PORT = 0
SPI_DEVICE = 0
# Hardware SPI usage:
disp = LCD.PCD8544(DC, RST, spi=SPI.SpiDev(SPI_PORT, SPI_DEVICE, max_speed_hz=4000000))
# Initialize library.
disp.begin(contrast=40)
# Clear display.
disp.clear()
disp.display()
font = ImageFont.load_default()
# Load image and convert to 1 bit color.
image = Image.new('1', (LCD.LCDWIDTH, LCD.LCDHEIGHT))
draw = ImageDraw.Draw(image)
draw.rectangle((0,0,LCD.LCDWIDTH,LCD.LCDHEIGHT), outline=255, fill=255)
draw.text((3,24), 'Welcome to EW', font=font)
#Display Image
disp.image(image)
disp.display()
time.sleep(1.0)
image = Image.open('pi_logo.png').convert('1')
# Display image.
disp.image(image)
disp.display()
time.sleep(0.5)
print('Press Ctrl-C to quit.')
while True:
#Create animation of Flying Bird
image2 = Image.open('bird_1.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_2.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_3.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_4.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_5.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
image2 = Image.open('bird_4.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_3.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
image2 = Image.open('bird_2.png').convert('1')
# Display image.
disp.image(image2)
disp.display()
time.sleep(0.1)
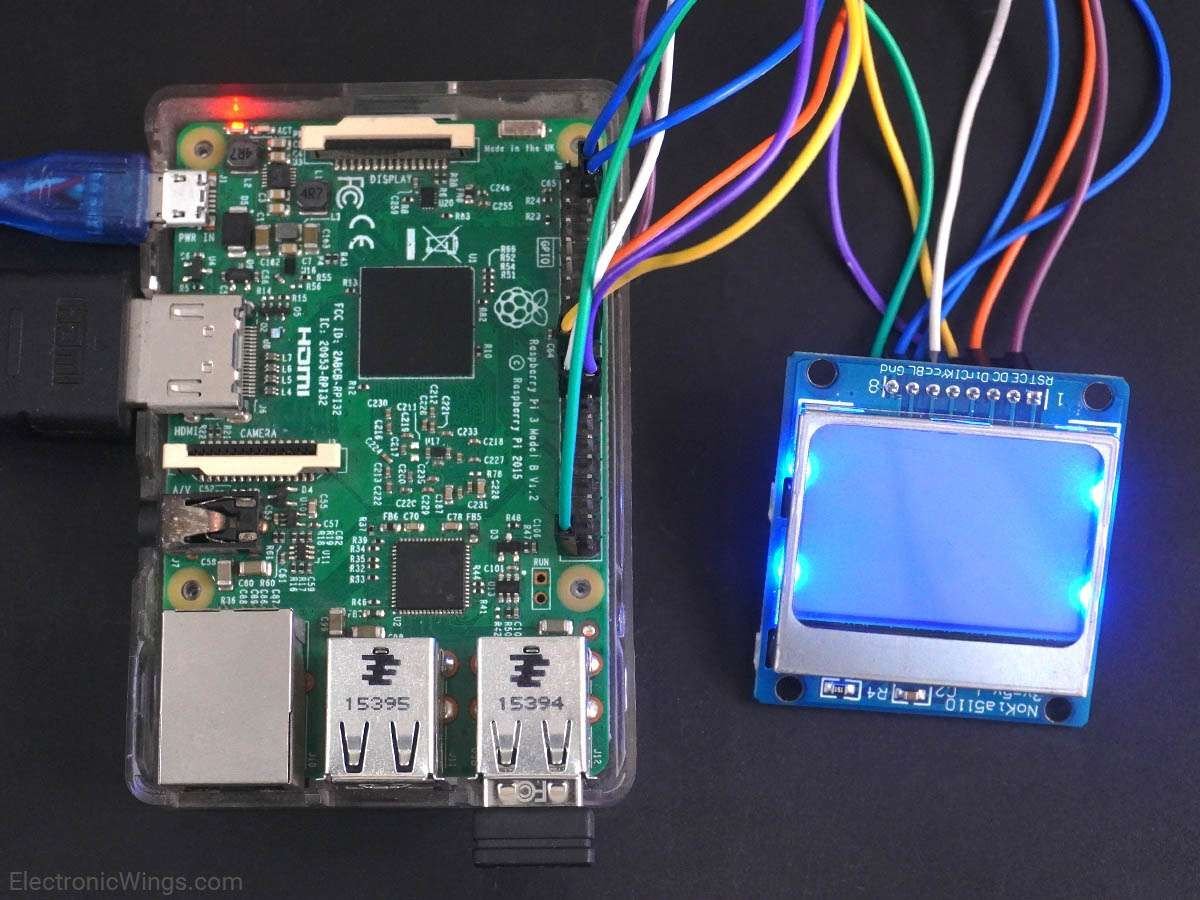
Output Video of Nokia5110 Display using Raspberry Pi
Components Used |
||
---|---|---|
Nokia5110 Graphical Display Nokia5110 is 48x84 dot LCD display with Serial Peripheral Interface (SPI) Connectivity. It was designed for cell phones. It is also used in embedded applications. |
X 1 | |
Raspberry Pi 4B Raspberry Pi 4BRaspberry Pi 4B |
X 1 | |
Raspberry Pi Zero Raspberry Pi Zero |
X 1 |