Overview of DHT11 Sensor
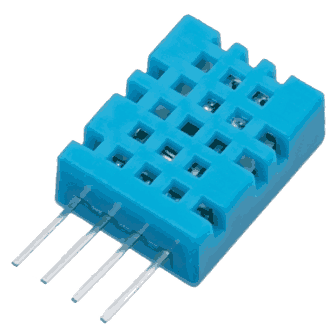
- DHT11 sensor measures and provides humidity and temperature values serially over a single wire.
- It can measure relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
- It has 4 pins; one of which is used for data communication in serial form.
- Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of a frame.
For more information about the DHT11 sensor and how to use it, refer the topic DHT11 sensor in the sensors and modules topic.
Connection Diagram of DHT11 with Raspberry Pi
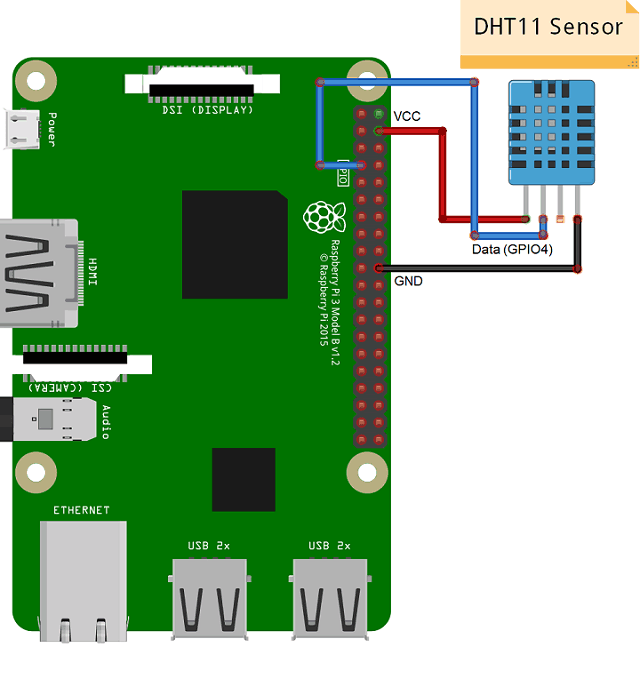
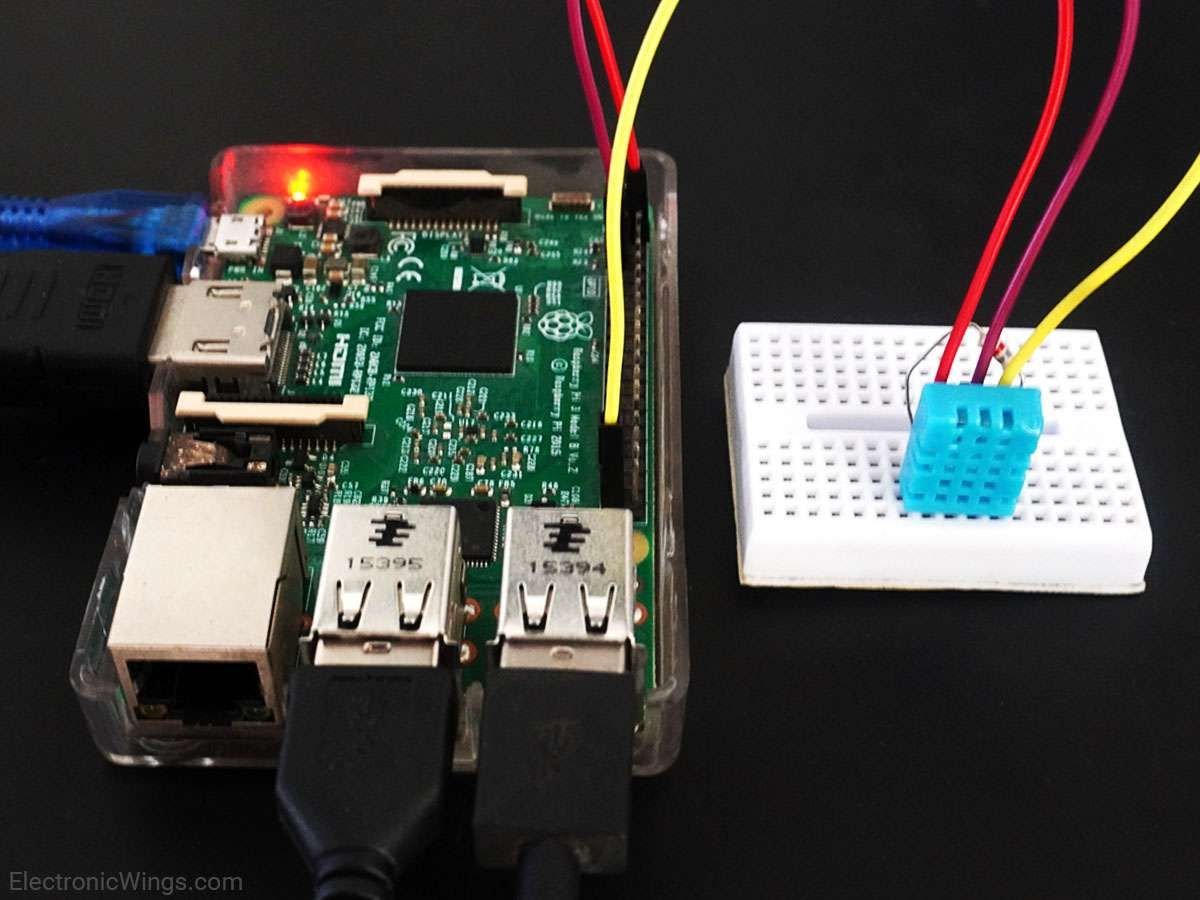
Read Temperature and Humidity using DHT11 and Raspberry Pi
- Here, we are going to interface the DHT11 sensor with Raspberry Pi 3 and display Humidity and Temperature on the terminal.
- We will be using the DHT Sensor Python library by Adafruit from GitHub. The Adafruit Python DHT Sensor library is created to read the Humidity and Temperature on Raspberry Pi or Beaglebone Black. It is developed for DHT series sensors like DHT11, DHT22, or AM2302.
Download Adafruit DHT Sensor library from here.
- Extract the library and install it in the same root directory of the downloaded library by executing the following command,
sudo python setup.py install
- Once the library and its dependencies have been installed, open the example sketch named simpletest from the library kept in the examples folder.
- In this code, raspberry Pi reads Humidity and Temperature from the DHT11 sensor and prints them on the terminal. But, it read and displays the value only once. So, here we made a change in the program to print value continuously.
Note:
- Assign proper sensor type to the sensor variable in this library. Here, we are using DHT11 sensor.
sensor = Adafruit_DHT.DHT11
- If anyone is using sensor DHT22 then we need to assign Adafruit_DHT.DHT22 to the sensor variable shown above.
- Also, comment out the Beaglebone pin definition and uncomment pin declaration for Raspberry Pi.
- Then assign pin no. to which the DHT sensor’s data pin is connected. Here, data out of the DHT11 sensor is connected to GPIO4. As shown in the above interfacing diagram.
DHT11 Python Program for Raspberry Pi
#!/usr/bin/python
# Copyright (c) 2014 Adafruit Industries
# Author: Tony DiCola
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
import Adafruit_DHT
# Sensor should be set to Adafruit_DHT.DHT11,
# Adafruit_DHT.DHT22, or Adafruit_DHT.AM2302.
sensor = Adafruit_DHT.DHT11
# Example using a Beaglebone Black with DHT sensor
# connected to pin P8_11.
#pin = 'P8_11'
# Example using a Raspberry Pi with DHT sensor
# connected to GPIO4.
pin = 4
# Try to grab a sensor reading. Use the read_retry method which will retry up
# to 15 times to get a sensor reading (waiting 2 seconds between each retry).
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
print('Temp={0:0.1f}*C Humidity={1:0.1f}%'.format(temperature, humidity))
'''
# Note that sometimes you won't get a reading and
# the results will be null (because Linux can't
# guarantee the timing of calls to read the sensor).
# If this happens try again!
if humidity is not None and temperature is not None:
print('Temp={0:0.1f}*C Humidity={1:0.1f}%'.format(temperature, humidity))
else:
print('Failed to get reading. Try again!')
'''
.jpg)
DHT11 Output
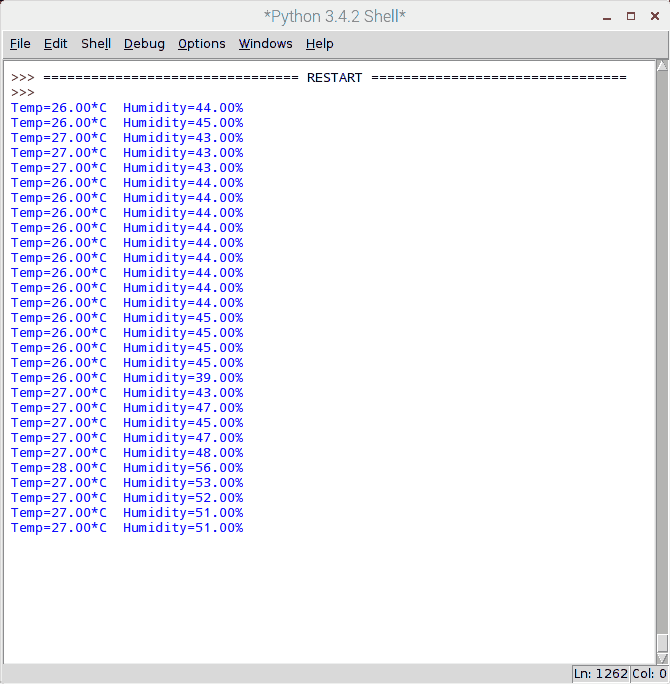
Components Used |
||
---|---|---|
Raspberry Pi 4B Raspberry Pi 4BRaspberry Pi 4B |
X 1 | |
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
Raspberry Pi Zero Raspberry Pi Zero |
X 1 |
Downloads |
||
---|---|---|
|
Adafruit Python DHT library | Download |